Java Layout Management: Prevent Column Positioning Pitfalls
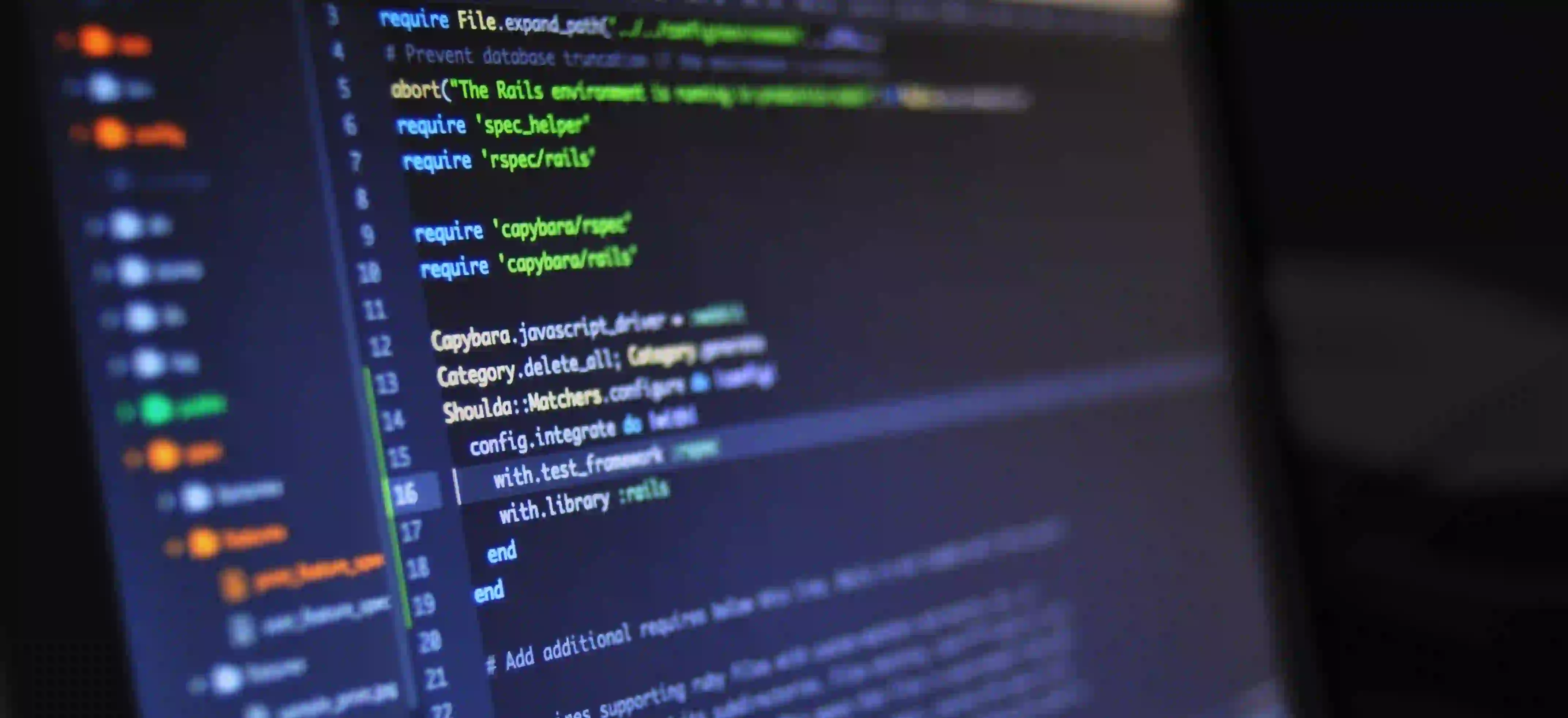
Java Layout Management: Prevent Column Positioning Pitfalls
When developing applications in Java, especially GUI (Graphical User Interface) applications, layout management is a crucial component that can significantly affect user experience. Poor layout can create confusion or frustration for the users. To ensure a smooth interface, understanding various layout managers in Java is fundamental. This article will explore how to effectively manage layouts in Java and prevent common positioning pitfalls, especially concerning column positioning.
Understanding Java Layout Managers
Java's AWT (Abstract Window Toolkit) and Swing libraries offer several layout managers. Each of these managers has its unique characteristics and best use cases. The key layout managers include:
- FlowLayout
- BorderLayout
- GridLayout
- BoxLayout
- GridBagLayout
Each manager serves a distinct purpose, and understanding when to use each one can prevent many common layout blunders.
