Mastering Word Count with MapReduce in Akka: A Beginner's Guide
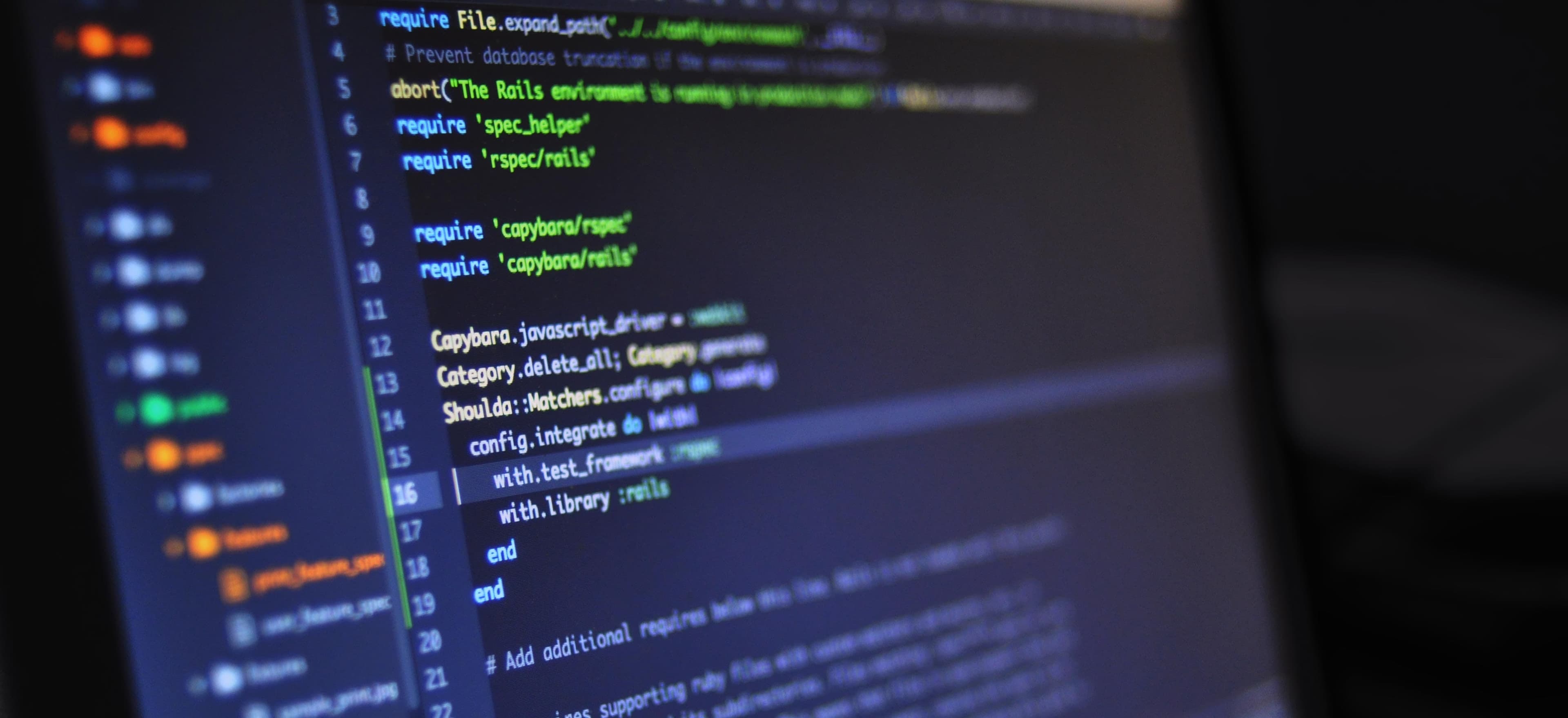
- Published on
Mastering Word Count with MapReduce in Akka: A Beginner's Guide
Word counting is a fundamental exercise in understanding data processing concepts, particularly in the context of distributed computing. In this blog post, we'll explore how to implement the Word Count program using MapReduce principles in Akka, a powerful toolkit for building concurrent applications on the JVM.
What is MapReduce?
MapReduce is a programming model and an associated implementation for processing and generating large data sets. It operates in two phases:
- Map Phase: The input data is divided into small chunks, processed in parallel, and transformed into key-value pairs.
