Common Pitfalls of Functional Programming in Java
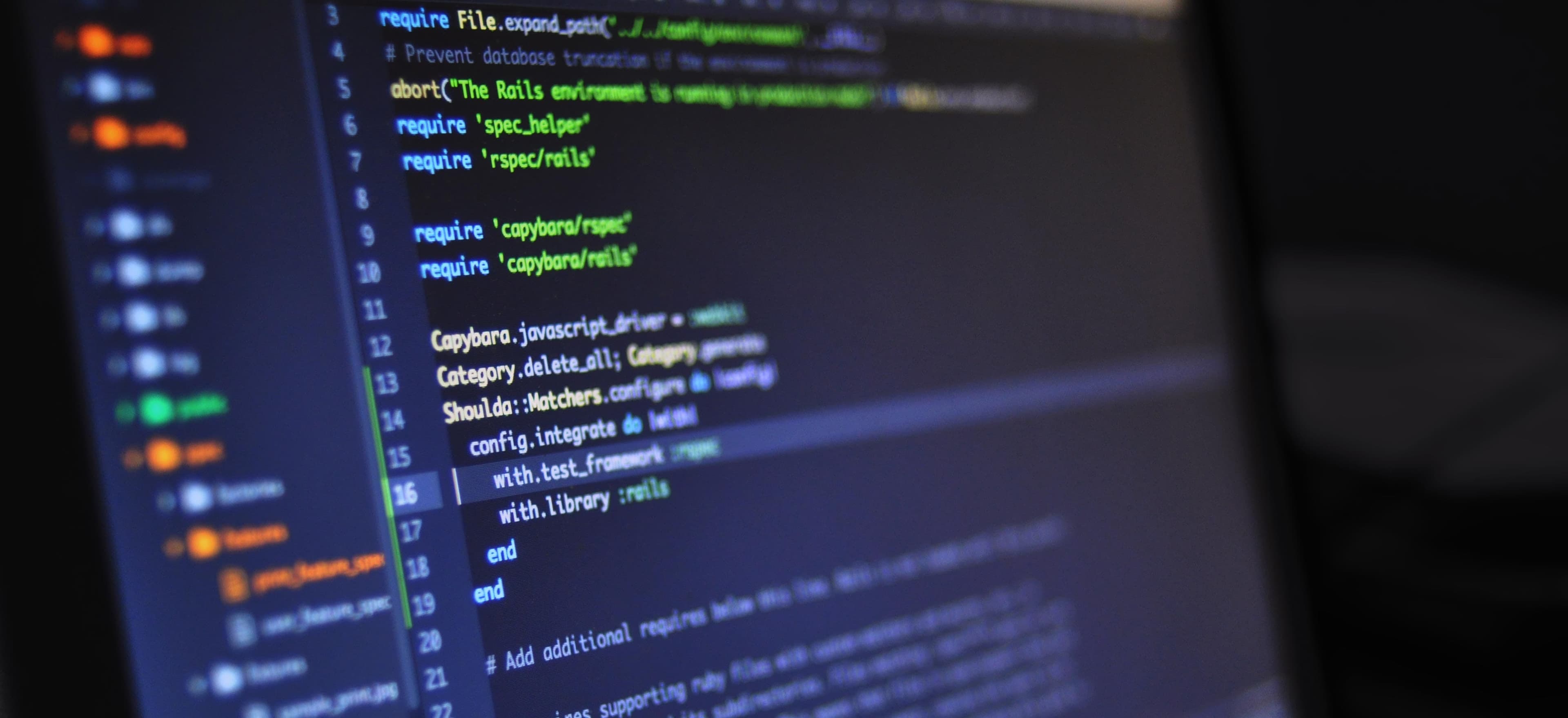
- Published on
Common Pitfalls of Functional Programming in Java
Functional programming is an increasingly popular paradigm in Java, especially with the introduction of Java 8. It offers powerful tools such as Lambdas, Streams, and functional interfaces. However, despite its advantages, several pitfalls can trip up developers who are new to this approach. In this post, we'll discuss these common pitfalls, provide illustrative code snippets, and explain the reasoning behind best practices.
Understanding Functional Programming
Before delving into the pitfalls, it’s critical to understand the foundation of functional programming (FP). FP emphasizes immutability, first-class functions, and declarative code as opposed to the imperative style of traditional object-oriented programming. In Java, this is made easier with:
- Lambdas: An expressive way to implement functional interfaces.
