Mitigating Threats in Data Flow Diagrams Effectively
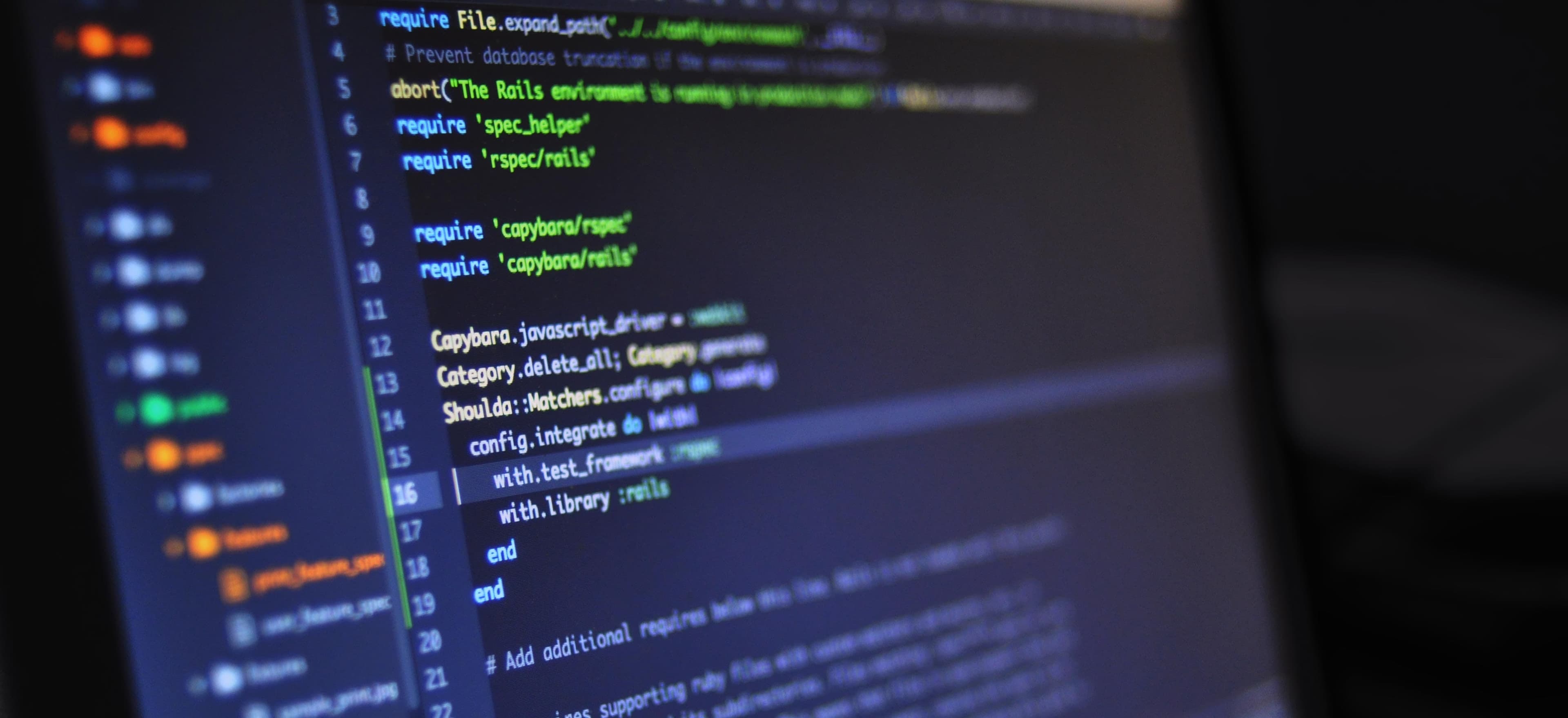
- Published on
Mitigating Threats in Data Flow Diagrams Effectively
Data flow diagrams (DFDs) play a crucial role in understanding the flow of information within a system. As a representation of data movement, they help engineers, analysts, and stakeholders visualize how data enters, processes, and exits an application. However, as with all system design artifacts, DFDs must consider security threats to safeguard sensitive information. This blog post guides you through the process of recognizing and mitigating threats in data flow diagrams effectively.
What is a Data Flow Diagram?
Data flow diagrams are visual representations that depict the flow of data in a system. They consist of:
- Processes: Represented by circles or ovals that transform input data into output data.
