Handling Java Garbage Collection in High-Demand Scenarios
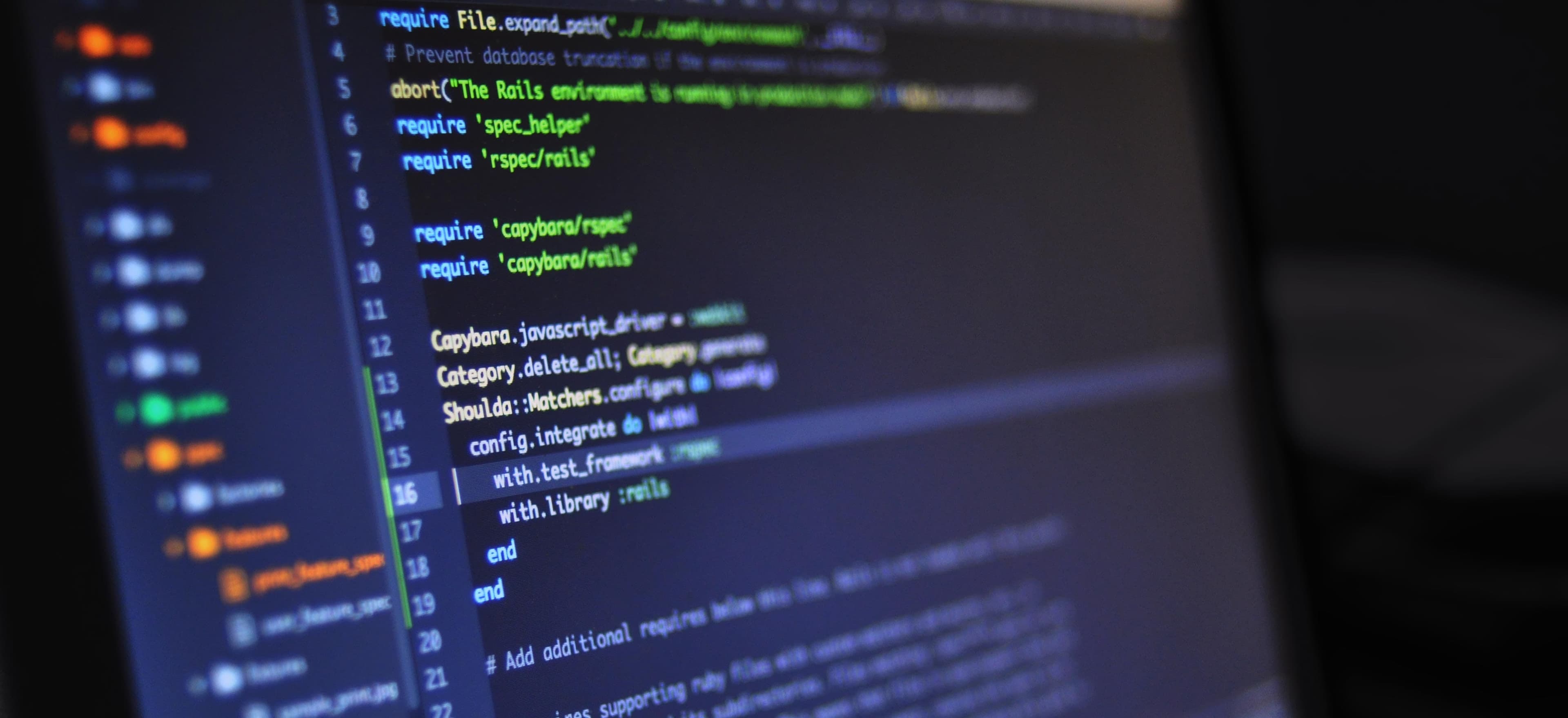
- Published on
Handling Java Garbage Collection in High-Demand Scenarios
Java developers often face the intricate challenge of managing memory efficiently, especially when dealing with high-demand scenarios. Garbage Collection (GC) is one of Java’s most powerful features, designed to automatically reclaim memory used by objects that are no longer needed. However, in high-load situations, the very system designed to optimize performance can turn into a bottleneck. In this blog post, we will explore how to handle Java Garbage Collection effectively, especially in demanding applications.
Understanding Garbage Collection in Java
Garbage Collection in Java handles deallocating memory for objects that are no longer in use. This frees developers from manual memory management tasks, reducing memory leaks and fragmentation. However, GC also has performance implications, particularly in systems with high memory usage.
