Overcoming Common Pitfalls in Salesforce REST API Integration
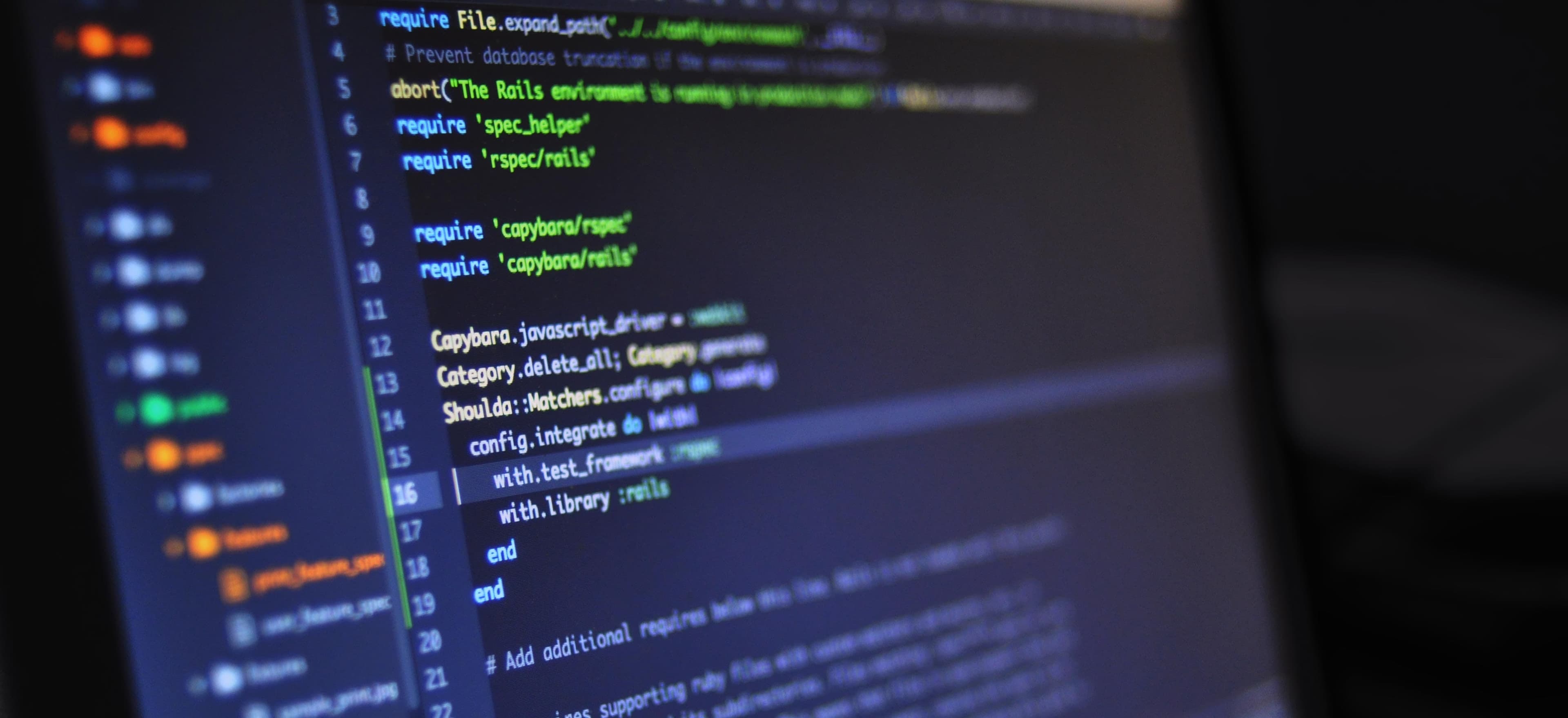
- Published on
Overcoming Common Pitfalls in Salesforce REST API Integration
Salesforce is a leading customer relationship management (CRM) platform that provides a robust REST API, allowing developers to interact programmatically with Salesforce data and applications. However, integrating with the Salesforce REST API can be challenging due to several common pitfalls that developers may encounter. This blog post aims to highlight these pitfalls and provide guidance on how to overcome them effectively.
Understanding Salesforce REST API
Before diving into integration issues, it’s crucial to understand what the Salesforce REST API is and how it works. The Salesforce REST API allows users to perform CRUD (Create, Read, Update, Delete) operations on Salesforce objects using standard HTTP verbs: GET
, POST
, PATCH
, and DELETE
.
This API is JSON-based, making it lightweight and easy to use for interacting with web services. If you want to explore the full API documentation, check out the official Salesforce REST API documentation.
Common Pitfalls in Salesforce REST API Integration
1. Not Handling Authentication Correctly
One of the most critical aspects of interacting with the Salesforce REST API is authentication. Salesforce uses OAuth 2.0 for authentication—specifically, the "Bearer Token" approach. Failing to handle this correctly can lead to unauthorized access errors.
