Mastering Spring Bean Lifecycle: Common Pitfalls to Avoid
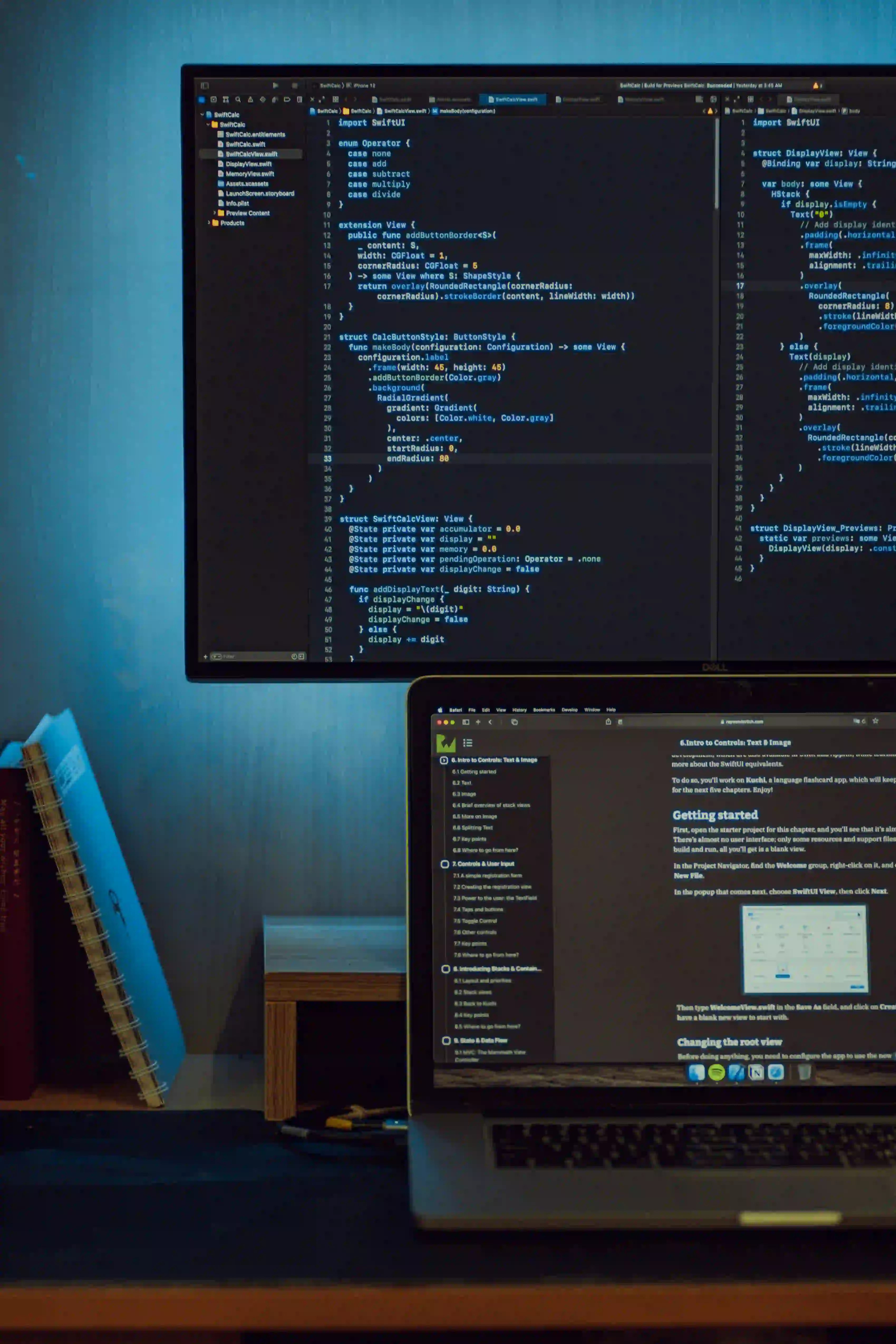
Mastering the Spring Bean Lifecycle: Common Pitfalls to Avoid
Spring framework is known for its robust architecture, which offers flexibility and simplicity in managing application components. At the core of this architecture is the concept of beans. Understanding the Spring Bean Lifecycle is crucial for effectively managing resources and optimizing application behavior.
In this blog post, we will delve into the Spring Bean Lifecycle, exploring its phases and addressing common pitfalls to avoid. By the end, you will have a comprehensive understanding of how Spring manages beans and the best practices to ensure smooth application development.
Understanding Spring Bean Lifecycle
Before we dive into the pitfalls, let’s review the fundamental phases of the Spring Bean Lifecycle:
- Instantiation: A Spring container creates a bean instance.
- Populate Properties: Values are injected into the bean's properties.
