Common Pitfalls When Mapping JSON with Jackson
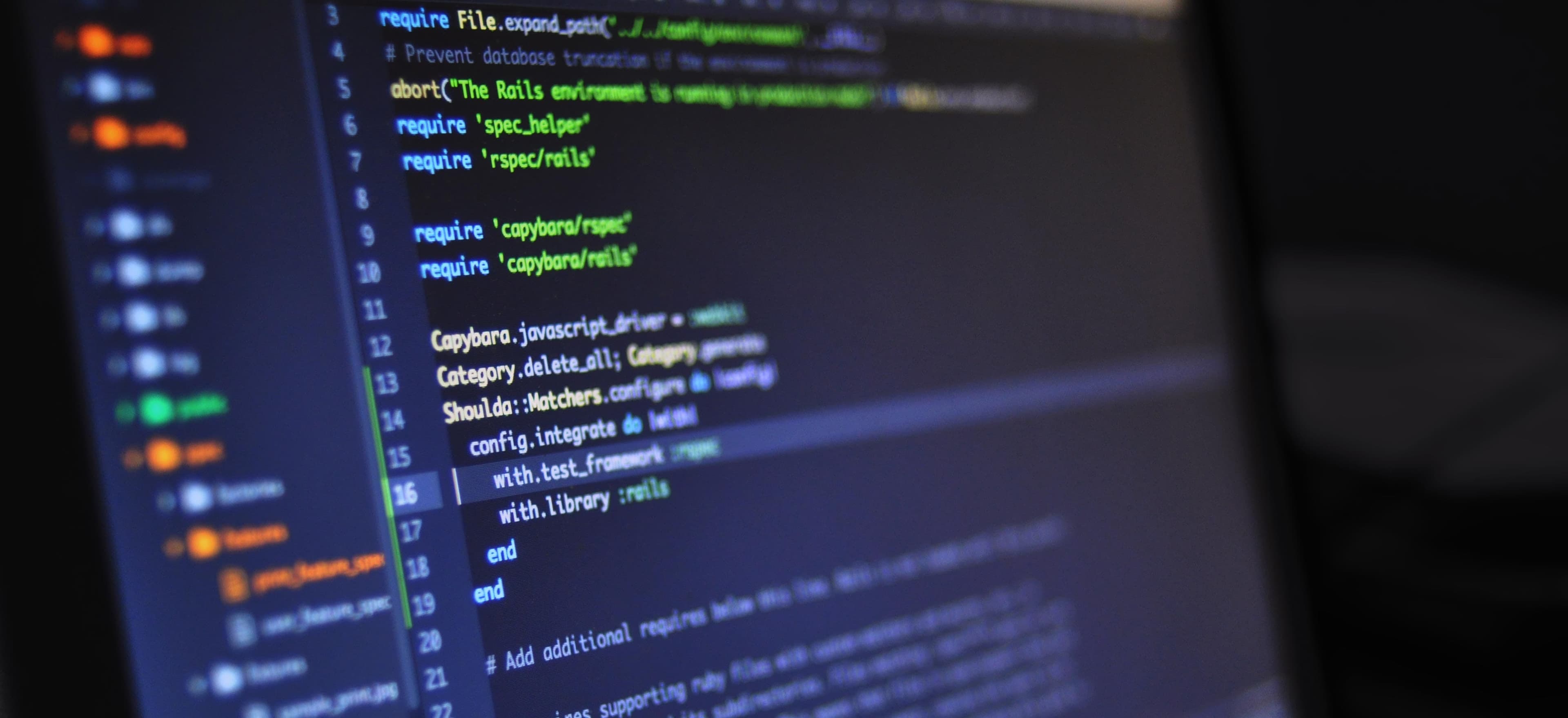
- Published on
Common Pitfalls When Mapping JSON with Jackson
Jackson is a powerful library for configuring and manipulating JSON in Java applications. It comes with a variety of features that greatly simplify the process of converting Java objects to JSON and vice versa. However, while using Jackson, developers can run into several common pitfalls that may hinder their productivity or lead to unexpected results. In this post, we will address these common mistakes, provide recommendations, and demonstrate how to avoid them using exemplary code snippets.
