Mastering Cross-Process Communication in JBoss BPM
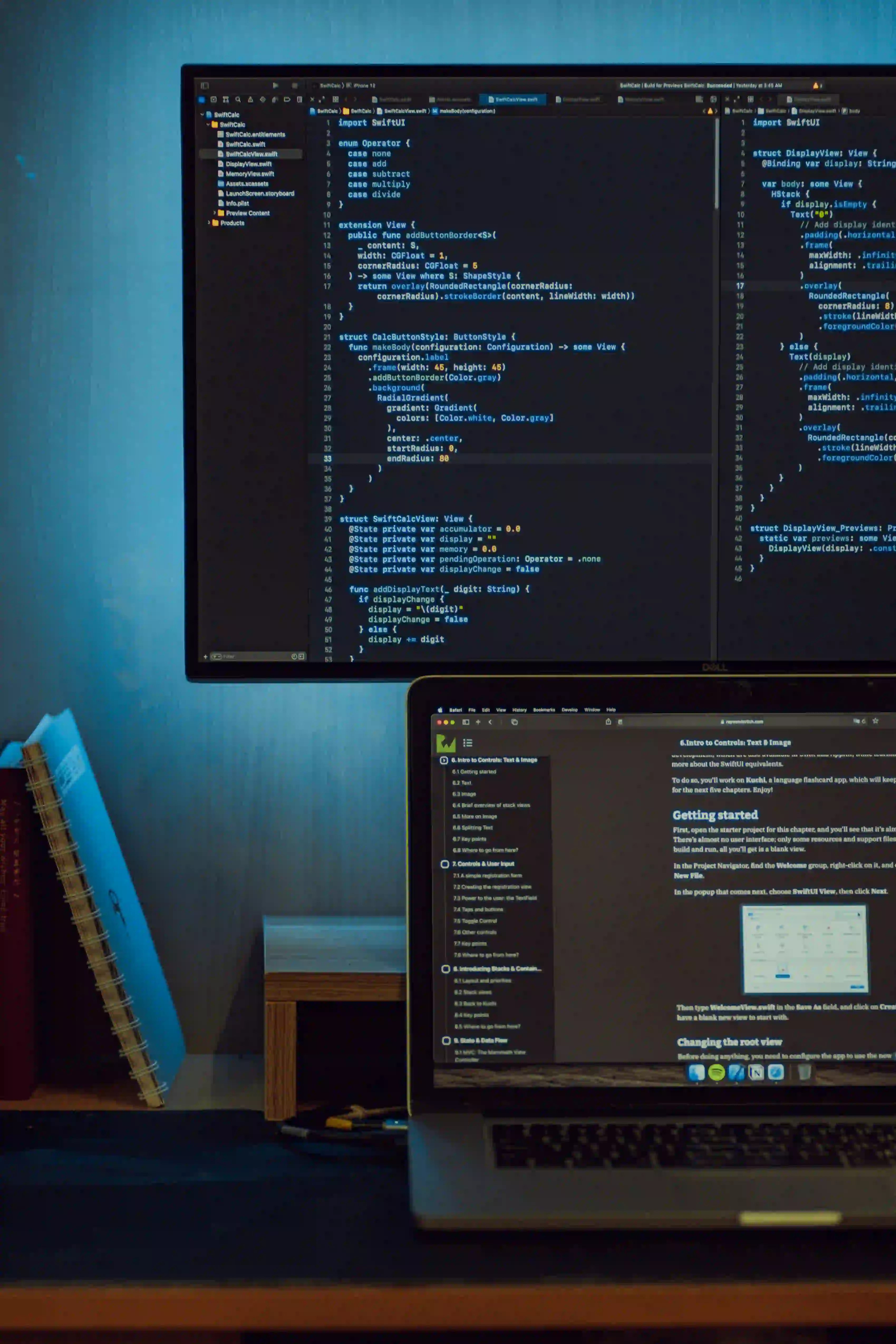
Mastering Cross-Process Communication in JBoss BPM
Cross-Process Communication (CPC) in JBoss BPM (Business Process Management) is a crucial aspect of building scalable and maintainable enterprise systems. As organizations grow, the need for processes to interact seamlessly becomes paramount. JBoss BPM, being a robust platform, provides various techniques to facilitate this requirement. This blog post will explore these methods and provide code examples with thorough explanations, guiding you toward mastering CPC.
Understanding Cross-Process Communication
Before we delve into the implementation aspects, let’s define what we mean by Cross-Process Communication in the context of JBoss BPM. In simple terms, it refers to the ability of different business processes to communicate and collaborate effectively, exchanging information and triggering actions across various workflows.
This communication is essential for:
- Data Sharing: Allowing multiple processes to access and modify shared data.
- Event Handling: Enabling one process to react to events triggered by another process.
- Orchestration: Coordinating the execution of related processes, ensuring they work harmoniously.
Understanding these principles lays the foundation for implementing effective communication strategies in JBoss BPM.
