Resolving Git Server Challenges with Java-based Solutions
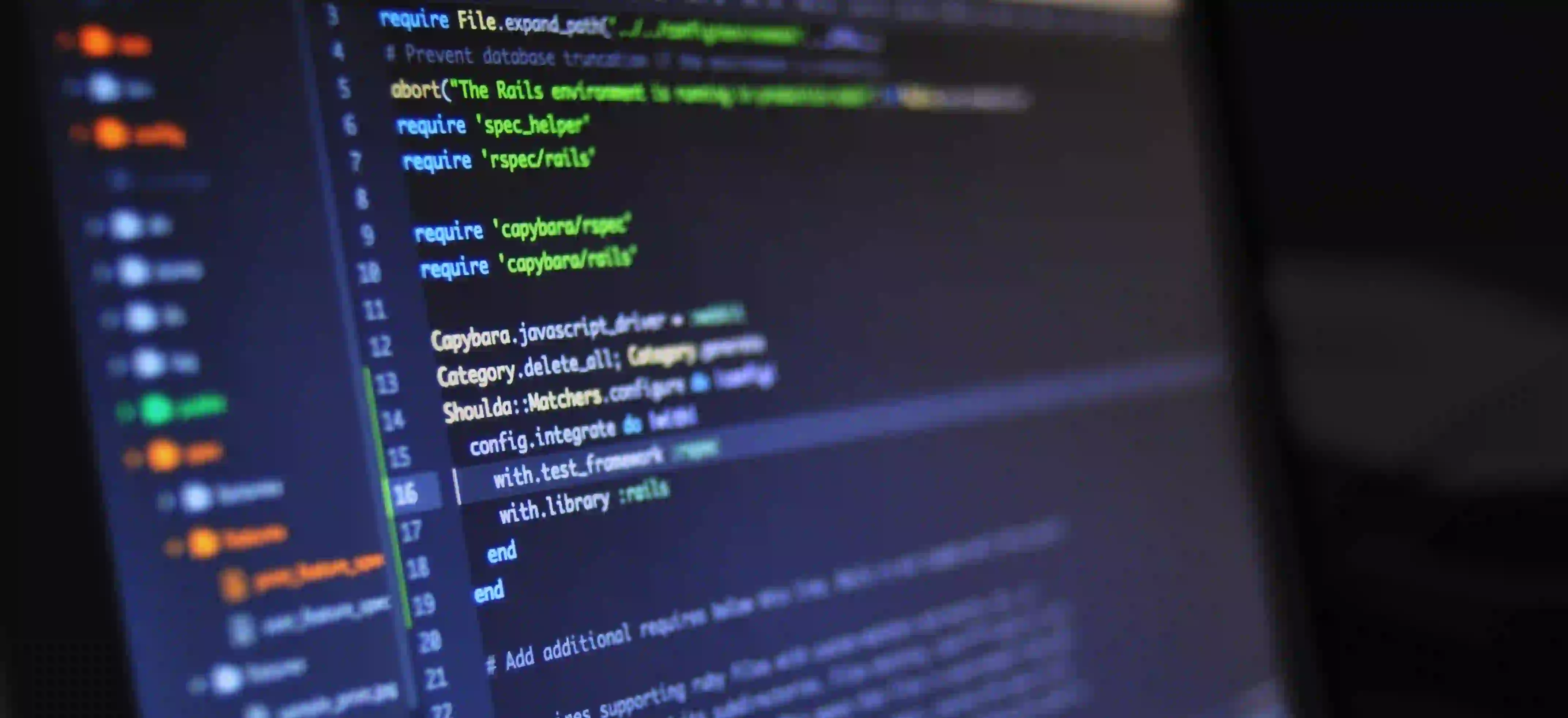
Resolving Git Server Challenges with Java-based Solutions
In the world of development, managing version control effectively is crucial. Git servers like Gogs provide a lightweight solution for self-hosted Git repositories. However, what happens when you face challenges with these servers? In this blog post, we'll explore how Java-based solutions can resolve common issues associated with Git servers, particularly those highlighted in the insightful article, Overcoming Gogs: Common Self-Hosted Git Server Issues.
Understanding the Common Challenges with Self-Hosted Git Servers
Before we dive into Java solutions, let us first understand the common issues developers face with Git servers like Gogs. These challenges may include:
- Authentication Problems: Managing users and permissions can get messy.
- Performance Issues: Slow response times can hinder workflow.
