Overcoming Common Pitfalls in JCuda for GPGPU Development
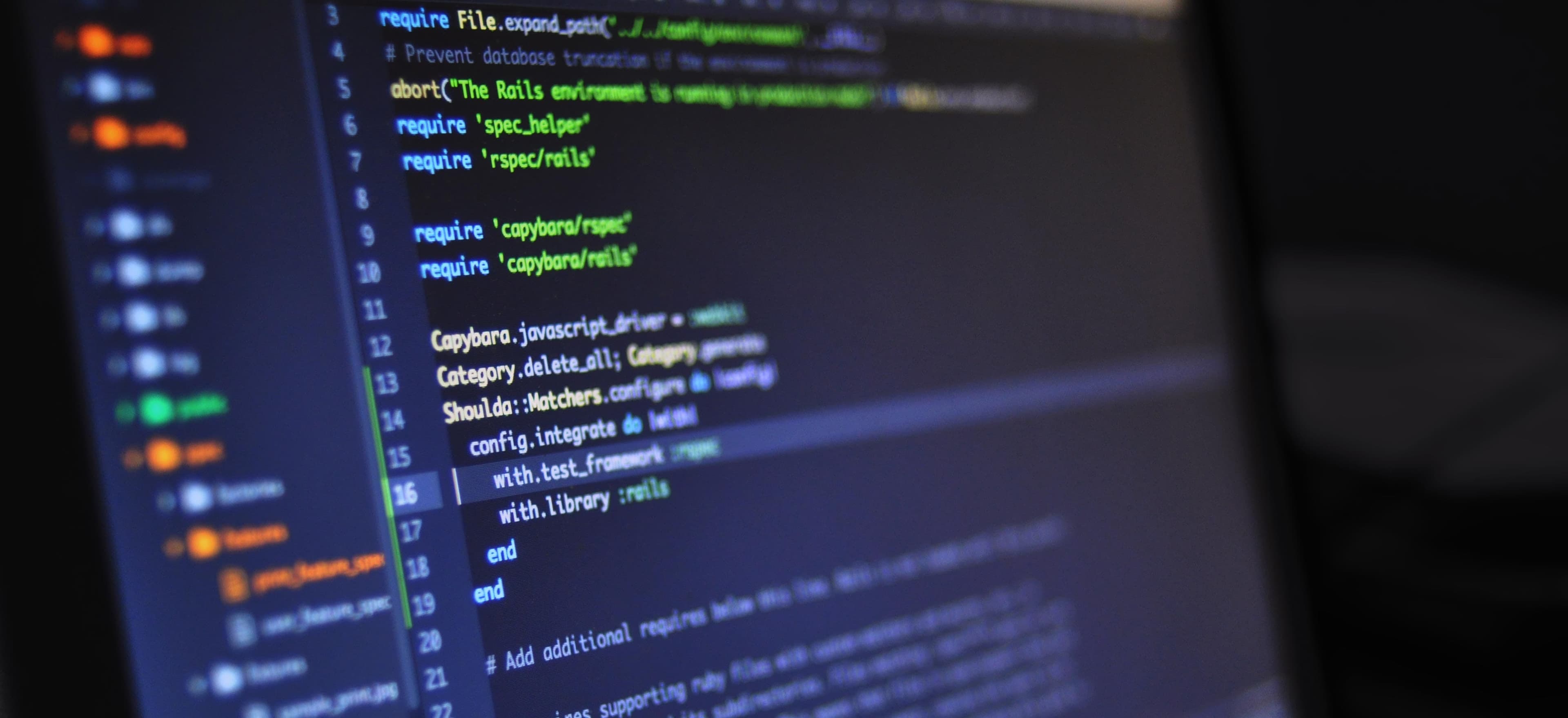
- Published on
Overcoming Common Pitfalls in JCuda for GPGPU Development
General-Purpose computing on Graphics Processing Units (GPGPU) has revolutionized the way we perceive performance in computing tasks that require immense parallel processing capabilities. JCuda is one of the prominent frameworks that allows Java developers to utilize the power of GPU computing, providing a bridge between Java applications and CUDA APIs. While the potential is enormous, developers often face various challenges while working with JCuda. In this article, we will explore some common pitfalls in JCuda development and discuss effective strategies to overcome them.
Understanding JCuda
JCuda enables developers to write programs in Java that utilize CUDA, NVIDIA's parallel computing architecture. It offers native class bindings to CUDA functions and also supports running CUDA kernels and handling memory. By incorporating JCuda, you can accelerate computationally heavy tasks, such as machine learning, graphics, and simulations, which can drastically increase the efficiency of Java applications.
Common Pitfalls in JCuda Development
1. Mismanaging GPU Resources
One of the most common pitfalls developers encounter is the improper management of GPU resources. GPU memory is limited compared to system memory, and failing to free memory can quickly lead to out-of-memory errors.
