Mastering Java 8: Avoiding Common Decorator Mistakes
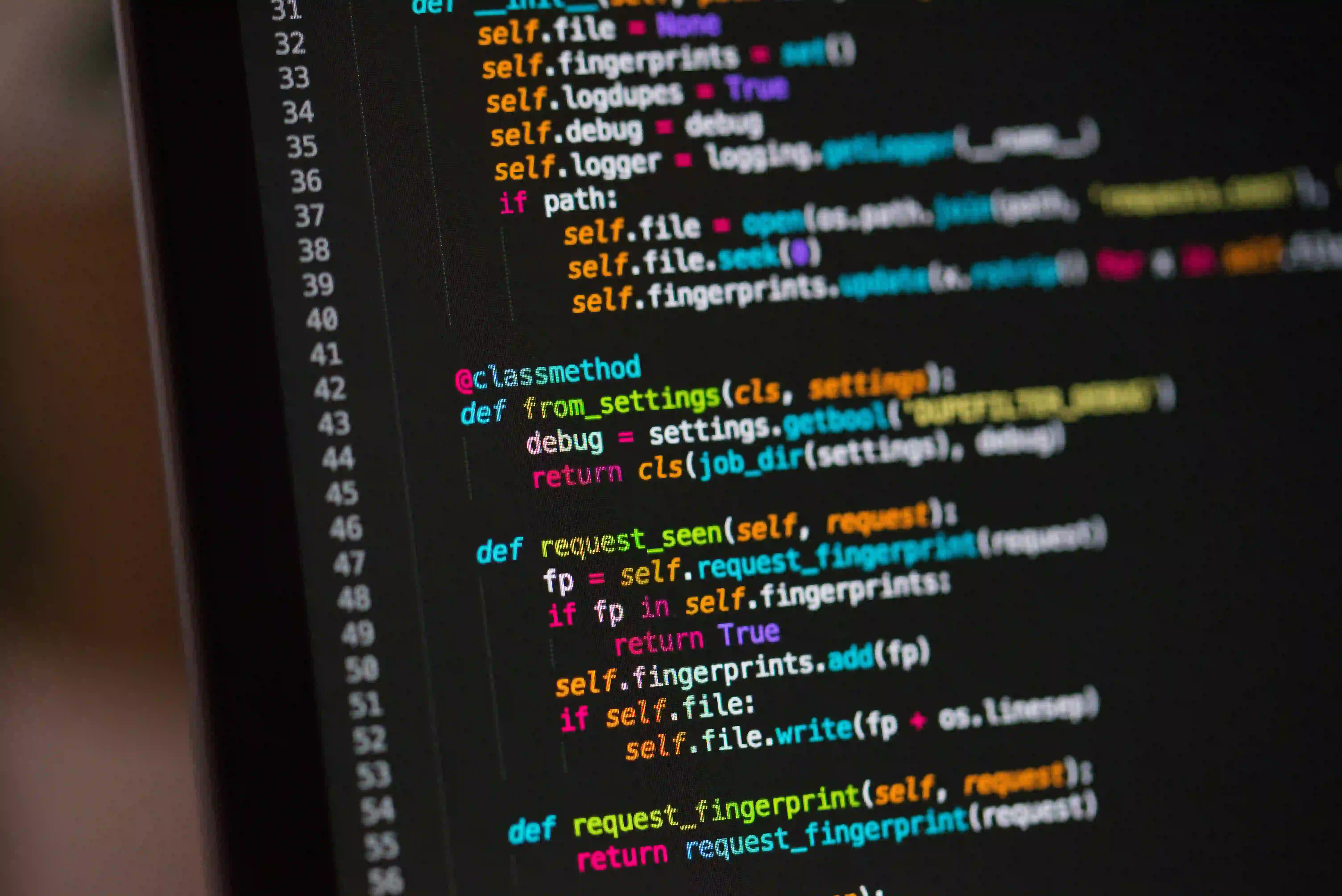
Mastering Java 8: Avoiding Common Decorator Mistakes
Java 8 has revolutionized the way we approach programming in Java, introducing powerful features like lambda expressions, streams, and the decorator pattern. While the decorator pattern is a valuable design pattern for extending the functionality of objects, it can be fraught with common pitfalls that many developers encounter. In this blog post, we will explore these common mistakes and how to avoid them while mastering the implementation of decorators.
What is the Decorator Pattern?
Before diving into the common mistakes, let's clarify what the decorator pattern is. The decorator pattern is a structural design pattern that enables you to add new functionalities to existing objects without altering their structure. This is achieved by creating a set of decorator classes that are used to wrap concrete components.
Basic Structure of the Decorator Pattern
The core components of the decorator pattern are as follows:
- Component: An interface that defines the operations that can be performed.
