Why Java 8 Lambdas Might Confuse New Developers
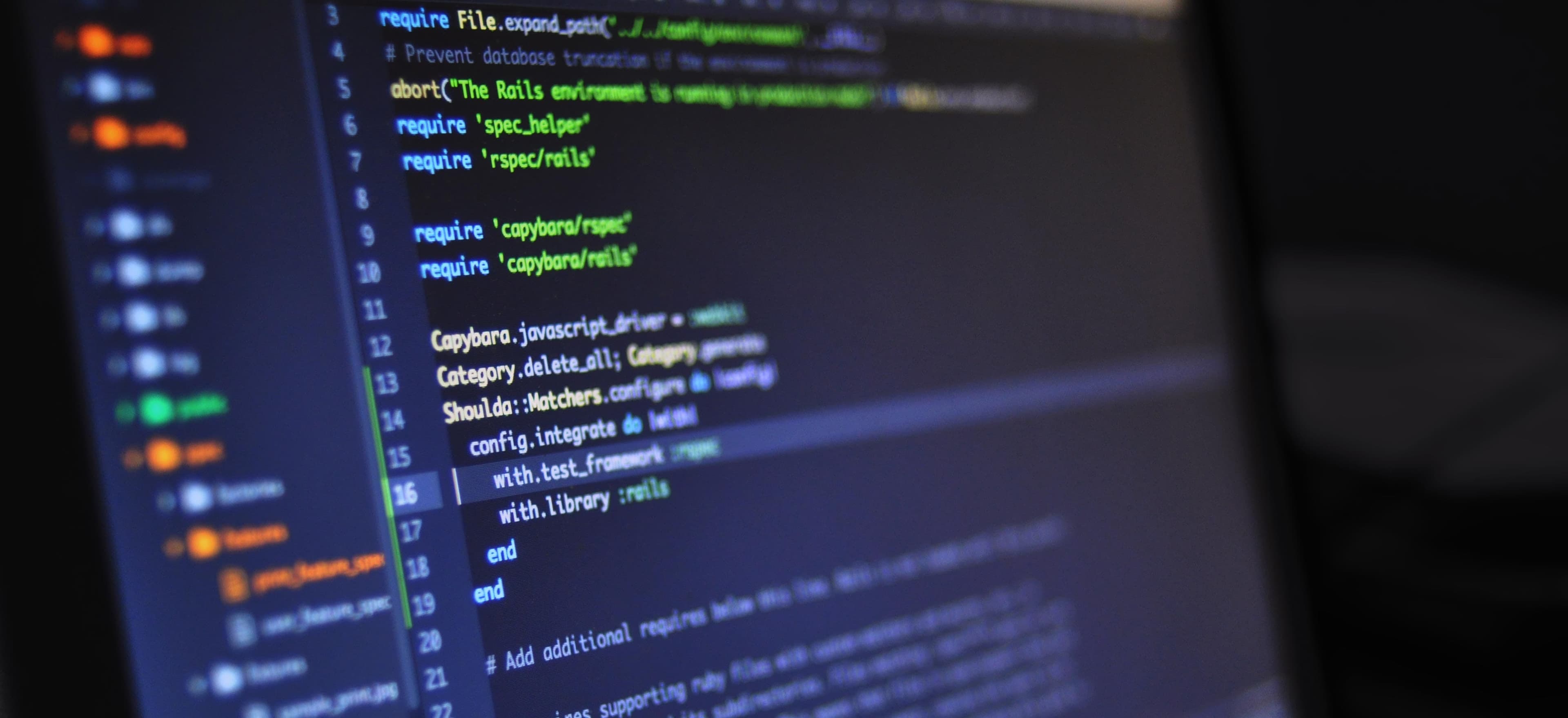
- Published on
Why Java 8 Lambdas Might Confuse New Developers
Java, one of the most popular programming languages, underwent a significant evolution with the introduction of Java 8. This version came with a plethora of features aimed at simplifying and enhancing code readability and performance. Among these features, lambda expressions stand out as both powerful and, at times, perplexing for new developers. In this blog post, we will dive into what lambdas are, why they can be confusing for those just starting with Java, and strategies to help ease understanding.
What are Lambda Expressions?
Lambda expressions provide a concise way to represent functional interfaces. A functional interface is an interface that contains exactly one abstract method. They are particularly useful for working with Java collections and streams, allowing developers to express instances of single-method interfaces (like Runnable, Callable, etc.) in a more succinct manner.
