Overcoming Java's Verbosity: Kotlin Builder Patterns in Python
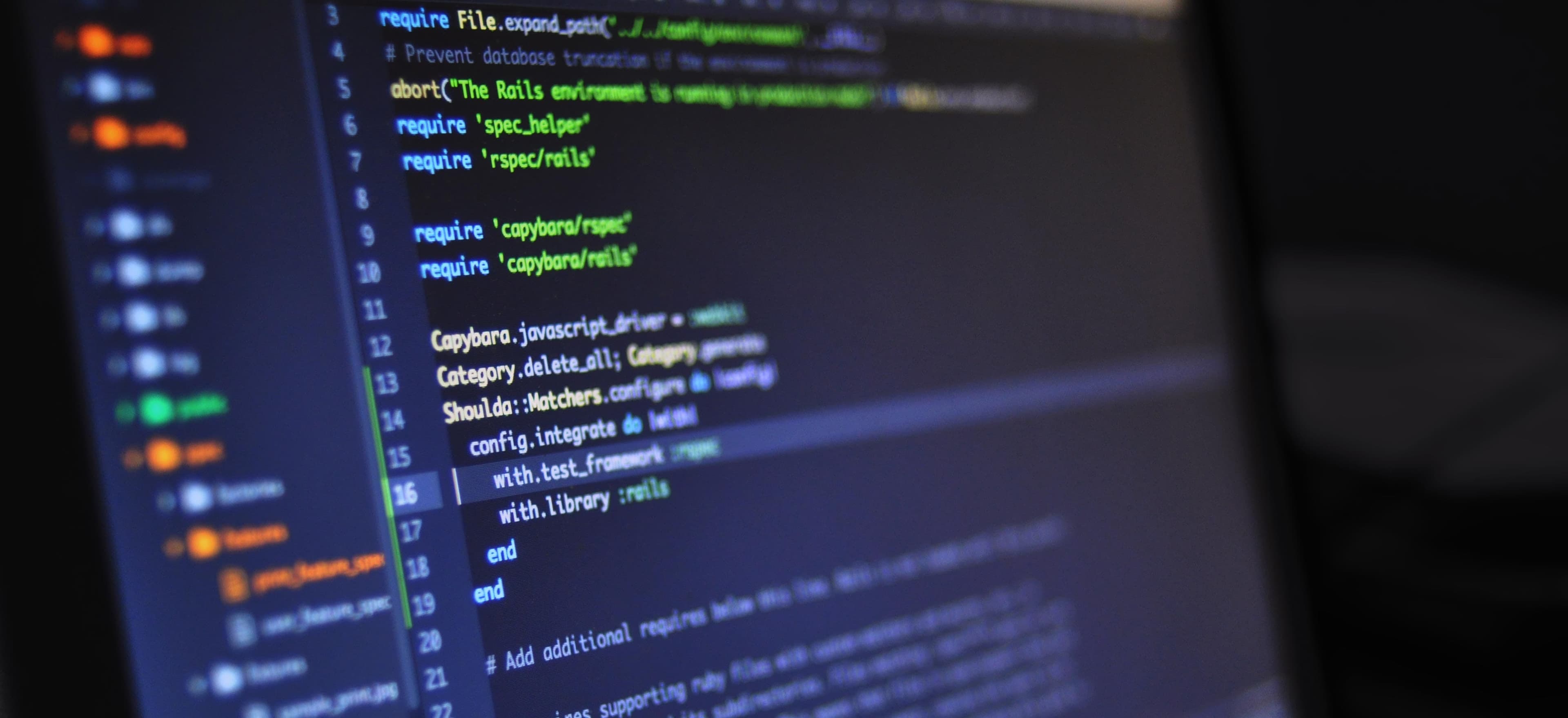
- Published on
Overcoming Java's Verbosity: Kotlin Builder Patterns in Python
Java is a powerful, widely used programming language known for its portability and performance. However, one criticism that often arises is its verbosity. Developers frequently find themselves writing extensive code just to achieve simple functionality. Meanwhile, Kotlin, a modern programming language, offers elegant solutions to this verbosity through features like the Builder Pattern.
In this blog post, we will explore the Builder Pattern as implemented in Kotlin and how we can replicate this concise and readable style using Python. Along the way, we will dive deeply into the design, showcase code snippets, and highlight the benefits of each approach.
