Run Java Apps Portably: Skip the Installation Hassle!
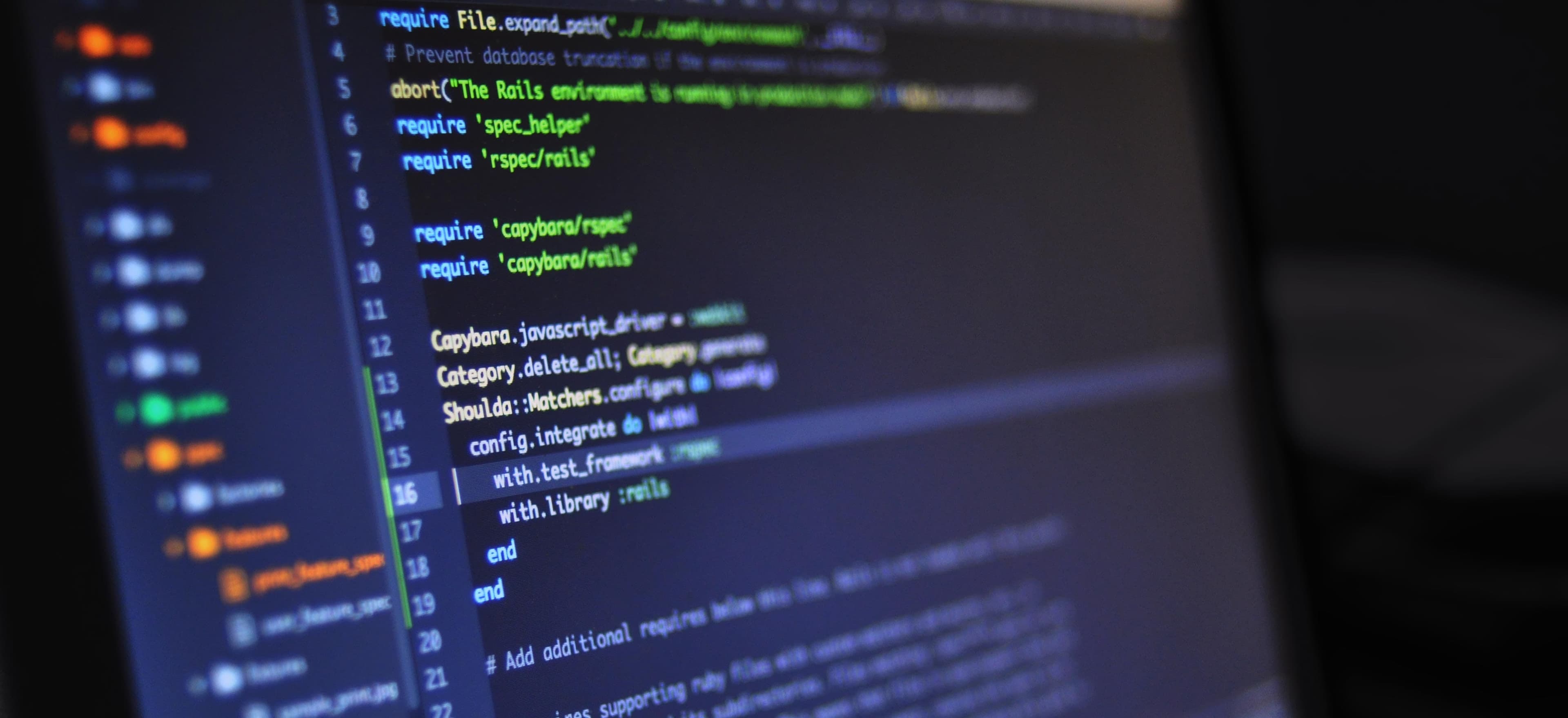
- Published on
Run Java Apps Portably: Skip the Installation Hassle!
In today's fast-paced world, flexibility and convenience are paramount. Imagine if you could run your favorite Java applications without the cumbersome process of installation; that would save time and effort, wouldn't it? Portability in Java applications is not just a dream. It’s achievable! This guide will walk you through everything you need to know about running Java applications portably.