- Set Bean Name: The bean is given an identifier.
- Set Bean Factory: The bean can access its parent factory for resource requirements.
- Post-process Before Initialization: Custom actions can be defined via
BeanPostProcessor
before initialization. - Initialize: Initialization methods are invoked.
- Post-process After Initialization: Custom actions can occur after the initialization.
- Ready for use: The bean is ready to serve its designated purpose.
- Destruction: The bean is removed from the Spring container, and cleanup is performed if necessary.
With these phases in mind, let’s explore the common pitfalls developers encounter when working with the Spring Bean Lifecycle.
Pitfall 1: Ignoring Bean Scope
Why It Matters
Each bean in Spring can be defined with a specific scope. The default scope is singleton, meaning only one instance exists in the container. However, you can define beans with different scopes depending on your application needs, like prototype, request, session, etc.
Example Code
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("prototype")
public class MyPrototypeBean {
public MyPrototypeBean() {
// Constructor
}
}
What to Avoid
Not specifying bean scope can lead to memory issues and unwanted side effects. For instance, using a singleton bean when you need a prototype can cause state sharing between components, which can lead to unexpected behavior.
Best Practice
Always evaluate the requirements of your beans and choose the scope that aligns with their usage.
Pitfall 2: Dependent Beans Initialization
Why It Matters
In applications involving multiple beans which depend on each other, the order of initialization can lead to problems where a bean is not fully initialized before it is needed by another bean.
Example Code
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class A {
private final B b;
@Autowired
public A(B b) {
this.b = b;
}
}
@Component
public class B {
private final A a;
@Autowired
public B(A a) {
this.a = a;
}
}
What to Avoid
Circular dependencies can occur, leading to BeanCurrentlyInCreationException
. This can be frustrating, especially for new developers not familiar with this issue.
Best Practice
Reorganize beans to avoid circular dependencies, perhaps by introducing more abstraction or using method injection instead of constructor injection.
Pitfall 3: Not Leveraging @PostConstruct
and @PreDestroy
Why It Matters
@PostConstruct
is crucial for defining initialization logic after the bean’s properties have been set. @PreDestroy
allows you to specify cleanup logic before the bean is destroyed.
Example Code
import javax.annotation.PostConstruct;
import javax.annotation.PreDestroy;
@Component
public class MyBean {
@PostConstruct
public void init() {
System.out.println("Bean initialized");
}
@PreDestroy
public void destroy() {
System.out.println("Bean destroyed");
}
}
What to Avoid
Neglecting these annotations can result in sensitive resources remaining open longer than necessary, leading to resource leaks and inconsistent program states.
Best Practice
Always utilize @PostConstruct
for setup tasks and @PreDestroy
to clean up resources to ensure your beans manage their lifecycle properly.
Pitfall 4: Overusing BeanPostProcessor
Why It Matters
BeanPostProcessor
allows you to modify new bean instances, providing excellent opportunities for cross-cutting concerns. However, excessive use can lead to complex and hard-to-maintain code.
What to Avoid
Overcomplicating your bean configuration can make your application brittle, as it couples different concerns together in an unmanaged way.
Best Practice
Use BeanPostProcessor
judiciously. Stick to configuration concerns, and ensure it's adding value by simplifying the behavior or enforcing consistent practices across beans instead of creating unmanageable complexity.
Pitfall 5: Misunderstanding Destruction Phase
Why It Matters
It’s vital to understand how and when beans are destroyed, especially for singleton beans. Not implementing the destruction logic can leave open connections or memory leaks.
Example Code
import javax.annotation.PreDestroy;
@Component
public class MySingletonBean {
@PreDestroy
public void cleanup() {
// Cleanup resources
System.out.println("Cleanup resources here");
}
}
What to Avoid
Failing to handle resource cleanup can lead to significant performance issues, especially in long-running applications or server environments.
Best Practice
Always implement destruction logic for beans that manage external resources or stateful connections. This approach ensures a responsive and high-performing application.
The Bottom Line
Mastering the Spring Bean Lifecycle is essential for creating efficient and maintainable applications. By recognizing common pitfalls and implementing best practices, you can avoid issues that may lead to significant challenges down the line.
Remember, the lifecycle of a bean in Spring is more than just instantiation; it involves careful management and understanding of initialization and destruction processes. By adhering to proper practices around scope, dependencies, initializer methods, and clean-up routines, you will harness the full power of Spring’s dependency injection and management capabilities.
For further reading on Spring Beans, consider checking out the official Spring documentation.
Additional Resources
- Spring Framework Documentation
- Spring Bean Scopes
- Circular Dependency in Spring
Equipped with this knowledge, you're now ready to tackle Spring applications with confidence. Happy coding!