Mastering Reusable Android Dialogs for Efficient UI
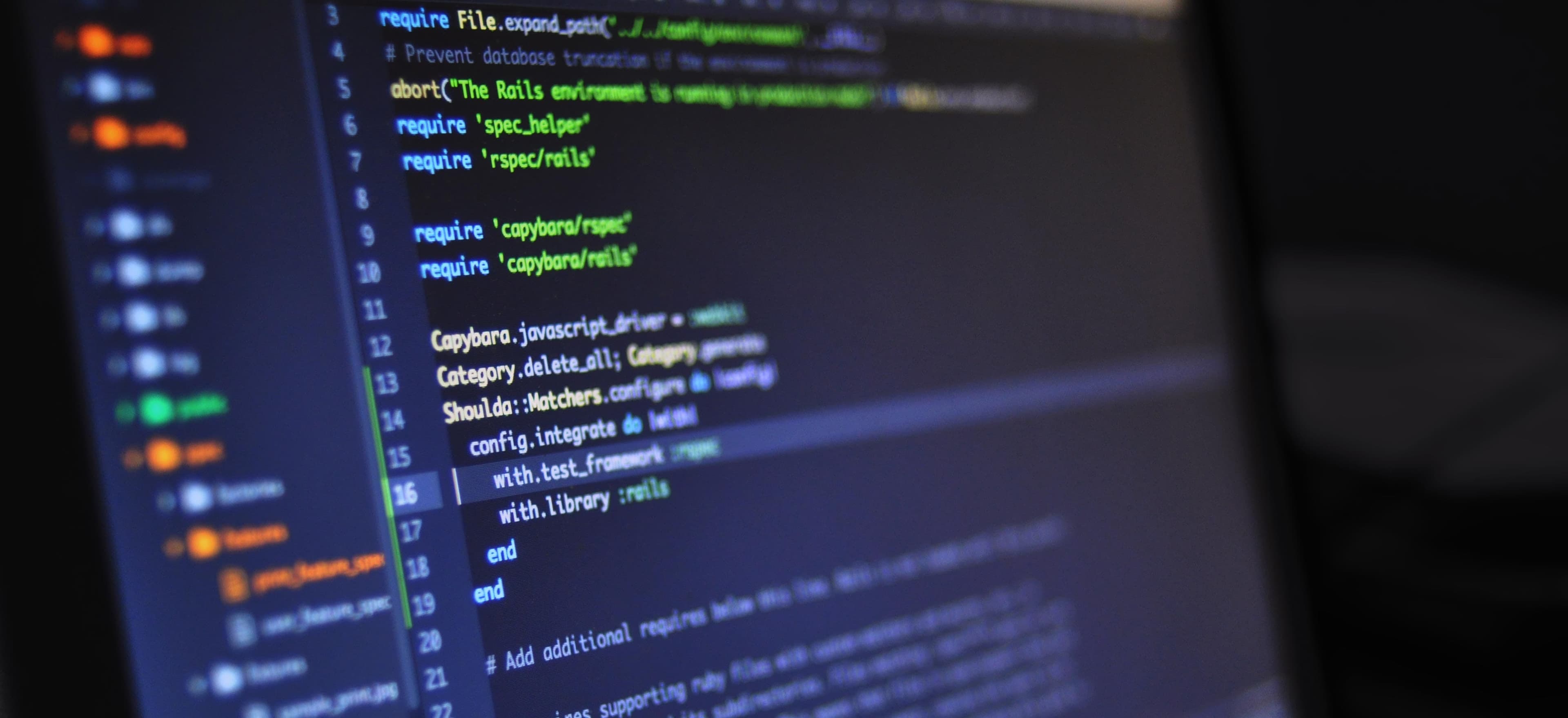
- Published on
Mastering Reusable Android Dialogs for Efficient UI
Dialogs are an integral part of Android application development. They enable you to communicate information to users, prompt them for decisions, or display a series of options. However, as your app grows, managing dialogs can become cumbersome. In this blog post, we'll explore how to create reusable Android dialogs that enhance user experience while maintaining cleaner code.
Why Reusable Dialogs?
Creating reusable dialogs in Android has several benefits:
-
Consistent User Experience: When the same dialog design and behavior are used throughout your app, users find it easier to navigate and understand your interface.
-
Reduced Code Duplication: Instead of rewriting dialog code in multiple activities or fragments, you can encapsulate the dialog logic in a single class.
-
Easier Maintenance: Changes can be made in one place, and they'll propagate throughout your app, making it easier to implement features and fix bugs.
-
Enhanced Testing: Isolated dialog components can be tested more effectively.
Now, let's take a closer look at how to implement these reusable dialogs in Android.
Creating a Base Dialog
We'll start by creating a base dialog class that inherits from DialogFragment
. This will allow us to manage our dialogs' lifecycle conveniently.
