Java Solutions for Waste Management in Post-Apocalyptic Scenarios
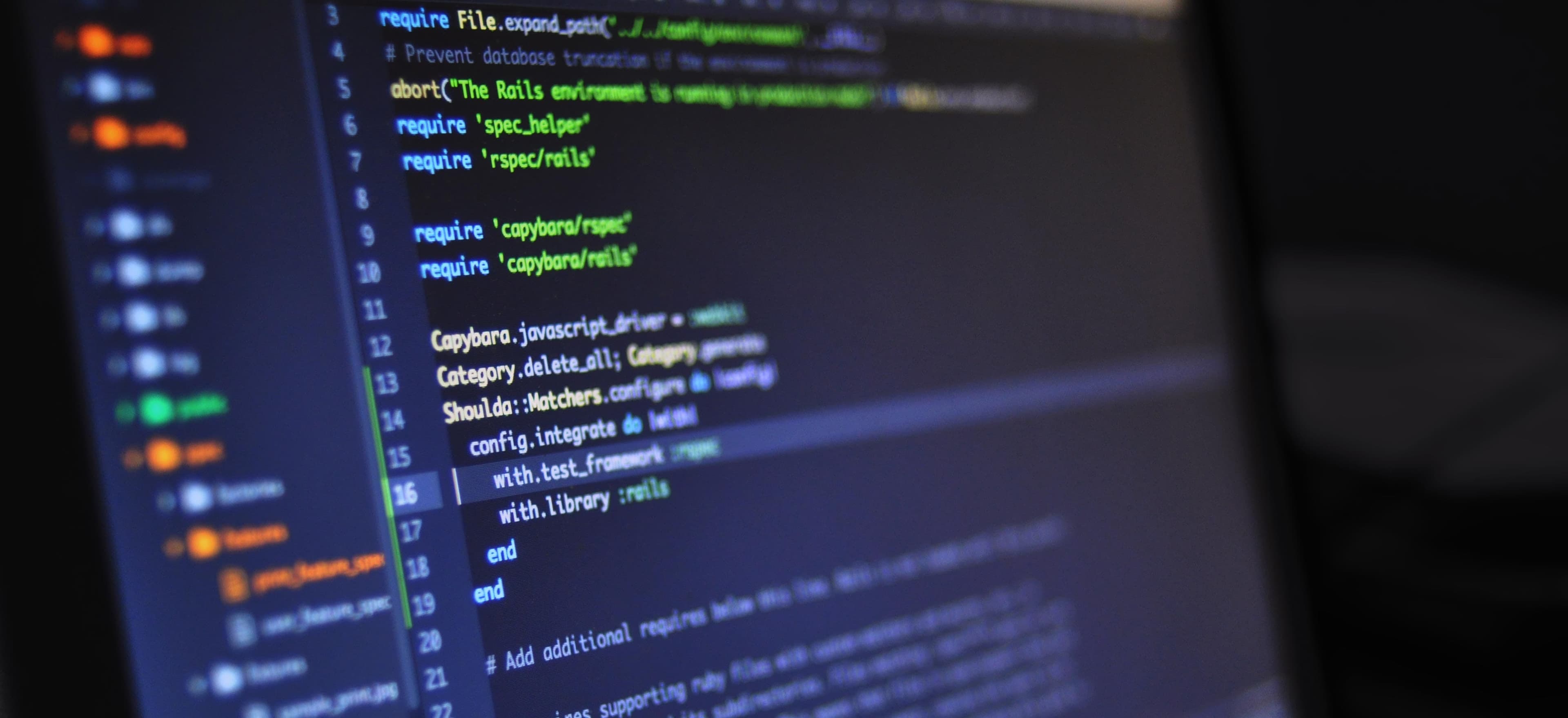
- Published on
Java Solutions for Waste Management in Post-Apocalyptic Scenarios
In a world faced with dwindling resources and ever-increasing waste, the challenges of waste management become paramount. This is especially relevant in post-apocalyptic scenarios, where infrastructures are obliterated, and traditional waste management systems are no longer viable. Java, a robust programming language known for its portability and performance, can offer innovative solutions to these complex situations. In this blog post, we will explore how Java can be utilized for waste management in such scenarios and provide detailed code examples.
Understanding Waste Management Challenges
Waste management is not merely about disposal; it's a comprehensive process that involves collection, transportation, processing, recycling, and disposal. In a post-apocalyptic environment, these challenges are compounded by logistical issues, environmental hazards, and societal breakdowns. Post-Apocalyptic Waste Management Challenges dives deeper into these complexities, highlighting how critical it is to approach waste management thoughtfully.
