Mastering Langium: Tackling Common DSL Creation Errors
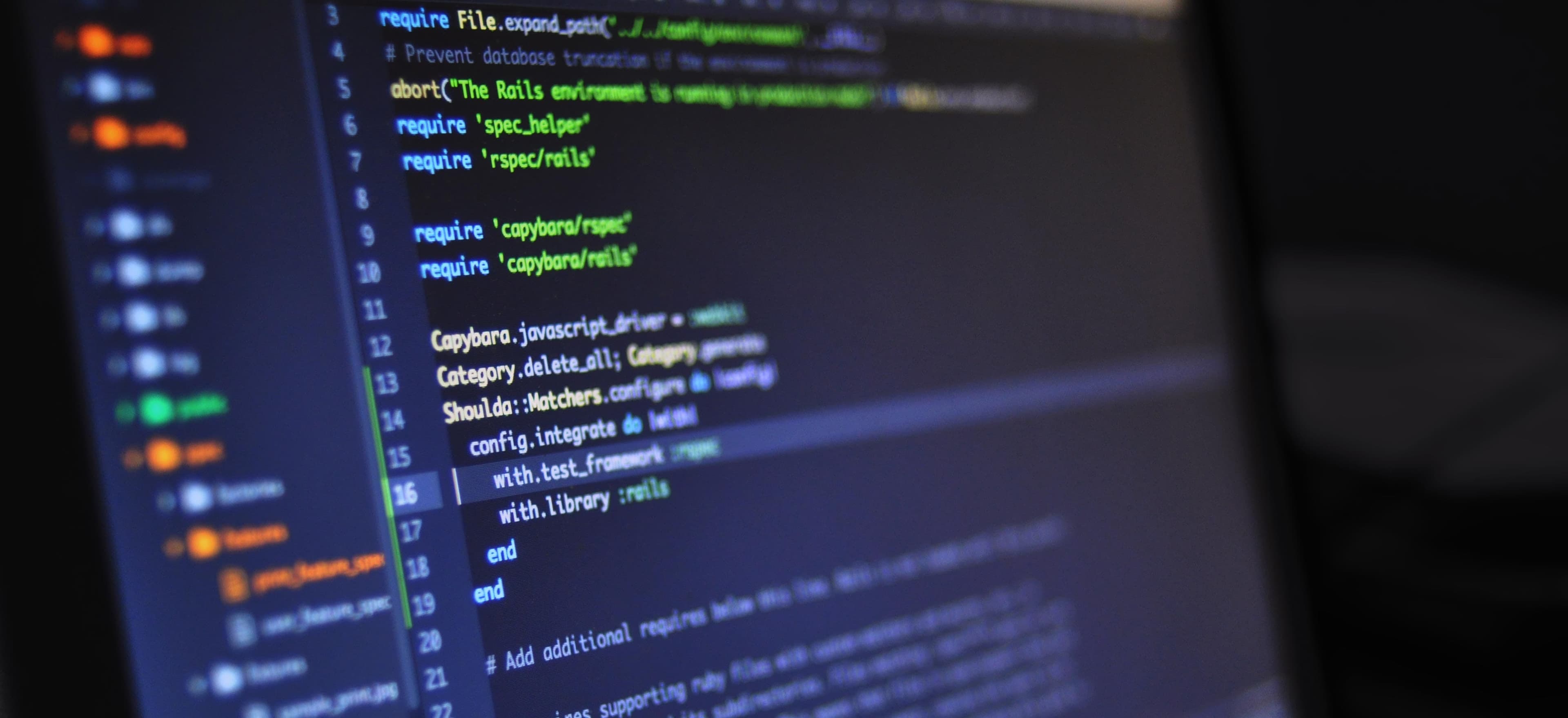
- Published on
Mastering Langium: Tackling Common DSL Creation Errors
In the world of software development, the ability to create domain-specific languages (DSLs) can significantly enhance productivity and code clarity. Langium is a powerful framework tailored for crafting such languages within the context of TypeScript and the Language Server Protocol (LSP). However, creating a DSL is not without its challenges. This blog post aims to guide you through common DSL creation errors in Langium, ensuring that you can harness its full potential effectively.
Table of Contents
- What is Langium?
