Common Pitfalls When Parsing XML in Java
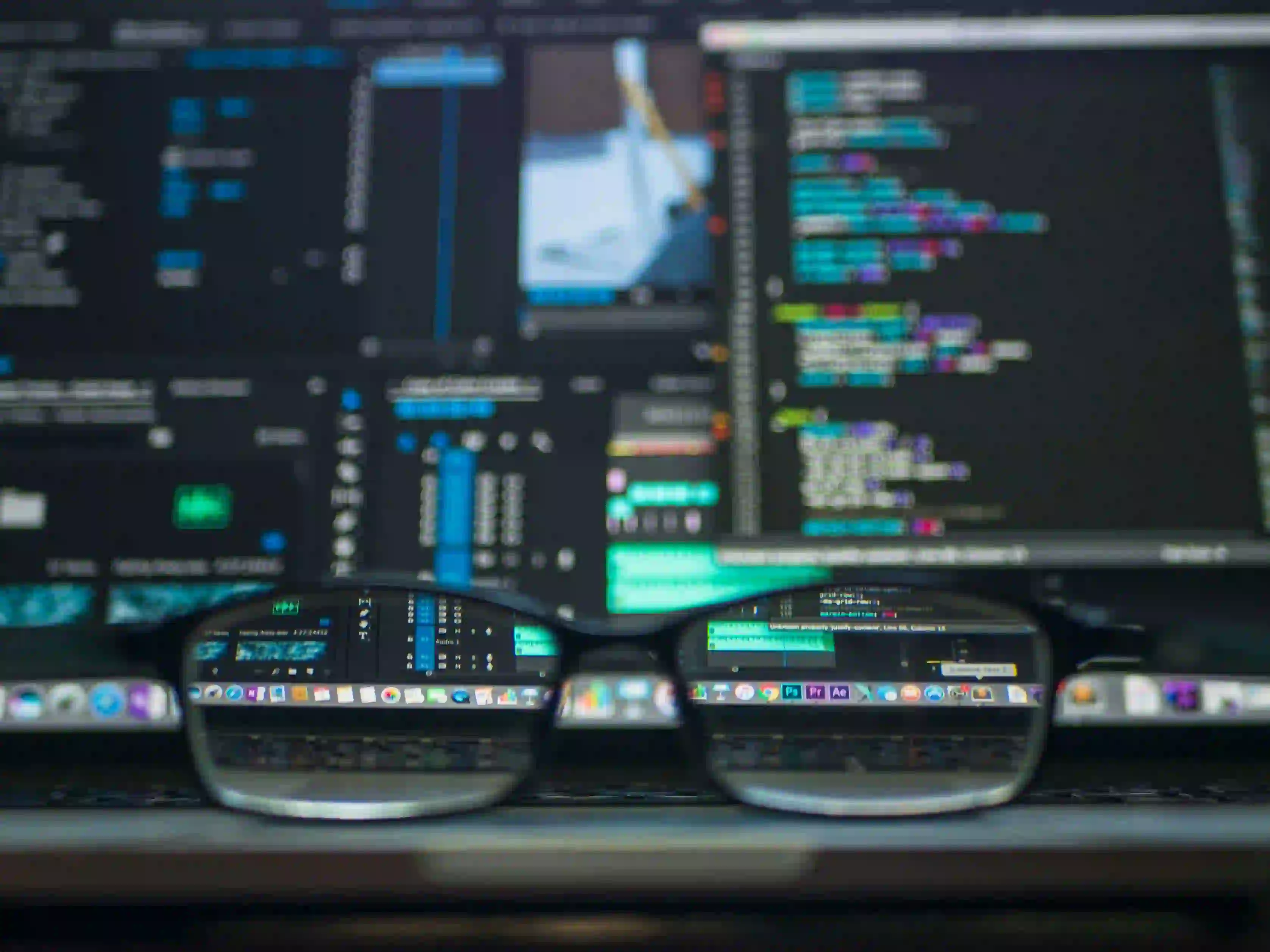
Common Pitfalls When Parsing XML in Java
XML (Extensible Markup Language) is a widely-used format for data interchange. Its versatility makes it a staple in web services, configuration files, and more. However, parsing XML in Java can be riddled with challenges that can lead to frustrating bugs or inefficient code. In this blog post, we’ll explore common pitfalls faced when parsing XML in Java and how to avoid them.
Understanding XML Parsing in Java
Java provides multiple libraries for XML parsing, including:
- DOM (Document Object Model): Loads the entire XML document into memory as a tree structure.
- SAX (Simple API for XML): An event-driven, serial-access mechanism to read XML.
- StAX (Streaming API for XML): A pull-parsing mechanism, allowing more control over parsing.
While these libraries offer flexibility, improper usage can lead to issues. Let’s break down the most common pitfalls.
1. Choosing the Wrong Parsing Method
Pitfall
Choosing the wrong XML parsing method for your use case can lead to performance issues or complex code.
Solution
Understand the nature of the XML data you are working with.