Example Code Snippet
Here's a basic Java example of how to authenticate via OAuth 2.0 using the Salesforce REST API:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.nio.charset.StandardCharsets;
public class SalesforceAuth {
private static final String TOKEN_URL = "https://login.salesforce.com/services/oauth2/token";
private static final String CLIENT_ID = "your_client_id";
private static final String CLIENT_SECRET = "your_client_secret";
private static final String USERNAME = "your_username";
private static final String PASSWORD = "your_password_and_security_token";
public static void main(String[] args) {
try {
String params = String.format("grant_type=password&client_id=%s&client_secret=%s&username=%s&password=%s",
CLIENT_ID, CLIENT_SECRET, USERNAME, PASSWORD);
URL url = new URL(TOKEN_URL);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.getOutputStream().write(params.getBytes(StandardCharsets.UTF_8));
if (conn.getResponseCode() == 200) {
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String responseLine;
StringBuilder response = new StringBuilder();
while ((responseLine = in.readLine()) != null) {
response.append(responseLine);
}
in.close();
// Print the access token
System.out.println("Access Token: " + response.toString());
} else {
System.out.println("Error: " + conn.getResponseCode());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Matters
This code snippet shows how to handle authentication through a password grant. It's essential to replace placeholders with real credentials and understand that security tokens are required for your account. Failing to handle authentication correctly will cause your API calls to fail. The correct approach ensures you have the proper access to Salesforce resources.
2. Inadequate Error Handling
Another pitfall is not implementing robust error handling. The Salesforce REST API provides various response codes, and it is essential for developers to understand them. Ignoring error codes can inhibit the ability to troubleshoot and maintain the code.
Example Code Snippet
Here’s an enhancement of the previous code snippet with error handling:
// Add this method to your existing SalesforceAuth class
private static void handleResponse(HttpURLConnection conn) throws Exception {
int responseCode = conn.getResponseCode();
if (responseCode == 200) {
// Handle success
} else {
// Throw exception with details
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getErrorStream()));
String errorResponse = "";
String responseLine;
while ((responseLine = in.readLine()) != null) {
errorResponse += responseLine;
}
in.close();
throw new Exception("Error: " + responseCode + ", Details: " + errorResponse);
}
}
Why This Matters
This enhancement ensures that if the API call fails, the developer is immediately made aware of what went wrong. It streamlines the debugging process, allowing for quicker resolutions. Without proper error handling, you may face challenges in identifying issues quickly.
3. Exceeding API Limits
Salesforce imposes limits on the number of API calls that can be made in a given time frame. These limits vary depending on the edition of Salesforce you are using. Exceeding these limits results in a 403 (forbidden) status and immediate impact on application functionality.
How to Mitigate
- Batch Requests: Instead of making a large number of individual requests, use batch requests.
// Pseudocode for batch requests
String batchRequestBody = "[{\"method\":\"POST\",\"url\":\"/services/data/vXX.X/sobjects/Account/\",\"richInput\":{...}}, {...}]";
- Monitor Usage: Regularly monitor API usage in Salesforce setup to avoid reaching limits.
4. Ignoring Data Model
Salesforce has a unique data model structure—objects, fields, relationships, and more. Skipping the step of understanding the relationships and business rules can lead to failed integrations.
Recommendations
-
Understand Salesforce Objects: Take the time to map out the necessary objects and how they interact with each other.
-
Utilize SOQL: When retrieving data, use Salesforce Object Query Language (SOQL) to maximize efficiency:
String query = "SELECT Id, Name FROM Account WHERE Industry='Technology'";
5. Not Utilizing the Right Tools
Finally, many developers try to reinvent the wheel instead of using available tools and libraries, leading to increased development time and opportunities for errors.
Recommended Libraries
- Salesforce SDKs: Use SDKs provided by Salesforce for various programming languages.
- Postman: For testing API requests without coding.
- Joogly: A Java library to simplify Salesforce API interactions.
Closing Thoughts
Integrating with the Salesforce REST API can be a powerful method for enhancing your business solutions. By understanding and overcoming common pitfalls, you can streamline your integration processes, maintain effective communication with Salesforce, and ultimately provide better solutions for your organization.
For more deep dives into Salesforce API best practices and nuances, consider exploring Salesforce Developer Blog for ongoing insights and updates.
A Final Look
Navigating the complexities of the Salesforce REST API is crucial for successful integrations. By being aware of common pitfalls—such as authentication mishaps, inadequate error handling, and exceeding limits—you can effectively troubleshoot issues and implement robust solutions. If you're interested in further enhancing your knowledge about API interactions, feel free to check out Salesforce Developer Community for forums and discussions.
Happy coding!
Checkout our other articles