Maximizing Test Performance with Testcontainers
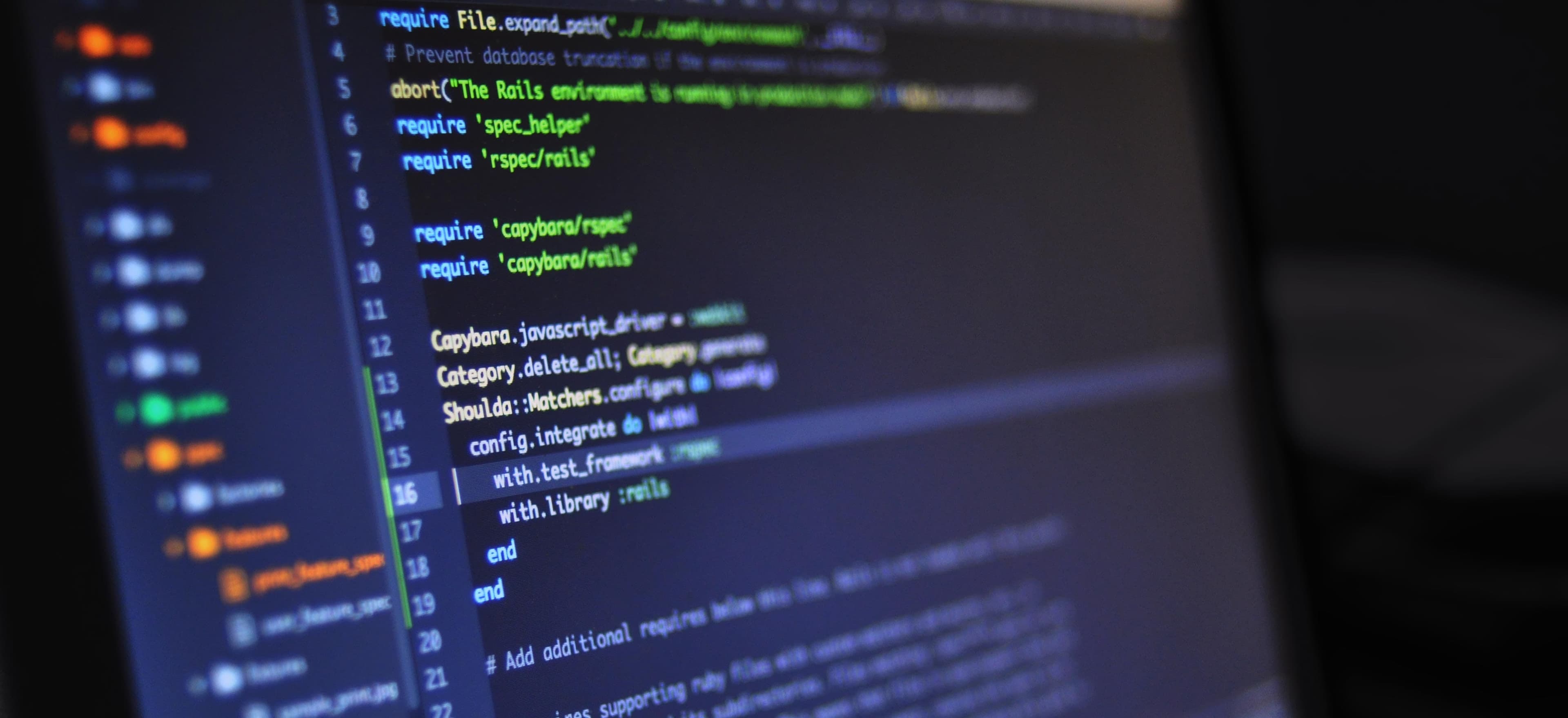
- Published on
Maximizing Test Performance with Testcontainers
When developing applications, especially those involving microservices, integration tests can get complicated. Enter Testcontainers—a powerful Java library that simplifies the testing of applications that rely on external resources. In this blog post, we'll explore how Testcontainers can help enhance your testing performance and improve overall software quality.
What Is Testcontainers?
Testcontainers is a Java library that supports JUnit tests. It allows developers to run their tests in lightweight, disposable Docker containers. By doing this, you can easily set up a consistent testing environment that mimics production conditions without worrying about the clutter that often accompanies testing with traditional methods.
The main advantages of using Testcontainers include:
- Isolation: Each test can run in a clean environment.
