Top 5 Challenges Developers Face with Selenium 4
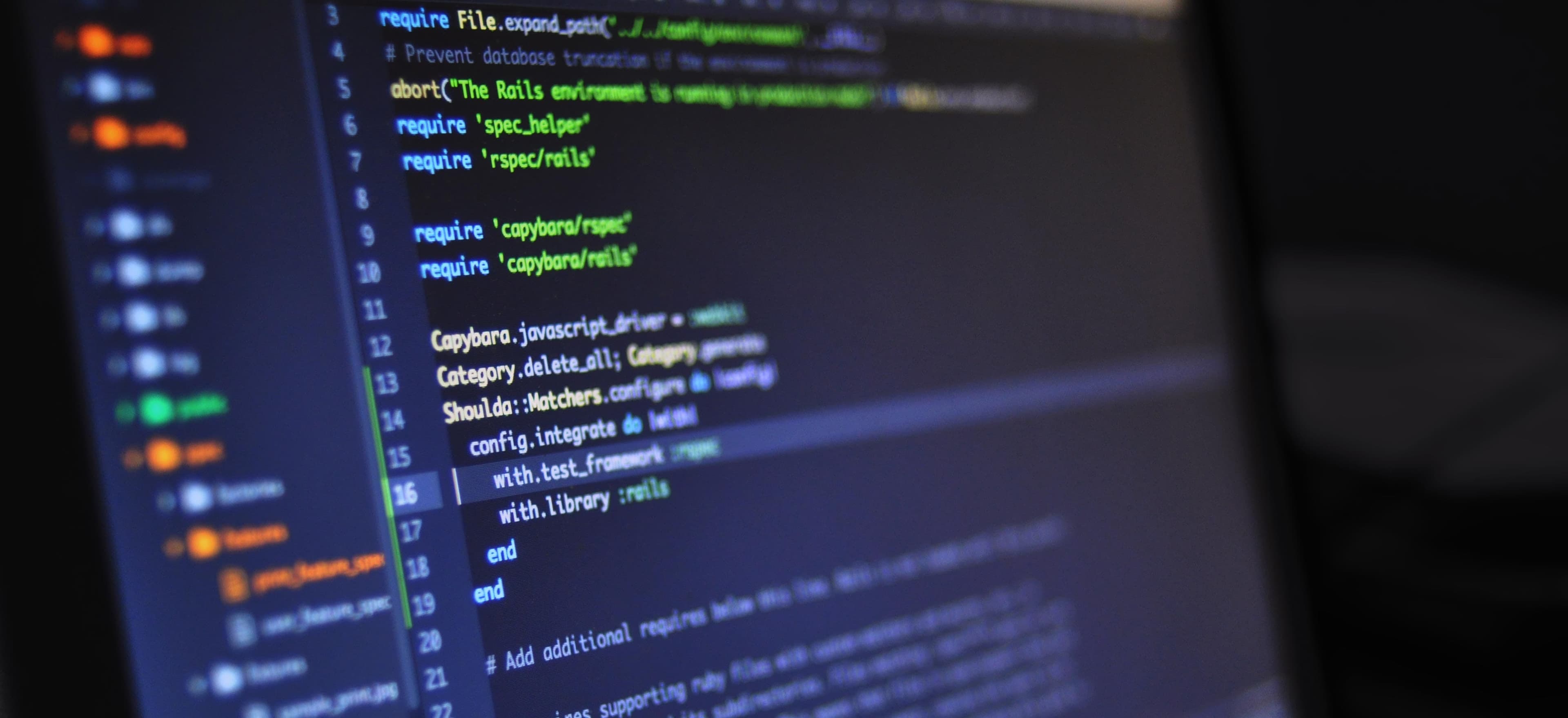
- Published on
Top 5 Challenges Developers Face with Selenium 4
Selenium has long been a preferred tool for automating web browsers, providing an excellent framework to write tests in various programming languages. With the launch of Selenium 4, developers welcomed an array of new features, but not without encountering several challenges. In this blog post, we will delve into the top five challenges that developers face while working with Selenium 4 and how they can tackle these obstacles effectively.
1. Learning Curve
The Challenge
Selenium 4 introduces a plethora of changes and enhancements compared to its predecessor. While new features such as the WebDriver specification and native support for Chrome DevTools are powerful, they necessitate a shift in how developers write their tests. Understanding these changes can overwhelm newcomers and even seasoned developers.
Solution
The best way to overcome this challenge is to invest time in comprehensive documentation and tutorials. The official Selenium 4 documentation is an excellent resource for developers aiming to grasp the nuances of the new framework. Additionally, online courses and tutorials can help to build familiarity with the latest features and functionalities.