Core Communication Techniques in JBoss BPM
JBoss BPM provides several methods for achieving Cross-Process Communication, including:
- Message Events
- Service Tasks
- Signal Events
- RESTful APIs
Let’s explore these techniques in detail with practical examples.
1. Message Events
Message events are the primary way for one process to send a message to another. They can be classified into two types: start message events and intermediate message events.
Start Message Event Example
Consider two processes, OrderProcess
and ShippingProcess
. The OrderProcess
sends a message to initiate the ShippingProcess
.
BPMN Model for OrderProcess:
<process id="OrderProcess" name="Order Process" isExecutable="true">
...
<startEvent id="StartOrderEvent" name="Start Order" />
<intermediateCatchEvent id="WaitForShippingEvent">
<messageEventDefinition messageRef="ShippingMessage" />
</intermediateCatchEvent>
...
</process>
Message Sending Code:
In the OrderProcess, after an order is confirmed, we send a message to the ShippingProcess.
@MessageGateway
public class OrderGateway {
@Inject
private KieSession kieSession;
public void sendShippingMessage(Order order) {
ShippingMessage message = new ShippingMessage(order);
kieSession.signalEvent("ShippingEvent", message);
}
}
Why Use This?
This approach allows us to decouple the OrderProcess
from the ShippingProcess
, promoting easier changes and maintenance.
2. Service Tasks
Service tasks are a way to integrate backend services or other processes. You can use them to interact with external systems or even trigger other processes.
Example of a Service Task
For instance, let’s say we want to trigger the ShippingProcess
through a service task after the order processing is complete.
<serviceTask id="StartShippingProcess" name="Start Shipping Process" implementedBy="JavaDelegate">
<extensionElements>
<bpmn:field name="orderId" expression="${orderId}"/>
</extensionElements>
</serviceTask>
Java Implementation:
public class StartShippingProcess implements JavaDelegate {
@Inject
private RuntimeService runtimeService;
@Override
public void execute(DelegateExecution execution) {
String orderId = (String) execution.getVariable("orderId");
Map<String, Object> variables = new HashMap<>();
variables.put("orderId", orderId);
runtimeService.startProcessInstanceByKey("ShippingProcess", variables);
}
}
Why Use This?
Using service tasks enables automation of process transitions, reducing manual error and ensuring that processes are triggered reliably.
3. Signal Events
Signal events allow you to broadcast messages that can be caught by multiple processes. This is particularly useful when several processes need to listen for changes or updates.
Signal Example
For instance, when a payment is processed, multiple processes might need to act on that event.
BPMN Model:
<process id="PaymentProcess">
...
<signalEventDefinition id="PaymentProcessedSignal" />
...
</process>
<process id="OrderProcess">
...
<intermediateCatchEvent id="WaitForPaymentSignal">
<signalEventDefinition signalRef="PaymentProcessedSignal" />
</intermediateCatchEvent>
...
</process>
Java Implementation:
To signal other processes when a payment is processed:
public void processPayment(Payment payment) {
// Code to process payment
runtimeService.signalEventReceived("PaymentProcessedSignal");
}
Why Use This?
Signal events facilitate a loosely coupled architecture, allowing different components to react to domain events without direct dependencies.
4. RESTful APIs
In modern architectures, especially microservices, RESTful APIs are essential. JBoss BPM can expose processes via REST, making them accessible from different services or applications.
RESTful Implementation
You can expose a process with a simple annotation in JBoss BPM:
@Path("/order")
public class OrderEndpoint {
@Inject
private RuntimeService runtimeService;
@POST
@Path("/create")
@Consumes(MediaType.APPLICATION_JSON)
public Response createOrder(Order order) {
runtimeService.startProcessInstanceByKey("OrderProcess", order);
return Response.status(Response.Status.CREATED).build();
}
}
Why Use This?
RESTful API integration promotes interoperability between various systems, enabling you to build more flexible and scalable architectures.
Best Practices for Effective Cross-Process Communication
To truly master Cross-Process Communication in JBoss BPM, consider the following best practices:
- Maintain Loose Coupling: Use message events and signals to ensure processes are not tightly integrated, allowing independent updates.
- Centralized Error Handling: Implement a centralized error-handling mechanism to manage exceptions across processes.
- Utilize BPMN Standards: Follow BPMN standards to ensure your process definitions are clear and maintainable.
- Monitoring and Logging: Implement monitoring tools to track process execution and communication, ensuring visibility into system behavior.
- Testing: Rigorously test the communication paths and scenarios to identify any breaking points in interactions.
Closing the Chapter
Mastering Cross-Process Communication in JBoss BPM is vital for any organization looking to build robust, scalable, and maintainable enterprise systems. The techniques discussed in this post, including message events, service tasks, signal events, and RESTful APIs, form the backbone of effective communication strategies.
By incorporating these patterns and adhering to best practices, you can ensure that your processes work together seamlessly, ultimately enhancing workflow efficiency and flexibility. As you continue to explore JBoss BPM, remember that effective communication is the key that unlocks the potential of your enterprise processes.
For more information on enhancing your knowledge of JBoss BPM, check out the official documentation for further insights and in-depth guides on the platform. Happy coding!