Mastering Java Deployment: Tackling Self-Hosted Git Problems
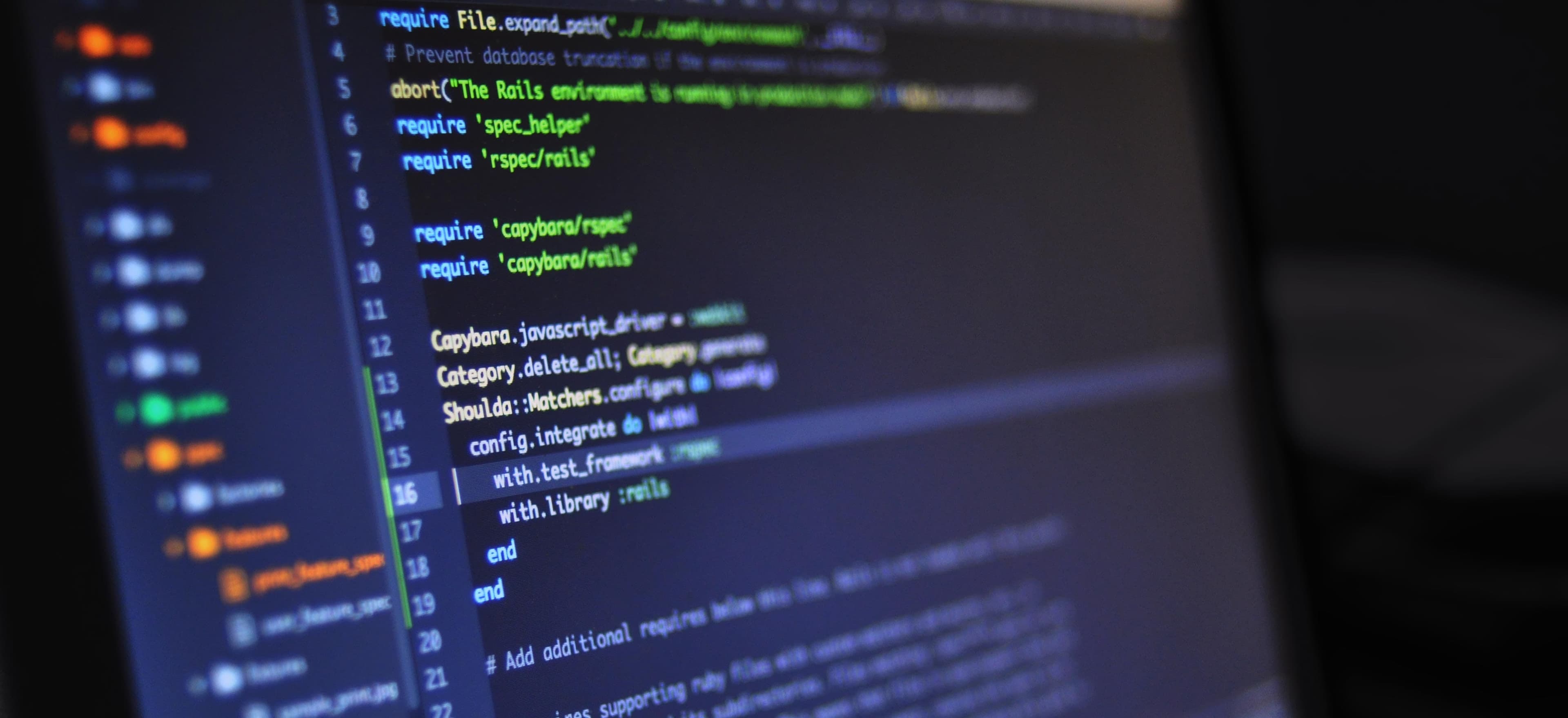
- Published on
Mastering Java Deployment: Tackling Self-Hosted Git Problems
In the realm of software development, efficient project management and code versioning are crucial. This necessity grows when your team commits to using self-hosted Git servers. When deploying Java applications, developers often face unique challenges. This post explores common issues related to self-hosted Git servers and strategies to tackle them, with a specific focus on deploying Java applications.
Understanding Self-Hosted Git Servers
Self-hosted Git servers like Gogs offer unparalleled control over your versioning environment. They allow for custom configurations, data sovereignty, and secure access control. However, they can also present various challenges, especially when it comes to seamless integration with Java deployment workflows.