- Data Stores: Rectangular boxes that hold the data generated or used by processes.
- Data Flows: Arrows indicate how data moves between processes, data stores, and external entities.
- External Entities: Sources or destinations for data, typically shown as squares, that interact with the system.
Here's a basic example of a DFD:
[External Entity] --> [Process] --> [Data Store]
In understanding DFDs, it is essential to acknowledge that they describe how information flows rather than their actual implementation. This abstraction provides a clearer picture of potential vulnerabilities.
Recognizing Common Threats
Before diving into mitigation strategies, it is crucial to identify common threats associated with data flow. Some notable threats include:
- Unauthorized Access: External entities accessing data stores or processes without proper authorization.
- Data Leakage: Sensitive data being exposed or transmitted insecurely, following poorly defined flows.
- Data Corruption: Malicious modification of data during transit or storage.
- Denial of Service (DoS): Overloading processes or data stores that lead to unavailability.
- Replay Attacks: Intercepting and reusing valid data flows to perform unauthorized actions.
By identifying these threats, teams can take proactive steps to enhance security.
Mitigation Strategies
1. Implementing Access Controls
Access controls restrict who can interact with processes and data stores. To protect your DFD:
- Role-Based Access Control (RBAC): Assign permissions based on user roles, ensuring users can only access what is necessary for their functions.
- Least Privilege Principle: Grant users the minimum level of access required, which can significantly reduce the attack surface.
public class UserAccess {
private List<String> roles;
public UserAccess(List<String> roles) {
this.roles = roles;
}
public boolean hasAccess(String resource) {
return roles.contains(determineRole(resource));
}
private String determineRole(String resource) {
// Logic to determine the role based on the requested resource
return "";
}
}
In the code snippet above, the UserAccess
class manages user permissions dynamically. Maintaining user roles enables fine-grained control over access to critical data flow components.
2. Securing Data Transmission
Data transmission can be vulnerable to threats such as interception or leakage. Implementing encryption is a robust mitigation strategy:
- SSL/TLS: Secure communication channels using Transport Layer Security. Encrypt data in transit to prevent eavesdropping.
- Data Masking: Mask sensitive information when displayed or transmitted.
Example of utilizing encryption in Java:
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class EncryptionExample {
public static byte[] encrypt(String data) throws Exception {
SecretKey key = KeyGenerator.getInstance("AES").generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data.getBytes());
}
}
The code above utilizes the Java Cryptography Architecture (JCA) to encrypt data using AES encryption. This addition to your DFD can greatly enhance security by ensuring that even if data is intercepted, it remains unreadable.
3. Data Integrity Measures
Ensuring data integrity is vital to prevent corruption. Measures to consider include:
- Checksumming: Generating a checksum when data is created and comparing it during retrieval ensures that data has not been tampered with.
- Digitally Signing Data: Using signatures to authenticate the source and ensure that data has not been altered in transit.
A simple checksumming implementation in Java:
import java.security.MessageDigest;
public class DataIntegrity {
public static String generateChecksum(String input) throws Exception {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(input.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
}
}
In this code, generateChecksum
creates a SHA-256 hash of the input data, which can be used to validate data integrity effectively.
4. Monitoring and Logging
Monitoring interactions with data flow components can help detect suspicious patterns:
- Intrusion Detection Systems (IDS): Monitor network traffic for unusual activities.
- Audit Logs: Maintain logs of access and transactions to identify potential breaches or unauthorized access.
Here is a sample logging utility in Java:
import java.util.logging.Logger;
public class LoggingUtility {
private static final Logger LOGGER = Logger.getLogger(LoggingUtility.class.getName());
public static void logAccess(String message) {
LOGGER.info(message);
}
public static void logError(String message) {
LOGGER.severe(message);
}
}
This utility class provides basic logging functionality that could be used to track operations related to the DFD.
My Closing Thoughts on the Matter
Data flow diagrams are essential tools for understanding and designing information systems. However, without vigilant threat mitigation strategies, they can become pathways for vulnerabilities. By implementing access controls, securing data transmission, ensuring integrity, and establishing monitoring protocols, organizations can fortify their data flow diagrams against potential threats.
For further reading, consult the following resources:
- OWASP Secure Coding Practices
- Understanding Data Flow Diagrams
By following these guidelines and staying proactive, you can ensure that your data flow diagrams serve their intended purpose without compromising data security.
Checkout our other articles