Navigating Java Integrations for Chatbot Success
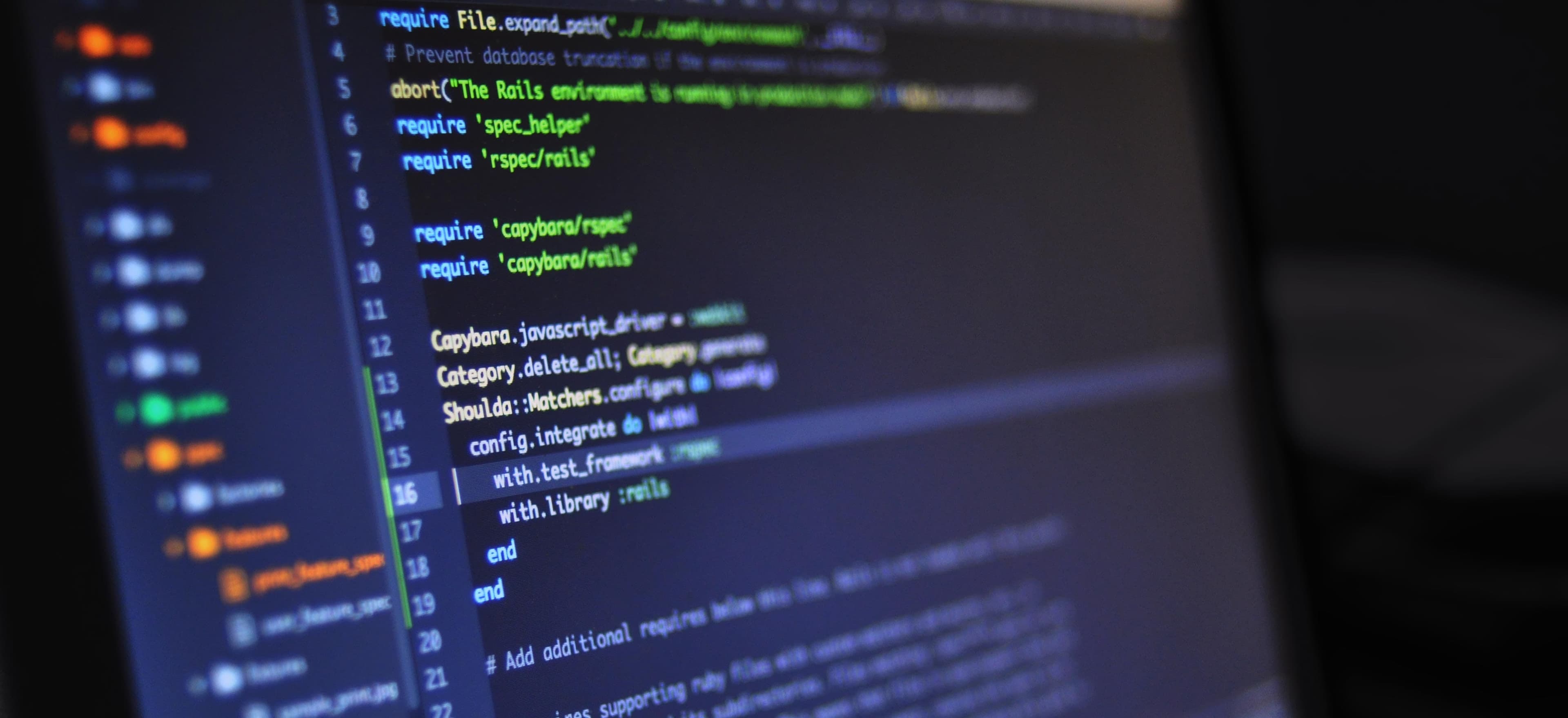
- Published on
Navigating Java Integrations for Chatbot Success
In the evolving landscape of technology, chatbots have emerged as a pivotal component in enhancing user experience. From handling customer queries to providing real-time assistance, the potential applications of chatbots are vast. This article focuses on how Java can be effectively utilized to integrate various functionalities into chatbots, ensuring they are not only user-friendly but also highly efficient.
Why Choose Java for Chatbot Development?
Java is a powerful programming language, well-suited for developing robust applications, including chatbots. Here are a few reasons why Java is a great choice:
- Platform Independence: Java's write-once, run-anywhere philosophy means that code can be executed on any device that has the Java Runtime Environment (JRE).
- Strong Community Support: Java boasts a large developer community, ensuring support, libraries, and frameworks that can accelerate development.
