Common Pitfalls in Auto-Publishing with Spring Boot APIs
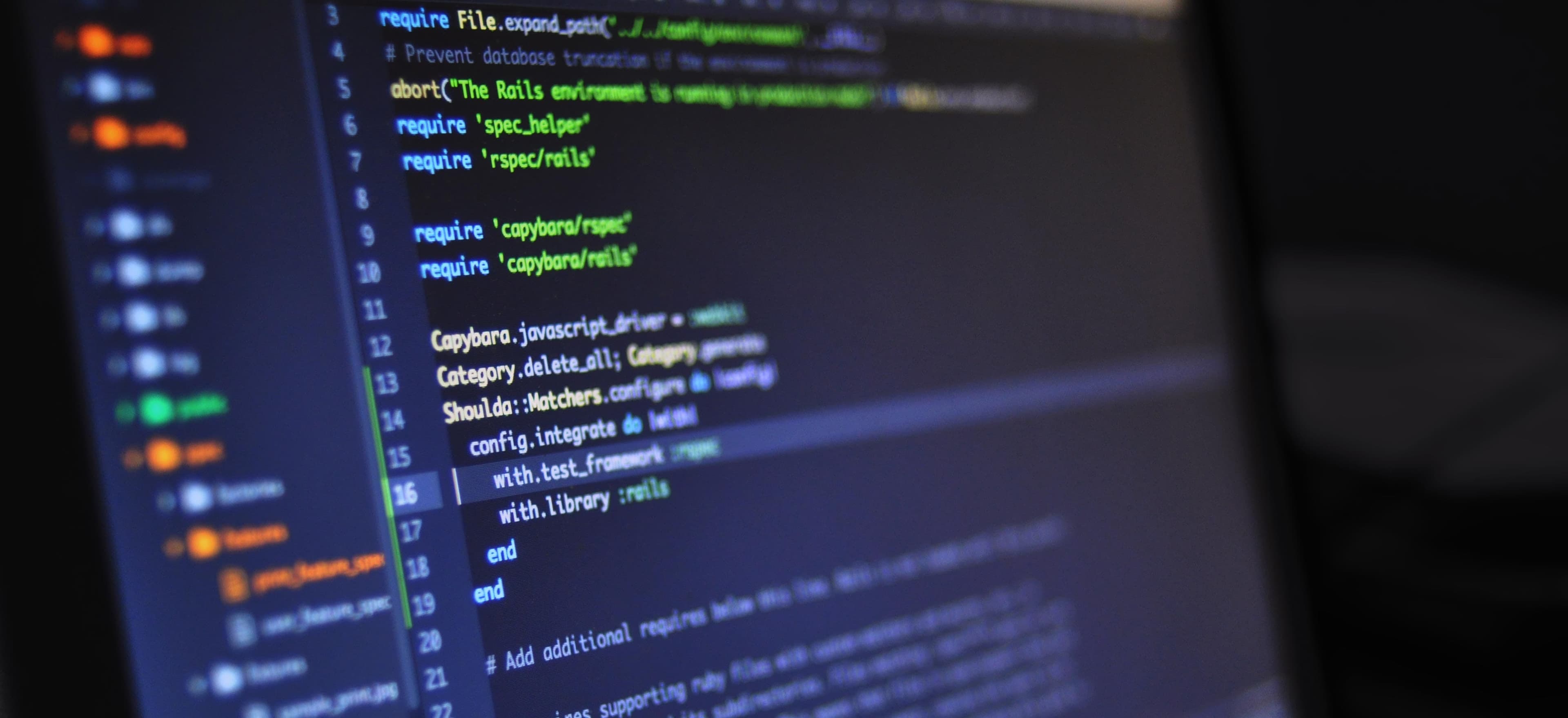
- Published on
Common Pitfalls in Auto-Publishing with Spring Boot APIs
In the ever-evolving landscape of software development, Spring Boot has emerged as a go-to framework for building robust, production-ready applications. Auto-publishing APIs can lead to significant efficiencies. However, it's essential to navigate this process cautiously, as pitfalls can lead to deployment delays or application failures. This blog post will explore common pitfalls in auto-publishing with Spring Boot APIs, enhancing your understanding and guiding you through effective practices.
Understanding Auto-Publishing in Spring Boot
Before diving deep into the pitfalls, let's clarify what auto-publishing means in the context of Spring Boot. Auto-publishing refers to the automatic deployment of applications, particularly when there are updates or changes pushed to a repository. Automating this process can reduce human error and speed up the deployment cycle. But as with any automated process, challenges can arise.
Common Pitfalls
1. Misconfigured Application Properties
One of the most common pitfalls in auto-publishing is an oversight in application properties. Spring Boot lets you externalize application configuration, which is crucial for the different environments your application operates in—development, testing, and production.