Understanding the Builder Pattern
The Builder Pattern is a design pattern that allows for the step-by-step construction of complex objects. It is particularly useful when an object requires many parameters, some of which may be optional. Instead of a convoluted constructor, the Builder Pattern allows you to create a more readable and maintainable approach to object creation.
A Simple Example in Kotlin
Let's first consider a straightforward example of the Builder Pattern in Kotlin.
data class User(val name: String, val age: Int, val email: String?) {
class Builder {
var name: String = ""
var age: Int = 0
var email: String? = null
fun setName(name: String) = apply { this.name = name }
fun setAge(age: Int) = apply { this.age = age }
fun setEmail(email: String?) = apply { this.email = email }
fun build() = User(name, age, email)
}
}
fun main() {
val user = User.Builder()
.setName("Alice")
.setAge(30)
.setEmail("alice@example.com")
.build()
println(user)
}
Commentary
-
Data Classes: In Kotlin, the
data class
feature simplifies the creation of classes that primarily hold data, encapsulating standard functionalities liketoString
,equals
, andhashCode
. -
Apply Function: The
apply
function allows us to set properties succinctly while returning the Builder instance itself, enabling a fluid API. -
The build() Method: Finalizes the object creation.
Advantages of Kotlin's Builder Pattern
- Clarity and Readability: The chaining of methods makes it easy to understand the object being constructed.
- Immutability: Data classes promote immutability unless specified otherwise.
- Optional Parameters: Easily manage optional parameters.
Replicating the Builder Pattern in Python
Python, while not as verbose as Java, still benefits from adopting the Builder Pattern to enhance clarity. Here’s how we can implement a similar pattern in Python.
Python Implementation
class User:
def __init__(self, name: str, age: int, email: str = None):
self.name = name
self.age = age
self.email = email
def __repr__(self):
return f"User(name='{self.name}', age={self.age}, email='{self.email}')"
class UserBuilder:
def __init__(self):
self.name = ""
self.age = 0
self.email = None
def set_name(self, name: str):
self.name = name
return self
def set_age(self, age: int):
self.age = age
return self
def set_email(self, email: str):
self.email = email
return self
def build(self):
return User(self.name, self.age, self.email)
# Example usage
if __name__ == "__main__":
user = UserBuilder() \
.set_name("Alice") \
.set_age(30) \
.set_email("alice@example.com") \
.build()
print(user)
Commentary
- Function Chaining: Methods return
self
, allowing for fluid chaining. - Default Arguments: Python's function definition supports default arguments, making them optional.
- String Representation: The
__repr__
method provides a human-readable format for the object.
Benefits of Python's Builder Pattern
- Lightweight: Less boilerplate code compared to Java.
- Dynamic Typing: Offers more flexibility and speed in development.
- Rich Standard Library: Python provides numerous built-in modules to complement the Builder Pattern.
Performance Considerations
It's worth mentioning that choosing between Kotlin and Python puts a spotlight on performance. Kotlin is statically typed, which can lead to better performance in larger applications. In contrast, Python’s dynamic nature can introduce overhead but allows for rapid development.
However, in contexts where readibility and maintainability are key, both Kotlin and Python shine through their implementation of the Builder Pattern.
Use Cases for Builder Pattern
The Builder Pattern can be applied in various scenarios, such as:
- Creating Complex JSON Objects: Where nested structures require clear construction.
- Configuring Objects with Many Optional Parameters: When there are many optional configurations, the Builder Pattern eliminates cumbersome constructors.
Bringing It All Together
The Builder Pattern provides a thoughtful and elegant solution to manage the complexity of object construction. Both Kotlin and Python facilitate this pattern but each has its unique advantages.
Kotlin's static typing and language features offer a structured approach, lending itself well to robust applications. Meanwhile, Python’s brevity and expressiveness provide an adaptable and efficient development experience.
By adopting the Builder Pattern, developers can overcome the verbosity often found in Java, resulting in clearer, more maintainable code in both Kotlin and Python. As you choose your tools, consider not only performance and efficiency but also clarity and ease of use.
For more information on design patterns, you might find the Design Patterns in Python resource helpful. Additionally, the official Kotlin documentation is an excellent source for those wanting to delve deeper into Kotlin's features.
Would you implement the Builder Pattern in other programming languages? Share your experiences in the comments below!
Checkout our other articles