Java Solutions for Efficient Waste Management in Crisis
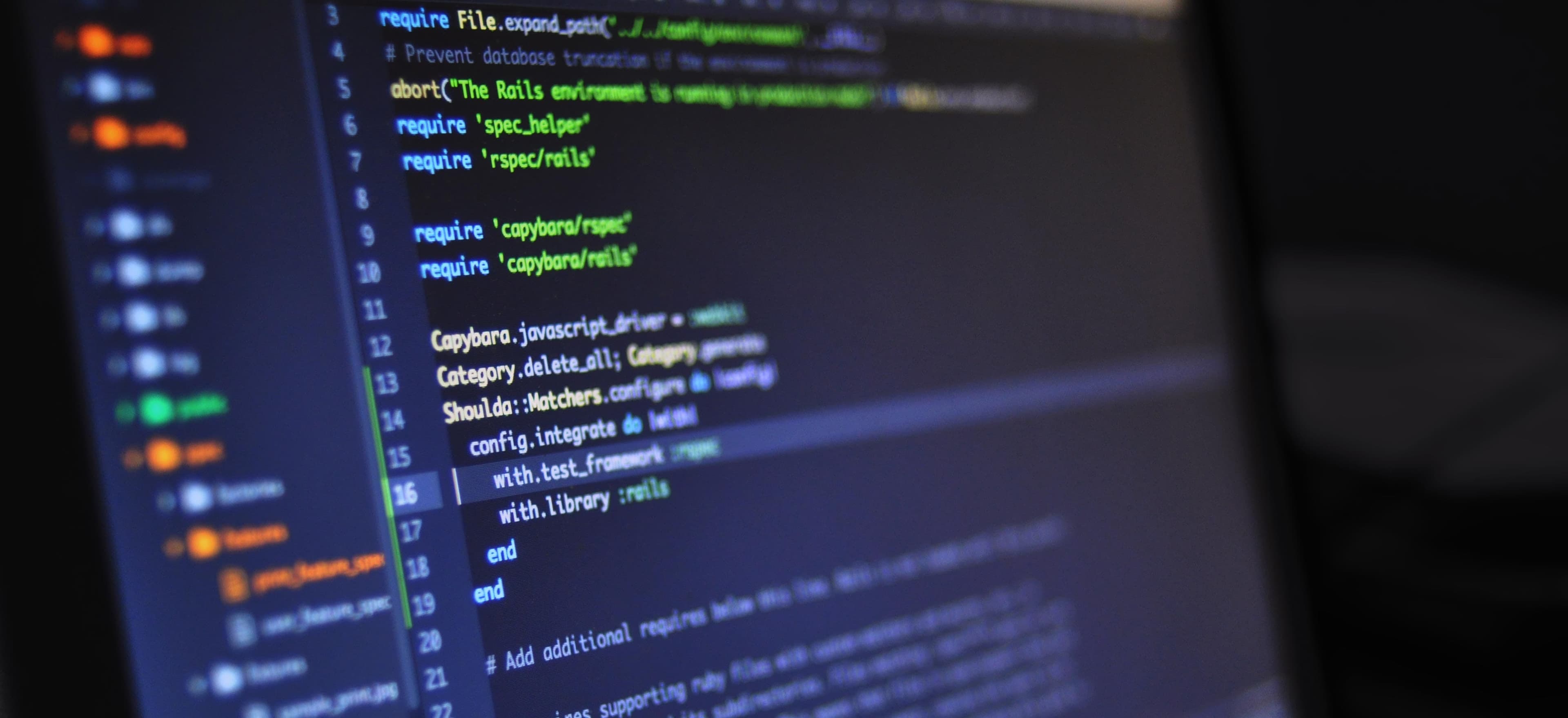
- Published on
Java Solutions for Efficient Waste Management in Crisis
In recent times, efficient waste management has emerged as a critical issue, especially in crisis situations. Just imagine a post-apocalyptic world, as discussed in the Post-Apocalyptic Waste Management Challenges. In such scenarios, effective systems must be implemented to handle waste effectively and sustainably. Today, we will explore how Java can be utilized to develop efficient waste management systems that can be deployed even during challenging times.
The Importance of Waste Management in Crisis
Waste management is crucial in any society, but its importance multiplies in a crisis scenario. Poor waste disposal leads to environmental degradation, public health issues, and can exacerbate the situation at hand. Therefore, a well-thought-out strategy becomes a necessity.
