Solving Media Query Issues in JavaFX WebView
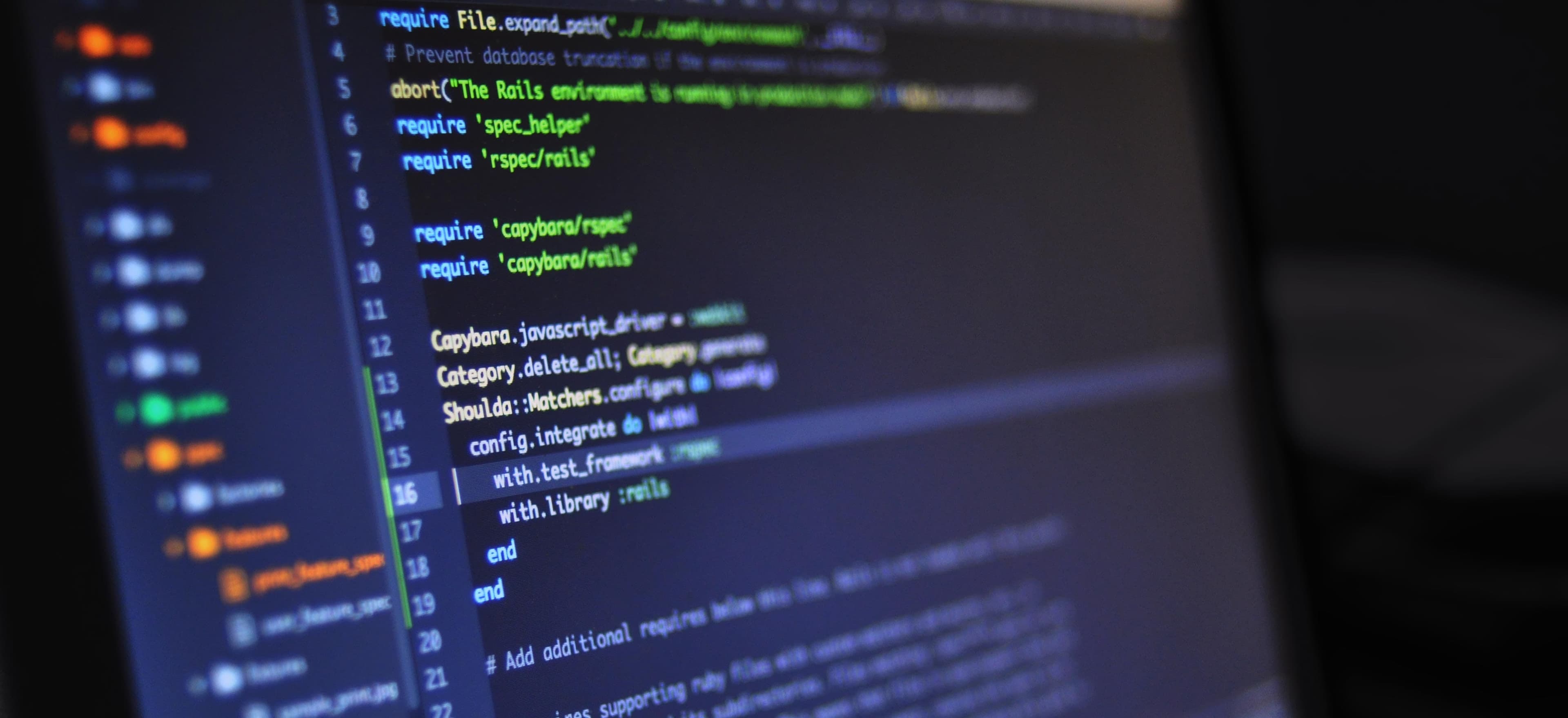
- Published on
Solving Media Query Issues in JavaFX WebView
JavaFX is a powerful framework for building rich desktop applications in Java. One of its notable features is the WebView
component, which allows Java applications to display web content. However, when it comes to responsive design and handling media queries, developers often encounter challenges. This post will outline common issues with media queries in JavaFX's WebView and provide practical solutions to ensure that your web content adapts beautifully across varying display devices.