1. FlowLayout
FlowLayout arranges components in a row, moving to the next line when the row is filled. It’s ideal for simple forms with a few components.
import javax.swing.*;
import java.awt.*;
public class FlowLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("FlowLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300,200);
frame.setLayout(new FlowLayout());
frame.add(new JButton("Button 1"));
frame.add(new JButton("Button 2"));
frame.add(new JButton("Button 3"));
frame.setVisible(true);
}
}
Why: In this example, FlowLayout is utilized for a straightforward horizontal layout. With limited components, FlowLayout prevents cramping, enhances readability, and adjusts based on the frame's size.
2. BorderLayout
BorderLayout divides the container into five regions: North, South, East, West, and Center. This is perfect for applications that need to organize their components into these sections.
import javax.swing.*;
import java.awt.*;
public class BorderLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("BorderLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400,300);
frame.setLayout(new BorderLayout());
frame.add(new JButton("North"), BorderLayout.NORTH);
frame.add(new JButton("South"), BorderLayout.SOUTH);
frame.add(new JButton("East"), BorderLayout.EAST);
frame.add(new JButton("West"), BorderLayout.WEST);
frame.add(new JButton("Center"), BorderLayout.CENTER);
frame.setVisible(true);
}
}
Why: This design clearly delineates the component areas. It’s excellent for displays where you want to feature a primary component centrally with additional components flanking it, thus preventing overlap or misalignment.
3. GridLayout
GridLayout allows for the creation of a grid of components. This is an effective way to manage a fixed number of components in rows and columns, especially for creating forms.
import javax.swing.*;
import java.awt.*;
public class GridLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300,200);
frame.setLayout(new GridLayout(2, 2));
frame.add(new JButton("Button 1"));
frame.add(new JButton("Button 2"));
frame.add(new JButton("Button 3"));
frame.add(new JButton("Button 4"));
frame.setVisible(true);
}
}
Why: This example shows a 2x2 grid. GridLayout is straightforward and prevents components from overlapping while ensuring they maintain a consistent size, suitable for a uniform look.
4. BoxLayout
BoxLayout aligns components either vertically or horizontally. It allows for varying sizes of components while maintaining alignment.
import javax.swing.*;
import java.awt.*;
public class BoxLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("BoxLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300,200);
frame.setLayout(new BoxLayout(frame.getContentPane(), BoxLayout.Y_AXIS));
frame.add(new JButton("Button 1"));
frame.add(Box.createVerticalStrut(10));
frame.add(new JButton("Button 2"));
frame.add(Box.createVerticalStrut(10));
frame.add(new JButton("Button 3"));
frame.setVisible(true);
}
}
Why: With BoxLayout, you can create flexible and elegantly aligned layouts that better adapt to the varying sizes of components, enhancing the appearance of your GUI.
5. GridBagLayout
For fine-tuned control, GridBagLayout allows you to specify the position and size of components in a flexible grid. It is complex but very powerful for intricate layouts.
import javax.swing.*;
import java.awt.*;
public class GridBagLayoutExample {
public static void main(String[] args) {
JFrame frame = new JFrame("GridBagLayout Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400,300);
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridx = 0;
gbc.gridy = 0;
gbc.fill = GridBagConstraints.HORIZONTAL;
frame.add(new JButton("Button 1"), gbc);
gbc.gridx = 1;
gbc.gridy = 0;
frame.add(new JButton("Button 2"), gbc);
gbc.gridx = 0;
gbc.gridy = 1;
gbc.gridwidth = 2; // Take up 2 columns
frame.add(new JButton("Button 3"), gbc);
frame.setVisible(true);
}
}
Why: GridBagLayout offers a high degree of flexibility in layout management, allowing you to specify constraints for each component. With it, column positioning becomes less of a gamble and more of a structured arrangement.
Best Practices for Column Positioning
To avoid common pitfalls in column positioning, consider the following best practices:
-
Understand Your Layout Needs: Before selecting a layout manager, clarify your space requirements and how you want your components to behave. Document your expected UI flow to better select the appropriate layout.
-
Test Responsiveness: Always test your layout in various window sizes. Resize your window and ensure it behaves as intended.
-
Utilize Component Size Preferences: Make use of the preferred, minimum and maximum sizes of components. Understanding how components behave under varying circumstances can prevent misalignment.
-
Separation of Concerns: Keep your layout management code separate from your business logic. It helps in maintaining and debugging your code.
-
Reference Existing Knowledge: Articles like Master Column Positioning: Avoid Common Layout Blunders! provide additional insight into layout practices.
The Closing Argument
Efficient layout management in Java is critical for developing a user-friendly GUI. Choosing the right layout manager and adhering to best practices can help avoid common pitfalls. Whether you opt for FlowLayout, BorderLayout, GridLayout, BoxLayout, or GridBagLayout, understanding when and how to use them will lead to a more intuitive and pleasant user experience.
By incorporating these techniques into your development routine, you can significantly improve the column positioning in your Java applications. Ideally, this knowledge will empower you to create more sophisticated user interfaces that cater to your users' needs seamlessly. Happy coding!