Struggling to Choose Java Libraries? Here’s Your Guide!
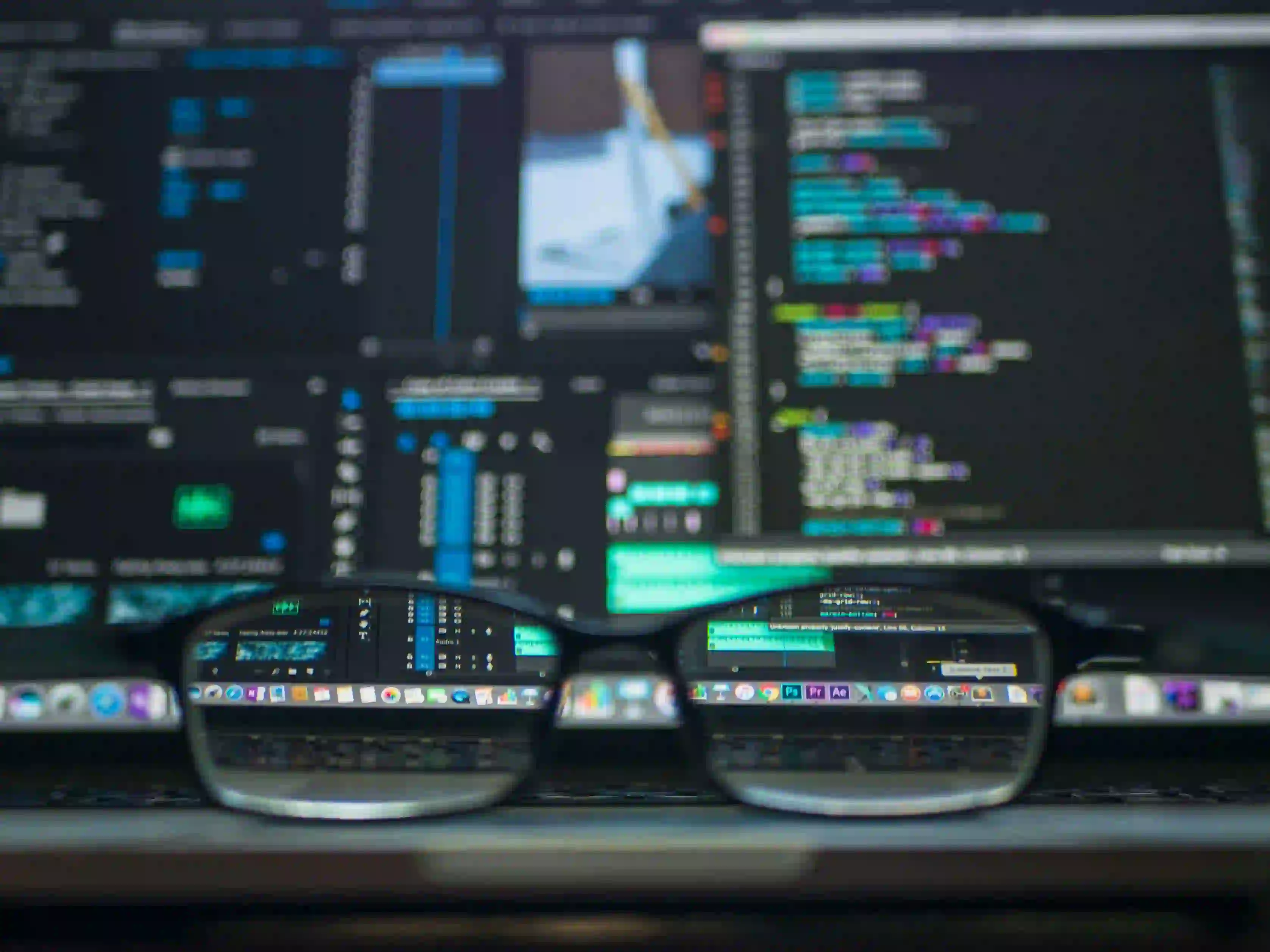
Struggling to Choose Java Libraries? Here’s Your Guide!
Java is one of the most versatile programming languages available today, widely used in everything from web applications to mobile devices. With its extensive ecosystem, Java developers often face the daunting task of selecting suitable libraries to enhance their projects. Whether you're working on a small personal project or a large-scale enterprise application, utilizing the right libraries can drastically improve your productivity and code quality. In this blog post, we will explore essential Java libraries and offer guidance on how to make the best choices.
What is a Java Library?
A Java library is a collection of pre-written code that developers can use to optimize their applications. These libraries provide reusable functions or classes to simplify complex tasks, ranging from database connectivity to user interface design. For example, instead of writing your own code for JSON handling, you can leverage libraries like Jackson or Gson.
The Importance of Choosing the Right Libraries
Selecting the right libraries can have a profound impact on:
- Development Speed: Libraries save time by eliminating the need to write boilerplate code.
- Code Quality: Popular libraries are often well-tested, reducing bugs in your application.
- Maintainability: Utilizing commonly used libraries ensures better documentation and community support.
- Performance: Libraries can be optimized for speed and efficiency at scale.