Understanding Media Queries
Media queries are a critical component of responsive web design. They allow developers to apply CSS styles based on the conditions of the viewport, such as its width, height, or resolution. Here's an example of a basic media query:
@media (max-width: 600px) {
body {
background-color: lightblue;
}
}
In this code snippet, if the viewport width is 600 pixels or smaller, the background color of the body changes to light blue.
The Challenge with JavaFX WebView
While web content runs smoothly in WebView, you may find that media queries do not function as expected. The reason behind this stems from WebView using a different rendering engine—WebKit—which occasionally leads to inconsistencies when interpreting CSS.
Specifically, the JavaFX WebView might not recognize styling changes at certain viewport sizes due to how it calculates the dimensions of the rendered web page, especially in older Java versions.
Practical Solutions to Media Query Issues in JavaFX WebView
1. Set the Correct Initial Size
The first step towards resolving media queries issues is to ensure that WebView is initialized with the appropriate dimensions. JavaFX does have a setPrefSize
method, and it is essential to use it correctly.
WebView webView = new WebView();
webView.setPrefSize(800, 600); // Set the preferred size to a common desktop resolution
Setting an appropriate size might help the content to load in a way that respects the media queries defined in your CSS.
2. Use the Correct Viewport Meta Tag
Another common issue is related to the viewport not being set correctly. The HTML meta viewport tag is fundamental for responsive designs to work. Make sure to include the following tag in the HTML you load into your WebView:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
This line ensures that the viewport width is set to the device width and helps the media queries apply CSS correctly.
3. An Example of Efficient Implementation
Here’s a complete example of how to load a simple HTML document with media queries into JavaFX’s WebView
.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.web.WebEngine;
import javafx.scene.web.WebView;
import javafx.stage.Stage;
public class MediaQueryWebView extends Application {
@Override
public void start(Stage stage) {
WebView webView = new WebView();
WebEngine webEngine = webView.getEngine();
webEngine.loadContent(getHtmlContent());
Scene scene = new Scene(webView);
stage.setScene(scene);
stage.setTitle("Media Queries in JavaFX WebView");
stage.show();
}
private String getHtmlContent() {
// Create a simple HTML with media queries
return "<html>" +
"<head>" +
"<meta name='viewport' content='width=device-width, initial-scale=1.0'>" +
"<style>" +
"body { font-family: Arial, sans-serif; }" +
"@media (max-width: 600px) {" +
"body { background-color: lightblue; }" +
"}" +
"</style>" +
"</head>" +
"<body>" +
"<h1>Responsive Design Example</h1>" +
"<p>Resize the window to see the effect of the media query!</p>" +
"</body>" +
"</html>";
}
public static void main(String[] args) {
launch(args);
}
}
In this example, the WebView
is initialized, a viewport meta tag is included, and media queries are tested. Resize the application window, and watch how the background color changes, confirming that your media queries work as expected.
4. Debugging with Developer Tools
If you experience further issues with media queries not working, it can sometimes help to debug the CSS directly within the WebView. Right-clicking and selecting "Inspect" (if available) can expose helpful information. You can also consider validating CSS on platforms such as W3C CSS Validation Service.
5. Check Compatibility Issues
Depending on the version of JavaFX you are using, some rendering issues might occur. It's advisable to keep your Java and JavaFX libraries up to date, as fixes and improvements are continuously integrated. Also, refer to articles such as How to Make Media Queries Work in Internet Explorer to draw comparisons on how media queries are processed in different environments.
The Last Word
Solving media query issues in JavaFX WebView combines a mix of correct display settings, proper HTML structure, and continual testing. By implementing the given solutions—like setting viewport dimensions and using the correct meta tags—you will significantly enhance the responsiveness of your web content in JavaFX applications.
If you're keen on current best practices, keep an eye on future updates to JavaFX and its components. Responsive design is critical in today's applications, and understanding how to work with WebView effectively will provide a solid foundation for your development efforts.
Further Reading
By following this guide, you’re well on your way to mastering media queries in JavaFX WebView and creating stunning, responsive applications. Happy coding!