Choosing the Right Exception Handling Method in JUnit
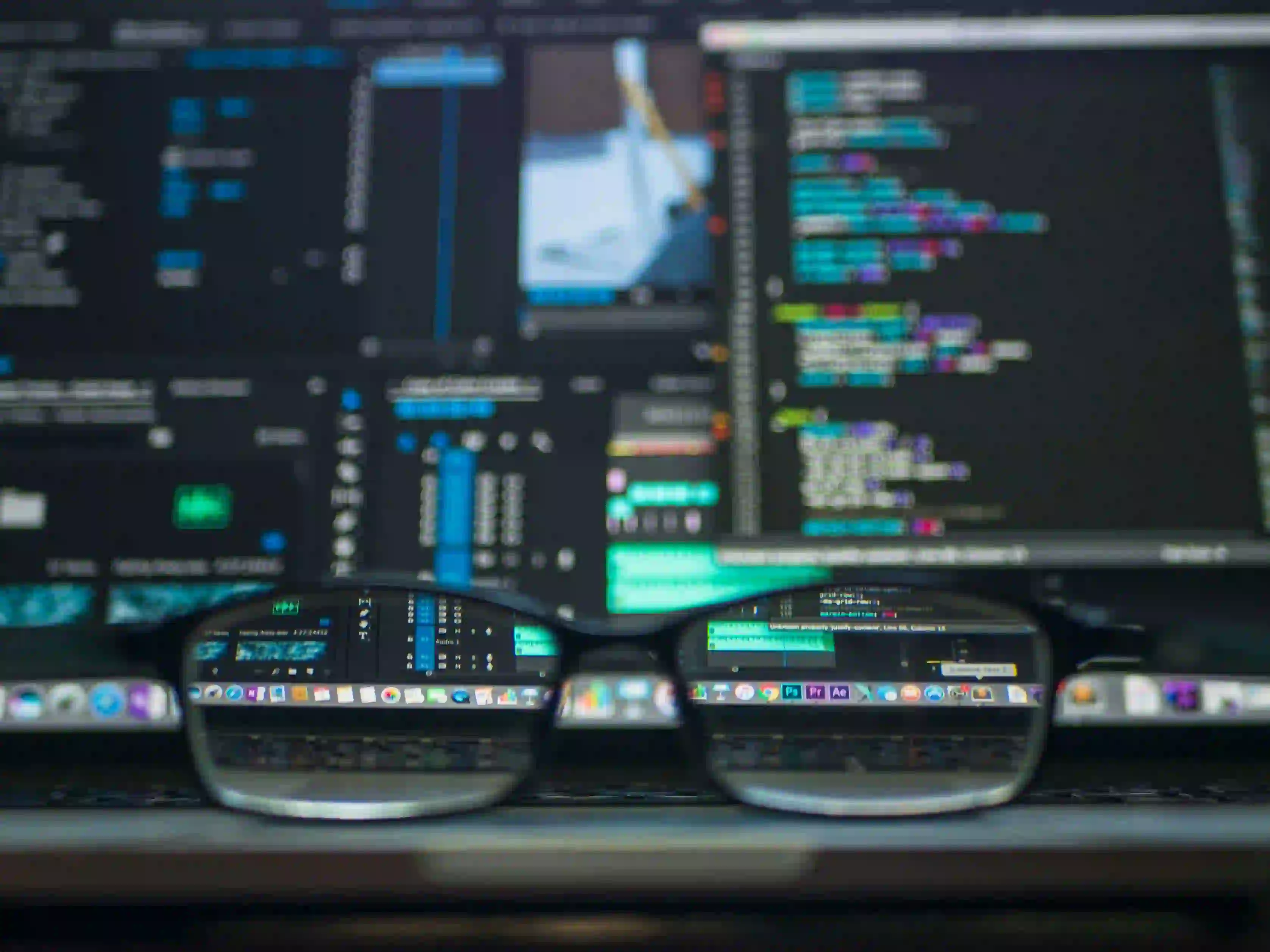
Choosing the Right Exception Handling Method in JUnit
Exception handling in Java is a critical part of developing robust applications. Similarly, testing these applications requires careful consideration of how exceptions are handled during unit testing. JUnit, the widely used testing framework for Java, offers various ways to handle exceptions in your tests. This blog post will explore the different methods available for handling exceptions in JUnit, their implications, and when to use each.
Why Exception Handling is Important
In programming, exceptions are events that can disrupt the normal flow of a program. Proper exception handling ensures that the program can deal with these disruptions gracefully, and testing the existence and quality of exception handling in your code is equally important. Inadequate exception management can lead to uncaught exceptions, program crashes, and ultimately, a poor user experience.
