Unlocking the Hidden Complexity of Java 20 Features
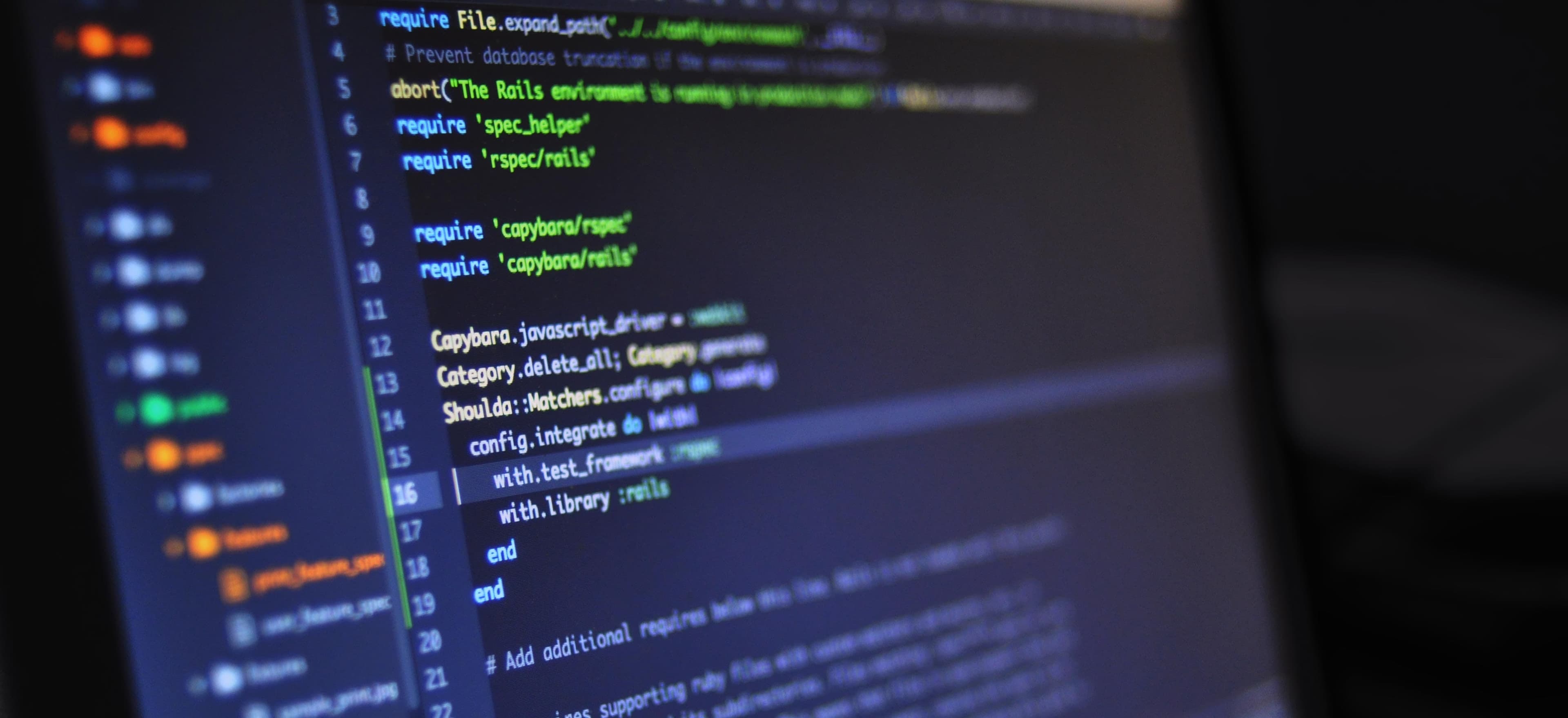
- Published on
Unlocking the Hidden Complexity of Java 20 Features
Java is continuously evolving, with each new version introducing exciting features designed to enhance developer productivity and improve code efficiency. Java 20 is no exception. This version, released in March 2023, adds several enhancements that delve deeper into the language's capabilities. In this blog post, we'll explore these features, walking through their functionalities with engaging examples that reveal their underlying significance.
The Highlights of Java 20
Before we dive into the specific features, let’s quickly outline some of the key improvements in Java 20:
- Record Patterns
- Pattern Matching for Switch (Preview)
- Scoped Values (Incubator)
- Foreign Function & Memory (Incubator)
- Virtual Threads (Preview)
Let’s explore each of these features in detail, emphasizing their importance in modern Java development.
1. Record Patterns
Introduced in Java 14 as a preview feature, Record Patterns have gained stability in Java 20. This feature allows developers to deconstruct records in a more concise and readable manner.