- ConcreteComponent: A class that implements the component interface.
- Decorator: An abstract class that implements the component interface and contains a reference to a component object.
- ConcreteDecorator: Classes that extend the decorator class, adding functionality.
Here's a succinct example of a coffee shop context.
// The Component Interface
public interface Coffee {
String getDescription();
double cost();
}
// Concrete Component
public class SimpleCoffee implements Coffee {
@Override
public String getDescription() {
return "Simple Coffee";
}
@Override
public double cost() {
return 2.00;
}
}
// The Decorator
public abstract class CoffeeDecorator implements Coffee {
protected Coffee coffee;
public CoffeeDecorator(Coffee coffee) {
this.coffee = coffee;
}
@Override
public String getDescription() {
return coffee.getDescription();
}
@Override
public double cost() {
return coffee.cost();
}
}
// Concrete Decorators
public class MilkDecorator extends CoffeeDecorator {
public MilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Milk";
}
@Override
public double cost() {
return coffee.cost() + 0.50;
}
}
public class SugarDecorator extends CoffeeDecorator {
public SugarDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Sugar";
}
@Override
public double cost() {
return coffee.cost() + 0.20;
}
}
In this example, multiple decorators can be applied to create a customized coffee order.
Common Decorator Mistakes and How to Avoid Them
Mistake 1: Not Utilizing Interface Segregation
One of the simplest mistakes developers make is combining functionality that should be separated into different decorators.
Why It Matters
Combining multiple functionalities can lead to bloated decorators that become difficult to manage and test. Instead, adhere to the Interface Segregation Principle by keeping your decorators focused.
Solution: Create Separate Decorators
For example, if you need a FullCreamMilkDecorator and a LowFatMilkDecorator, it’s good practice to create individual decorators.
Code Example
public class FullCreamMilkDecorator extends CoffeeDecorator {
public FullCreamMilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Full Cream Milk";
}
@Override
public double cost() {
return coffee.cost() + 0.75;
}
}
public class LowFatMilkDecorator extends CoffeeDecorator {
public LowFatMilkDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Low Fat Milk";
}
@Override
public double cost() {
return coffee.cost() + 0.60;
}
}
Mistake 2: Ignoring Code Reusability
Developers might duplicate code across multiple decorators. This leads to more difficult maintenance and potential bugs in future updates.
Why It Matters
Code duplication clutters your codebase and makes it more challenging to implement changes or fixes.
Solution: Use Superclass Methods Wisely
Utilize superclass methods to reduce redundancy. Ensure decorators call the base methods wherever applicable.
For instance, in our CoffeeDecorator
, instead of repeating getDescription()
and cost()
in every decorator, leverage the existing implementation.
Mistake 3: Over-engineering Simple Decorators
Sometimes, developers might introduce excessive complexity into decorators. Adding too many layers may confuse future maintainers.
Why It Matters
Over-engineering can lead to performance issues and make the code harder to understand.
Solution: Assess the Complexity
Keep your decorators straightforward. If a decorator is adding minor features, consider if it needs to exist at all.
Example
If our SugarDecorator
merely adds a single feature, we should simplify how we implement it, ensuring clarity.
public class SugarDecorator extends CoffeeDecorator {
public SugarDecorator(Coffee coffee) {
super(coffee);
}
@Override
public String getDescription() {
return coffee.getDescription() + ", Sugar";
}
@Override
public double cost() {
return coffee.cost() + 0.20;
}
}
Best Practices for Decorators in Java 8
Use Java 8 Features
Java 8 introduced significant enhancements to the language that can work well with decorators.
Stream API
Consider integrating the Stream API when handling lists of components. This allows efficient processing and enhancements to collections of items.
List<Coffee> coffeeList = Arrays.asList(new SimpleCoffee(), new MilkDecorator(new SimpleCoffee()));
// Calculating total cost with Java 8 Stream
double totalCost = coffeeList.stream()
.mapToDouble(Coffee::cost)
.sum();
Leverage Lambda Expressions
In some cases, decorators can be rethought as functional interfaces. This can make your code cleaner and reduce boilerplate.
@FunctionalInterface
public interface Coffee {
String getDescription();
double cost();
}
// Lambda Representation
Coffee coffee = () -> {
return "Lambda Coffee";
};
Final Thoughts
Mastering the decorator pattern in Java 8 can significantly enhance your software design and functionality while maintaining clean code.
Avoiding common pitfalls, such as code duplication, over-complication, and poor separation of concerns, will facilitate ease of maintenance and readability.
To dive deeper into the decorator pattern and Java 8 features, you may find the following resources valuable:
With this foundational knowledge, you are well-equipped to leverage the decorator pattern effectively in your Java projects, enhancing your software's extensibility and maintainability. Happy coding!