Why Java Conversion Puzzles Can Mislead Developers
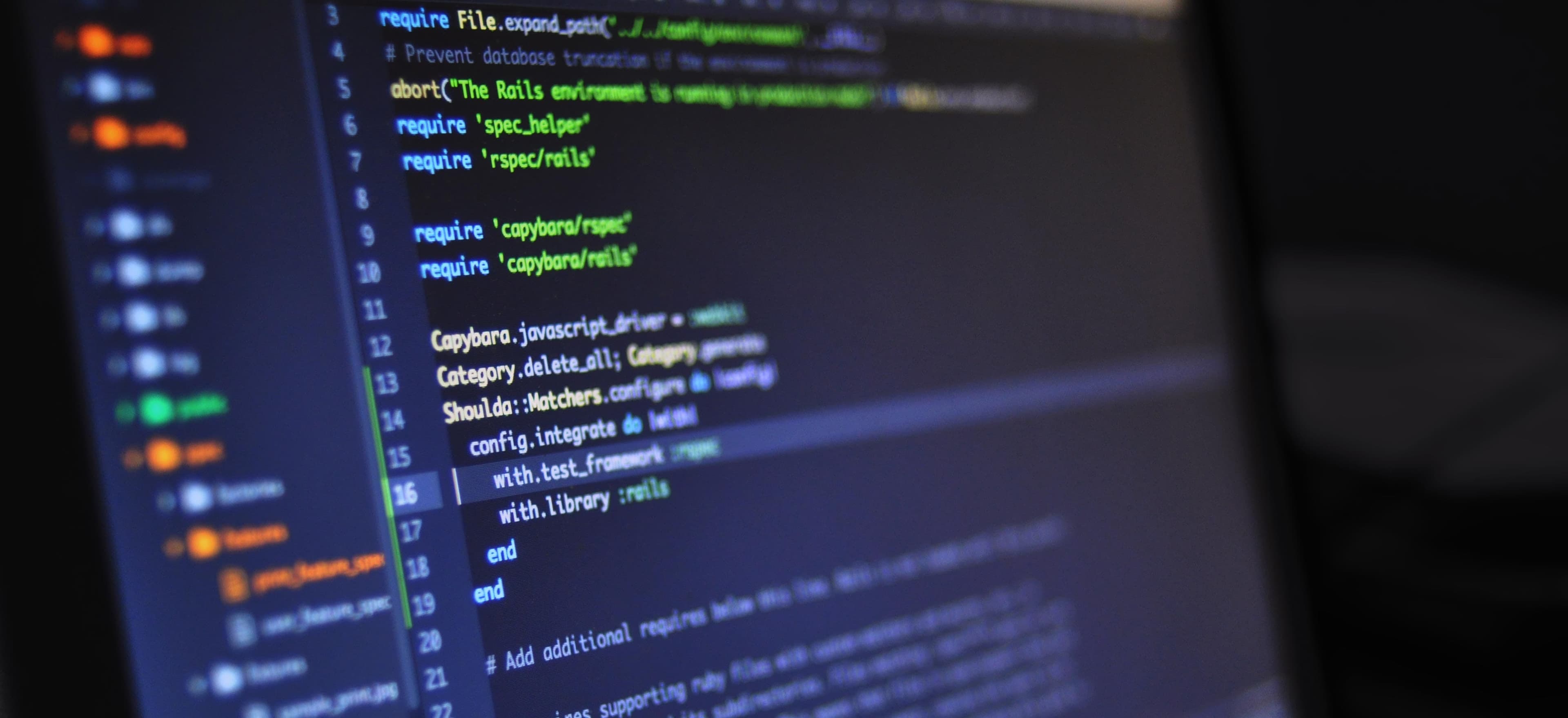
- Published on
Why Java Conversion Puzzles Can Mislead Developers
Every developer has encountered conversion puzzles in Java, often leaving them baffled. These coding scenarios usually involve converting one type of data to another—like from an int to a String or from an Object to a more specific type. While these puzzles can be entertaining brain teasers, they can also mislead developers regarding Java's type system and true behavior. In this blog post, we will discuss various Java conversion puzzles, explain why they can cause confusion, and provide best practices to avoid these pitfalls.