Solution: Always Release Resources
Make it a standard practice to release GPU resources after their use. Here’s an example:
import jcuda.runtime.JCuda;
import jcuda.runtime.JCuda.*;
import jcuda.Pointer;
// After finishing operations
Pointer devicePtr;
JCuda.cudaFree(devicePtr);
In this code snippet, cudaFree
is used to deallocate memory on the GPU, ensuring that resources are managed effectively. Regularly freeing memory minimizes the risk of running into resource limitations.
2. Not Handling Exceptions Properly
Many newcomers to JCuda might overlook the importance of exception handling. CUDA operations can fail for various reasons (e.g., memory issues, kernel execution failures), but without proper exception handling, these failures can lead to hard-to-diagnose bugs.
Solution: Implement Exception Handling
Wrap your JCuda calls in try-catch blocks and check for errors using cudaGetLastError()
after each kernel launch. Here’s a conceptual approach:
try {
// Kernel launch
myKernel<<<blocks, threads>>>(...);
// Check for errors
int error = JCuda.cudaGetLastError();
if (error != JCuda.cudaSuccess) {
System.err.println("Error Code: " + errorString(error));
}
} catch (Exception e) {
e.printStackTrace();
}
By correctly handling exceptions, you can identify and resolve issues quicker, thus avoiding significant time losses in debugging.
3. Inaccurate Kernel Memory Management
Improperly managing memory while transferring data between the host (CPU) and device (GPU) can lead to performance degradation. Developers often forget to optimize these transfers, which can become costly in terms of computation time.
Solution: Minimize CPU-GPU Data Transfers
Keep the data transfers between CPU and GPU to a minimum. Here’s how you can manage memory effectively:
// Allocate memory on the device
Pointer deviceData;
JCuda.cudaMalloc(deviceData, size);
// Transfer data from host to device
JCuda.cudaMemcpy(deviceData, hostData, size, cudaMemcpyHostToDevice);
Use pinned (page-locked) memory for improved data transfer rates:
// Allocate pinned memory
cudaMallocHost(hostData, size);
By reducing the frequency of memory transfers and utilizing pinned memory, you can significantly improve the performance of your application.
4. Ignoring Performance Optimization
When developing GPU programs, overlooking kernel optimization can lead to suboptimal performance. Many developers rely on naive parallelization without considering memory access patterns or shared memory usage.
Solution: Optimize Kernel Execution Plans
Carefully evaluate your kernel design. Use shared memory efficiently and minimize divergent branching among threads. Here's an example of utilizing shared memory:
__global__ void optimizedKernel(int *data) {
__shared__ int sharedData[BLOCK_SIZE];
int tid = threadIdx.x;
sharedData[tid] = data[tid];
__syncthreads();
// Perform calculations...
}
In the above CUDA kernel, shared memory is employed, which is faster than global memory. Such optimizations can lead to significant performance gains.
5. Debugging Challenges
Debugging GPU code can be exceptionally challenging, as traditional debugging tools often fall short. Developers can find it difficult to trace issues, primarily when they involve memory corruption or memory access violations.
Solution: Utilize JCuda's Debugging Tools
Familiarize yourself with debugging tools like NVIDIA Visual Profiler to analyze performance and memory access patterns. Furthermore, ensure you have adequate logging in your application:
logger.info("Kernel execution started");
myKernel<<<blocks, threads>>>(...);
logger.info("Kernel execution finished");
By integrating logging, you can capture significant information to help troubleshoot cases where unexpected behaviors arise.
6. Having Insufficient Error Reporting
It is common for developers to overlook the significance of reporting errors effectively while developing with JCuda. Insufficient error reporting can lead to confusion and result in prolonged debugging sessions.
Solution: Enhance Error Reporting
Make error reporting more informative. For instance, combine error messages with the context in which they occur:
public void checkCudaError() {
int error = JCuda.cudaGetLastError();
if (error != JCuda.cudaSuccess) {
throw new RuntimeException("CUDA Error: " + JCuda.cudaGetErrorString(error));
}
}
An effective error reporting system informs you not only about what went wrong but also where the error occurred, making troubleshooting much more efficient.
Closing Remarks
JCuda opens a world of possibilities for Java developers willing to harness the power of parallel computing. However, it is imperative to be mindful of common pitfalls in GPGPU development. By managing resources efficiently, implementing proper error handling, optimizing data transfers, refining kernel designs, utilizing effective debugging tools, and enhancing error reporting, you can elevate your JCuda development experience and application performance.
If you wish to dive deeper into JCuda, consider visiting the official JCuda Documentation. Remember, the road to mastering GPU development is challenging but rewarding. Happy coding!
Checkout our other articles