Integrating Java Backends for React Native Barcode Solutions
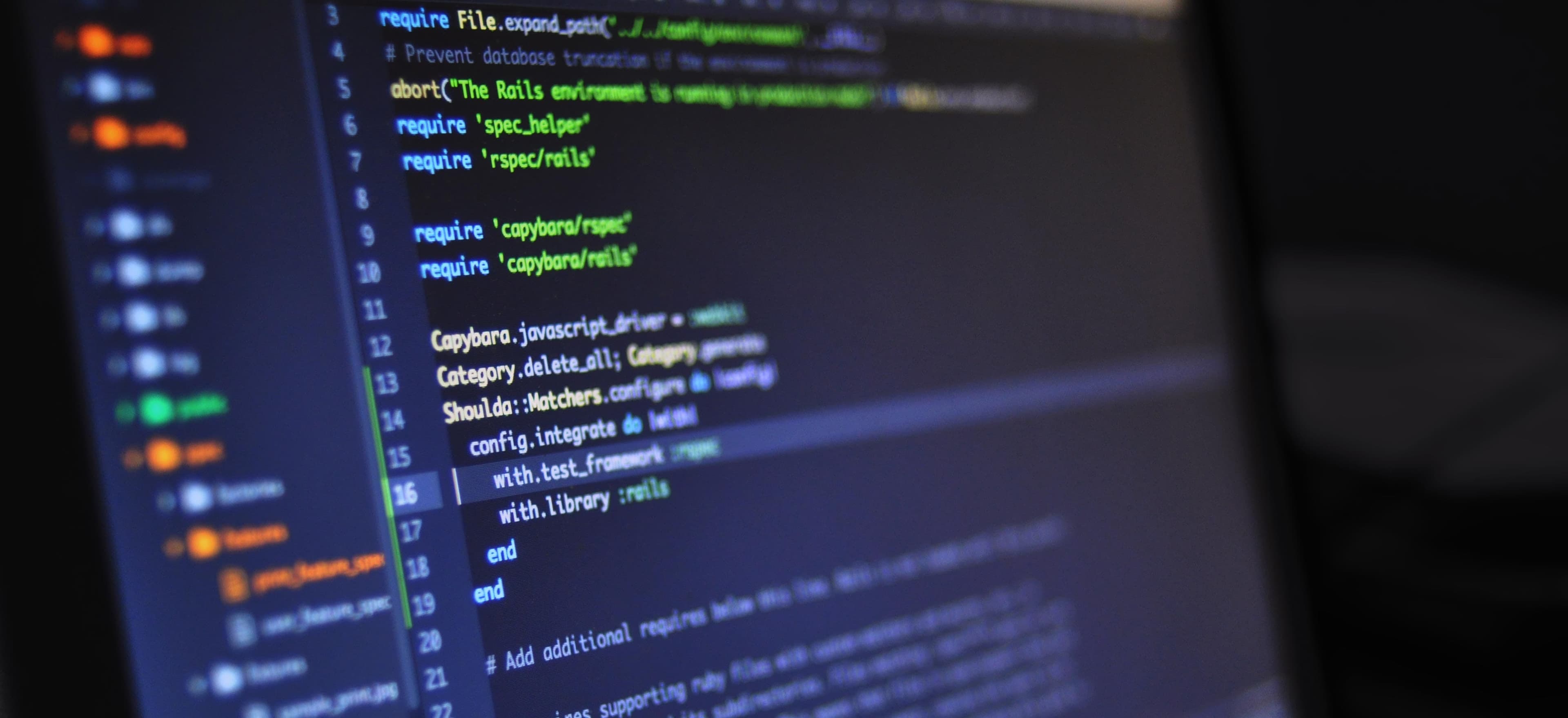
- Published on
Integrating Java Backends for React Native Barcode Solutions
As mobile applications proliferate in our technology-driven society, the growing need for effective backend services becomes increasingly apparent. This rings especially true for applications that implement barcode scanning features. Integrating a Java backend with a React Native frontend can provide a seamless experience for users requiring barcode-related functionalities.
In this blog post, we will explore how to set up a Java backend for a React Native application specifically designed for barcode processing. We will also reference a helpful article titled Mastering React Native: Building a Barcode Widget as a foundational piece for understanding barcode widget development.
Understanding the Components
What is React Native?
React Native is a powerful framework that allows developers to build mobile applications using JavaScript and React. One of its strengths is the capacity to leverage native components for user interface performance while maintaining a codebase that’s largely consistent across platforms (Android and iOS).
The Role of a Java Backend
Java is a robust and widely-used programming language, particularly in enterprise environments. Its reliability, security features, and extensive libraries make it an excellent choice for backend development. By implementing a Java backend, you can manage various services, including database connections, user authentication, and barcode processing logic.