Understanding Type Conversion in Java
Java is a statically typed language, meaning variables must be declared with a specific type. This rigidity helps catch errors at compile time, but it can also lead to some deceptively confusing situations, especially when dealing with type conversion. There are primarily two types of type conversion in Java: implicit (or automatic) conversion and explicit (or manual) conversion.
-
Implicit Conversion: Java does some automatic type conversion for you. For example, when you assign an int to a double, Java will automatically convert the int to a double.
int num = 10; double result = num; // num is implicitly converted to double
-
Explicit Conversion: Sometimes, you need to convert types manually, especially when you might lose precision, like converting a double to an int.
double d = 9.78; int num = (int) d; // Type cast, precision loss occurs.
Understanding these conversions is critical, but some Java puzzles can obscure these fundamentals.
Common Java Conversion Puzzles
Let's dive into some common puzzles and their misconceptions.
Puzzle 1: Integer Overflow
Consider the following code snippet:
int a = Integer.MAX_VALUE;
int b = a + 1;
System.out.println(b);
Expected Output: You might think you'll see 2147483648
, but instead, the output is -2147483648
due to integer overflow.
Why This Happens: When the value exceeds the maximum limit for an int, it wraps around to the negative side of the number line. This can mislead developers into thinking that Java can handle larger integers natively.
Puzzle 2: String vs. Object
Another common confusion arises when dealing with String objects.
String str = "Hello";
Object obj = str; // Implicit conversion from String to Object
if (obj instanceof String) {
String localStr = (String) obj; // Explicit conversion back to String
System.out.println(localStr);
}
Misleading Point: The puzzle can mislead developers about the implicit conversion. They may assume that Objects can't be safely cast back to their original type without runtime errors. However, as demonstrated, if you check its type using instanceof
, the conversion is safe.
Puzzle 3: Wrapping and Unwrapping
When dealing with boxed types, we can encounter strange behaviors:
Integer x = new Integer(1000);
Integer y = 1000;
System.out.println(x == y); // False
System.out.println(x.equals(y)); // True
Why This Happens: The first comparison checks for reference equality, while the second checks for value equality. The two Integer objects have different references even though they represent the same value, causing confusion.
Strategies to Avoid Misleading Conversion Confusion
-
Practice With Real Scenarios: Engage with exercises on type conversion. Websites like LeetCode or HackerRank provide coding challenges that can help clarify these concepts.
-
Use Enums or Constants Instead of Magic Numbers: Using enums can mitigate some conversion issues. For example, when comparing values, define constants rather than using raw int values:
enum Status { SUCCESS, FAILURE; }
Using enums can help you avoid pitfalls related to implicit conversion.
-
Be Mindful of Auto-boxing and Unboxing: Java automatically converts between primitive types and their corresponding wrapper classes. This feature can save time but can also lead to unexpected behavior:
Integer wrapperValue = 1; int primitiveValue = wrapperValue; // Auto-unboxing // When it might fail wrapperValue = null; int number = wrapperValue; // Throws NullPointerException
Use in-place checks to prevent unnecessary exceptions and ensure clarity.
-
Verify Types Before Casting: Always use
instanceof
to check types before casting. Doing so will prevent ClassCastException during execution. -
Stay Updated: Java evolves, and new features may offer better ways for type handling. Familiarize yourself with language updates by visiting Oracle's Java Documentation.
Final Thoughts
Java conversion puzzles often provide important learning experiences. However, they can mislead due to assumptions about type safety, equality, and overflow. By understanding the intricacies of types and applying best practices, developers can avoid confusion and streamline their coding process.
Whether you're a novice or an experienced developer, remembering these insights can dramatically improve your coding skills and prevent errors down the line. Be sure to explore the numerous online resources available, engage with the community, and continue enhancing your understanding of Java.
By approaching Java conversion scenarios with a clear awareness of how type conversion works, you can navigate around pitfalls and embrace the benefits of a strong type system. Happy coding!