Step 1: Setup Your BaseDialog Class
Create a new class called BaseDialog.java
:
import android.app.Dialog;
import android.content.DialogInterface;
import android.os.Bundle;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AlertDialog;
import androidx.fragment.app.DialogFragment;
public abstract class BaseDialog extends DialogFragment {
protected abstract String getDialogTitle();
protected abstract String getDialogMessage();
protected abstract void onPositiveButtonClick();
protected abstract void onNegativeButtonClick();
@NonNull
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setTitle(getDialogTitle())
.setMessage(getDialogMessage())
.setPositiveButton("OK", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
onPositiveButtonClick();
}
})
.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int id) {
onNegativeButtonClick();
}
});
return builder.create();
}
}
Explanation of the Code
-
Abstract Methods: The abstract methods,
getDialogTitle()
,getDialogMessage()
,onPositiveButtonClick()
, andonNegativeButtonClick()
, are placeholders. They must be overridden by any class that extendsBaseDialog
, ensuring that a dialog title and message are always provided. -
Dialog Construction: In
onCreateDialog()
, we build the dialog usingAlertDialog.Builder
, which is a user-friendly way of constructing dialogs in Android. The OK and Cancel buttons are set to execute the respective methods defined in the child class.
Step 2: Implementing a Specific Dialog
Now that we have a base class, we can create specific dialogs by extending BaseDialog
. Let's create a confirmation dialog as an example.
ConfirmationDialog.java
public class ConfirmationDialog extends BaseDialog {
private final String title;
private final String message;
private final Runnable onConfirm;
public ConfirmationDialog(String title, String message, Runnable onConfirm) {
this.title = title;
this.message = message;
this.onConfirm = onConfirm;
}
@Override
protected String getDialogTitle() {
return title;
}
@Override
protected String getDialogMessage() {
return message;
}
@Override
protected void onPositiveButtonClick() {
onConfirm.run();
}
@Override
protected void onNegativeButtonClick() {
// Do nothing or handle cancellation
}
}
Explanation of the Code
-
Constructor Parameters: The
ConfirmationDialog
takes title, message, and an action (as aRunnable
) that defines what happens when the user confirms their choice. -
Override Methods: The methods overridden from the base class supply the dialog with its title and message. We also define what happens on a positive button click—executing the
onConfirm
action passed when the dialog was created.
Step 3: Using the Dialog in an Activity
To use ConfirmationDialog
, simply create an instance and show it in your activity:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
findViewById(R.id.show_dialog_button).setOnClickListener(v -> {
ConfirmationDialog dialog = new ConfirmationDialog("Delete Item",
"Are you sure you want to delete this item?",
() -> {
// Handle confirm action
Toast.makeText(this, "Item Deleted!", Toast.LENGTH_SHORT).show();
});
dialog.show(getSupportFragmentManager(), "ConfirmationDialog");
});
}
}
Explanation of the Code
-
Creating the Dialog: When the user clicks the button, a
ConfirmationDialog
is instantiated with a title, a message, and a confirmation action. -
Dialog Display: The dialog is shown using the
show
method of theDialogFragment
, passing the fragment manager and a unique tag.
Step 4: Customizing the Dialog
You may want to create different types of dialogs that require additional fields, such as a text input dialog. This can be done by further extending BaseDialog
.
InputDialog.java
public class InputDialog extends BaseDialog {
private EditText inputField;
private final String title;
private final String message;
private final Consumer<String> onInputProvided;
public InputDialog(String title, String message, Consumer<String> onInputProvided) {
this.title = title;
this.message = message;
this.onInputProvided = onInputProvided;
}
@Override
protected String getDialogTitle() {
return title;
}
@Override
protected String getDialogMessage() {
return message;
}
@Override
public Dialog onCreateDialog(Bundle savedInstanceState) {
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
inputField = new EditText(getActivity());
builder.setTitle(getDialogTitle())
.setMessage(getDialogMessage())
.setView(inputField)
.setPositiveButton("OK", (dialog, id) -> onInputProvided.accept(inputField.getText().toString()))
.setNegativeButton("Cancel", (dialog, id) -> dialog.dismiss());
return builder.create();
}
}
Explanation of the Code
-
Input Field: The dialog now includes an
EditText
field for user input. -
Consumer Interface: We use the
Consumer<String>
interface, allowing us to capture input data from the dialog easily. This is a more dynamic approach for handling input.
The Last Word
By mastering reusable Android dialogs, you can create a more efficient UI. The use of base classes helps maintain consistency across your application, reduces duplication, and provides an easier way to manage dialog-related tasks. Check out Android's official documentation for more information on dialogs and their usage.
Implementing these dialogs not only results in a better user experience but also a more maintainable codebase. Go ahead and try these strategies in your own projects to enhance your app's UI efficiency!
Further Reading
By following the patterns discussed in this post, you can ensure your Android applications are not only more functional but also more enjoyable for your users. Happy coding!
Checkout our other articles