JUnit facilitates testing for exceptions not only to ensure that your application behaves as expected but also to meet quality assurance standards. Therefore, selecting the right exception handling method in JUnit can significantly impact the quality of your tests.
Common Exception Handling Methods in JUnit
JUnit provides several ways to handle exceptions during testing. Let's dive into the most prevalent approaches and highlight their advantages and use cases.
1. Using @Test(expected = ExceptionType.class)
One of the simplest ways to test for exceptions in JUnit is by employing the @Test
annotation with the expected
parameter. This method allows you to specify the type of exception that you expect to be thrown.
Example
import org.junit.Test;
public class ArithmeticTest {
@Test(expected = ArithmeticException.class)
public void divisionByZeroShouldThrowException() {
int result = divide(10, 0);
}
private int divide(int numerator, int denominator) {
return numerator / denominator; // This will trigger an ArithmeticException
}
}
Discussion
In this example, the divisionByZeroShouldThrowException
method is designed to test the behavior of a division operation where the denominator is zero. The test passes if an ArithmeticException
is thrown and fails if it is not. This method is straightforward and works well for simple test cases.
When to Use:
- Use this approach when you expect a specific exception and do not need to inspect the exception's properties.
- It is suitable for simple tests and straightforward exception handling scenarios.
2. Using try-catch
Blocks
Another common method to handle exceptions in JUnit is by using try-catch
blocks. This approach allows for more granular control over exception handling and lets you inspect exception details.
Example
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void divisionByZeroShouldThrowException() {
try {
divide(10, 0);
} catch (ArithmeticException e) {
assertEquals("Division by zero is not allowed.", e.getMessage());
return; // Return successfully because the exception was caught
}
throw new AssertionError("Expected ArithmeticException not thrown");
}
private int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new ArithmeticException("Division by zero is not allowed.");
}
return numerator / denominator;
}
}
Discussion
In this example, we use a try-catch
block to catch an ArithmeticException
. We can further check the exception's message to ensure the error handling code is appropriate. If the exception isn't thrown, we manually throw an AssertionError
, indicating that the test has failed.
When to Use:
- This approach is beneficial when you need to verify specific properties of the thrown exception, such as the message or type.
- It works well for complex scenarios where you want to conditionally handle exceptions or perform additional logic.
3. Using JUnit's AssertThrows
If you're using JUnit 5, the Assertions.assertThrows
method provides a modern and concise way to test for exceptions. This method allows you to assert an exception is thrown and inspect it directly without using a try-catch
.
Example
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertThrows;
public class MathUtilsTest {
@Test
public void divisionByZeroShouldThrowException() {
ArithmeticException exception = assertThrows(ArithmeticException.class, () -> {
divide(10, 0);
});
assertEquals("Division by zero is not allowed.", exception.getMessage());
}
private int divide(int numerator, int denominator) {
if (denominator == 0) {
throw new ArithmeticException("Division by zero is not allowed.");
}
return numerator / denominator;
}
}
Discussion
The assertThrows
method takes two parameters: the expected exception class and an executable that contains the code that should throw the exception. If the specified exception is thrown, it can also return the exception instance for further assertions.
When to Use:
- This method is recommended when you are using JUnit 5.
- It is advantageous for cleaner, more readable tests and allows for exception inspection without boilerplate code.
Special Considerations
- Custom Exceptions: If your application uses custom exceptions, you can apply the same strategies mentioned above. Just specify your custom exception class in place of
ArithmeticException
. - Multiple Exceptions: If a method might throw multiple different exceptions, you may use multiple test cases or combine approaches.
- Performance: When testing performance-intensive methods, consider how exceptions might impact timing and resource management.
The Closing Argument
Selecting the right method for exception handling in JUnit can significantly improve the maintainability and clarity of your tests. The key is to choose the method that best suits your testing goals:
- Use
@Test(expected = ExceptionType.class)
for straightforward exception checking. - Use
try-catch
blocks when you need more detail about the exception and control over the testing flow. - Utilize
assertThrows
in JUnit 5 for cleaner, more concise assertions.
As with all coding practices, familiarity with these methods will empower you to write better tests and, ultimately, more robust code. For more in-depth insights on exception handling in Java, you can visit The Java™ Tutorials and JUnit 5 Documentation.
As you refine your testing skills, remember that effective exception handling is indispensable—not just in your tests, but as a fundamental principle of software design. Happy testing!