- Streams: A sequence of elements supporting sequential and parallel aggregate operations.
While these features enhance productivity and code clarity, they have their complexities.
Pitfall 1: Overusing Lambdas
One of the common mistakes when adopting functional programming in Java is the overuse of lambdas. While they provide a conciseness that can make code look cleaner, using them excessively can lead to code that is harder to read and maintain.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
// Using a lambda for filtering
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("A"))
.collect(Collectors.toList());
Why It Matters
Using lambdas for simple operations is beneficial, but excessively chaining operations can obfuscate the intent of the code. Always prioritize clarity. If a sequence of operations becomes too involved, consider breaking it down into named methods or using a more straightforward approach.
Pitfall 2: Ignoring Performance
While using streams can lead to elegant solutions, overlooking their performance implications can result in inefficient code. Here's a cautionary example:
List<Integer> largeList = IntStream.range(1, 1000000).boxed().collect(Collectors.toList());
// Inefficient filtering
List<Integer> filteredList = largeList.stream()
.filter(num -> num % 2 == 0)
.collect(Collectors.toList());
Why It Matters
Streams, especially parallel streams, can improve performance, but they also introduce overhead. Always measure performance impacts using tools like JMH (Java Microbenchmark Harness) before adopting these solutions, particularly for large datasets.
Pitfall 3: Mutable State in Lambdas
Lambdas should be stateless. A common error is capturing mutable state leading to unpredictable results. Here's an example:
List<Integer> numbers = Arrays.asList(1, 2, 3);
int threshold = 2;
// Mutable state captured in the lambda
numbers.forEach(number -> {
if (number > threshold) {
threshold++;
System.out.println(number);
}
});
Why It Matters
In this code, the threshold
variable can change unpredictably due to its mutable nature, which could lead to side effects. To maintain functional programming principles, avoid captured mutable variables by using final or effectively final variables.
Pitfall 4: Not Embracing Immutability
Another common oversight is neglecting immutability, which can introduce bugs and side effects in your code. Here's an example of how mutable structures can cause issues:
List<String> originalList = new ArrayList<>(Arrays.asList("A", "B", "C"));
// Mutable operation
List<String> modifiedList = originalList.stream()
.map(name -> {
originalList.add("D");
return name.toLowerCase();
})
.collect(Collectors.toList());
System.out.println(modifiedList);
System.out.println(originalList);
Why It Matters
In this case, modifying originalList
during the stream processing can yield unexpected behavior. Embrace immutability and avoid changing the data structure you are iterating over. You can achieve this through immutable collections offered in libraries like Guava or Java's own Collections.unmodifiableList()
method.
Pitfall 5: Misunderstanding Optional
The Optional
type is a powerful feature that prevents null values. However, its misuse can lead to a bloated codebase. Consider this example:
Optional<String> optional = Optional.of("Hello");
optional.ifPresent(name -> {
// Using Optional improperly
if (optional.isPresent()) {
System.out.println(optional.get());
}
});
Why It Matters
The above implementation unnecessarily calls isPresent()
before ifPresent()
, adding complexity to the code. Instead, leverage ifPresent
directly, or explore other methods provided by Optional
, like map
, orElse
, or flatMap
.
Pitfall 6: Confusing Side Effects with Functional Programming
Functional programming is about creating pure functions—functions that always produce the same output for the same input without side effects. If your functions modify external state, you are not leveraging FP properly.
int total = 0;
List<Integer> values = Arrays.asList(1, 2, 3);
// Function with side effect
values.forEach(value -> total += value);
System.out.println(total);
Why It Matters
This example illustrates a side effect (modifying total
) that contradicts functional principles. Instead, consider returning a result without modifying any external state:
int sum = values.stream().mapToInt(Integer::intValue).sum();
Closing the Chapter
Functional programming in Java offers a wealth of opportunities for clean, efficient, and elegant code. However, pitfalls abound for the uninformed. By adhering to principles like immutability, avoiding mutable state in lambdas, and properly leveraging the Optional
type, developers can maximize the benefits of this paradigm.
Embrace these practices and avoid the common pitfalls we discussed in this article to enhance not only your Java skills but also the maintainability and clarity of your code.
For more insights into functional programming concepts in Java, check Oracle's official guide on Functional Programming. You can also explore community resources for advanced topics at Baeldung’s guide on Java 8 Streams.
Stay safe and happy coding!