Why Composition Trumps Inheritance in Modern Development
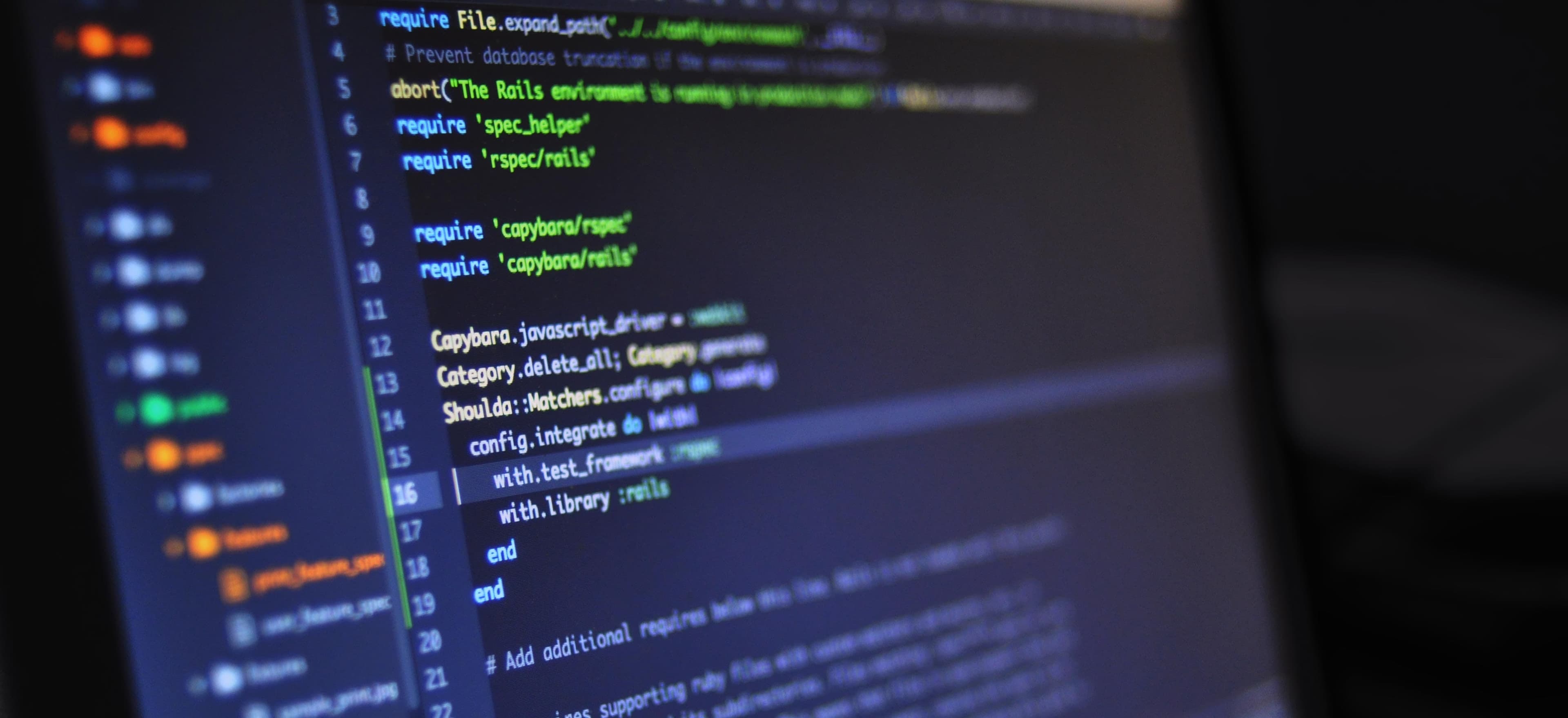
- Published on
Why Composition Trumps Inheritance in Modern Development
In the world of software development, the design of software architecture can significantly influence a project's maintainability, flexibility, and scalability. The choice between composition and inheritance has long been a topic of debate. While inheritance has its place, especially in object-oriented programming, composition has proven to be a more robust and flexible approach in modern development paradigms. In this blog post, we will explore why composition is often preferred over inheritance, with a few code examples to illustrate the concepts involved.
