Common Pitfalls When Using XMLBeans for XML Handling
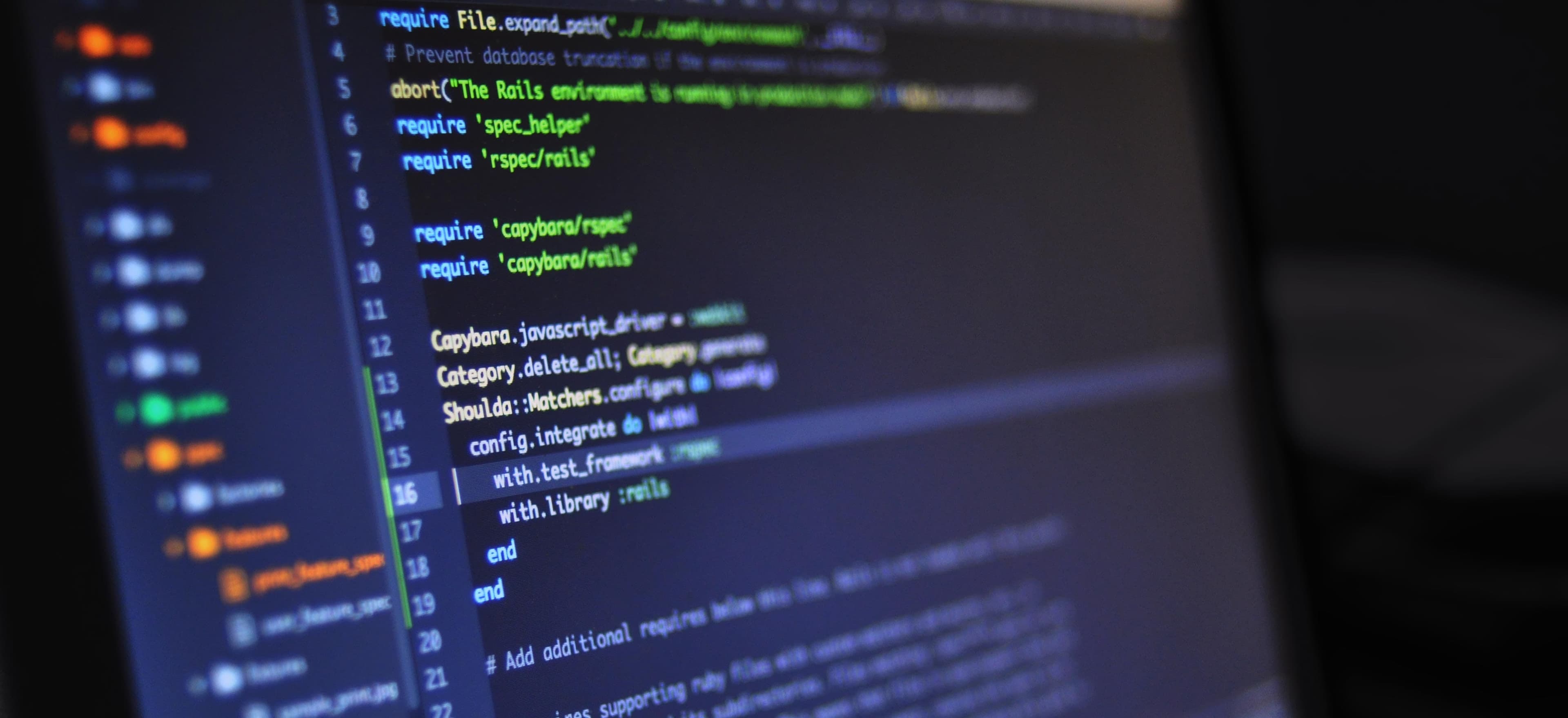
- Published on
Common Pitfalls When Using XMLBeans for XML Handling
XML handling is a crucial aspect of software development, especially when dealing with data exchange formats. In Java, one of the powerful tools for dealing with XML is XMLBeans. Despite its robustness and flexibility, developers often stumble upon some common pitfalls. This blog post delves into these pitfalls, aiming to equip you with the insights needed to avoid them effectively.
What is XMLBeans?
Before we dive into the pitfalls, it’s essential to understand what XMLBeans does. XMLBeans is a framework that allows Java developers to access and manipulate XML documents. With XMLBeans, XML files can be bound to Java objects, which simplifies XML processing significantly.
For further reading on XMLBeans, check out the official XMLBeans documentation.