GC Basics
Java has several types of garbage collectors. The most prominent include:
- Serial Garbage Collector: A simple collector that uses a single thread for garbage collection.
- Parallel Garbage Collector: Uses multiple threads for managing heap space, suitable for multi-core machines.
- Concurrent Mark-Sweep (CMS): Divides garbage collection into phases, reducing pause times.
- G1 Garbage Collector: Designed for applications with large heaps and low latency requirements.
Choosing the right garbage collector for your application can make a significant difference in performance.
Challenges in High-Demand Scenarios
In scenarios where you have numerous simultaneous users or large data processing, garbage collection can become problematic. Common issues include:
- Long GC Pauses: These can occur at inopportune moments, affecting user experience.
- Memory Leaks: If objects are not correctly dereferenced, memory can be consumed unnecessarily, leading to OutOfMemoryErrors.
- Increased Throughput: As the load increases, the frequency of garbage collection may rise, impacting overall application performance.
Example of GC Pause
Consider a web application with a sudden spike in user traffic. If the application uses the default garbage collector, a long GC pause could disrupt service. Below is a simplified example to illustrate:
public class TrafficSimulator {
public static void main(String[] args) {
while (true) {
simulateTraffic();
try {
Thread.sleep(1000); // Simulates time between traffic spikes
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private static void simulateTraffic() {
// Simulates costly object creation during high user demand
for (int i = 0; i < 10000; i++) {
new Object();
}
}
}
Analysis of GC Effects
In the example above, as the number of user requests grows, the application repeatedly creates new objects, leading to frequent garbage collections. This is a straightforward illustration of how GC can slow down performance during high-load scenarios.
Strategies to Optimize Garbage Collection
Mitigating the challenges of garbage collection requires carefully strategizing and implementing several best practices. Here are some effective methods:
1. Choose the Right Garbage Collector
Selecting the appropriate garbage collector based on your application's requirements is crucial. For low-latency applications, consider using the G1 collector or the Z Garbage Collector (ZGC), which is designed for environments needing low pause times.
2. Tune JVM Parameters
Java allows tuning heap sizes and other parameters to optimize GC performance. For example:
java -Xms512m -Xmx4g -XX:+UseG1GC -XX:MaxGCPauseMillis=200 -jar MyApplication.jar
Here, -Xms sets the initial heap size, -Xmx sets the maximum heap size, and -XX:MaxGCPauseMillis is used to specify the desired maximum pause time for G1 GC.
3. Analyze Memory Usage
Use tools like Java VisualVM or JProfiler to visualize memory usage over time. By identifying memory consumption patterns, you can make informed decisions about optimizations.
Example Snippet to Monitor Memory
public class MemoryMonitor {
public static void main(String[] args) {
Runtime runtime = Runtime.getRuntime();
long memoryUsed = runtime.totalMemory() - runtime.freeMemory();
System.out.println("Memory used: " + memoryUsed / 1024 / 1024 + " MB");
}
}
4. Minimize Object Creation
In high-demand scenarios, reducing the number of objects can dramatically impact GC performance. Employ object pooling or reusing existing objects when possible.
Object Pooling Example
import java.util.ArrayList;
import java.util.List;
public class ObjectPool {
private List<Object> availableObjects = new ArrayList<>();
public Object borrowObject() {
if (availableObjects.isEmpty()) {
return new Object(); // Create new if pool is empty
}
return availableObjects.remove(availableObjects.size() - 1);
}
public void returnObject(Object obj) {
availableObjects.add(obj);
}
}
Monitor and Adjust Regularly
Regular monitoring of memory usage and garbage collection metrics will help you stay ahead of potential issues. Incorporate tools like Elasticsearch, Logstash, and Kibana (ELK Stack) to allow real-time monitoring and analysis.
Closing the Chapter
Handling Java garbage collection in high-demand scenarios is an art that blends the right tools, techniques, and an understanding of the underlying principles. By choosing the appropriate garbage collector, tuning JVM parameters, reducing object creation, and engaging in continuous monitoring, developers can mitigate the adverse effects of garbage collection on application performance.
For more insights into memory management challenges in extreme situations, you may want to explore the article titled "Post-Apocalyptic Waste Management Challenges". This will deepen your understanding of resource management in unique and demanding contexts.
By adopting the strategies shared in this post, Java developers can better prepare for the demands of modern software applications and ensure smooth, uninterrupted service. Happy coding!
Checkout our other articles