Example Code Snippet
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.devtools.DevTools;
public class Selenium4Example {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Create a DevTools session for Chrome
DevTools devTools = ((ChromeDriver) driver).getDevTools();
devTools.createSession();
// Your testing code goes here
driver.quit();
}
}
In the example above, we utilize the DevTools
interface for Chrome, which provides access to the Chrome DevTools Protocol, allowing developers to interact more deeply with the browser. This is part of the new learning curve associated with Selenium 4.
2. Handling Dynamic Elements
The Challenge
Web applications today are increasingly interactive, which means that many elements on a web page may load dynamically after the initial page load. Selenium 3 had workarounds for waiting, but the methods can be less efficient or lead to flaky tests.
Solution
Selenium 4 improves on implicit and explicit waits, allowing for better handling of dynamic content. The WebDriverWait combined with expected conditions remains a reliable approach.
Example Code Snippet
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
public class DynamicElementsExample {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("http://your-web-app.com");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
// Wait for the dynamic element to be visible
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElementId")));
driver.quit();
}
}
In this code, we wait for a dynamic element to become visible before proceeding. This ensures that we handle timing issues more gracefully compared to hard-coded sleep statements, thereby reducing test flakiness.
3. Lack of Built-in Listeners
The Challenge
One of the significant changes in Selenium 4 is the absence of built-in listeners for test execution. This change can complicate logging and debugging, as developers often rely on listeners to capture events throughout the testing lifecycle.
Solution
To effectively manage logging in Selenium 4, developers can implement custom listeners. By creating listener classes, you can react to test events such as failures or successes and log accordingly. Frameworks like TestNG or JUnit can aid in integrating these listeners.
Example Code Snippet
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class CustomTestListener implements ITestListener{
@Override
public void onTestSuccess(ITestResult result) {
System.out.println(result.getMethod().getMethodName() + " was successful!");
}
@Override
public void onTestFailure(ITestResult result) {
System.err.println(result.getMethod().getMethodName() + " failed. Error: " + result.getThrowable().getMessage());
}
}
In the code snippet above, we implement a simple ITestListener
where we capture the success or failure of test methods, providing a basic structure for logging during the test execution phase.
4. Difficulties in Troubleshooting
The Challenge
With evolving software systems, troubleshooting when tests fail becomes more complex. Selenium 4 introduces new capabilities, but the advance can obscure root causes when issues arise, leading to prolonged debugging sessions for developers.
Solution
Maintaining practical logging practices and using unit tests alongside Selenium can help isolate issues better. Further, integrating browser dev tools with your testing can provide more insights into what fails during the automation run.
Good Practices
- Use Clear Logging: Ensure that each step in your automated tests is logged clearly. Use the logging capabilities of your testing framework to capture details.
- Debugger Usage: Familiarize yourself with the debugging capabilities of your IDE to step through Selenium tests, enabling a more granular look at where failures happen.
5. Cross-Browser Testing
The Challenge
Cross-browser testing remains an ongoing issue with any web automation tool. While Selenium is designed to work across multiple browsers, inconsistencies still arise. In Selenium 4, there are refinements, but minor discrepancies can impact test results.
Solution
Using Selenium Grid effectively can help alleviate cross-browser testing issues. Selenium Grid allows for running tests in multiple browsers and environments, providing consistency across tests.
Example Code Snippet
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import java.net.MalformedURLException;
import java.net.URL;
public class SeleniumGridExample {
public static void main(String[] args) throws MalformedURLException {
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
WebDriver driver = new RemoteWebDriver(new URL("http://localhost:4444/wd/hub"), capabilities);
driver.get("http://your-web-app.com");
// Your testing logic
driver.quit();
}
}
This code snippet is a straightforward illustration of how to utilize Selenium Grid. By specifying desired capabilities, we can run tests across a remote server, ensuring that we cover various browser versions and configurations.
The Closing Argument
While Selenium 4 brings exciting new features, it is not without its challenges. Acknowledging these issues can help developers adapt their testing strategies more effectively. Embracing new learning resources, refining debugging practices, and utilizing tools like Selenium Grid can make a significant difference in overcoming these hurdles.
Navigating the landscape of web automation with Selenium 4 might feel daunting at first, but with the right tools, resources, and mindset, developers can harness Selenium's power for automated testing like never before. For further reading on automating web applications using Selenium, you may check out SeleniumHQ or explore the broader implications of web automation on testing processes. Happy testing!
Checkout our other articles