Categories of Java Libraries
Before diving into specific libraries, it's helpful to categorize them based on functionalities. Here are some of the main types:
- Web Development: Libraries that facilitate the development of web applications.
- Data Processing: Libraries designed for handling data manipulation.
- Testing: Libraries to assist in writing unit tests.
- Dependency Injection: Libraries that manage how components in a system interact.
- Concurrency: Libraries focused on various aspects of concurrent programming.
Let’s explore some popular libraries in these categories.
Top Java Libraries for Web Development
Spring Framework
Spring is a widely-used framework for building enterprise applications. It provides features like dependency injection, aspect-oriented programming, and transaction management.
@Configuration
public class AppConfig {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
The @Configuration
annotation indicates that this class is a source of bean definitions. The @Bean
annotation tells Spring to manage the lifecycle of myBean
. This helps you automatically manage dependency injections without verbose code.
Why Spring? It simplifies the development process and is modular enough that you can use it as much or as little as you need.
JavaServer Faces (JSF)
JSF is another popular framework that simplifies UI development for web applications. It provides reusable UI components and integrates seamlessly with Java EE.
<h:form>
<h:inputText value="#{bean.inputValue}" />
<h:commandButton value="Submit" action="#{bean.submit}" />
</h:form>
JSF uses a component-centric model, making your web pages more maintainable. This XML-like syntax allows you to create elegant forms without diving deep into HTML.
Why JSF? If you want a framework designed specifically for component-based UIs on the server-side, JSF is a great choice.
Libraries for Data Processing
Apache Commons
The Apache Commons library boasts a suite of reusable Java components. One specific module, Commons Lang, provides utilities for working with Java core classes.
String str = "Hello, World!";
String reversed = StringUtils.reverse(str);
System.out.println(reversed); // Outputs: !dlroW ,olleH
In this example, StringUtils.reverse()
is used from Apache Commons to quickly reverse a string. This operation is concise, showcasing the library's utility methods that reduce the amount of custom code you have to write.
Why Apache Commons? It's highly optimized, widely used, and offers an extensive range of functionalities that can simplify your Java programming endeavors.
Google Guava
Guava is another powerful library that provides collections, caching, primitives support, concurrency libraries, and more.
ImmutableList<String> immutableList = ImmutableList.of("Apple", "Banana", "Cherry");
for (String fruit : immutableList) {
System.out.println(fruit);
}
Using ImmutableList
, you can create a list that cannot be modified. This provides safety from accidental changes in your collections.
Why Guava? It's designed for performance and simplicity, making it easier to work with Java collections and concurrency.
Testing Libraries
JUnit
JUnit is the de facto library for unit testing in Java. Writing tests with JUnit is straightforward.
import org.junit.Test;
import static org.junit.Assert.*;
public class MyTests {
@Test
public void testAddition() {
assertEquals(5, 2 + 3);
}
}
JUnit allows you to write simple tests with annotations like @Test
, drastically improving the structure and maintainability of your tests.
Why JUnit? Its simplicity, coupled with extensive documentation and community support, makes it the go-to choice for Java testing.
Mockito
Mockito is a popular mocking framework that integrates well with JUnit.
import static org.mockito.Mockito.*;
@Test
public void testService() {
MyService myService = mock(MyService.class);
when(myService.getData()).thenReturn("Mocked Data");
assertEquals("Mocked Data", myService.getData());
}
Here, mock()
creates a mock object of MyService
, allowing you to define behaviors using when()
.
Why Mockito? It simplifies the testing of interactions, ensuring that your unit tests remain focused on the logic you want to validate rather than the clutter of dependencies.
Libraries for Dependency Injection
Dagger
Dagger is a compile-time dependency injection framework that is used for Java and Android.
@Component
interface UserComponent {
User getUser();
}
@Module
class UserModule {
@Provides
User provideUser() {
return new User("John Doe");
}
}
In this Dagger example, components and modules are established to provide dependencies where needed.
Why Dagger? Its powerful compile-time checks ensure that you get the benefits of dependency injection without the runtime cost.
Libraries for Concurrency
RxJava
RxJava is an implementation of the reactive programming paradigm that simplifies asynchronous programming.
Observable.just("Hello", "World")
.map(String::toUpperCase)
.subscribe(System.out::println);
This code creates an observable for a list of strings, transforming them to uppercase asynchronously.
Why RxJava? It offers a powerful way to work with asynchronous data streams without blocking your application.
Lessons Learned
Choosing the right Java libraries can make all the difference in the success and maintainability of your application. As we've discussed, libraries like Spring, JSF, Apache Commons, Guava, JUnit, Mockito, Dagger, and RxJava offer a wide range of functionalities that cater to various aspects of development.
Before selecting any library, consider factors such as:
- Community Support: Check if the library is well-documented and supported.
- Performance: Evaluate whether the library meets your performance requirements.
- Updates and Stability: Use libraries that are actively maintained and provide regular updates.
- Ease of Learning: Opt for libraries with a less steep learning curve, especially if you are new to Java.
By strategically choosing libraries that align with the needs of your projects, you can focus on what truly matters: building great applications! For additional insights, you can visit resources like Baeldung for Java tutorials and Maven Central to explore library options.
Happy coding!