Overcoming Common CI/CD Bottlenecks in DevOps Implementation
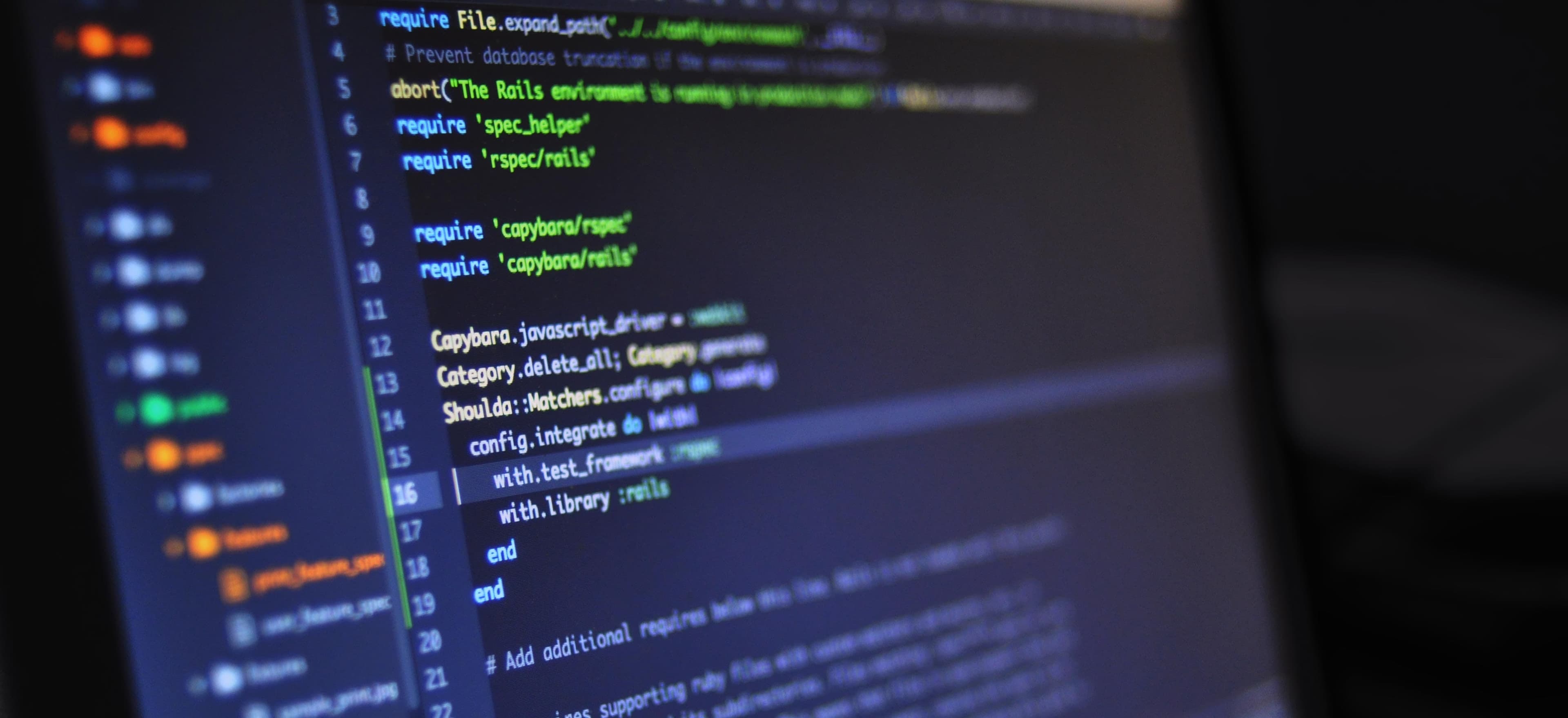
- Published on
Overcoming Common CI/CD Bottlenecks in DevOps Implementation
The advent of DevOps has transformed software development and deployment, making it more efficient and reliable. Continuous Integration (CI) and Continuous Deployment (CD) are key components of this transformation. However, organizations often face bottlenecks in their CI/CD pipelines that can hamper productivity and efficiency. In this blog post, we will explore common CI/CD bottlenecks and offer practical solutions to overcome them.
Understanding CI/CD Bottlenecks
Before tackling the bottlenecks, it's essential to understand what CI/CD entails. CI involves integrating code changes frequently and validating them through automated tests. CD extends this by ensuring that these validated changes are automatically deployed to production environments.
