Tackling Git Server Challenges in Java Development Environments
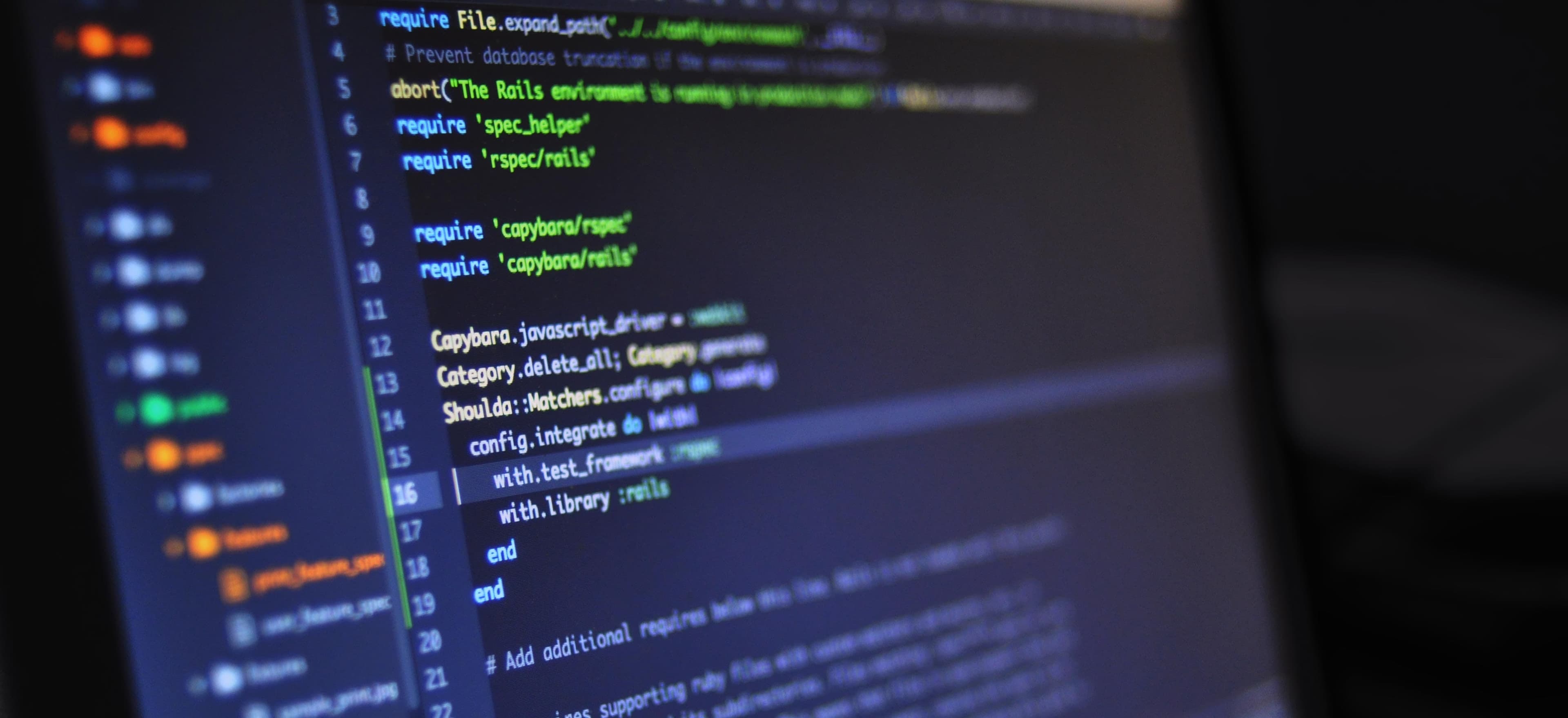
- Published on
Tackling Git Server Challenges in Java Development Environments
In the realm of software development, Git serves as the fundamental backbone for managing source code. When it comes to Java development, configuring and maintaining a Git server is vital for seamless team collaboration. However, like any tool, Git servers can present their own set of challenges. In this post, we'll explore common issues developers face with self-hosted Git servers and provide practical solutions tailored for Java environments. For context, feel free to check out Overcoming Gogs: Common Self-Hosted Git Server Issues.