- Consistency: Containers ensure that tests run the same way across different machines.
- Flexibility: Testcontainers supports a wide variety of databases, message brokers, and many other technologies.
Getting Started with Testcontainers
Prerequisites
To get started, make sure you have the following:
- Java 8 or higher
- Maven or Gradle
- Docker installed and running on your machine
Adding Testcontainers to Your Project
First, add Testcontainers to your project dependencies. If you are using Maven, add the following to your pom.xml
:
<dependency>
<groupId>org.testcontainers</groupId>
<artifactId>testcontainers</artifactId>
<version>1.16.3</version> <!-- Check for the latest version -->
<scope>test</scope>
</dependency>
If you are using Gradle, add this to your build.gradle
:
testImplementation 'org.testcontainers:testcontainers:1.16.3' // Check for the latest version
Creating a Simple Test
Let's look at a simple integration test involving a PostgreSQL database:
import org.junit.jupiter.api.Test;
import org.testcontainers.containers.PostgreSQLContainer;
import static org.assertj.core.api.Assertions.assertThat;
public class DatabaseTest {
private static final PostgreSQLContainer<?> postgres =
new PostgreSQLContainer<>("postgres:latest")
.withDatabaseName("test_db")
.withUsername("user")
.withPassword("password");
static {
postgres.start();
}
@Test
public void testDatabaseConnection() {
String jdbcUrl = postgres.getJdbcUrl();
String username = postgres.getUsername();
String password = postgres.getPassword();
// Assuming you have a method to get the connection
// that is mocked or operates on a DataSource.
DataSource ds = getDataSource(jdbcUrl, username, password);
try (Connection connection = ds.getConnection()) {
assertThat(connection).isNotNull();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Explanation of the Code
- PostgreSQLContainer: This is a specific container setup for PostgreSQL. Testcontainers supports various other databases such as MySQL, MongoDB, and Cassandra, among others.
- Static Block: The PostgreSQL container is started automatically before any tests run. This is a straightforward way to ensure the container is ready for your tests.
- Connection Test: The test case establishes a connection to the database to ensure it's working as expected.
By using Testcontainers, we minimized setup time for tests, allowing us to focus on writing code rather than configuring databases manually.
Advanced Features of Testcontainers
Customizing Containers
In addition to default configurations, you can customize your containers to fit specific scenarios. For instance, you may need to run specific initialization scripts at container startup. Here’s how:
static {
postgres.withInitScript("init.sql"); // Place your init script in resources
postgres.start();
}
This bootstraps your database with necessary schemas, making it ready for testing right from the get-go.
Using Docker Compose
Sometimes, your application may rely on multiple containers (e.g., a web app with a database). In such cases, Testcontainers offers support for Docker Compose:
import org.testcontainers.containers.DockerComposeContainer;
public class MyAppIT {
private static DockerComposeContainer<?> composeContainer =
new DockerComposeContainer<>(new File("src/test/resources/docker-compose.yml"))
.withExposedService("myapp_1", 8080); // Exposing specific service
static {
composeContainer.start();
}
// Write your tests
}
Here, we have defined a Docker Compose setup in a YAML file, allowing for extensive configurations, including network setups and service dependencies.
For more details on using Docker Compose with Testcontainers, visit the official Testcontainers documentation.
Mapping Test Performance Improvements
Using Testcontainers not only helps in creating a robust testing environment but can also significantly enhance test performance through its various features:
-
Parallel Execution: Running multiple tests simultaneously can be achieved since containers are isolated. This improves the speed of your test suites.
-
Reusability: With the use of reusable containers, you can ensure that heavy resources don’t need to be spun up or torn down for every test run.
-
Skip Unnecessary Tests: Quickly skip tests involving certain external factors. For instance, if Docker is down, Testcontainers can skip those tests gracefully without breaking the entire test suite.
My Closing Thoughts on the Matter
Testcontainers is a powerful tool that can greatly improve the testing experience for Java developers. Its ability to create isolated, consistent environments improves test accuracy and helps avoid the "works on my machine" problem commonly faced in development.
By leveraging Testcontainers, developers can spend more time focusing on what really matters—writing and improving their code. If you're looking to enhance your test suite, consider integrating Testcontainers into your workflow today!
For further reading on Testcontainers and its integrations, you can check the following resources:
Start testing smarter today with Testcontainers—your tests will thank you for it!