Bottlenecks can occur at various stages of the CI/CD pipeline, often due to manual processes, poor tool integration, or inefficient testing procedures. Let's dive deeper into these challenges and discover how to resolve them.
1. Slow Build Times
The Problem
Slow build times are among the most prevalent bottlenecks in CI/CD pipelines. As projects grow in size and complexity, longer build times can hinder rapid feedback and deployment. This can frustrate developers and lead to diminished productivity.
Solutions
-
Parallel Builds: Implement parallel builds to speed up the process. Tools like Jenkins allow parallel execution of jobs, thus reducing overall build time.
pipeline { agent any stages { stage('Build') { parallel { stage('Build Service A') { steps { sh 'cd serviceA && ./build.sh' } } stage('Build Service B') { steps { sh 'cd serviceB && ./build.sh' } } } } } }
Why it works: By executing multiple builds concurrently, you significantly cut the time required for the overall build process.
-
Incremental Builds: Use tools that support incremental builds. This means only changes since the last build are compiled, rather than the entire codebase.
2. Insufficient Test Coverage
The Problem
Often, teams may not achieve the desired level of test coverage, resulting in gaps that may go unnoticed until deployment. Insufficient tests can lead to critical errors hitting production.
Solutions
-
Automated Testing: The first step is to automate your testing. Use frameworks like JUnit for unit testing or Selenium for end-to-end tests.
import org.junit.Test; import static org.junit.Assert.*; public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
Why it works: Automated tests allow for consistent validation of the codebase, enabling developers to catch errors early and often.
-
Continuous Feedback: Implement automated feedback loops where tests are run with every code commit. This practice ensures that any deviations are immediately flagged.
3. Deployment Failures
The Problem
Deployment failures can occur due to various reasons such as environment inconsistencies, dependency issues, or lack of rollback processes. Frequent failures can lead to significant downtime and trust issues.
Solutions
-
Containerization: Use containerization tools like Docker to create consistent environments across development, testing, and production.
Dockerfile Example:
FROM openjdk:11 COPY target/my-app.jar my-app.jar ENTRYPOINT ["java","-jar","/my-app.jar"]
Why it works: By encapsulating your application in a Docker container, you ensure that it behaves the same regardless of where it runs.
-
Canary Releases and Blue-Green Deployments: These deployment strategies allow for safer rollouts. By deploying to a subset of users first (canary releases) or switching between environments (blue-green deployments), problems can be detected before the entire user base is affected.
4. Poor Tool Integration
The Problem
Many organizations use a mix of tools that may not integrate well, leading to manual interventions, additional error potential, and increased time to deploy.
Solutions
-
Choosing the Right Tools: Opt for tools that can work seamlessly together. For CI/CD, platforms like GitLab and Jenkins provide plugins and built-in integration capabilities.
-
API Automation: Utilize APIs to ensure that different systems can communicate effectively. For example, integrating issue-tracking tools with CI servers ensures that any failed builds automatically flag relevant issues.
5. Lack of Monitoring and Feedback
The Problem
A prevalent issue in many CI/CD pipelines is the lack of monitoring following deployment. Without effective feedback channels, it becomes difficult to identify problems when applications are live.
Solutions
-
Live Monitoring and Alerts: Use monitoring tools like Prometheus or Grafana to keep an eye on application performance and user experience.
Example Monitoring Config:
global: scrape_interval: 15s scrape_configs: - job_name: 'my-app' static_configs: - targets: ['localhost:8080']
Why it works: Continuous monitoring enables real-time insights into application performance, allowing teams to react swiftly to issues.
-
Automated Rollbacks: Implement strategies that allow your application to revert to a stable state automatically upon detecting failures.
To Wrap Things Up
Overcoming CI/CD bottlenecks is crucial for organizations striving for efficiency and reliability in their software delivery processes. By focusing on enhancing build performance, ensuring adequate testing, adopting better deployment strategies, improving tool integration, and setting up robust monitoring, teams can significantly improve their CI/CD implementation.
Remember, the journey to a smooth CI/CD pipeline is continuous. Regularly review and iterate your processes based on team feedback and technological advancements. For more information on establishing a robust DevOps culture, check out DevOps for Dummies or explore Continuous Delivery concepts that further enhance the understanding of CI/CD practices.
With these strategies in place, you can build a more effective CI/CD pipeline that drives your DevOps efforts forward.
This blog post aims to guide developers, engineers, and organizations in overcoming CI/CD bottlenecks efficiently, reinforcing the importance of continuous improvement in software deployment processes.