Example: Using Record Patterns
Consider the following record definition:
public record Point(int x, int y) {}
With record patterns, we can create a method that checks the position of a point and deconstructs it:
public String describePoint(Object obj) {
if (obj instanceof Point(int x, int y)) {
return "Point at coordinates: (" + x + ", " + y + ")";
}
return "Not a point!";
}
Why Use Record Patterns?
- Conciseness: It reduces boilerplate code.
- Readability: The structure makes it evident what properties we are accessing.
- Type Safety: The compiler guarantees that
obj
is indeed aPoint
before accessing its members.
Record patterns enhance code simplicity and make it easier to manage domain models.
2. Pattern Matching for Switch (Preview)
Another exciting feature is Pattern Matching for Switch. This aims to simplify conditional logic by allowing patterns to be matched against data in switch statements.
Example: Using Pattern Matching for Switch
Here’s how you could use this feature for handling various types:
public String handleObject(Object obj) {
return switch (obj) {
case String s -> "String: " + s;
case Integer i -> "Integer: " + i;
case Point p -> "Point with coordinates: " + p.x() + ", " + p.y();
default -> "Unknown type";
};
}
Why Pattern Match in Switch?
- Clarity: Each case is neatly defined.
- Type Casting Elimination: There's no need for instance checks and casting.
- Improved Readability: This approach makes the control flow more comprehensible.
By leveraging switch statements in this way, Java developers can write clearer and more maintainable conditional logic.
3. Scoped Values (Incubator)
Scoped values are a novel advanced feature that allows developers to define values with a limited scope, significantly reducing context propagation in concurrent programming.
Example: Using Scoped Values
Here's how you might use scoped values:
import java.util.concurrent.ScopeValue;
public class ScopedExample {
static final ScopeValue<Integer> scopedId = ScopeValue.newInstance();
public void run() {
scopedId.set(42);
// Code within this context has access to scopedId
runAsync();
}
private void runAsync() {
System.out.println("Scoped ID: " + scopedId.get());
}
}
Why Scoped Values?
- Concurrency Handling: Addresses issues that arise with traditional thread-local variables.
- Performance: As it minimizes the context-switching overhead associated with thread-local variables.
- Simplified Context Management: Anyone can inject a scoped context without disrupting the whole system.
Scoped values stand to improve managing context in multi-threaded applications in Java.
4. Foreign Function & Memory (Incubator)
Java 20 also introduces Foreign Function & Memory API, which provides a way to interact with code and data outside of the Java runtime, primarily useful for calling native libraries.
Example: Interfacing with Native Code
Here’s a look at calling a native C function:
import jdk.incubator.foreign.*;
public class ForeignFunctionExample {
static {
System.loadLibrary("native");
}
public static void main(String[] args) {
String result = callNativeFunction();
System.out.println("Result from native code: " + result);
}
private static String callNativeFunction() {
// Assume FFI libraries are set up
}
}
Why Use the Foreign Function & Memory API?
- Performance: Access native libraries directly can yield significant performance gains.
- Interoperability: This feature bridges the gap between Java and other languages.
- Efficient Memory Usage: Developers can manage memory on the heap or stack within the Java context.
This API opens new doors for high-performance applications needing low-level processing.
5. Virtual Threads (Preview)
Virtual Threads are set to revolutionize Java concurrency by allowing developers to write highly scalable applications without the complexity of traditional thread management.
Example: Using Virtual Threads
Here’s how virtual threads work in a simple example:
public class VirtualThreadExample {
public static void main(String[] args) {
Runnable task = () -> {
System.out.println("Executing task in virtual thread");
};
Thread.ofVirtual().start(task);
}
}
Why Utilize Virtual Threads?
- Easier Concurrency: Virtual threads can be created in a way that is more straightforward than traditional threads.
- Lightweight: They consume fewer resources, allowing applications to scale massively.
- Non-blocking I/O: Java can execute more simultaneous I/O operations, improving overall application responsiveness.
Virtual threads are a game-changer for developers looking to build concurrent applications efficiently.
Key Takeaways
Java 20 is packed with features that empower developers with new paradigms and possibilities. Record Patterns streamline record management, Pattern Matching simplifies logical flows, Scoped Values enhance concurrency handling, the Foreign Function & Memory API allows seamless integration with native code, and Virtual Threads provide a new route to concurrency.
For a deeper dive into Java, consider visiting the official Java documentation and follow the updates from Baeldung. Embracing these new features can significantly enhance your Java programming capabilities, leading to cleaner, maintainable, and more efficient applications.
As we continue to explore the depths of Java, it’s clear that the future holds even more innovative changes promising to redefine how we code. Embrace these advancements and unlock the hidden potential within Java 20!