Java, a robust programming language known for its portability and performance, can be tailored to create systems that manage waste more efficiently. By leveraging Java's characteristics, we can build solutions that will help in waste sorting, recycling, tracking, and disposal.
Core Challenges in Waste Management
Before jumping into Java solutions, let’s address some core challenges:
- Inventory Tracking: Knowing what waste is being generated and where it is fated for disposal.
- Sorting Efficiency: Quickly and accurately sorting waste into appropriate categories.
- Resource Recovery: Identifying materials that can be recycled and reused.
- Data Management: Keeping track of waste statistics for informed decision-making.
With these in mind, we can design a systematic approach to tackle the challenges effectively.
Designing a Java-based Waste Management System
We will outline a simple Java-based solution for a waste management system that encompasses the challenges mentioned above.
Step 1: Define Waste Classes
To manage different types of waste, we can create Java classes that represent different waste types. For example:
public abstract class Waste {
protected String type;
protected int weight; // in kilograms
public Waste(String type, int weight) {
this.type = type;
this.weight = weight;
}
public abstract void recycle();
public String getType() {
return type;
}
public int getWeight() {
return weight;
}
}
public class PlasticWaste extends Waste {
public PlasticWaste(int weight) {
super("Plastic", weight);
}
@Override
public void recycle() {
System.out.println("Recycling plastic waste of weight: " + weight + " kg");
}
}
Why This Code?
By defining an abstract Waste
class and extending it for different categories, we manage waste in a categorized manner. The abstract method recycle()
enables each waste type to define its recycling protocol, ensuring flexibility and adherence to the specific needs of various waste kinds.
Step 2: Implement a Waste Sorting System
Next, we establish a system that can receive waste and sort it accordingly. Here is an example of how to create a simple sorting algorithm:
import java.util.ArrayList;
import java.util.List;
public class WasteManager {
private List<Waste> wastes;
public WasteManager() {
wastes = new ArrayList<>();
}
public void addWaste(Waste waste) {
wastes.add(waste);
}
public void sortWaste() {
for (Waste waste : wastes) {
waste.recycle(); // the specific recycling method of each waste type
}
}
public void printWasteSummary() {
System.out.println("Waste Summary:");
// ... code to print waste details
}
}
Why This Code?
This code snippet highlights how easy it is to manage multiple waste items. With a List to hold various waste types, the sortWaste()
method iterates through the list, calling the specific recycling method for each type of waste added. This flexibility is fundamental in a real-world scenario where various waste items arrive consistently.
Step 3: Implement Tracking with Databases
To facilitate data management efficiently, we can integrate Java with a database. Here is a rudimentary implementation using JDBC (Java Database Connectivity):
import java.sql.*;
public class DatabaseHandler {
private Connection connection;
public DatabaseHandler(String dbUrl, String user, String password) throws SQLException {
connection = DriverManager.getConnection(dbUrl, user, password);
}
public void addWasteToDatabase(Waste waste) throws SQLException {
String query = "INSERT INTO waste_management (type, weight) VALUES (?, ?)";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, waste.getType());
preparedStatement.setInt(2, waste.getWeight());
preparedStatement.executeUpdate();
System.out.println("Waste added to database: " + waste.getType());
}
public void close() throws SQLException {
if (connection != null) {
connection.close();
}
}
}
Why This Code?
Using JDBC allows the application to log waste data persistently. The addWasteToDatabase()
method inserts waste data into a database, providing a reliable way to track statistics over time. This information is essential for making informed decisions about resource recovery and waste management strategies.
My Closing Thoughts on the Matter
The essence of an effective waste management strategy, especially in crisis situations, lies in efficient tracking, sorting, and recycling of materials. Utilizing Java, we can create robust systems tailored to tackle waste management challenges.
With the use of classes that represent different waste types, sorting systems that articulate specific recycling methods, and database integration for tracking and analysis, Java proves to be a formidable ally in establishing efficient waste management solutions, even in apocalyptic scenarios.
As highlighted in the existing article "Post-Apocalyptic Waste Management Challenges," we should always be ready to adapt our approaches to meet the needs of any situation. By implementing Java-based solutions, we can turn potential challenges into opportunities for sustainability and innovation in waste management.
Further Reading
For more in-depth insights into the challenges surrounding waste management, do check out the article "Post-Apocalyptic Waste Management Challenges" here.
With the correct systems in place, even the most daunting waste management challenges can be tackled!
Checkout our other articles