- Backup and Recovery: Ensuring that data is safely backed up and easily recoverable.
- Integration with Continuous Deployment: Automating deployments can be complicated.
The good news is that Java, a robust and versatile programming language, can be used to tackle these difficulties.
Leveraging Java for Git Server Solutions
1. Authentication with Spring Security
One of the most frequent hiccups with Git servers is user authentication issues. Implementing robust authentication mechanisms is crucial for any Git server operation. This is where Java's Spring Security comes into play.
Code Snippet: Basic Authentication Setup
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.web.SecurityFilterChain;
@EnableWebSecurity
public class SecurityConfig {
protected SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll() // Allow public access
.anyRequest().authenticated() // Authenticate all other requests
.and()
.httpBasic(); // Use Basic authentication
return http.build();
}
}
Commentary: Why Use Spring Security
- Flexibility: Allows custom configurations based on your application's authentication needs.
- Integration: Seamlessly integrates with various persistence stores like SQL databases or NoSQL options.
This configuration simplifies managing user permissions and strengthens your Git server's security stance.
2. Improving Performance with Concurrent Processing
Performance is often a bottleneck, especially in large organizations. Using Java's concurrency utilities can significantly enhance performance.
Code Snippet: Parallel Downloads
import java.util.concurrent.*;
public class RepositoryDownloader {
private final ExecutorService executor = Executors.newFixedThreadPool(10);
public void downloadRepositories(List<String> repositoryUrls) {
List<Future<Void>> futures = new ArrayList<>();
for (String url : repositoryUrls) {
futures.add(executor.submit(() -> {
downloadRepository(url);
return null;
}));
}
// Wait for all downloads to finish
for (Future<Void> future : futures) {
try {
future.get(); // Block until the download completes
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
}
private void downloadRepository(String url) {
// Implementation for downloading from Git repository.
}
}
Commentary: Why Use Concurrency
- Speed: By enabling parallel downloads, users do not have to wait sequentially, minimizing downtime.
- Efficiency: Utilizes multiple threads, leading to better CPU usage and faster execution.
Incorporating these concepts will allow your Git server to handle multiple requests efficiently, providing a better experience for users.
3. Implementing Backup Solutions with Spring Boot
Data loss can be a nightmare. Implementing a backup solution in a Java environment can save a lot of trouble down the road.
Code Snippet: Scheduled Backups
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class BackupService {
@Scheduled(cron = "0 0 2 * * ?") // Executes at 2 AM every day
public void backupData() {
// Code to backup Git repositories
System.out.println("Backing up repositories...");
}
}
Commentary: Why Use Scheduled Tasks
- Automation: Automates the backup process, allowing teams to focus on development.
- Reliability: A standard backup process ensures data integrity and peace of mind.
With this strategy, you can set the Git server to back up automatically at specific intervals, further ensuring data safety.
4. Integrating CI/CD Pipelines
The integration of Continuous Integration/Continuous Deployment (CI/CD) with Git servers can streamline workflows. Java offers various libraries that can help achieve this.
Code Snippet: Trigger Build on Git Push
import org.eclipse.jgit.api.Git;
public class GitEventListener {
public void onPushEvent(String repositoryPath) {
try (Git git = Git.open(new File(repositoryPath))) {
// Trigger build process here
triggerBuild(git);
} catch (IOException e) {
e.printStackTrace();
}
}
private void triggerBuild(Git git) {
// Code to trigger CI/CD processes
System.out.println("Triggering CI/CD pipeline...");
}
}
Commentary: Why Automate Deployments
- Efficiency: Reduces manual errors and speeds up the deployment process.
- Consistency: Makes sure code is tested and deployed in the same way every time.
Automating this process will allow developers to focus on writing code instead of getting bogged down with deployment processes.
Bringing It All Together
Managing a self-hosted Git server like Gogs requires an understanding of its challenges and how to effectively solve them. Utilizing Java-based solutions, from user authentication to performance enhancement, backup strategies, and CI/CD integration, can radically improve your Git server management experience.
For further insights into addressing common Git server issues, check out the article, Overcoming Gogs: Common Self-Hosted Git Server Issues.
By integrating these Java techniques into your workflows, you not only tackle existing problems but also lay the groundwork for a more efficient, reliable, and secure development environment.
Incorporating these practices will not only resolve pressing issues but will also promote a culture of continuous improvement in your software development lifecycle. Happy coding!