Avoiding Common Pitfalls in Serialization Proxy Pattern
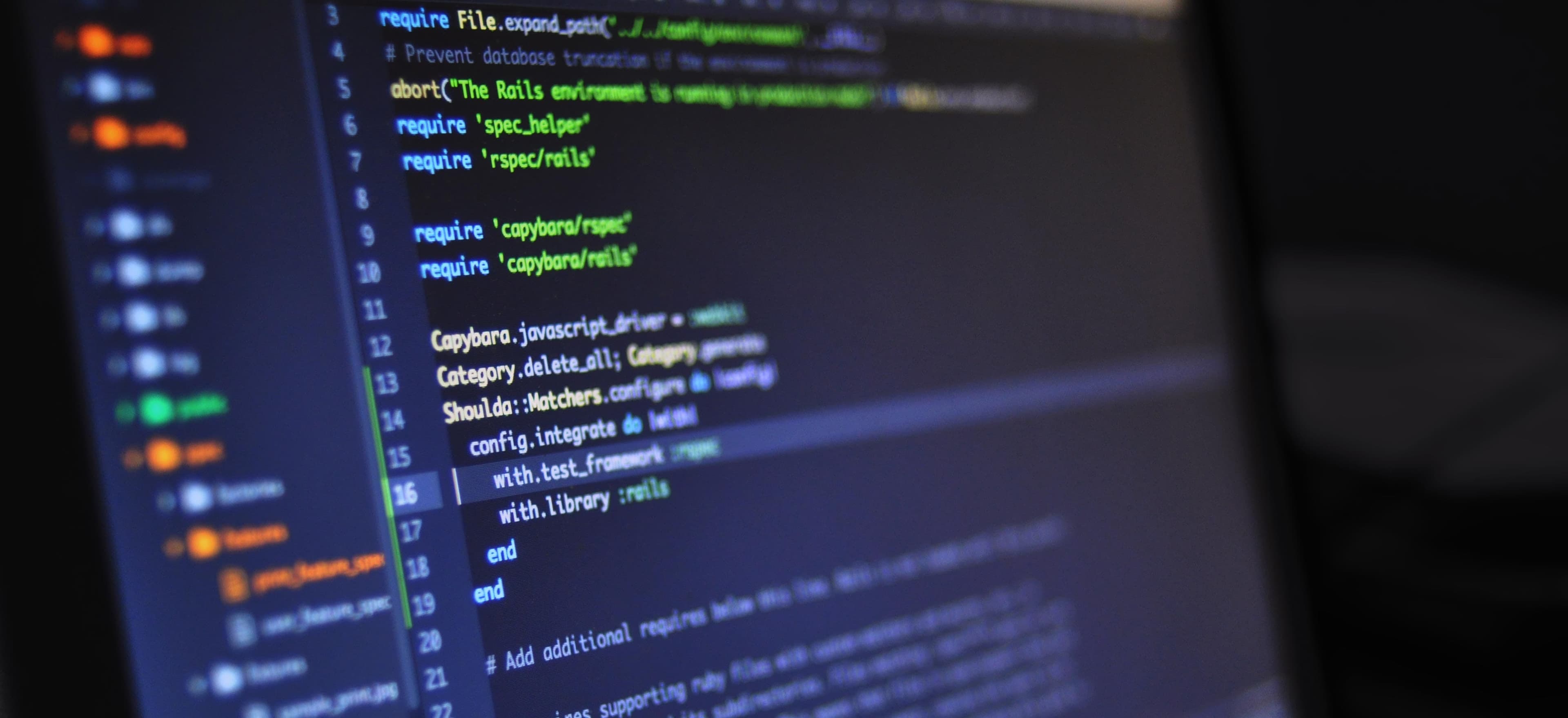
- Published on
Avoiding Common Pitfalls in Serialization Proxy Pattern
Serialization in Java plays a crucial role in object persistence, enabling the conversion of an object's state into a byte stream, making it easier to save or transmit objects. However, when it comes to serialization, several challenges arise, especially regarding security, compatibility, and performance. The Serialization Proxy Pattern is a well-established design pattern aimed at addressing these challenges. In this blog post, we will explore the Serialization Proxy Pattern, its benefits, and common pitfalls to avoid when implementing it.