What is Java Portability?
Java was designed to be platform-independent. This means you can write code once and run it anywhere, giving rise to the popular mantra, “Write Once, Run Anywhere”. But what does portability mean in the practical sense?
- It refers to the ability to run a Java application on any machine without needing to install it first.
- It allows you to carry your applications on a USB stick, external hard drive, or even cloud storage, making it easy to use them on different systems.
Why Run Java Apps Portably?
-
Convenience: No need to worry about installation prerequisites, configurations, or possible compatibility issues.
-
Performance: You will experience minimal overhead since the ported version can be optimized for its specific environment.
-
Testing: It enhances the testing process as you can easily transfer your application across different environments.
-
No installations required: This eco-friendly approach means less clutter on systems.
Elements of a Portable Java App
To achieve a portable Java application, you must consider several elements:
-
Bundling JRE: Including a specific Java Runtime Environment (JRE) version with your application ensures that it runs correctly on systems without the required version installed.
-
Using Relative Paths: Avoid absolute paths in application code that may not exist on other systems.
-
Configuration Files: Store configurations in local files that the application can read and modify.
Step-by-Step Guide on Running Java Apps Portably
Here is a practical guide to turn your Java applications into portable applications.
Step 1: Package Your Java Application
Let's start with a simple Java application:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
To compile this code, use the following command in your terminal:
javac HelloWorld.java
Once compiled, you will get a HelloWorld.class
file which can be run using:
java HelloWorld
Step 2: Bundle Your JRE
Why Bundle? Choosing to bundle JRE with your application ensures that it runs smoothly, irrespective of the Java installation on the host machine.
To bundle your JRE:
- Download a JRE distribution.
- Create a folder structure like so:
YourApp/
├── jre/
│ └── (JRE files here)
├── HelloWorld.class
└── run.bat (or run.sh for Linux)
Your application directory now contains the JRE alongside your application class.
Step 3: Create a Run Script
This is an essential step for portability. The script allows the user to run the application without needing additional commands.
Windows Batch File (run.bat):
@echo off
set PATH=%cd%\jre\bin;%PATH%
java HelloWorld
pause
Linux Shell Script (run.sh):
#!/bin/bash
export PATH=$(pwd)/jre/bin:$PATH
java HelloWorld
Why Use Scripts? Scripts streamline the process. They automatically set the necessary environment before running your application.
Make sure to give execution permissions to your shell script:
chmod +x run.sh
Step 4: Avoid Absolute Paths
Using absolute file paths in your application can quickly lead to portability issues. Instead, rely on relative paths. Here’s an example of how to read a file in a portable way:
import java.io.*;
public class PortableApp {
public static void main(String[] args) {
try {
File file = new File("config.properties");
BufferedReader reader = new BufferedReader(new FileReader(file));
System.out.println("Reading file: " + file.getAbsolutePath());
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this snippet, config.properties is expected to be within the same directory as your executable. The use of relative paths ensures the application functions as intended across different environments.
Step 5: Test the Portable Application
Before distribution, it's essential to test your application on various configurations:
- Different Operating Systems: Run it on Windows, Linux, and macOS.
- Different Java Versions: Ensure compatibility with the bundled JRE.
Use Virtual Machines or containers for hassle-free testing. Tools like Docker can simulate environments efficiently.
Distributing Your Ported Java Application
Once tested, you may want to share your portable Java application. Package everything into a ZIP file and create clear instructions for users.
- Documentation: Provide a README file explaining how to run the application using the run script.
- Licensing: If applicable, provide relevant licensing information and source code.
Lessons Learned
Running Java applications portably can significantly enhance convenience and flexibility. By following the steps outlined in this guide, you can skip the installation hassle and carry your Java apps anywhere with ease. From packaging JRE to using relative paths, each step contributes to a smooth running experience on various systems.
As you embrace the benefits of portability, remember to stay ahead of updates in the Java ecosystem. The Java Community Process is an excellent resource for discovering new advancements.
Feel free to reach out and share your experiences or questions regarding creating portable Java applications. Happy coding!