Understanding Jackson Annotations
Before we dive into the pitfalls, let’s briefly review how Jackson works. Jackson uses a variety of annotations to control JSON serialization and deserialization, such as @JsonProperty
, @JsonIgnore
, and @JsonCreator
. Understanding these basic annotations is crucial for effectively manipulating JSON data.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("name")
private String username;
@JsonProperty("age")
private int userAge;
// Getters and Setters
}
Pitfall 1: Ignoring Default Constructors
One of the simplest mistakes is forgetting to define a default (no-argument) constructor for classes that you plan to deserialize. Jackson requires a no-argument constructor to instantiate an object during the deserialization process.
Solution: Always ensure that your classes have a no-argument constructor.
public class User {
private String username;
private int userAge;
public User() {
// Default constructor
}
// Parameterized constructor, Getters and Setters
}
Pitfall 2: Incorrect Field Accessibility
Jackson does not have direct access to private fields unless you tell it to. If your fields are private, they need appropriate getters/setters for Jackson to serialize or deserialize the data.
Solution: Use public getters and setters.
public class User {
private String username;
// Getter
public String getUsername() {
return username;
}
// Setter
public void setUsername(String username) {
this.username = username;
}
}
Pitfall 3: Mismatched Field Names
A frequent issue occurs when the field names in the JSON do not match the names of the fields in your Java class. Java's naming conventions often differ from Jackson's expectations, which can lead to null
values in deserialized objects.
Solution: Use the @JsonProperty
annotation to map JSON keys to appropriate Java fields.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
@JsonProperty("name")
private String username;
@JsonProperty("age")
private int userAge;
// Getters and Setters
}
Pitfall 4: Not Handling Date Formats Properly
When dealing with dates, the default date format used by Jackson may not match the format of your input JSON. It can lead to JsonMappingException
if Jackson cannot parse the format properly.
Solution: Specify the date format using the @JsonFormat
annotation.
import com.fasterxml.jackson.annotation.JsonFormat;
public class User {
@JsonFormat(pattern = "yyyy-MM-dd")
private Date birthDate;
// Getter and Setter
}
Pitfall 5: Nested Objects Handling
Many developers expect Jackson to automatically handle nested objects without additional configuration. While it can deserialize nested structures, proper planning is needed to ensure correct mapping.
Solution: Make sure to create separate classes for nested objects and apply the necessary Jackson annotations.
public class Address {
private String street;
private String city;
// Getters and Setters
}
public class User {
private String username;
private Address address;
// Getters and Setters
}
Pitfall 6: Not Configuring Null Value Handling
By default, Jackson will serialize null
values into your JSON output, which may not be desirable. Sometimes, you might want to omit fields that are null
.
Solution: Use the @JsonInclude
annotation to control this behavior.
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
@JsonInclude(Include.NON_NULL)
public class User {
private String username;
private String email;
// Getters and Setters
}
Pitfall 7: Overlooking Composition with Collections
When working with collections, such as lists or sets, developers might forget to specify the generic type. This oversight can lead to runtime exceptions or incorrect deserialization.
Solution: Always use the proper generic types with collections.
import java.util.List;
public class UsersGroup {
private List<User> users;
// Getters and Setters
}
Pitfall 8: Failing to Handle Polymorphic Types
If you have a class hierarchy and want to deserialize a polymorphic type, failing to configure polymorphic type handling can result in issues. Default deserialization will not understand which subclass to instantiate.
Solution: Use the @JsonTypeInfo
and @JsonSubTypes
annotations to maintain information about the type on the serialized JSON.
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type")
@JsonSubTypes({
@JsonSubTypes.Type(value = AdminUser.class, name = "admin"),
@JsonSubTypes.Type(value = RegularUser.class, name = "regular")
})
public abstract class User {
private String username;
// Getters and Setters
}
Wrapping Up
Jackson is a robust library that greatly simplifies JSON parsing in Java applications. However, avoiding the common pitfalls discussed above is crucial for effective usage. By being aware of default constructors, accessibility rules, field naming conventions, and proper annotations, you can ensure a smoother experience while working with JSON mapping in Jackson.
For more in-depth information about Jackson, consider visiting the official documentation. This resource can provide further insights and advanced configurations to help you refine your JSON handling capabilities.
By understanding and implementing the solutions to these pitfalls, you can harness the full power of Jackson in your Java projects, leading to cleaner code and more robust applications. Happy coding!