Challenges in Packaging Spring Boot as a WAR File
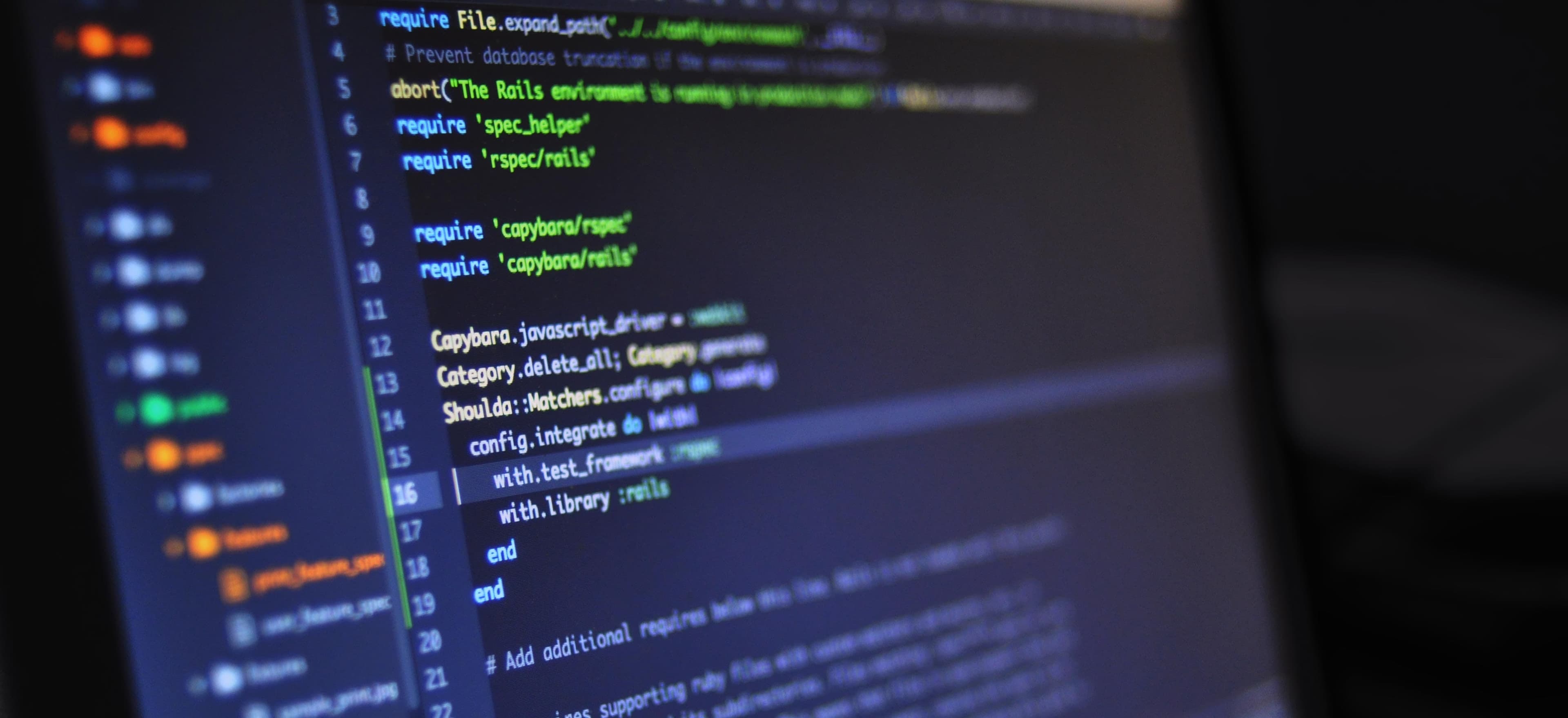
- Published on
Challenges in Packaging Spring Boot as a WAR File
Spring Boot has gained immense popularity for its convenience and efficiency in building stand-alone, production-grade applications. By default, Spring Boot applications are packaged as JAR files. However, there are scenarios where packaging a Spring Boot application as a WAR (Web Application Archive) file is necessary—especially for deployment on traditional application servers such as Tomcat or JBoss.
In this post, we will explore the challenges you may encounter when packaging Spring Boot applications as WAR files, discuss strategies to overcome these challenges, and provide illustrative code snippets for better understanding.