- Reduce Phase: The key-value pairs produced by the map phase are aggregated by key to produce the final count or result.
MapReduce is designed to enable scalability and fault tolerance. In our case, we will leverage Akka's actor model, which excels in handling concurrency by distributing tasks across various actors corresponding to the Map and Reduce phases.
Setting Up Akka
Before diving into the code, you will need to set up an Akka project. Ensure you have the following in your build.sbt
file:
name := "word-count-akka"
version := "0.1"
scalaVersion := "2.13.8"
// Add Akka dependencies
libraryDependencies ++= Seq(
"com.typesafe.akka" %% "akka-actor" % "2.6.16",
"com.typesafe.akka" %% "akka-stream" % "2.6.16",
"com.typesafe.akka" %% "akka-testkit" % "2.6.16" % Test
)
Once you have your environment set, create a main application file. Let us break down our implementation step by step.
Word Count Implementation
Step 1: Defining Messages
In Akka, we define messages that can be sent between actors. In our case, we will implement messages for Start
, WordCount
, and Result
.
case class Start(text: String) // Message to initiate the processing
case class WordCount(word: String) // Message containing a word to count
case class Result(word: String, count: Int) // Result message to store final counts
Step 2: Creating the Map Actor
The Map Actor is responsible for processing the input text, mapping each word to a WordCount
message.
import akka.actor.{Actor, ActorRef, ActorSystem, Props}
class MapActor(reduceActor: ActorRef) extends Actor {
def receive: Receive = {
case Start(text) =>
val words = text.split("\\W+").filter(_.nonEmpty) // Splitting words
words.foreach { word =>
reduceActor ! WordCount(word.toLowerCase) // Send each word to the Reduce Actor
}
}
}
Step 3: Creating the Reduce Actor
The Reduce Actor aggregates word counts. It maintains a mutable map to track occurrences of each word.
import scala.collection.mutable
class ReduceActor extends Actor {
private val wordCounts = mutable.Map[String, Int]().withDefaultValue(0)
def receive: Receive = {
case WordCount(word) =>
wordCounts(word) += 1 // Incrementing the count for the word
case "printResult" =>
// Print the results
println("Word Count Results:")
wordCounts.foreach { case (word, count) =>
println(s"$word: $count")
}
}
}
Step 4: Main Application
Now, let's bring everything together in the main application.
object WordCountApp extends App {
val system = ActorSystem("WordCountSystem")
val reduceActor = system.actorOf(Props[ReduceActor], "reduceActor")
val mapActor = system.actorOf(Props(new MapActor(reduceActor)), "mapActor")
val inputText = "Akka is a toolkit for building concurrent applications on the JVM. Akka helps to build resilient and responsive systems."
mapActor ! Start(inputText) // Start the word counting process
// Allow some time for processing and then request results
Thread.sleep(1000)
reduceActor ! "printResult"
system.terminate() // Terminate the actor system
}
Code Breakdown
Let’s summarize the core principles reflected in our code:
- Actor Model: By using Akka's actor model, we encapsulate the logic for both mapping and reducing within dedicated actors. This promotes separation of concerns and makes the system easier to manage.
- Message Passing: Actors communicate through messages. This decouples the actors and provides a robust means of data sharing within concurrent environments.
- State Management: The Reduce Actor maintains state through a mutable map of word counts. Default values make it easy to initialize counts for new words.
Benefits of Using Akka for Word Count
- Concurrency and Parallelism: Akka’s actors allow you to run the Map and Reduce phases concurrently, significantly speeding up processing for larger datasets.
- Scalability: Akka’s architecture allows your application to scale up easily by adding more actors. Moreover, you can distribute actors across multiple nodes in a cluster.
- Fault Tolerance: It retains resilience in case of individual actor failures, ensuring that your word counting continues uninterrupted.
Wrapping Up
In this guide, we delved into the basics of MapReduce with Akka by implementing a simple yet effective word count application. We studied how to define messages, organize our actors, and leverage the power of Akka to handle concurrency.
For further reading on Akka and its features, consider checking out Akka Documentation.
With this foundational understanding and the example provided, you should feel equipped to explore more complex data processing tasks using Akka. Happy coding!
References
- Akka Basics
- Scala Documentation
- MapReduce Overview
Feel free to reach out in the comments if you have any questions or need further assistance!
Checkout our other articles