Understanding Inheritance
Inheritance allows a class to inherit the properties and methods of another class, promoting code reuse. However, it can lead to a tightly coupled architecture. Here’s a simple example in Java:
class Animal {
void sound() {
System.out.println("Animal makes sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
In this example, the Dog
class inherits from the Animal
class and overrides the sound()
method. On the surface, this seems effective for code reuse. But let's delve deeper.
The Issues with Inheritance
-
Tight Coupling: The
Dog
class is tightly coupled to theAnimal
class, which means changes inAnimal
can inadvertently affectDog
. This dependency complicates refactoring and testing. -
Fragile Base Class Problem: If the base class (in our case,
Animal
) changes, it can break derived classes (likeDog
) that rely on its implementation. -
Limited Flexibility: Inheritance creates a rigid structure that limits how we can mix behavior. For instance, if you want to create a
Cat
that can alsoSwim
, you cannot easily do this without creating an awkward inheritance hierarchy.
The Power of Composition
Composition, in contrast, allows for a more flexible approach to building classes. Instead of creating a hierarchical structure, composition enables classes to be composed of different parts, each responsible for specific behaviors.
How Composition Works
Consider an example of a Bird
that can both Fly
and Swim
. Instead of using inheritance, we can create interfaces for behaviors and implement these behaviors in the Bird
class.
interface Flyable {
void fly();
}
interface Swimmable {
void swim();
}
class Duck implements Flyable, Swimmable {
public void fly() {
System.out.println("Duck can fly");
}
public void swim() {
System.out.println("Duck can swim");
}
}
In this example, the Duck
class implements both Flyable
and Swimmable
interfaces. This approach provides several benefits:
Benefits of Composition
-
Loose Coupling: Each behavior is implemented in its own interface. Changing how
Duck
flies or swims won't affect the other functionality. -
Greater Flexibility: You can easily create new classes like
Penguin
that canSwim
but notFly
, simply by implementing theSwimmable
interface. -
Reusability: The interfaces allow different classes to share functionality without inheritance hierarchies. You could have a
Plane
class implementingFlyable
without creating an unnecessary link toBird
.
Composition Over Inheritance
In the context of modern application development, here are a few more reasons why composition is favored:
-
Easier to Understand: Code that utilizes composition is often more straightforward and easier for developers to understand. The relationships between classes are explicit and not hidden under layers of inheritance.
-
Improved Testing: When behaviors are decoupled, unit tests can target interfaces independently, leading to clearer and more maintainable tests.
-
Behavioral Flexibility: By allowing objects to compose behaviors at runtime, behavior change can be handled more gracefully.
A Real-World Example of Composition
Let’s expand on a practical example from a simple e-commerce application. Imagine we need to model payment processing. With composition, we can manage different payment methods without creating complex hierarchies.
interface PaymentMethod {
void pay(double amount);
}
class CreditCard implements PaymentMethod {
@Override
public void pay(double amount) {
System.out.println("Paid " + amount + " using Credit Card");
}
}
class PayPal implements PaymentMethod {
@Override
public void pay(double amount) {
System.out.println("Paid " + amount + " using PayPal");
}
}
class Order {
private PaymentMethod paymentMethod;
public Order(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
public void checkout(double amount) {
paymentMethod.pay(amount);
}
}
In this example, the Order
class can accept any type of PaymentMethod
. This allows for easy extension if we need to add new payment methods in the future, such as BankTransfer
or Cryptocurrency
.
My Closing Thoughts on the Matter
While inheritance can be useful in certain contexts, the drawbacks often outweigh the benefits. Composition offers a more flexible, maintainable, and scalable approach to software design. It enhances code reusability, promotes separation of concerns, and minimizes the chances of introducing bugs through tightly-coupled dependencies.
By embracing composition, developers can create systems that are easier to change, extend, and test. This shift in mindset, from inheritance to composition, arms us with the tools needed to tackle the complex challenges of modern software development effectively.
For further reading, explore the concepts of SOLID Principles or dive into more complex design patterns that use composition effectively, such as the Strategy Pattern and Decorator Pattern.
References
- Martin, R. C. (2002). Clean Code: A Handbook of Agile Software Craftsmanship.
- Freeman, E., & Robinson, B. (2004). Head First Object-Oriented Analysis and Design.
By consistently applying the principle of composition over inheritance, developers can not only enhance the quality of their individual codebases but also contribute to the overall agility and adaptability of their teams and organizations.