Choosing the Right Language: High vs Low Level Dilemmas
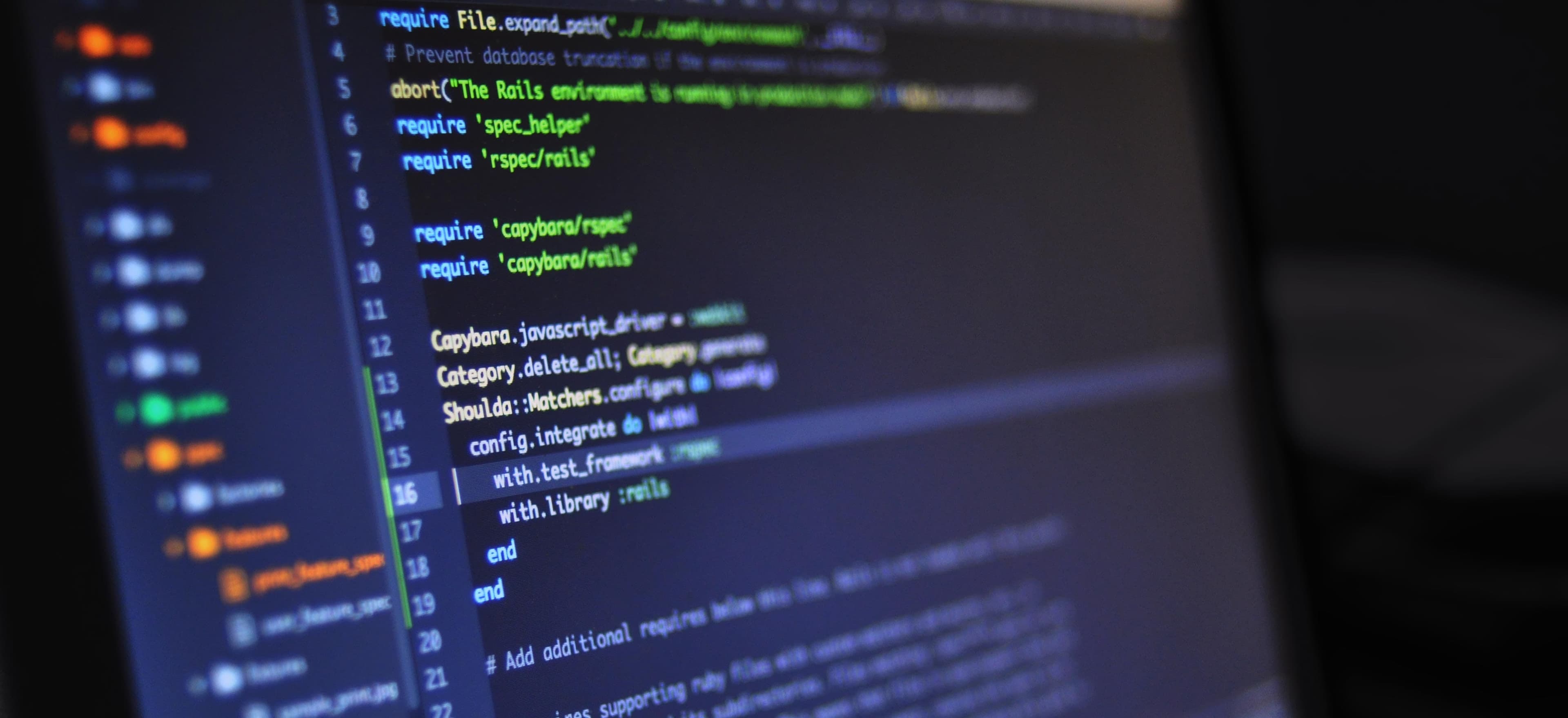
- Published on
Choosing the Right Language: High vs Low-Level Dilemmas
When delving into the world of programming and software development, one has to confront a fundamental question: what programming language should be chosen for a project? This question opens up a Pandora's box of considerations, particularly when distinguishing between high-level and low-level languages. In this blog post, we will explore the dilemmas surrounding the choice between these types of languages, their distinct characteristics, and how they affect project decisions.
Understanding High-Level and Low-Level Languages
High-Level Languages
High-level languages are designed to be easy for humans to read and write. They abstract away much of the complexity associated with machine code, allowing developers to focus on solving problems rather than managing memory and system architecture.
Key Characteristics of High-Level Languages
- Abstraction: High-level languages provide abstractions over the machine’s hardware. For instance, you do not need to manage memory manually.
- Readability: Code written in high-level languages is typically more readable and closer to natural language, making it easier for developers to understand and maintain.
- Portability: High-level languages can often run on various platforms without modification. For example, Python code can run on both Windows and Unix systems with minimal changes.