Setting Up Your Java Backend
Before diving into code, let's outline the high-level steps involved in setting up your Java backend.
- Install Java: Ensure you have the Java Development Kit (JDK) installed on your machine.
- Choose a Framework: Popular frameworks like Spring Boot can simplify the setup process. For this tutorial, we will use Spring Boot for its ease of use.
- Set Up Dependencies: Set up Maven or Gradle dependencies required for your project.
- Create RESTful Services: Define your REST controllers to handle barcode-related requests.
- Integrate with a Database: Optionally, you might want to store barcode information in a database.
Step 1: Install Java and Spring Boot
First, you’ll need to install the Java Development Kit (JDK). Follow the instructions on the official Oracle website to get the necessary files. You will also install Spring Boot, which you can bootstrap using Spring Initializr.
Sample Project Structure
Once you have your Java environment set up, creating a new Spring Boot project will generate a structure along these lines:
src
└── main
├── java
│ └── com
│ └── example
│ └── barcodeapp
│ ├── BarcodeController.java
│ └── Application.java
└── resources
└── application.properties
Step 2: Building a Simple Barcode Controller
Let’s create a BarcodeController
that will handle barcode scan requests.
BarcodeController.java
package com.example.barcodeapp;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/barcode")
public class BarcodeController {
@GetMapping("/{barcode}")
public ResponseEntity<String> getBarcodeDetails(@PathVariable String barcode) {
// Simulated barcode processing logic
String barcodeDetails = "Details of barcode: " + barcode;
// Return details
return ResponseEntity.ok(barcodeDetails);
}
}
Why This Code?
@RestController
: This annotation indicates that the class is a controller where every method returns a domain object instead of a view.@RequestMapping("/api/barcode")
: It defines a base URL for the controller.@GetMapping("/{barcode}")
: This annotation ensures that requests to this method are handled for GET requests containing a barcode as part of the URL.ResponseEntity<String>
: This allows you to build responses with more flexibility, such as HTTP status codes.
Step 3: Configuring the Application Properties
Next, we will configure the application.properties
file to set up any required configurations.
application.properties
server.port=8080
spring.datasource.url=jdbc:mysql://localhost:3306/barcode_db
spring.datasource.username=root
spring.datasource.password=password
Why This Configuration?
server.port
: This specifies the port on which your Spring Boot application will run.spring.datasource
parameters: These configurations are required for setting up database connections. You can adapt them to your database setup.
React Native Communication with Java Backend
Setting Up the React Native Frontend
Now that we have our backend ready, we can focus on connecting it with a React Native application. If you’re starting from scratch, use the command below to create a new React Native project:
npx react-native init barcodeScannerApp
Fetching Barcode Data in React Native
Let’s create a simple component that fetches the details of a barcode using our Java backend.
BarcodeScanner.js
import React, { useState } from 'react';
import { View, Text, Button, TextInput } from 'react-native';
const BarcodeScanner = () => {
const [barcode, setBarcode] = useState('');
const [details, setDetails] = useState('');
const fetchBarcodeDetails = async () => {
try {
const response = await fetch(`http://localhost:8080/api/barcode/${barcode}`);
const data = await response.text();
setDetails(data);
} catch (error) {
console.error('Error fetching barcode details:', error);
}
};
return (
<View>
<TextInput
placeholder="Enter Barcode"
value={barcode}
onChangeText={setBarcode}
/>
<Button title="Fetch Details" onPress={fetchBarcodeDetails} />
<Text>{details}</Text>
</View>
);
};
export default BarcodeScanner;
Why This Component?
- TextInput: This allows users to input a barcode for fetching its details.
- Button: When pressed, this triggers the function to fetch barcode data.
- fetch: The fetch API sends a GET request to your Java backend to retrieve barcode details.
Testing the Connection
Ensure your Java backend is running on localhost at port 8080 and load your React Native app using:
npx react-native run-android // for Android
npx react-native run-ios // for iOS
Input a barcode in the provided TextInput and hit "Fetch Details" to see the response from your backend.
Summary
In this post, we demonstrated how to establish a Java backend to handle barcode-related requests and connect it to a React Native frontend. By bridging these technologies, you create a powerful application capable of leveraging barcode functionalities.
If you're looking for a deeper dive into barcode integration within React Native, be sure to check Mastering React Native: Building a Barcode Widget.
Additional Resources
For further learning, consider reading the following resources:
By mastering these integrations, you will be well on your way to creating efficient and user-friendly applications in the modern tech landscape. Happy coding!
Checkout our other articles