Overcoming Latency Issues in Real-Time Uber Data Analysis
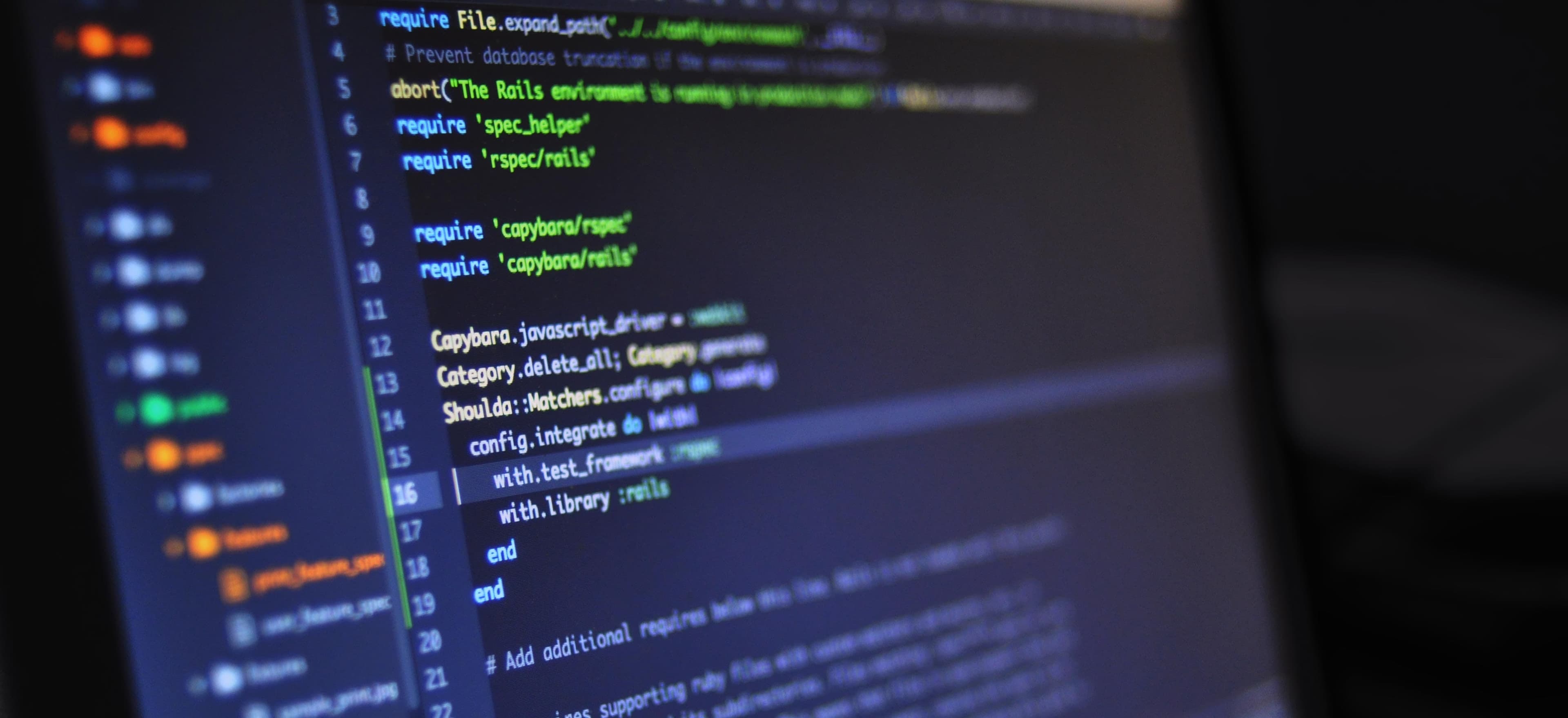
- Published on
Overcoming Latency Issues in Real-Time Uber Data Analysis
In today's fast-paced world, real-time data analysis is becoming crucial for businesses. For example, Uber, with its complex logistics and dynamic pricing model, relies heavily on real-time data processing. Latency issues can significantly hinder the effectiveness of such analyses. In this blog post, we will explore various strategies to overcome these latency challenges, specifically in the context of Uber's data analysis.
