Common Pitfalls in Building a Spring Boot API
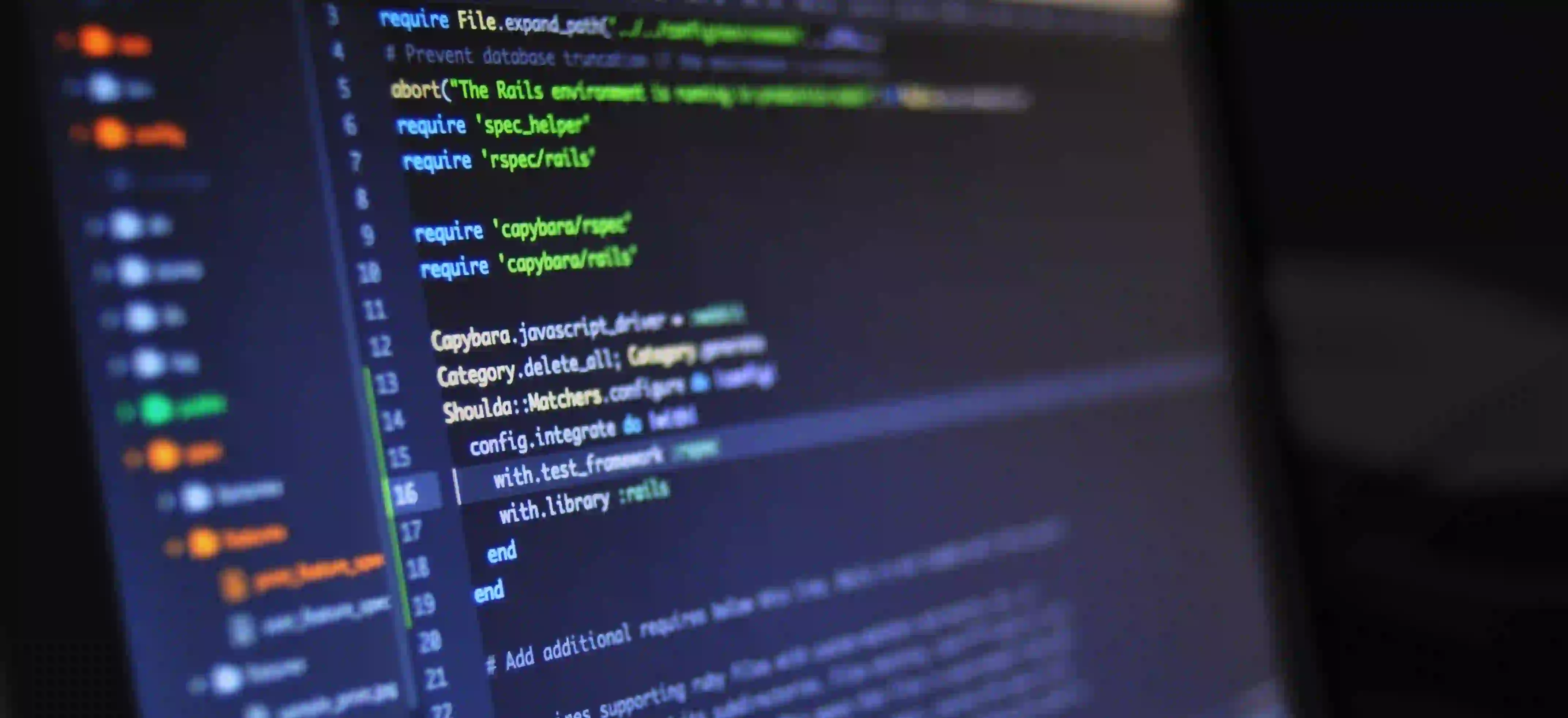
Common Pitfalls in Building a Spring Boot API
Building APIs with Spring Boot has become a popular choice for many developers. Its seamless integration with the Spring ecosystem, combined with its reliance on Java, makes it an attractive option for those looking to create robust applications. However, building an API is not without its challenges. In this post, we will explore some common pitfalls in building a Spring Boot API, how to avoid them, and best practices to ensure your API remains stable and maintainable.
Table of Contents
- 1. Poor API Design
- 2. Ignoring Error Handling
- 3. Not Securing the API
- 4. Neglecting Testing
- 5. Failing to Document the API
- 6. Incorrect Database Management
- 7. Overlooking Performance Tuning
- 8. Conclusion
1. Poor API Design
A poorly designed API can lead to confusion for end-users and developers alike. When designing your API, make sure to adhere to RESTful principles. Use descriptive URIs, appropriate HTTP methods, and consistent response structures.