Key Challenges
- Resource Scarcity: Limited access to resources makes traditional waste management solutions ineffective.
- Logistical Issues: Transportation of waste becomes dangerous and complicated.
- Public Health and Safety: Improper waste management can lead to public health crises.
- Environmental Awareness: Even in a crisis, we must cultivate a sense of responsibility for the environment.
Java's Role in Waste Management
Java can be pivotal in addressing these challenges through various applications, including mobile apps, data analysis, and decision support systems. Let's explore how these applications can be developed using Java.
Java Mobile Applications
In post-apocalyptic scenarios, mobile applications can be critical for community coordination. They can help track waste locations, facilitate recycling, and distribute responsibilities among survivors.
Example: Tracking Waste with a Simple Java Application
import java.util.ArrayList;
import java.util.HashMap;
public class WasteTracker {
private HashMap<String, ArrayList<String>> wasteMap;
public WasteTracker() {
wasteMap = new HashMap<>();
}
// Method to add waste locations
public void addWasteLocation(String area, String wasteType) {
wasteMap.putIfAbsent(area, new ArrayList<>());
wasteMap.get(area).add(wasteType);
}
// Method to display waste locations
public void displayWasteLocations() {
for (String area : wasteMap.keySet()) {
System.out.println("Area: " + area + " | Waste Types: " + wasteMap.get(area));
}
}
public static void main(String[] args) {
WasteTracker tracker = new WasteTracker();
tracker.addWasteLocation("Downtown", "Plastic");
tracker.addWasteLocation("Downtown", "Organic");
tracker.addWasteLocation("Uptown", "Hazardous");
tracker.displayWasteLocations();
}
}
Why this code? This simple Java application enables the community to log and track waste types in different areas. Using a HashMap for efficient storage provides a quick lookup for waste locations, which is essential for better resource allocation.
Data Analysis for Waste Management
Another critical aspect is analyzing waste data to make informed decisions. Java excels in data handling, thanks to libraries like Apache Commons and Java Streams.
Example: Analyzing Waste Metrics
import java.util.Arrays;
import java.util.List;
import java.util.OptionalDouble;
public class WasteAnalytics {
// Method to calculate average waste per area
public static double calculateAverageWaste(List<Integer> wasteAmounts) {
OptionalDouble average = wasteAmounts.stream().mapToInt(i -> i).average();
return average.orElse(0);
}
public static void main(String[] args) {
List<Integer> wasteData = Arrays.asList(100, 200, 150, 300, 250);
double averageWaste = calculateAverageWaste(wasteData);
System.out.println("Average Waste: " + averageWaste + "kg");
}
}
Why this code? By utilizing Java Streams, this code snippet efficiently calculates the average waste produced in a designated area. It provides insights into waste production, allowing for targeted interventions.
Decision Support Systems
A decision support system can guide leaders in resource allocation and strategy formulation. This is crucial in crisis management scenarios, where every decision can significantly impact survival.
Example: Simple Decision Support with Java
import java.util.Scanner;
public class DecisionSupport {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter current waste level (1-10): ");
int wasteLevel = scanner.nextInt();
if (wasteLevel > 7) {
System.out.println("Action Required: Implement cleanup operations!");
} else {
System.out.println("Waste levels are manageable.");
}
scanner.close();
}
}
Why this code? This basic decision support system evaluates waste levels to determine if action is necessary. Even in a simplified form, it emphasizes the importance of quick decision-making based on data.
Enhancing Community Engagement
Engaging the community fosters a collective responsibility for waste management. Creating online forums or chat applications using Java can encourage sharing of best practices, recycling tips, and waste reduction strategies.
The Closing Argument
In summary, Java provides multiple avenues to enhance waste management in post-apocalyptic scenarios through mobile applications, data analysis, and decision support systems. As we grapple with the complexities of waste management, it's crucial to tap into technological solutions that not only make processes efficient but also engage and empower communities.
For further reading, don't forget to check out the Post-Apocalyptic Waste Management Challenges article. It offers a comprehensive examination of the myriad challenges and potential strategies to tackle waste management in dire circumstances.
With the right tools and community involvement, we can develop a more sustainable approach to waste management, even in the most challenging conditions. Whether you're a seasoned Java developer or a novice, consider the vast potential that lies in applying your skills to make a meaningful difference in waste management.
Checkout our other articles