- Use DOM for smaller XML documents when you need to navigate the structure arbitrarily.
- Use SAX for large files where memory efficiency is a concern and you need a one-pass read.
- Use StAX if you require more control over the parsing process without loading the entire document.
Code Example: DOM vs. SAX
Here’s a simple comparison of DOM and SAX parsing:
// DOM Parsing Example
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import org.w3c.dom.Document;
public class DOMParserExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("data.xml");
// Now you can manipulate the XML data
} catch (Exception e) {
e.printStackTrace();
}
}
}
// SAX Parsing Example
import org.xml.sax.*;
import org.xml.sax.helpers.DefaultHandler;
import javax.xml.parsers.*;
public class SAXParserExample {
public static void main(String[] args) {
try {
SAXParserFactory factory = SAXParserFactory.newInstance();
SAXParser saxParser = factory.newSAXParser();
DefaultHandler handler = new DefaultHandler() {
@Override
public void startElement(String uri, String localName, String qName, Attributes attributes) {
System.out.println("Start Element: " + qName);
}
};
saxParser.parse("data.xml", handler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters
Choosing the right parser is crucial for maintaining efficient resource usage. Using DOM for large files can lead to OutOfMemory exceptions, while SAX requires less overhead when handling extensive XML data.
2. Improper Error Handling
Pitfall
Failing to handle exceptions during parsing can lead to partially read documents or silent failures.
Solution
Implement robust error handling mechanisms. Java's XML parsers throw a variety of exceptions, such as SAXException
, ParserConfigurationException
, and IOException
.
Code Example: Exception Handling in SAX
try {
// parsing code
} catch (ParserConfigurationException e) {
System.err.println("Parser configuration error: " + e.getMessage());
} catch (SAXException e) {
System.err.println("Parsing error: " + e.getMessage());
} catch (IOException e) {
System.err.println("IO error: " + e.getMessage());
}
Why This Matters
Proper error handling is essential for debugging and maintaining code. Ignoring exceptions might mask the underlying issues, making them harder to trace later.
3. Ignoring XML Namespaces
Pitfall
Ignoring XML namespaces can lead to critical errors in data extraction and manipulation.
Solution
Understand namespaces when working with XML documents. Properly handle them in your code, especially if your XML uses them extensively.
Code Example: Handling Namespaces
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.DocumentBuilder;
import org.w3c.dom.*;
public class NamespaceExample {
public static void main(String[] args) {
try {
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
factory.setNamespaceAware(true);
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse("namespaced_data.xml");
NodeList nodes = doc.getElementsByTagNameNS("http://example.com/schema", "elementName");
for (int i = 0; i < nodes.getLength(); i++) {
Element element = (Element) nodes.item(i);
System.out.println("Element: " + element.getTextContent());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters
XML namespaces help avoid element name collisions, especially when combining XML documents from different sources. Neglecting them can result in data not being read correctly.
4. Performance Issues
Pitfall
Inefficient parsing logic can lead to performance bottlenecks. This is often caused by looping through nodes without leveraging the XML structure effectively.
Solution
Optimize your parsing algorithm. Use built-in filtering and selectors to limit the nodes processed.
Code Example: Efficient Node Processing
NodeList nodeList = doc.getElementsByTagName("item"); // Optimized node selection
for (int i = 0; i < nodeList.getLength(); i++) {
Element item = (Element) nodeList.item(i);
// Process only interested nodes
System.out.println(item.getAttribute("id"));
}
Why This Matters
Processing unnecessary nodes can slow down your application, especially for large XML documents. Being efficient in selecting and processing only what's needed significantly boosts performance.
5. Not Using External Libraries
Pitfall
Reinventing the wheel by writing your XML parsing logic from scratch can lead to code duplication and potential bugs.
Solution
Utilize existing libraries and frameworks. Libraries like JAXB (Java Architecture for XML Binding) simplify XML binding to Java objects.
Code Example: JAXB Usage
import javax.xml.bind.JAXBContext;
import javax.xml.bind.Unmarshaller;
import java.io.File;
public class JAXBExample {
public static void main(String[] args) {
try {
File file = new File("data.xml");
JAXBContext jaxbContext = JAXBContext.newInstance(YourDataClass.class);
Unmarshaller jaxbUnmarshaller = jaxbContext.createUnmarshaller();
YourDataClass data = (YourDataClass) jaxbUnmarshaller.unmarshal(file);
// Now you can work with a Java representation of your XML data
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters
Using well-tested libraries can save development time and improve the reliability of your code. They often come with better performance and support.
To Wrap Things Up
Parsing XML in Java doesn’t have to be a daunting task. By avoiding common pitfalls such as choosing the wrong parsing method, improper error handling, ignoring namespaces, and neglecting performance optimization, you can streamline your XML manipulation process.
For further reading and deeper insights, consider checking out:
- Java XML Parsing Tutorial
- XML Parsing with SAX
- Understanding DOM vs SAX vs StAX
With the right knowledge and tools, you can handle XML parsing efficiently and effectively in your Java applications.