- Understanding DSLs
- Common Errors in DSL Creation
- Syntax Errors
- Semantic Errors
- Generation Issues
- Best Practices for DSL Development
- Conclusion
What is Langium?
Langium is a flexible framework that allows developers to build programming languages and DSLs with ease. It offers users tools for syntax definition, parsing, type checking, and code generation. The framework integrates tightly with existing TypeScript infrastructure, thereby allowing developers to use modern tools and editors.
For an in-depth introduction to Langium, check out the Langium Documentation.
Understanding DSLs
A domain-specific language is a specialized language tailored for a particular problem domain. Unlike general-purpose programming languages (like Java or C++), DSLs enable more expressive syntax and semantics within a narrower domain.
Example of a DSL
Consider a DSL for describing a simple workflow process:
Process {
task TaskName {
actions {
action ActionName;
}
}
}
In this snippet, we've defined a DSL for a process consisting of tasks and actions. This clarity allows domain experts to contribute directly to the workflow definition without delving into general programming terminology.
Common Errors in DSL Creation
Even seasoned developers can encounter errors when creating DSLs with Langium. Below, we discuss some of the most common pitfalls and provide solutions.
1. Syntax Errors
Syntax errors occur when the structure of your DSL code deviates from the defined grammar. These can often lead to frustrating runtime errors.
Example of a Syntax Error
// Error: Missing semicolon in action declaration
Process {
task TaskName {
actions {
action ActionName
}
}
}
Solution: Always ensure that you follow the grammar rules strictly. In popular DSLs, most statements require a semicolon to indicate the end, as this is the case in many C-like languages.
2. Semantic Errors
While syntax errors deal with the structure of your code, semantic errors occur when the code is syntactically correct but incorrect in terms of meaning. This can include undeclared variables, mismatched types, or logic errors.
Example of a Semantic Error
Process {
task TaskName {
actions {
action UndefinedAction; // Error: Undefined action
}
}
}
Solution: To prevent semantic errors, implement comprehensive validation mechanisms. Langium's type system can help catch such issues at compile time. Make sure to include thorough checks to verify that every action and variable being referenced is defined and valid.
3. Generation Issues
Another common area where developers face challenges is in code generation. Problems may arise when converting the DSL into runnable code due to incorrect template configurations or generator logic.
Example of a Generation Issue
export function generateCode(process: Process): string {
return `Defining a process: ${process.name}`;
// Potential issue: If 'process.name' is undefined, runtime error could occur.
}
Solution: Always validate your input shapes before attempting to generate code. Utilize optional chaining or default values to handle undefined cases gracefully.
export function generateCode(process: Process): string {
return `Defining a process: ${process.name || 'Unnamed Process'}`;
}
Best Practices for DSL Development
While the above errors are common, adhering to best practices can significantly smooth your DSL development journey.
1. Start Simple
One of the best strategies for developing a DSL is to begin with a simple, minimal grammar. Focus on a small subset of features initially and expand from there. This often helps in identifying errors early.
2. Write Comprehensive Tests
Develop a suite of tests to cover various use cases. This will help catch issues at an early stage and ensure that your DSL’s functionalities behave as expected. Use test frameworks compatible with TypeScript, like Jest or Mocha, for this purpose.
3. Utilize Visual Studio Code Extensions
Langium comes with built-in support for the Language Server Protocol, which means you can leverage Visual Studio Code or other LSP-compatible editors for enhanced development experiences. These tools provide syntax highlighting, auto-completion, and error checking, making language creation smoother.
4. Collaborate with Domain Experts
Discuss your DSL's design and structure with domain experts throughout the development process. Their insights can help refine the language’s syntax and semantics, thus avoiding the creation of a language that is difficult to use.
5. Refer to Documentation and Community Resources
Regularly consult the Langium Documentation and community resources for tips, examples, and troubleshooting techniques. Engaging with the community can provide answers to many common queries.
Final Thoughts
Creating a DSL with Langium can be a rewarding yet challenging endeavor. By understanding the common errors that arise during the process, applying best practices, and using available resources, you can minimize frustrations and maximize productivity.
Whether you're embarking on your first DSL creation or looking to refine an existing one, remember that the goal is to enable clearer communication, more efficient implementation, and ultimately, a language that serves your specific domain effectively. Happy coding!