Mastering Nested ComputeIfAbsent in Java: Common Pitfalls
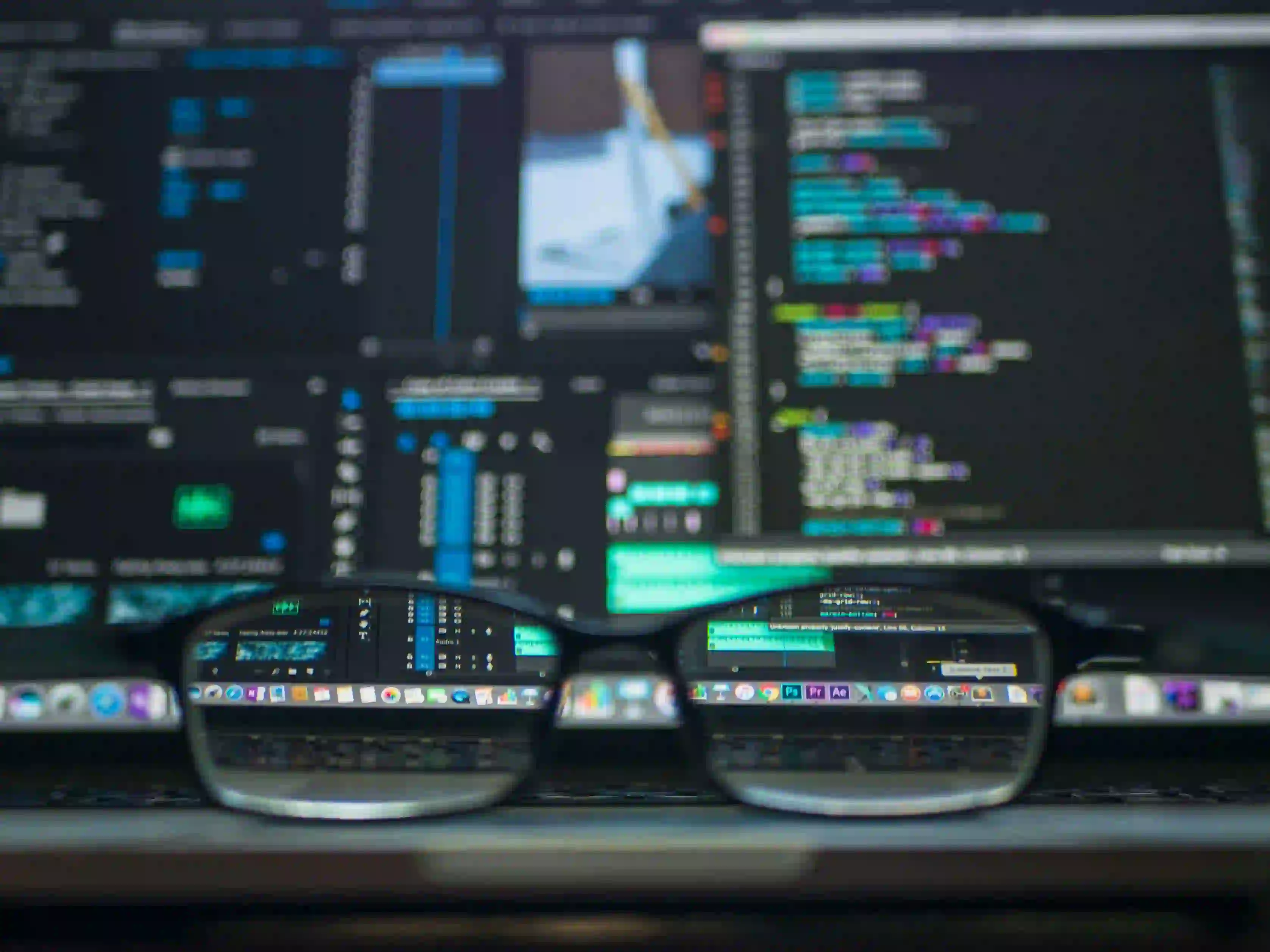
Mastering Nested computeIfAbsent
in Java: Common Pitfalls
In the realm of Java development, Map
is a powerful data structure that offers a multitude of ways to manage key-value pairs. One of the lesser-known yet highly versatile methods introduced in Java 8 is computeIfAbsent
. This method allows you to compute a value for a given key if it is not already mapped. While it can simplify your code and minimize boilerplate, using computeIfAbsent
improperly—especially in nested situations—can lead to unexpected behaviors and bugs.
In this blog post, we will look closely at nested computeIfAbsent
usage in Java, common pitfalls developers encounter, and best practices to avoid them.