- Integration Capabilities: Java integrates seamlessly with various APIs and third-party services, which is a boon for chatbot functionalities.
- Scalability: Java applications can easily be scaled to accommodate growing user demands without compromising performance.
Getting Started with Chatbot Development in Java
If you are new to chatbot development, a good starting point is to build a simple chatbot using Java and an API for Natural Language Processing (NLP), such as the one provided by Dialogflow.
Sample Chatbot Interaction in Java
Below, we present a simple implementation using Java to interact with Dialogflow:
import com.google.api.client.googleapis.javanet.GoogleNetHttpTransport;
import com.google.api.client.json.JsonFactory;
import com.google.api.client.json.jackson2.JacksonFactory;
import com.google.api.services.dialogflow.v2.Dialogflow;
import com.google.api.services.dialogflow.v2.model.QueryInput;
import com.google.api.services.dialogflow.v2.model.QueryResult;
import java.io.IOException;
public class Chatbot {
private static final String PROJECT_ID = "your-project-id";
public QueryResult detectIntentTexts(String sessionId, String text) throws Exception {
Dialogflow dialogflow = createDialogflowService();
QueryInput queryInput = new QueryInput().setText(new TextInput().setText(text).setLanguageCode("en"));
// Call Dialogflow to detect intent
DetectIntentRequest request = new DetectIntentRequest().setQueryInput(queryInput);
DetectIntentResponse response = dialogflow.projects().agent().sessions().detectIntent(PROJECT_ID, sessionId, request).execute();
return response.getQueryResult();
}
private Dialogflow createDialogflowService() throws Exception {
JsonFactory jsonFactory = JacksonFactory.getDefaultInstance();
return new Dialogflow.Builder(GoogleNetHttpTransport.newTrustedHttpTransport(), jsonFactory, null)
.setApplicationName("Dialogflow Integration").build();
}
}
Code Explanation
- Libraries: We are using Dialogflow’s Java library to simplify our integrative functionalities.
- Detect Intent: The
detectIntentTexts
method is vital; it sends user messages to Dialogflow and retrieves responses. This is how chatbots interpret user queries. - Modularity: The use of helper methods makes the code clean and manageable, allowing for easy modifications and enhancements.
Understanding Chatbot Functionality
When developing chatbots, it is crucial to understand the steps involved in processing user input. The above code snippet illustrates just one dimension—how to process text input. However, there are several common challenges developers face:
- Handling Context: Bots need to maintain conversation context to provide meaningful interactions.
- Understanding User Intent: Accurately interpreting user input to invoke the correct responses.
- Managing External APIs: Interfacing with other systems or services can be intricate.
For insights on conquering these challenges, you can check this article: Overcoming Common Challenges in Dialogflow Chatbot Development.
Advanced Integrations
As your chatbot development matures, incorporating advanced functionalities becomes essential:
- Webhook Integration: Integrating webhooks can take user interaction to the next level, allowing your bot to provide dynamic content based on user needs.
- Machine Learning: Implementing machine learning algorithms can improve your bot's learning capabilities and enhance user experience.
- Analytics and Monitoring: Implementing analytics features enables you to track usage patterns, allowing for the continuous improvement of your bot.
Implementing Webhook in Java
Let’s see how to create a simple webhook service in Java.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.*;
@SpringBootApplication
@RestController
public class WebhookService {
public static void main(String[] args) {
SpringApplication.run(WebhookService.class, args);
}
@PostMapping("/webhook")
public String handleWebhook(@RequestBody String incomingWebhookData) {
// Process the data received from Dialogflow
return "Webhook received. Data processed.";
}
}
Code Explanation
- Spring Boot: Using Spring Boot simplifies the setup process for creating a RESTful application.
- Receiving Data: The
@PostMapping
annotation indicates that the webhook will respond to POST requests. - Future Development: The structure allows for easy implementation of logic to process any incoming webhook data, making it highly adaptable.
The Last Word
With its strong features, Java stands out as an excellent language for chatbot development. The ability to integrate with powerful NLP platforms like Dialogflow, alongside implementing webhooks and leveraging libraries, enables developers to create responsive, intelligent chatbots that cater to user needs.
Remember, as with all development efforts, continuous learning and adapting are key. Embrace the challenges mentioned above with creativity and innovation, and your chatbot project will undoubtedly succeed.
In case you're interested in diving deeper into overcoming common challenges you’ll face during the development process, you can visit: Overcoming Common Challenges in Dialogflow Chatbot Development.
Happy coding!
Checkout our other articles