Basic Syntax
The syntax of a lambda expression is quite simple:
(parameters) -> expression
For example, here's a lambda expression that adds two numbers:
(int a, int b) -> a + b
The Benefits of Using Lambdas
There are several compelling reasons to leverage lambda expressions in Java:
- Conciseness: Lambdas reduce boilerplate code, allowing for more readable and maintainable codebases.
- Higher-order functions: Lambdas enable the passing of functionality as parameters.
- Enhanced Performance: The introduction of streams in Java 8 allows for parallel processing, which can enhance performance in certain scenarios, especially with large datasets.
The Confusion Around Java 8 Lambdas
1. Functional Interfaces
One of the most common sources of confusion is the concept of functional interfaces. New developers often grapple with what differentiates a functional interface from a regular interface. To clarify:
- A functional interface has only one abstract method.
This concept ties back to lambda expressions, which can only be used in places where a functional interface is expected.
Here’s an example of a functional interface:
@FunctionalInterface
public interface MathOperation {
int operate(int a, int b);
}
You can use a lambda expression to implement it:
MathOperation addition = (a, b) -> a + b;
2. Scope and Closure
When newcomers to Java 8 start writing lambdas, they often run into issues with variable scope and closure. Lambdas can access variables from their enclosing scope, but those variables must be effectively final. This means that the variable's value cannot change after it is initialized.
For instance, consider this example:
int number = 10;
Consumer<Integer> printNumber = (num) -> System.out.println(num + number);
printNumber.accept(5);
In this example, the lambda can access the number
variable, because it’s effectively final (it doesn’t change after assignment).
However, if you tried to modify number
within the lambda, it would result in a compilation error, which can be a frustrating point for new developers.
3. Type Inference
Java’s type inference may also add to the confusion. In many programming languages, especially dynamically typed ones, you do not have to worry about defining the data types explicitly. But Java, being statically typed, does need type definitions.
Consider the example:
List<String> names = Arrays.asList("John", "Jane", "Jack");
names.forEach(name -> System.out.println(name));
Here, Java infers that name
is of type String
. While this can simplify the code, new developers may still find it difficult to grasp initially because they are accustomed to explicitly defining types.
4. Integration with Streams API
The integration of lambdas with the Streams API offers unparalleled functionality. However, the concept of streams and how they work can be overwhelming for those just beginning their programming journey.
Here's a basic example of using a stream with lambda:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.filter(num -> num % 2 == 0) // this is the lambda expression
.forEach(System.out::println);
While the above code is clear for those who understand it, new developers may struggle at first with the concept of streams, filter and forEach methods, and why lambdas are necessary here.
Strategies for Learning
While lambdas may initially seem confusing, there are several strategies that new developers can employ to ease their understanding:
1. Practice with Examples
Hands-on practice is crucial. Experiment with creating and using functional interfaces and lambdas in various scenarios. The more you do it, the more intuitive it will become.
2. Break Down Complex Expressions
When encountering a complex lambda expression, break it down. Write out what the expression is doing in regular code before converting it to a lambda. For example, the following code:
List<String> filtered = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
Start by understanding what filter
and collect
are doing in a more verbose manner before translating it into a lambda.
3. Utilize Debugging Tools
Use debuggers to step through your code. This will help provide clarity on how values are being passed around and manipulated within your lambdas, letting you see their behavior in real-time.
4. Attend Workshops or Online Courses
There are various free resources available that walk through lambdas in detail. Platforms like Codecademy, Coursera, or Udemy offer comprehensive courses that cover these concepts in depth.
5. Engage with the Community
Join forums or communities to discuss your challenges. Websites like Stack Overflow provide a wide range of questions and solutions related to lambdas. Engaging with others can often clarify confusion.
Closing the Chapter
Java 8 lambdas represent a powerful advancement in the language, but they can certainly mystify new developers. A solid understanding of functional interfaces, scope, type inference, and how lambdas integrate with the Streams API is imperative to mastering them.
By following the strategies outlined above, developers can gradually demystify lambda expressions and embrace the substantial advantages they provide.
For more information on Java 8 and its features, consider checking out the official Java tutorials.
Happy coding!
By understanding the nuance of concepts like lambdas, developers can harness the full power of Java 8, writing cleaner and more efficient code.