Mastering Java Spring Microservices: Tackle These 30 FAQs
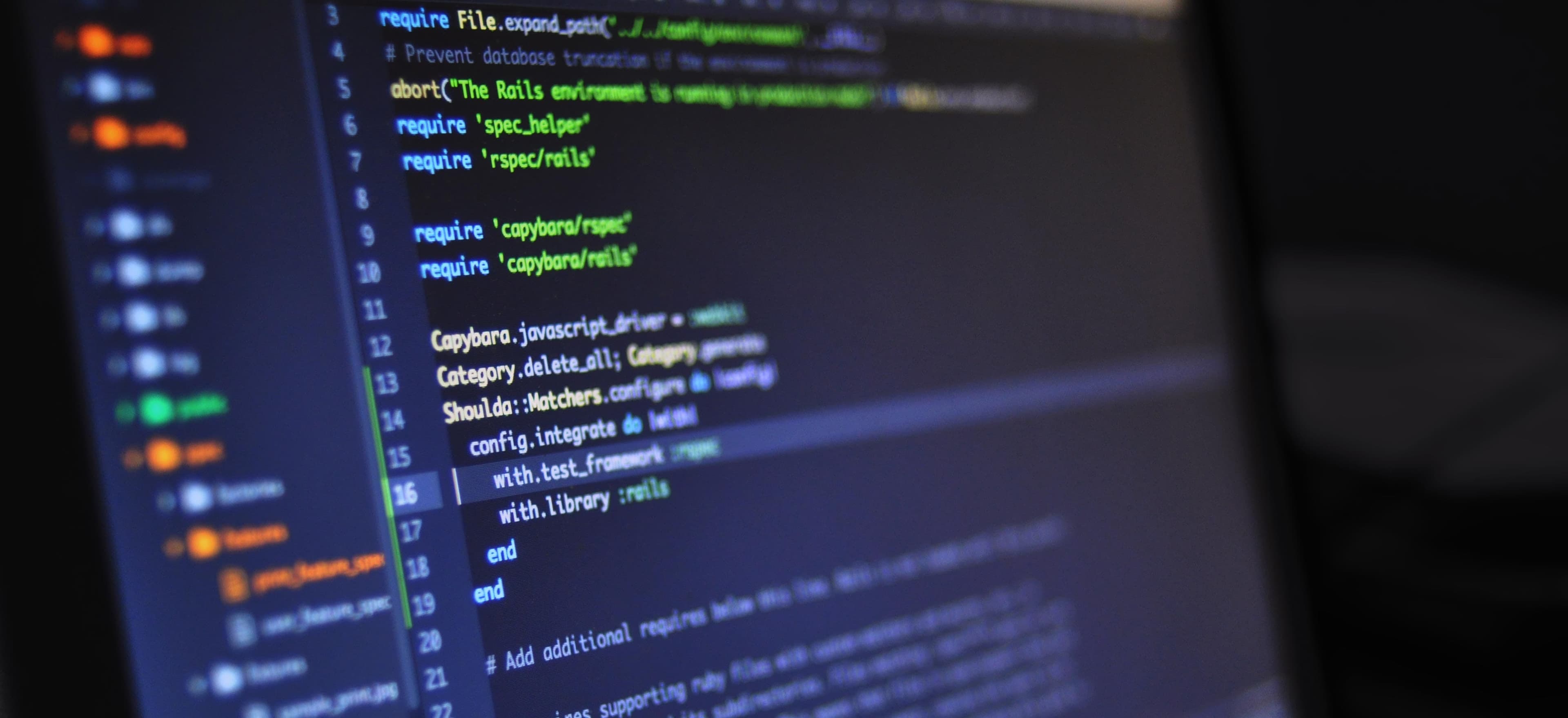
- Published on
Mastering Java Spring Microservices: Tackle These 30 FAQs
Microservices architecture has transformed the way we build software, breaking down applications into smaller, manageable services. In the Java ecosystem, Spring Framework plays a pivotal role in simplifying the development of microservices. Whether you are a seasoned developer or just starting with Java Spring, it’s crucial to have answers to commonly asked questions. This blog post outlines 30 frequently asked questions (FAQs) about Java Spring Microservices, paving a clearer path toward mastery.
Table of Contents
- What is a Microservice?