Understanding Latency in Real-Time Data Analysis
Latency refers to the delay before a transfer of data begins following an instruction. In real-time analytics, minimizing latency is vital for making timely decisions. High latency can lead to outdated information, which in turn might result in poor decision-making. The key is to identify where the latency occurs—whether it be in data collection, processing, or dissemination.
Types of Latency
- Data Ingestion Latency: The time taken to collect and input data for analysis.
- Processing Latency: The delay in processing data, typically due to algorithms or data access issues.
- Network Latency: The time it takes for data to travel across a network.
Strategies to Overcome Latency Issues
1. Utilizing Stream Processing Frameworks
Stream processing allows data to be processed in real-time as it arrives, instead of waiting for a batch of data. Frameworks like Apache Kafka and Apache Flink can help process events as they occur.
Example using Apache Kafka
Here is a simple illustration of how you can produce and consume messages using Kafka.
// Produce Data to Kafka
Properties properties = new Properties();
properties.put("bootstrap.servers", "localhost:9092");
properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
KafkaProducer<String, String> producer = new KafkaProducer<>(properties);
producer.send(new ProducerRecord<>("uber-data", "key", "value"));
producer.close();
Why Use Kafka? Kafka’s distributed architecture minimizes the risk of bottlenecks, allowing you to handle streams of data efficiently.
2. In-Memory Data Grids
In-memory data grids (IMDG) allow data to be stored and processed in memory, significantly improving access times.
Example using Hazelcast
// Initialize Hazelcast Instance
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance();
IMap<String, UberData> uberMap = hazelcastInstance.getMap("uberMap");
// Store Data in Memory
uberMap.put(driverId, uberData);
Why Use IMDG? They reduce the time spent on I/O operations, which is often a bottleneck in traditional data processing architectures.
3. Optimizing Algorithms
Inefficient algorithms can cause delays in data processing. Review your algorithms and make performance optimizations where necessary.
Example of Using Concurrent Collections
Using ConcurrentHashMap
can improve access times when dealing with cached data.
// Using ConcurrentHashMap
ConcurrentHashMap<String, UberData> concurrentMap = new ConcurrentHashMap<>();
concurrentMap.put(driverId, uberData);
Why Optimize Algorithms? Algorithms that can handle concurrent processing will vastly improve the throughput of data handling.
4. Enhanced Data Compression Techniques
Reducing the size of the data being transmitted can also mitigate network latency. Use efficient serialization and compression techniques.
Example using Snappy for Data Compression
import org.xerial.snappy.Snappy;
// Compressing Data
byte[] compressedData = Snappy.compress(data.getBytes());
// Decompressing Data
byte[] decompressedData = Snappy.uncompress(compressedData);
Why Use Compression? This reduces the volume of data sent over the network, lowering the time taken for transmission without compromising the quality of data.
5. CDNs for Geographically Distributed Data
Content Delivery Networks (CDNs) can help in distributing the data closer to the user, reducing the time it takes to access the information.
Why Use CDNs? By reducing geographic latency, CDNs help deliver data faster, making real-time analysis more efficient for global operations.
In Conclusion, Here is What Matters
Latency in real-time data analysis—especially in organizations like Uber—can present serious challenges. However, employing strategies such as stream processing, in-memory data grids, optimized algorithms, data compression, and leveraging CDNs can greatly enhance performance.
By understanding the nuances of latency and implementing these strategies, businesses can ensure they have the tools needed to harness their data effectively. Improved analytical capabilities will not only lead to better decision-making but also drive enhanced customer experiences.
Further Reading
If you're interested in learning more, consider examining the following resources:
- Apache Kafka Documentation
- Hazelcast Documentation
- Snappy Compression Library
By focusing on these aspects, organizations can take proactive steps to mitigate latency challenges in their data analysis processes, thus maintaining their competitive edge in the market.
Checkout our other articles