Avoiding Common Pitfalls When Injecting Collections in Spring
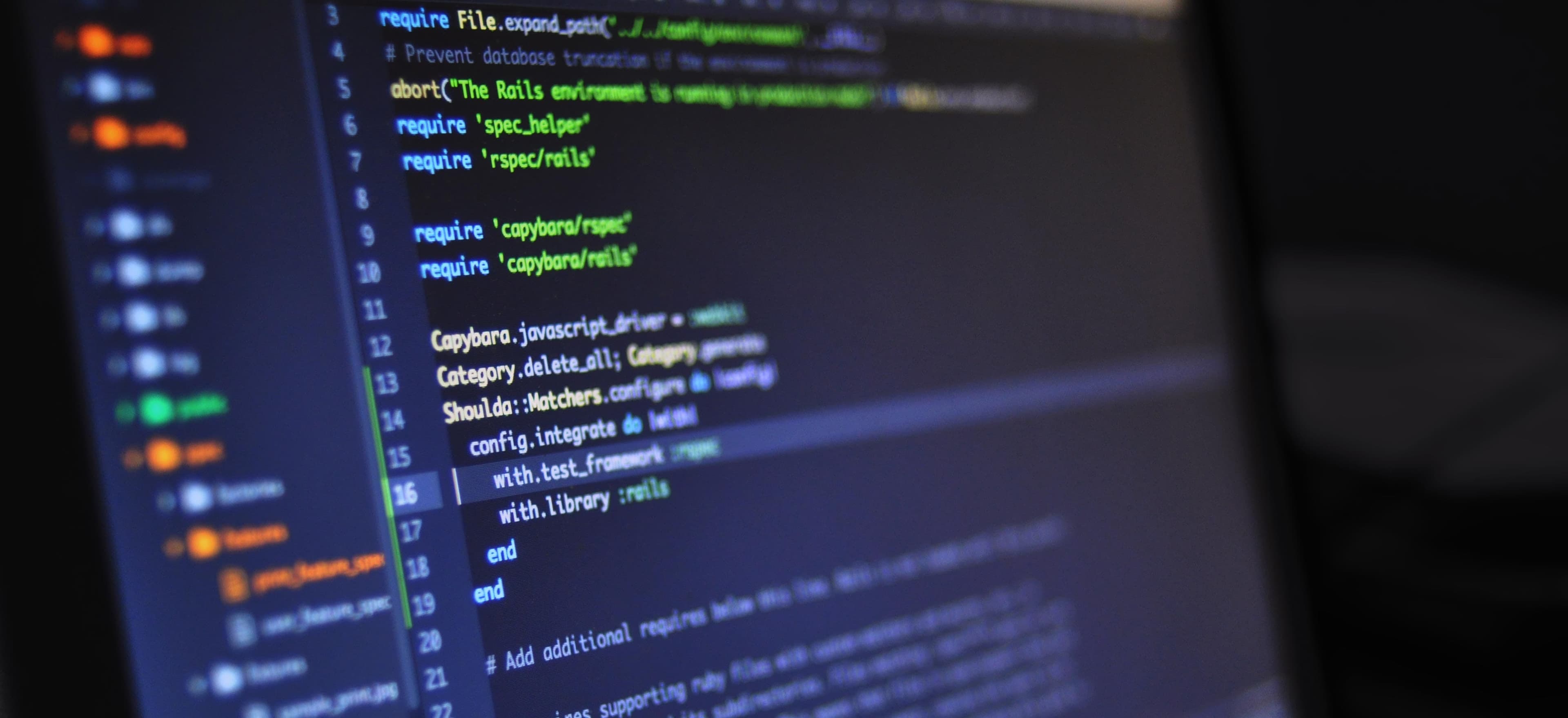
- Published on
Avoiding Common Pitfalls When Injecting Collections in Spring
Dependency injection is a core feature of the Spring Framework that simplifies the development of Java applications. Among the various types of dependencies, collections can sometimes be tricky to handle correctly. This blog post aims to guide you through the common pitfalls associated with injecting collections in Spring, providing best practices through illustrative code snippets.