For a more detailed discussion on common issues encountered with self-hosted Git servers, check out the article titled Overcoming Gogs: Common Self-Hosted Git Server Issues.
Why Choose Self-Hosted Git?
- Control: You dictate user access, repository settings, and backup procedures.
- Customization: Tailor your Git server configuration to meet the unique needs of your organization.
- Integration: Easily integrate with existing CI/CD tools.
Despite its benefits, Gogs can sometimes present roadblocks to your deployment process.
Key Challenges in Self-Hosted Git Deployment
1. Network Configuration Issues
One of the primary hurdles developers face is configuring the network correctly. Misconfigurations can lead to connectivity problems, making deployments frustrating. This is especially crucial when deploying Java applications that may require particular ports to be open.
Solution: Ensure that necessary ports (typically 22 for SSH and 80/443 for HTTP/HTTPS) are open in your firewall settings.
Example: Adjusting Firewall Settings (Linux)
# Check current firewall status
sudo ufw status
# Allow SSH connections
sudo ufw allow OpenSSH
# Allow HTTP
sudo ufw allow http
# Allow HTTPS
sudo ufw allow https
# Enable the firewall
sudo ufw enable
This code snippet ensures that your firewall is configured to allow essential traffic for Git operations, improving your deployment reliability.
2. Authentication Failures
Authentication problems can halt your deployment processes. Commonly, developers may face SSH key issues or token authentication failures.
Solution: Check your SSH keys and ensure that they are correctly added to your Git server under your profile settings.
Example: Adding SSH Key to Git Server
# Generate SSH key pair
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
# Add the SSH public key to the Git server
cat ~/.ssh/id_rsa.pub
The above commands generate an SSH key pair and output the public key so you can conveniently paste it into your Git server's settings.
3. Repository State Conflicts
Having multiple branches and merging changes can lead to conflicts, particularly when multiple developers are collaborating.
Solution: Utilize Git commands effectively to prevent or resolve conflicts.
Example: Using Git to Avoid Conflicts
# Before starting work, pull the latest changes
git pull origin main
# Create and checkout a new branch for your feature
git checkout -b feature/my-feature-name
# Work on your changes, then commit
git add .
git commit -m "Add my new feature"
# Pull changes again to ensure your branch is up-to-date
git pull origin main
# If no conflicts, push your branch
git push origin feature/my-feature-name
Regularly pulling the latest changes before branching can mitigate conflicts, especially root conflicts arising from differing versions of codebases.
Deploying Java Applications from Git
Now that we have identified some of the challenges, let’s take a look at how Java applications can be effectively deployed using a self-hosted Git server.
Step-by-Step Java Deployment Guide
-
Set Up Your Server Environment: Ensure your server is equipped with Java and any other needed dependencies, like Maven or Gradle.
-
Clone Your Repository: Clone the Git repository where your Java project resides.
git clone git@your-git-server.com:username/your-java-project.git
This command imports the project into your server directory, ready for deployment.
-
Build Your Java Project: Use your build tool (Maven or Gradle) to compile the project.
For Maven:
cd your-java-project mvn clean install
This command builds the project and ensures no errors exist in your Java code.
-
Deploy the Application: With your built artifacts in hand, deploy the application to your desired environment (e.g., a Tomcat server).
For example:
cp target/your-app.war /path/to/tomcat/webapps/
Your Java application is now active and can be accessed via the Tomcat server.
Maintaining Deployment Integrity
To ensure the integrity of your deployments, consider implementing a CI/CD pipeline. Tools like Jenkins can automate the deployment process.
Example: Jenkins Pipeline Configuration
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'git@your-git-server.com:username/your-java-project.git'
}
}
stage('Build') {
steps {
sh './gradlew build'
}
}
stage('Deploy') {
steps {
sh 'cp build/libs/your-app.jar /path/to/deployment/'
}
}
}
}
This Jenkins pipeline automates the build and deployment process, ensuring consistency and reducing manual errors.
Final Thoughts
Deploying Java applications using a self-hosted Git server like Gogs does come with its fair share of challenges, but with careful planning and implementation, these obstacles can be effectively managed. From ensuring proper network configurations to addressing authentication failures and repository conflicts, each hurdle can be overcome with the right strategies.
For developers looking to delve deeper into self-hosted Git server issues, I recommend exploring the article Overcoming Gogs: Common Self-Hosted Git Server Issues. Embrace the journey of mastering Java deployment, and turn challenges into a seamless code management experience!
Checkout our other articles