- Why Use Spring for Microservices?
- How Does Spring Boot Simplify Microservices?
- What is Spring Cloud?
- How to Set Up a Spring Boot Microservice?
- How do Microservices Communicate?
- What is Service Discovery?
- What is API Gateway in Microservices?
- How to Handle Configuration Management?
- What to Consider for Data Management in Microservices?
- How to Secure Microservices?
- How to Monitor and Log Microservices?
- What Are the Advantages of Using Docker with Spring?
- How to Handle Inter-Service Communication?
- What Role Does Spring Data Play?
- How to Implement Circuit Breaker Pattern?
- What is the Role of a Load Balancer?
- How to Handle Fault Tolerance?
- How to Scale Microservices?
- What is the Importance of API Versioning?
- How to Test a Spring Microservice?
- How to Deploy Spring Microservices?
- What is Continuous Integration and Continuous Deployment (CI/CD)?
- How to Handle Caching in Microservices?
- What are Some Common Design Patterns?
- How to Design for Failure?
- How to Optimize Performance?
- What is the Importance of Documentation?
- How to Use GraphQL with Spring?
- Where to Learn More?
Now, let’s dive into each FAQ to explain, clarify, and provide actionable insights.
1. What is a Microservice?
A microservice is an architectural style that structures an application as a collection of small, loosely coupled services. Each service can be developed, deployed, and scaled independently. Microservices align with the principles of agile development, allowing teams to work autonomously on individual services.
2. Why Use Spring for Microservices?
Spring Framework is a popular choice for building microservices due to its flexibility and robustness. The Spring ecosystem has various projects like Spring Boot and Spring Cloud that simplify the development and deployment of microservice-based applications.
3. How Does Spring Boot Simplify Microservices?
Spring Boot removes the complexity of setting up a Spring application. It provides:
- Auto-Configuration: Reduces boilerplate code.
- Embedded Servers: No need for WAR files.
- Production-Ready Features: Health checks, metrics, and externalized configuration.
Code Example: Basic Spring Boot Application
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyMicroserviceApplication {
public static void main(String[] args) {
SpringApplication.run(MyMicroserviceApplication.class, args);
}
}
In this example, @SpringBootApplication
combines several annotations that set up a Spring context, facilitate an embedded server, and allow for component scanning.
4. What is Spring Cloud?
Spring Cloud offers tools for developers to quickly build distributed systems. It provides services like configuration management, service discovery, circuit breakers, and more, making it easier to develop microservices.
5. How to Set Up a Spring Boot Microservice?
Creating a Spring Boot microservice involves:
-
Generating a Maven or Gradle Project: Access Spring Initializr to bootstrap your project with required dependencies.
-
Adding dependencies: In
pom.xml
orbuild.gradle
, include necessary dependencies like Spring Web and Spring Data JPA. -
Creating REST controllers to expose endpoints.
Example of a Simple REST Controller
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
6. How do Microservices Communicate?
Microservices communicate using various protocols, with HTTP/REST and message brokers (like RabbitMQ or Kafka) being the most common. The choice depends on the use case, such as synchronous or asynchronous communication.
7. What is Service Discovery?
Service discovery enables services to find each other without hardcoded endpoint addresses. Tools like Eureka or Consul help manage service registries and discovery.
8. What is API Gateway in Microservices?
An API Gateway serves as a single entry point to a microservices architecture. It handles requests by routing them to the appropriate services, enforcing security, and aggregating results.
9. How to Handle Configuration Management?
Spring Cloud Config provides server and client-side support for externalized configuration in a microservices architecture. You can manage and version your configuration through a central repository.
10. What to Consider for Data Management in Microservices?
Each microservice should have its own database to ensure loose coupling. Consider using Database per Service or Shared Database patterns based on your application's needs.
11. How to Secure Microservices?
Security in microservices can be achieved through several techniques, including:
- OAuth2 / OpenID Connect for authentication.
- API Gateway to implement security policies.
- Service Mesh technologies for mTLS communication.
12. How to Monitor and Log Microservices?
Use monitoring tools like Prometheus and Grafana, along with centralized logging solutions such as ELK Stack (Elasticsearch, Logstash, and Kibana) to aggregate logs from multiple microservices.
13. What Are the Advantages of Using Docker with Spring?
Docker allows you to containerize your microservices, making them easily portable and scalable. Benefits include:
- Consistency across environments.