What is the Serialization Proxy Pattern?
The Serialization Proxy Pattern uses an intermediary object, a proxy, to control the serialization and deserialization process. Instead of directly serializing the object, you serialize the proxy. This offers several advantages:
- Encapsulation of the Serialization Logic: You can hide sensitive parts of the object or its internal state.
- Control over Serialization: You decide what gets serialized and how, helping to maintain compatibility across different versions of a class.
- Increased Security: Reduces the risk associated with exposing the internals of your class during serialization.
How Does it Work?
To implement the Serialization Proxy Pattern, you typically follow these steps:
- Create a private static inner class (the proxy).
- Have the proxy implement
Serializable
. - In the proxy, define the fields that need to be serialized.
- In the original class, override the
writeReplace
andreadResolve
methods to facilitate usage of the proxy.
Example Code
Here is an illustrative example to clarify how this pattern works.
import java.io.*;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private String password; // sensitive data not to be serialized
public User(String username, String password) {
this.username = username;
this.password = password;
}
// This method returns the serialization proxy
private Object writeReplace() {
return new UserProxy(username);
}
// Factory method for creating a new User object from the proxy
private Object readResolve() {
return this; // In a real scenario, you would return a new User instance if needed
}
private static class UserProxy implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
public UserProxy(String username) {
this.username = username;
}
// When deserializing, create a new User object
private Object readResolve() {
// Logic to create a User instance
return new User(username, null); // `null` for sensitive data
}
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
}
Code Breakdown
Encapsulation: The sensitive password
field does not get serialized when the User
object is written to the stream. Instead, only the username
is serialized via the UserProxy
.
Control of State: The writeReplace
method ensures that a UserProxy
instance is serialized instead of the User
instance. This allows you to control precisely what state is saved.
Deserialization Logic: The readResolve
method in the UserProxy
class allows creating a new instance of User
without requiring sensitive data.
Common Pitfalls in the Serialization Proxy Pattern
Implementing the Serialization Proxy Pattern is not without its challenges. Below are some common pitfalls and how to avoid them.
1. Forgetting to Implement Serializable
Ensure that your proxy class (the inner class) implements Serializable
. Failing to do so will lead to a java.io.NotSerializableException
when attempting to serialize.
2. Ignoring serialVersionUID
Always declare a serialVersionUID
for both your main class and proxy class. Java uses this ID during the deserialization process to ensure that a loaded class matches the serialized version.
Example:
private static final long serialVersionUID = 1L; // unique for the version
3. Serialization of Non-Serializable Fields
If your original class contains references to other objects that are not serializable, this will lead to an error during serialization. You must avoid including such fields. Use transient fields to mark them explicitly.
Example:
private transient SomeNonSerializableClass nonSerializableField;
4. Mismanaging State during Deserialization
Ensure that your readResolve
method does not inadvertently return an incorrect state. Returning a new object without proper initialization may lead to inconsistent application behavior.
5. Lack of Versioning Support
Changes to the parent class must be handled properly to maintain backward compatibility. This means updating how data is serialized in the proxy and managing discrepancies between versions.
6. Performance Overhead
Creating an extra class (proxy) might seem like unnecessary overhead, but it's worth it for the security and flexibility it provides. However, always measure the impact of your design on performance during serialization, especially when dealing with large object graphs.
Final Considerations
In conclusion, the Serialization Proxy Pattern is a powerful technique for managing serialization in Java, enhancing security and maintainability. By encapsulating the serialization logic, developers can retain control over how objects are serialized and deserialized, preventing unintentional exposure of sensitive information.
To successfully implement this design pattern, it is essential to be aware of and avoid common pitfalls, such as forgetting to declare serialVersionUID
, ignoring the non-serializable fields, and managing the state appropriately during deserialization.
For more information on serialization best practices, you can refer to the Java documentation on serialization here.
By adhering to these guidelines and using the Serialization Proxy Pattern thoughtfully, you will write safer and more robust code in your Java applications.
References
- Effective Java by Joshua Bloch
- Java Documentation on Serialization
- Serialization and Deserialization in Java
Feel free to leave your comments or questions below. Happy coding!
Checkout our other articles