Understanding the Need for WAR Packaging
Before diving into the challenges, let’s clarify why one might want to package a Spring Boot application as a WAR file.
-
Legacy Systems: Many enterprises still rely on application servers for managing their Java applications. Adapting a Spring Boot application to fit in these existing infrastructures often requires WAR packaging.
-
Resource Management: Some organizations prefer using centralized management for logging, monitoring, and resource allocation, which traditional servers provide.
-
Upgradability: In very large applications that comprise multiple microservices, an organization might opt for WAR files to ensure compatibility with a standardized deployment process.
Now that we understand the motivation, let’s explore the challenges associated with WAR packaging.
1. Project Structure
Challenge
Spring Boot applications default to a structure conducive to JAR packaging, with the embedded server being a significant component in this structure. When switching to WAR packaging, the project structure needs some reorganization.
Solution
To transition smoothly, update the project structure to conform to the standard for WAR files. You will typically need to do two primary things:
- Change the
spring-boot-starter-web
dependency to includespring-boot-starter-tomcat
as provided scope. - Modify your application's main class to extend
SpringBootServletInitializer
.
Here is an example of the modified pom.xml
file:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
</dependencies>
And here's the main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
@SpringBootApplication
public class MySpringBootApplication extends SpringBootServletInitializer {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
Why?
By following these steps, you ensure that your Spring Boot application can be correctly packaged as a WAR file and deployed on an external container.
2. Handling External Configuration
Challenge
Spring Boot applications rely heavily on the convention-over-configuration paradigm, handling most configurations easily through application.properties
or application.yml
files. Transitioning to a WAR file can complicate matters, especially in externalized configuration.
Solution
Utilize the features of Spring Profiles to manage different configurations for your application. To implement this, you can create multiple application-{profile}.properties
files, and include the relevant method in your application to select the profile.
# Run your application with a specific profile
java -jar myapp.war --spring.profiles.active=development
Why?
Spring Profiles make it easy to switch configurations that can be tailored based on the environment (development, testing, production) where the WAR file is deployed. This flexibility is crucial in enterprise environments.
3. Classpath Issues and Dependencies
Challenge
In a JAR file, Spring Boot packages everything in a flat structure. In a WAR file, classloader hierarchy changes and can lead to compatibility issues, especially concerning dependencies.
Solution
Use the provided
scope for dependencies intended to be managed by the server, as shown in the earlier pom.xml
snippet. Additionally, consider shading or relocating dependencies with tools such as the Maven Shade Plugin to resolve conflicts. Here's an example configuration:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-shade-plugin</artifactId>
<version>3.2.4</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>shade</goal>
</goals>
<configuration>
<relocations>
<relocation>
<pattern>org.springframework.boot</pattern>
<shadedPattern>my.shadow.org.springframework.boot</shadedPattern>
</relocation>
</relocations>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
Why?
Shading allows you to bundle dependencies in such a way that they won't conflict with the server's existing libraries or those of other deployed applications.
4. Embedded vs. Standalone Containers
Challenge
Spring Boot allows for the embedding of the application server, which makes it incredibly versatile and easy to run. However, this can lead to confusion when transitioning to a WAR file, especially since the same features won't be available.
Solution
Ensure to understand the lifecycle and executor model of your target application server. If you're deploying to Tomcat or JBoss, familiarize yourself with their specific configurations, such as web.xml
or server.xml adjustments necessary for your Spring Boot application.
Here's an example of how to define a web.xml
for servlets in a WAR file:
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
Why?
This configuration is crucial to correctly initialize your Spring application's dispatcher servlet in an external container context.
Bringing It All Together
While packaging a Spring Boot application as a WAR file presents several challenges, knowing how to overcome them ensures a smoother transition.
We discussed project structure adjustments, managing external configurations, handling classpath issues, and understanding the differences between embedded and standalone containers.
For developers considering the move to WAR packaging with Spring Boot, leveraging the capabilities of Spring Profiles, the robustness of dependency management, and adhering to conventional structure is essential.
For further reading on Spring Boot and its deployment options, check the Spring Boot Documentation.
By addressing the challenges thoughtfully, you can enjoy the best of both worlds: the simplicity of Spring Boot and the robust capabilities of traditional application servers.