Table of Contents
- Understanding Dependency Injection in Spring
- Common Pitfalls
- 2.1 Failing to Autowire Collections
- 2.2 Types of Collections
- 2.3 Initialization of Collections
- Best Practices for Injecting Collections
- Conclusion
1. Understanding Dependency Injection in Spring
Dependency Injection (DI) is a design pattern that allows the Spring container to manage the instantiation and lifecycle of objects in an application. By using DI, you can focus on the business logic rather than the complexities of object creation. This is particularly beneficial when dealing with collections, such as lists and maps, that need to hold multiple dependencies.
Here's a simple example of using Spring's @Autowired
annotation to inject a list of beans:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.List;
@Component
public class VehicleService {
private final List<Vehicle> vehicles;
@Autowired
public VehicleService(List<Vehicle> vehicles) {
this.vehicles = vehicles;
}
public void serviceVehicles() {
for (Vehicle vehicle : vehicles) {
vehicle.service();
}
}
}
In this example, the VehicleService
class is dependent on a collection of Vehicle
beans. Spring's DI takes care of injecting the appropriate list of Vehicle
objects when it initializes VehicleService
.
2. Common Pitfalls
While injecting collections in Spring is generally straightforward, there are several pitfalls to watch out for:
2.1 Failing to Autowire Collections
One of the most common mistakes is forgetting to autowire a collection. If you don’t configure the injection correctly, you could end up with null values or throw a runtime exception.
Consider this incorrect example:
@Component
public class VehicleService {
private List<Vehicle> vehicles; // No @Autowired annotation
public VehicleService(List<Vehicle> vehicles) {
// vehicles will be null here
this.vehicles = vehicles;
}
}
Why This Matters:
Failing to use @Autowired
means Spring won't recognize this dependency and won't initialize it, leading to a NullPointerException
or empty collection when accessed.
2.2 Types of Collections
Not all collections work the same way in Spring. Lists, sets, and maps each have specific behaviors, especially when it comes to managing duplicates.
For instance:
- A
List
respects the order of elements and allows duplicates. - A
Set
does not allow duplicates. - A
Map
can hold key-value pairs but requires keys to be unique.
Here's an example of using a set:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.Set;
@Component
public class NotificationService {
private final Set<Notifier> notifiers;
@Autowired
public NotificationService(Set<Notifier> notifiers) {
this.notifiers = notifiers;
}
public void sendNotification(String message) {
for (Notifier notifier : notifiers) {
notifier.send(message);
}
}
}
Why This Matters: Choosing the wrong type of collection can lead to unexpected behaviors in your application. Always understand how the standard collection types in Java behave before injecting them.
2.3 Initialization of Collections
Another common pitfall comes from assuming that a collection will always be initialized. For instance, if there are no beans of a particular type defined, the injected collection may end up being empty.
An important example to note is when injecting lists. Here’s how to safely initialize them:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.util.Collections;
import java.util.List;
@Component
public class UserService {
private final List<User> users;
@Autowired
public UserService(List<User> users) {
this.users = users != null ? users : Collections.emptyList(); // Safe initialization
}
public void processUsers() {
for (User user : users) {
// Process each user
}
}
}
Why This Matters:
By ensuring that your collection is initialized safely, you avoid NullPointerException
and allow your application to run smoothly even when no beans are present.
3. Best Practices for Injecting Collections
3.1 Use the Constructor Injection
As demonstrated in the examples, constructor injection is generally preferred over field injection. It makes the dependencies explicit and allows for easier testing.
3.2 Be Clear about Component Scanning
Ensure that all beans you intend to inject are correctly annotated and picked up by component scanning. If your beans are not within the base package or explicitly defined in the configuration, you may encounter issues.
3.3 Handle Empty Collections Gracefully
Using empty collections instead of null helps prevent potential errors:
@Autowired
public UserService(List<User> users) {
this.users = Optional.ofNullable(users).orElse(Collections.emptyList());
}
Using Optional
allows for cleaner handling of potentially null collections.
3.4 Document Dependencies
Regardless of the collection type, documenting what a service expects in terms of dependencies can help maintain clarity and enhance code quality.
4. Conclusion
Injecting collections in Spring is straightforward yet can lead to common pitfalls if mishandled. By understanding the behavior of different collection types, using the appropriate annotations, and following best practices—like constructor injection and safe initialization—you can efficiently manage your dependencies.
Remember, a well-structured application is easier to maintain and scales better in the long run. For further reading on Spring DI, check out the official Spring Documentation.
Feel free to explore more about best practices in Spring and Java development through the related articles on this blog. Happy coding!