- Simplified dependency management.
- Efficient resource utilization.
14. How to Handle Inter-Service Communication?
Consider using RESTful APIs for synchronous communication and event-driven architecture for asynchronous communication. Tools like RabbitMQ and Apache Kafka can be beneficial here.
15. What Role Does Spring Data Play?
Spring Data simplifies database interactions in Spring applications. With repositories, you can read, update, delete, and query data with minimal boilerplate.
Example: Basic Repository Interface
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
This interface allows for CRUD operations without implementing methods manually, thanks to Spring Data's magic.
16. How to Implement Circuit Breaker Pattern?
Using Resilience4j or Hystrix, you can implement circuit breakers to prevent cascading failures in microservices by ensuring that if a service fails, the circuit will open, stopping further calls.
Circuit Breaker Example with Resilience4j
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import io.github.resilience4j.circuitbreaker.annotation.CircuitBreaker;
@RestController
public class UserService {
@GetMapping("/user")
@CircuitBreaker
public User getUser() {
// Call to an external or internal service
}
}
With this annotation, the getUser()
method is protected from faults.
17. What is the Role of a Load Balancer?
Load balancers distribute incoming traffic across multiple instances of a service. This helps avoid overloading single instances, improving application performance and reliability.
18. How to Handle Fault Tolerance?
Fault tolerance can be achieved through design patterns, circuit breakers, and retries. Techniques like bulkheads can isolate failures within a system, ensuring that not all services are affected by one failure.
19. How to Scale Microservices?
You can scale microservices by horizontally scaling the instances behind a load balancer. Container orchestration tools like Kubernetes facilitate automated scaling based on demand.
20. What is the Importance of API Versioning?
API versioning ensures backward compatibility, allowing clients to adopt new features without breaking existing functionality. Common practices include URL versioning and request header versioning.
21. How to Test a Spring Microservice?
Testing microservices can be approached using:
- Unit Tests: For individual components.
- Integration Tests: For service interactions.
- Contract Tests: To validate service contracts.
You can utilize libraries like JUnit and Mockito to write effective tests.
22. How to Deploy Spring Microservices?
Spring Microservices can be deployed on cloud platforms like AWS, Azure, or Google Cloud, or through container orchestration with Docker and Kubernetes.
23. What is Continuous Integration and Continuous Deployment (CI/CD)?
CI/CD pipelines automate the testing and deployment of applications, enabling rapid iterations and quicker releases. Tools like Jenkins and GitHub Actions streamline CI/CD processes for Spring applications.
24. How to Handle Caching in Microservices?
Caching can be used to enhance performance. Use popular caching solutions like Redis or Ehcache to cache frequently accessed data, reducing latency and database load.
25. What are Some Common Design Patterns?
Popular design patterns in microservices include:
- Saga Pattern for managing distributed transactions.
- Strangler Fig Pattern for gradual migration.
- Event Sourcing for capturing changes as a sequence of events.
26. How to Design for Failure?
Embrace the idea that failures will happen. Design with redundancies, use circuit breakers, and implement retries with exponential backoff strategies.
27. How to Optimize Performance?
Monitor your services regularly and employ techniques such as load testing, query optimization, and optimizing service interactions (e.g., minimizing network calls).
28. What is the Importance of Documentation?
Accurate documentation is crucial in microservices architecture for onboarding new developers and ensuring clarity between teams. Use tools like Swagger/OpenAPI for API documentation.
29. How to Use GraphQL with Spring?
Integrating GraphQL in a Spring application allows clients to request data more flexibly. You can use Spring Boot Starter GraphQL to build APIs that offer a more dynamic querying language.
30. Where to Learn More?
To further expand your knowledge on Spring Microservices, consider exploring the following resources:
- Spring Microservices in Action
- Building Microservices by Sam Newman
Final Considerations
Mastering Java Spring Microservices requires understanding both fundamental concepts and specific technologies within the Spring ecosystem. From building scalable services to effectively managing them, a structured approach will guide you through the complexities of microservice architectures. Dive deep into each topic, implement examples, and leverage community resources for a comprehensive learning experience.
As microservices continue to evolve, staying updated with best practices and tools will be invaluable for your development journey. Happy coding!
Checkout our other articles