Understanding Git Servers
A Git server is a repository hosting service, allowing developers to store their code and manage changes across teams. Self-hosted Git servers like Gogs, GitLab, and Bitbucket can significantly enhance control over your development process. However, they can also come with complexities. Below are some typical issues you may encounter, along with Java-centric solutions.
Common Issues with Self-Hosted Git Servers
- Performance Bottlenecks: As your team and projects grow, you might notice sluggish performance.
- User Access Management: Handling user permissions can become cumbersome, especially with multiple teams.
- Backup and Disaster Recovery: Losing your codebase due to a server crash can be catastrophic.
- Integration with CI/CD Tools: Lack of seamless integration with continuous integration and deployment tools can slow down your workflow.
Performance Bottlenecks
When many users access a Git server simultaneously, you may experience delays. Poor performance can arise from inadequate server resources or configuration issues.
Solution: Optimize Your Git Server Configuration
For Java developers using Gogs, a popular lightweight Git server, optimizing its configuration can lead to substantial improvements. Here’s how:
- Adjusting Process Limits: Increase the maximum number of file descriptors to handle numerous concurrent connections.
# Increase the number of allowed open file descriptors
echo "fs.file-max = 100000" >> /etc/sysctl.conf
sysctl -p
- Using SSH for Cloning: Secure Shell (SSH) is faster than HTTPS for cloning, so make sure your team uses SSH.
# Example SSH clone command
git clone git@yourgogshost:yourusername/yourrepository.git
Why This Matters
SSH connections tend to offer higher throughput and lower latency than HTTPS connections, especially beneficial when dealing with larger repositories.
User Access Management
Managing user access can get overwhelming with multiple developers across several projects.
Solution: Implement Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) assigns permissions based on user roles. This simplifies management and enhances security. Gogs supports RBAC, allowing you to classify users into roles such as Admin, Developer, or Viewer.
- Admin: Full rights to manage the repository.
- Developer: Rights to push and pull changes.
- Viewer: Read-only access.
Here's how to set it up in Gogs:
- Navigate to the Settings page of your repository.
- Under Collaborators, add users and assign them appropriate roles.
Why This Matters
RBAC minimizes the risk of accidental changes or unauthorized access, ensuring that only the right people have the right access.
Backup and Disaster Recovery
Unexpected server crashes can result in data loss. Regular backups are essential for disaster recovery.
Solution: Automating Backups
In a Java development environment, you can automate backups using scripts. Here’s a Bash script to back up your repositories:
#!/bin/bash
# Backup all repositories to a specified directory
BACKUP_DIR="/var/git/backups"
DATE=$(date +%Y%m%d_%H%M)
mkdir -p $BACKUP_DIR
cd /var/git/repositories
for repo in *; do
git clone --mirror $repo $BACKUP_DIR/$repo-$DATE.git
done
Why This Matters
Regularly backing up your code ensures that you can restore it in the event of a failure. The earlier you detect a problem, the easier it is to fix it.
Integration with CI/CD Tools
Continuous Integration (CI) and Continuous Deployment (CD) are essential components of modern software development.
Solution: Integrate with Jenkins and Git
You can integrate Gogs with Jenkins for automatic builds and deployments. Here’s how to set it up:
-
Install Jenkins: Follow the installation instructions on the Jenkins official site.
-
Add Git Repository in Jenkins:
- Navigate to your Jenkins dashboard.
- Click on “New Item,” enter a name, select “Freestyle project,” and click OK.
- Under the Source Code Management section, select Git and enter your repository URL.
Here’s an example of a Jenkinsfile for a simple Java project:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'mvn clean install'
}
}
}
stage('Deploy') {
steps {
script {
sh 'docker build -t myapp .'
sh 'docker run -d -p 8080:8080 myapp'
}
}
}
}
}
Why This Matters
Integrating CI/CD with your Git server helps automate testing and deployment, enabling faster and more reliable delivery of features.
Final Considerations
Managing a self-hosted Git server can be a challenging endeavor for Java developers. Performance bottlenecks, user access issues, backup strategy, and CI/CD integration are pivotal aspects that require attention. Implementing the solutions discussed in this post can significantly enhance your experience and streamline your development process.
Don't forget to refer back to the insights in the article Overcoming Gogs: Common Self-Hosted Git Server Issues for further details on handling specific challenges with Gogs. Embrace these strategies and watch your Java development workflow evolve into an efficient machine. Happy coding!