Understanding computeIfAbsent
Before diving into nested usage, let's clarify what computeIfAbsent
does:
V computeIfAbsent(K key, Function<? super K, ? extends V> mappingFunction);
- Key: The key whose absence in the map should trigger the computation.
- Mapping Function: A function that produces a value if the key is not already mapped.
If the key is present, its corresponding value will be returned. If not, the mapping function will be executed, the computed value will be stored in the map, and that value will be returned.
Basic Example
Here’s a simple example to illustrate its usage:
import java.util.HashMap;
import java.util.Map;
public class ComputeIfAbsentDemo {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
// Compute and populate the value if absent
Integer value = map.computeIfAbsent("key1", k -> 10 + k.length());
System.out.println("Value: " + value); // Outputs: Value: 14
}
}
In this case, since "key1" doesn't exist in the map, the lambda function computes the value based on the length of the key.
When to Use Nested computeIfAbsent
The real power of computeIfAbsent
emerges when it is used in nested structures, such as when having a Map
of Map
. Let's consider a scenario where you want to count occurrences of words in sentences grouped by sentences themselves.
Nested Example
Here’s how you might approach it:
import java.util.HashMap;
import java.util.Map;
public class NestedComputeIfAbsent {
public static void main(String[] args) {
Map<String, Map<String, Integer>> wordCountMap = new HashMap<>();
String sentence = "hello world hello java";
for (String word : sentence.split(" ")) {
Map<String, Integer> countMap = wordCountMap
.computeIfAbsent(sentence, s -> new HashMap<>());
countMap.computeIfAbsent(word, w -> 0);
countMap.put(word, countMap.get(word) + 1);
}
System.out.println(wordCountMap);
}
}
In this example, we create a map that associates each sentence with another map that counts the occurrences of each word in that sentence.
Common Pitfalls
While nested computeIfAbsent
can simplify your code, there are several pitfalls to watch out for:
1. Unchecked Value Generation
One common mistake is failing to ensure that the underlying data structures work as intended.
map.computeIfAbsent(key1, k -> {
// Heavy computation
return computeHeavyValue();
}).computeIfAbsent(key2, k -> new HashMap<>()).put("subKey", value);
In the above code, if key1
is absent, a heavy computation is performed even when key2
might not even be necessary. To mitigate this, narrow your computations to only those that are truly required.
2. Concurrent Modification
If you are working in a multi-threaded context, using computeIfAbsent
on nested maps can lead to ConcurrentModificationExceptions
.
Using a synchronized block or opting for a ConcurrentHashMap
can help alleviate these issues:
import java.util.concurrent.ConcurrentHashMap;
Map<String, ConcurrentHashMap<String, Integer>> concurrentWordCountMap =
new ConcurrentHashMap<>();
String sentence = "hello world hello java";
// Thread-safe handling of nested computeIfAbsent
for (String word : sentence.split(" ")) {
concurrentWordCountMap
.computeIfAbsent(sentence, s -> new ConcurrentHashMap<>())
.compute(word, (k, v) -> (v == null) ? 1 : v + 1);
}
Here, we utilize ConcurrentHashMap
to achieve thread-safety, ensuring that concurrent modifications do not result in errors.
3. Complex Logic within the Mapping Function
Placing complex logic inside the mapping function can obscure the intent of your code. Keep your mapping functions simple.
For example:
map.computeIfAbsent(key, k -> complexFunctionThatDoesManyThings());
Refactor any complex logic into separate functions:
private static Map<String, Integer> myMappingFunction(String key) {
Map<String, Integer> result = new HashMap<>();
// Logic to populate the result
return result;
}
map.computeIfAbsent(key, k -> myMappingFunction(k));
Best Practices for Using computeIfAbsent
-
Keep It Simple: Avoid complex logic inside the mapping function. Determine the logic beforehand and keep it separate.
-
Concurrency Awareness: When working in concurrent environments, use
ConcurrentHashMap
or synchronize access to methods that modify the map. -
Check for Null: Ensure your mapping function does not return null. Returning null can unintentionally remove the existing mapping. If unavoidable, handle null properly.
-
Minimize Side Effects: The mapping function should not cause side effects, as it can lead to hard-to-track bugs. Ensure the mapping is primarily functional.
-
Readability Matters: Use descriptive method names for your mapping functions. Clear code is easier to maintain and understand.
The Last Word
While computeIfAbsent
is undoubtedly powerful, using it in nested contexts can lead to complexity and potential bugs if not handled carefully. By understanding the pitfalls and adhering to best practices, you can effectively harness its capabilities while keeping your codebase robust and maintainable.
If you are interested in learning more about Java collections and their capabilities, consider exploring the official Java documentation.
With careful consideration and proper usage, mastering computeIfAbsent
will enhance your Java skills and your application's design. Happy coding!