Example: Instead of having an endpoint like:
@GetMapping("/getUser/{id}")
public User getUser(@PathVariable("id") Long id) {
return userService.getUserById(id);
}
Refactor it to a more RESTful approach:
@GetMapping("/users/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
Why this matters:
Following REST conventions makes your API more predictable. This creates a better experience for consumers and promotes a standardized approach to interaction.
2. Ignoring Error Handling
Uncaught exceptions in your API can cause your application to return generic error messages or, worse, no response at all. Proper error handling not only enhances user experience but also makes debugging easier.
Implement a global exception handler using @ControllerAdvice
:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFound(UserNotFoundException e) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(e.getMessage());
}
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGenericException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An unexpected error occurred.");
}
}
Why this matters:
This approach provides meaningful feedback to the API consumer, allowing them to understand what went wrong.
3. Not Securing the API
APIs can be vulnerable to unauthorized access if security is not adequately incorporated. Spring Security is a powerful tool for this purpose. Implementing security measures like OAuth2 or JWT (JSON Web Tokens) is crucial.
Example of basic JWT authentication:
First, add Spring Security dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
Then create a JWT utility class:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
@Component
public class JwtUtil {
private String secretKey = "yourSecretKey"; // Replace with a strong secret
public String generateToken(String username) {
return Jwts.builder()
.setSubject(username)
.signWith(SignatureAlgorithm.HS256, secretKey)
.compact();
}
public boolean validateToken(String token, String username) {
final String extractedUsername = extractUsername(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
}
// Additional methods for token extraction...
}
Why this matters:
In a world where data breaches and cyberattacks are commonplace, securing your API not only protects your application but also fosters trust with your users.
4. Neglecting Testing
Testing is often an overlooked step when developing APIs. Skipping testing phases can lead to instability and bugs. Utilize Spring Boot's testing features to create unit and integration tests.
Example: Using Spring's @WebMvcTest
for a controller:
@RunWith(SpringRunner.class)
@WebMvcTest(UserController.class)
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@MockBean
private UserService userService;
@Test
public void testGetUser() throws Exception {
User user = new User(1L, "John Doe");
when(userService.getUserById(1L)).thenReturn(user);
mockMvc.perform(get("/users/1"))
.andExpect(status().isOk())
.andExpect(jsonPath("$.name").value("John Doe"));
}
}
Why this matters:
Testing ensures that your code meets specifications, functions correctly, and can handle various scenarios. A reliable API is essential for maintaining user satisfaction.
5. Failing to Document the API
Documentation is critical for any API user. Without proper documentation, developers may struggle to understand how to use the API effectively. Tools like Swagger or Spring REST Docs can substantially facilitate this process.
Example of integrating Swagger:
Add the Swagger dependencies to your pom.xml
:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
Then, create a configuration class:
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.documentation.SpringFoxDocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(SpringFoxDocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.any())
.paths(PathSelectors.any())
.build();
}
}
Why this matters:
Good documentation allows other developers to integrate with your API efficiently. It reduces friction and promotes better usage, leading to more robust applications.
6. Incorrect Database Management
Database management can make or break your API. Make use of Spring Data JPA for database interactions, and make sure to follow best practices in data access.
Example: Utilizing Spring Data JPA repository:
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Why this matters:
Improper database management can lead to performance issues, data inconsistencies, and difficulty in scaling the application.
7. Overlooking Performance Tuning
As your API scales, performance might become a bottleneck. Always monitor and optimize your application's performance. Use Spring’s cache mechanism and consider asynchronous processing where applicable.
Example: Enabling caching:
@EnableCaching
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Why this matters:
Optimizing your API makes it capable of handling higher loads without degradation in performance, leading to better user experiences.
8. Conclusion
Building a Spring Boot API comes with its set of challenges. By recognizing common pitfalls such as poor design, neglecting security, ignoring testing, and failing to document, you can build a more robust, user-friendly API.
Remember, building an API is an iterative process. By consistently evaluating and improving your application, you can provide a valuable service to your users while honing your development skills.
If you're keen to dive deeper into Spring Boot development, consider checking out the official Spring documentation, or explore our articles on REST API best practices for more insights.
Happy coding!