# application.yml
server:
port: 8080
spring:
datasource:
url: jdbc:mysql://localhost:3306/exampledb
username: user
password: password
Why It's Important
Misconfiguration may lead to severe issues, such as:
- Connecting to the wrong database.
- Using incorrect credentials.
- Exposing your application to potential security vulnerabilities.
Fixing the Issue
To avoid misconfiguration, use profiles. Spring Boot allows you to create multiple application property files, such as application-dev.yml, application-test.yml, and application-prod.yml, each tailored to a specific environment.
2. Ignoring Error Handling
When you automate your deployments, the application might start behaving unexpectedly after a push. If proper error handling isn’t in place, these issues might go unnoticed in production.
@RestController
public class ExampleController {
@GetMapping("/example")
public ResponseEntity<String> example() {
try {
// Business logic
return ResponseEntity.ok("Success");
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An error occurred: " + e.getMessage());
}
}
}
Why It’s Important
The goal is to gracefully manage any exceptions your application may encounter. This provides users with feedback and logs the necessary details for developers to investigate.
Fixing Error Handling
Use Spring's built-in mechanisms, like @ControllerAdvice
, to create centralized exception handling:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An internal error occurred: " + e.getMessage());
}
}
3. Overlooking Caching Strategies
If your Spring Boot application leverages caching but you neglect to consider its behavior during deployments, you might face performance issues. Caching too aggressively can lead to outdated data being served.
Why It’s Important
It's essential to balance cache freshness and performance. If a new version of your API is published, the cache might serve old data, leading to inconsistent application states.
Fixing the Issue
Implement cache invalidation strategies. Consider using aspects of Spring Cache. Define expiration policies based on your use case.
@Cacheable(value = "products")
public List<Product> getProducts() {
// Fetch from database
}
@CacheEvict(value = "products", allEntries = true)
public void clearProductCache() {
// This method can be called when new products are added
}
4. Not Implementing Monitoring and Alerts
One of the most significant concerns when using auto-publishing is knowing when something goes wrong. Failing to set up monitoring and alerts can lead to unnoticed outages.
Why It's Important
You need to stay informed about your application status post-deployment. Automated alerts can help you mitigate risks more effectively.
Fixing the Issue
Use tools like Spring Boot Actuator to expose metrics and health endpoints. Pair it with a monitoring tool like Prometheus or Grafana, or a service like Datadog.
# application.yml
management:
endpoints:
web:
exposure:
include: health,info
5. Ignoring Security Practices
Security is a top concern in any automated environment. Properly handling sensitive data during auto-publishing is crucial.
Why It’s Important
Weak security measures can expose your application to attacks. Sensitive information, such as API keys and database passwords, must not be hardcoded.
Fixing the Issue
Utilize Spring Security to handle authentication and authorization. Also, consider using environment variables or a secrets manager for sensitive data management.
// Basic Auth with Spring Security
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
6. Lack of Versioning
Deploying new API versions without proper versioning can confuse API consumers and might lead to breaking changes.
Why It’s Important
Good versioning practices allow for backwards compatibility and clear communication between API providers and consumers.
Fixing the Issue
Implement API versioning through the URL or HTTP headers:
@GetMapping("/api/v1/example")
public ResponseEntity<String> exampleV1() {
return ResponseEntity.ok("Example V1");
}
@GetMapping("/api/v2/example")
public ResponseEntity<String> exampleV2() {
return ResponseEntity.ok("Example V2 with new features");
}
7. Inadequate Testing
Automating the deployment of APIs without adequate testing is akin to walking a tightrope without a safety net. Ignoring comprehensive tests can lead to deployment of unverified code.
Why It’s Important
Testing ensures that your code works as expected before it reaches the production environment.
Fixing Inadequate Testing
Incorporate unit tests, integration tests, and end-to-end tests using frameworks like JUnit and Mockito alongside continuous integration tools like Jenkins or GitHub Actions.
@SpringBootTest
class ExampleControllerTests {
@Autowired
private MockMvc mockMvc;
@Test
void exampleReturnsSuccess() throws Exception {
mockMvc.perform(get("/example"))
.andExpect(status().isOk())
.andExpect(content().string("Success"));
}
}
To Wrap Things Up
Auto-publishing Spring Boot APIs can streamline your deployment process. However, being aware of and avoiding common pitfalls is critical. From ensuring proper configuration to implementing robust monitoring and security measures, each step plays a vital role in the success of your deployment strategy.
For more insights on Spring Boot best practices, consider visiting Spring Official Documentation. By addressing these challenges, you'll enhance your application's resilience, performance, and security—all while enjoying the benefits of an automated deployment pipeline.
With these insights in hand, you can navigate auto-publishing more effectively, ensuring smooth transitions from development to production. Start applying these strategies today, and witness a significant enhancement in your deployment process!