Examples of high-level languages include Python, Ruby, and Java.
Low-Level Languages
Low-level languages, on the other hand, provide minimal abstraction from a computer's instruction set architecture. They give programmers direct control over hardware and memory management, leading to potential optimization benefits.
Key Characteristics of Low-Level Languages
- Control: Low-level languages offer greater control over hardware, which can be crucial for performance-intensive applications.
- Efficiency: By working closer to machine code, programs can be optimized for speed and resource utilization.
- Complexity: These languages are generally harder to read and write, which can lead to maintenance challenges.
Assembly language and C are prominent examples of low-level languages.
The High-Level vs. Low-Level Dilemma
Choosing the appropriate language usually involves balancing various factors such as project requirements, performance, team expertise, and future maintenance. Below are key considerations to navigate this dilemma.
Performance
If a project requires maximum performance—like real-time systems, graphics engines, or embedded systems—low-level languages may be more appropriate. They allow fine-tuning of hardware resources, which can be pivotal for performance.
Here’s a simple example in C that demonstrates low-level memory management:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr;
int size = 5;
arr = (int*)malloc(size * sizeof(int));
for(int i = 0; i < size; i++) {
arr[i] = i * 2; // Assigning values
}
for(int i = 0; i < size; i++) {
printf("%d ", arr[i]); // Displaying values
}
free(arr); // Manual memory management
return 0;
}
In this C program, we create an array using dynamic memory allocation. Using malloc
allows the program to allocate memory at runtime, offering control over memory management. This is the essence of low-level programming: the fine control that can yield performance gains.
Development Speed
High-level languages tend to enable faster development cycles. As they provide rich libraries and frameworks, developers can build and deploy applications swiftly. This advantage is particularly crucial for startups or projects with tight deadlines.
Consider the following Java example using a high-level approach to create a simple REST API:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
This code demonstrates how easy it is to create a simple web application using the Spring Boot framework in Java. We define a REST controller and an endpoint with just a few lines of code. High-level languages like Java provide libraries that offer extensive functionality out-of-the-box, speeding up development.
Team Expertise
The expertise of your team is a critical factor. If your team is well-versed in C and assembly, it makes sense to leverage low-level languages, especially if project requirements demand it. On the other hand, if your developers are more comfortable with high-level languages, efficiency and maintainability might be prioritized by opting for languages like Python or Java.
Consider the following table:
| Factor | High-Level Languages | Low-Level Languages | |---------------------|-------------------------------------|----------------------------------------| | Abstraction | High | Low | | Control | Limited | Greater | | Performance | Generally slower | Generally faster | | Readability | High | Low | | Learning Curve | Easier | Steeper | | Development Speed | Rapid | Slower due to complexity |
Choosing the Right Language: A Case Study
Let's examine a hypothetical case to illustrate the decision-making process. Suppose a company is developing a new video game. The requirements include high performance and the ability to handle real-time graphics and complex computations.
Given these requirements, the use of a low-level language, such as C++, would be suitable. C++ provides the performance needed to handle graphics rendering efficiently. Here is an example of a simple C++ function for rendering:
#include <iostream>
void render() {
// Pseudocode for rendering graphics
std::cout << "Rendering frame..." << std::endl;
}
Rendering frames in a game is a resource-intensive task. C++ minimizes latency, thereby providing smoother interactions and immersive gameplay.
Conversely, if the same company were creating a back-end service for player data management, a high-level language like Java could be utilized:
import java.util.HashMap;
public class PlayerData {
private HashMap<String, Integer> scores;
public PlayerData() {
scores = new HashMap<>();
}
public void addScore(String player, int score) {
scores.put(player, scores.getOrDefault(player, 0) + score);
}
public int getScore(String player) {
return scores.getOrDefault(player, 0);
}
}
This Java example illustrates how simple it is to manage player data using high-level constructs like HashMap
. Here, the focus is on development speed and maintainability.
Closing Remarks: The Balance of Choices
The choice between high-level and low-level languages is rarely black and white; it often involves navigating a range of trade-offs. Factors such as application performance, team expertise, and project timelines will influence your decision significantly.
The takeaway is clear: assess your project needs thoroughly. If performance is paramount and precision is required, do not hesitate to dive into low-level programming. Conversely, if rapid development and ease of maintainability are your priorities, high-level languages should be your go-to.
For more insights on programming language choices, check out IEEE Spectrum's Programming Language Rankings for updated trends and analysis. Additionally, you can explore Codecademy's Language Resources to enhance your understanding of different programming languages.
Ultimately, the right choice will lead to successful project outcomes, bridging the gap between vision and implementation. Happy coding!