Preventing Memory Leaks in Java: 5 Essential Tips
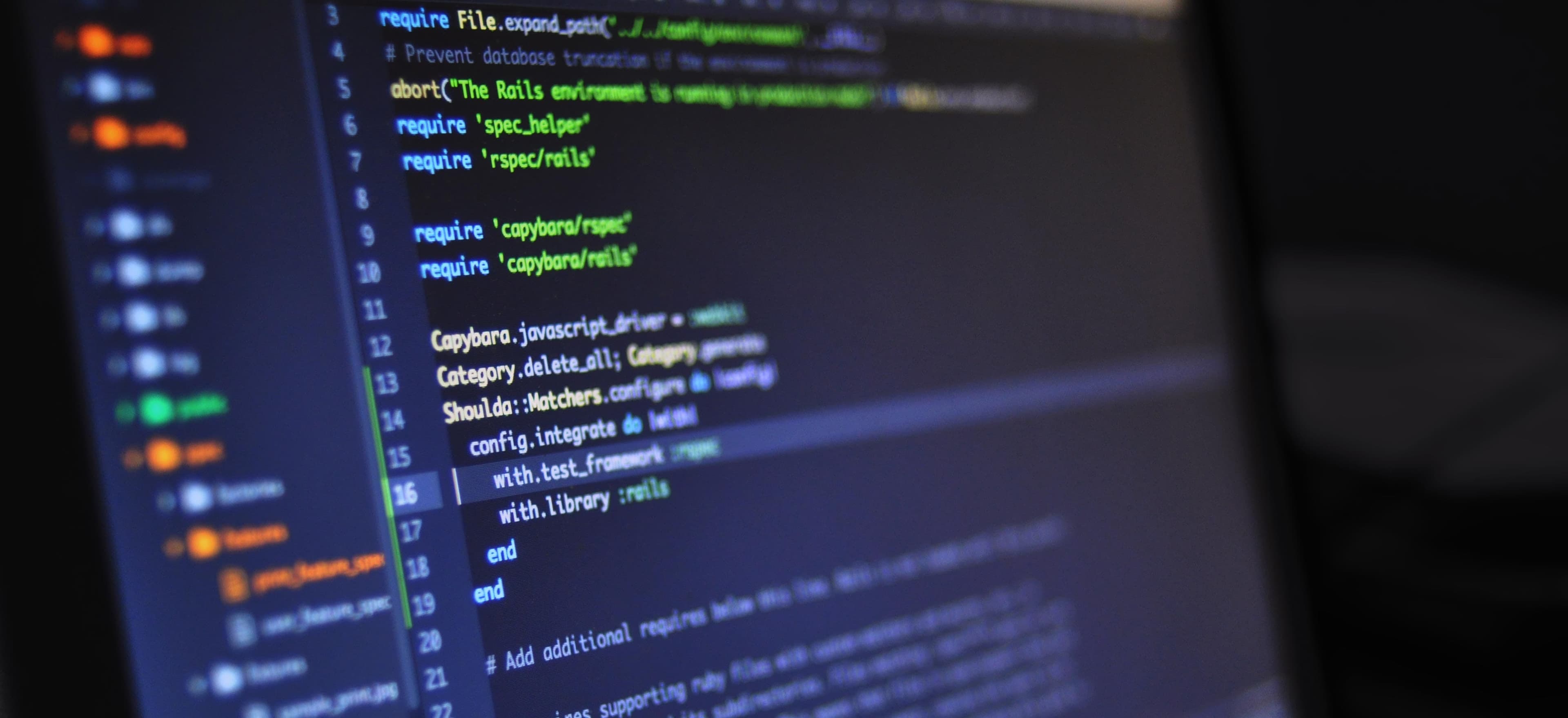
- Published on
Preventing Memory Leaks in Java: 5 Essential Tips
Memory management is a crucial aspect of software development in Java. Despite Java's robust garbage collection, memory leaks can still occur under specific conditions. In this blog post, we'll explore five essential tips to prevent memory leaks in your Java applications. These strategies will help you maintain optimal performance and resource utilization.
Understanding Memory Leaks in Java
Before diving into the tips, it’s vital to understand what a memory leak is. A memory leak occurs when a program fails to release memory that is no longer needed, causing the application to consume more and more memory over time. This can lead to performance degradation and eventually result in an application crash.
Common Causes of Memory Leaks in Java
- Unbounded Collections: Storing references in collections without proper management.
- Static References: Keeping unnecessary objects in static fields.
- Listeners and Callbacks: Not removing unused listeners can lead to retained references.
- ThreadLocal Variables: Unmanaged threads can consume memory unnecessarily.
Now, let’s explore our five essential tips to prevent memory leaks in Java!
Tip 1: Use Weak References Wisely
Example
import java.lang.ref.WeakReference;
public class Cache {
private WeakReference<MyObject> myObjectRef;
public void cacheObject(MyObject obj) {
myObjectRef = new WeakReference<>(obj);
}
public MyObject getCachedObject() {
return myObjectRef.get(); // returns null if the object has been garbage collected
}
}
Commentary
Using WeakReference
allows the garbage collector to reclaim memory for objects when they are no longer in use. This can be particularly useful for caching objects that can be recreated without significant overhead.
Tip 2: Avoid Unbounded Number of Collections
Example
import java.util.HashMap;
import java.util.Map;
public class DataStore {
private Map<String, String> dataMap;
public DataStore() {
dataMap = new HashMap<>();
}
public void addData(String key, String value) {
dataMap.put(key, value);
}
public void clearData() {
dataMap.clear(); // Important to release memory
}
}
Commentary
In the example above, memory can be reclaimed by calling clearData()
. Regularly clearing or pruning your collections helps limit the footprint of your application.
Tip 3: Remove Listeners
Example
import java.util.EventListener;
import java.util.ArrayList;
public class EventSource {
private ArrayList<EventListener> listeners = new ArrayList<>();
public void addListener(EventListener listener) {
listeners.add(listener);
}
public void removeListener(EventListener listener) {
listeners.remove(listener);
}
public void notifyListeners() {
for (EventListener listener : listeners) {
// Notify each listener
}
}
}
Commentary
Failure to remove listeners can lead to memory leaks since the listener will retain a reference to its source. Regularly remove listeners when they are no longer needed to allow for garbage collection.
Tip 4: Use Try-with-Resources
Example
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReaderExample {
public void readFile(String filePath) {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
The try-with-resources statement automatically closes resources like files or database connections. This practice prevents memory leaks by ensuring resources are released promptly.
Tip 5: Analyze Memory Usage Regularly
Using Java profilers and tools like VisualVM or Eclipse Memory Analyzer (MAT) allows you to monitor and analyze memory usage. Identifying memory leaks early on can save significant time and resources.
Example of VisualVM
- Download and Install VisuallVM: Go to VisualVM website for installation.
- Attach to Java Application: Connect VisualVM to your running Java application.
- Monitor Memory and CPU Usage: Keep an eye on memory consumption to identify leaks.
Bringing It All Together
Memory leaks can lead to significant issues in Java applications. By implementing the tips discussed in this blog post, such as using weak references, managing collections adequately, removing listeners, utilizing try-with-resources, and regularly analyzing memory usage, you can prevent these leaks effectively.
For additional insights, refer to our 5 Tips to Prevent Memory Leaks in SPAs. These principles can also be valuable in Java-based web applications.
By practicing these techniques, you will not only enhance your application's performance but also provide a better experience for end-users. Stay proactive about memory management and keep your Java applications running smoothly.
Checkout our other articles