Pitfall 1: Improper Schema Definition
One of the first challenges developers face is improperly defining the XML schema (XSD). A poorly designed XSD can lead to issues in object generation or unexpected behavior at runtime.
Solution:
Ensure that your XSD is well-defined. Validate the schema using various online XML validation tools or integrated development environments (IDEs) before using it with XMLBeans.
Example: Schema Definition
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="person">
<xs:complexType>
<xs:sequence>
<xs:element name="name" type="xs:string"/>
<xs:element name="age" type="xs:int"/>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:schema>
In this definition, the person
element has a name
and age
. Make sure to use appropriate data types and element definitions to prevent inconsistencies.
Pitfall 2: Ignoring Namespace Issues
XML is hierarchical and supports namespaces, which can be a source of confusion. Developers frequently overlook XML namespaces, leading to Unknown element
or Unexpected attribute
errors.
Solution:
Be sure to declare and use namespaces correctly in your XML and Java code.
Example: Namespace Declaration
<person xmlns="http://example.com/person">
<name>John Doe</name>
<age>30</age>
</person>
When reading this XML with XMLBeans, make sure to declare the namespace in your Java code accordingly.
XmlOptions options = new XmlOptions();
options.setLoadStripWhitespace();
Person person = PersonDocument.Factory.parse(xmlStream, options).getPerson();
By addressing the namespace clearly, you avoid confusion and potential errors.
Pitfall 3: Memory Management and Performance
XML processing can consume significant memory resources. This often happens when developers load large XML documents entirely into memory, which can lead to performance bottlenecks and crashes.
Solution:
Use streaming API available in XMLBeans for large XML files that allows processing the document in smaller chunks.
Example: Using Streaming API
import org.apache.xmlbeans.XmlOptions;
import org.apache.xmlbeans.XmlCursor;
try (FileInputStream fis = new FileInputStream("large-file.xml")) {
XmlOptions options = new XmlOptions();
options.setLoadStripWhitespace();
XmlCursor cursor = XmlCursor.Factory.parse(fis, options);
while (cursor.toNextSelection()) {
// Process each node
}
}
By processing the XML in a streaming manner, you can handle large XML files without hogging memory resources.
Pitfall 4: Lack of Error Handling
XML processing often encounters errors due to malformed XML, schema violations, or I/O issues. Developers may overlook implementing robust error handling, leading to silent failures.
Solution:
Always include comprehensive error handling around XMLBeans operations.
Example: Error Handling
try {
Person person = PersonDocument.Factory.parse("person.xml").getPerson();
} catch (XmlException e) {
System.err.println("Error parsing XML: " + e.getMessage());
} catch (IOException e) {
System.err.println("IO Error: " + e.getMessage());
}
By catching potential exceptions, you can understand the issues during runtime instead of facing unpredictable failures.
Pitfall 5: Not Adequately Testing XML Processing
Testing is often neglected, which can lead to issues in production. Some developers assume that if the code compiles, it is ready for deployment, which is not the case.
Solution:
Implement unit tests that validate XML handling logic.
Example: Using JUnit for Testing
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class PersonTest {
@Test
public void testPersonParsing() throws Exception {
Person person = PersonDocument.Factory.parse("test-person.xml").getPerson();
assertEquals("John Doe", person.getName());
assertEquals(30, person.getAge());
}
}
By conducting thorough tests, you ensure that your XML handling works as expected in various scenarios.
Pitfall 6: Forcing XML Structure Changes
XMLBeans provides XML binding that simplifies access to XML data. However, developers may try to change the XML structure dynamically, which can lead to complications.
Solution:
Instead of dynamically altering the XML, prefer creating a new version of the XML or refactor the schema to accommodate necessary changes naturally.
Example: Creating New XML Nodes
Person person = PersonDocument.Factory.newInstance().addNewPerson();
person.setName("Jane Doe");
person.setAge(25);
By following this approach, you maintain the integrity of your XML structure and avoid introducing latent bugs.
In Conclusion, Here is What Matters
Using XMLBeans to handle XML comes with its own set of challenges. However, by recognizing these common pitfalls and employing the solutions provided, you can significantly improve your XML handling capabilities. Always remember, thorough validation, strategic testing, and careful schema design are keys to successful XML management.
For further reading on advanced XML handling techniques, consider exploring this XML handling tutorial which discusses various libraries and approaches.
By adhering to best practices and learning from common mistakes, you’ll enhance your Java development skills and leverage XMLBeans more effectively. Happy coding!
Checkout our other articles