Streamlining Async Transaction Management in Spring 4.2
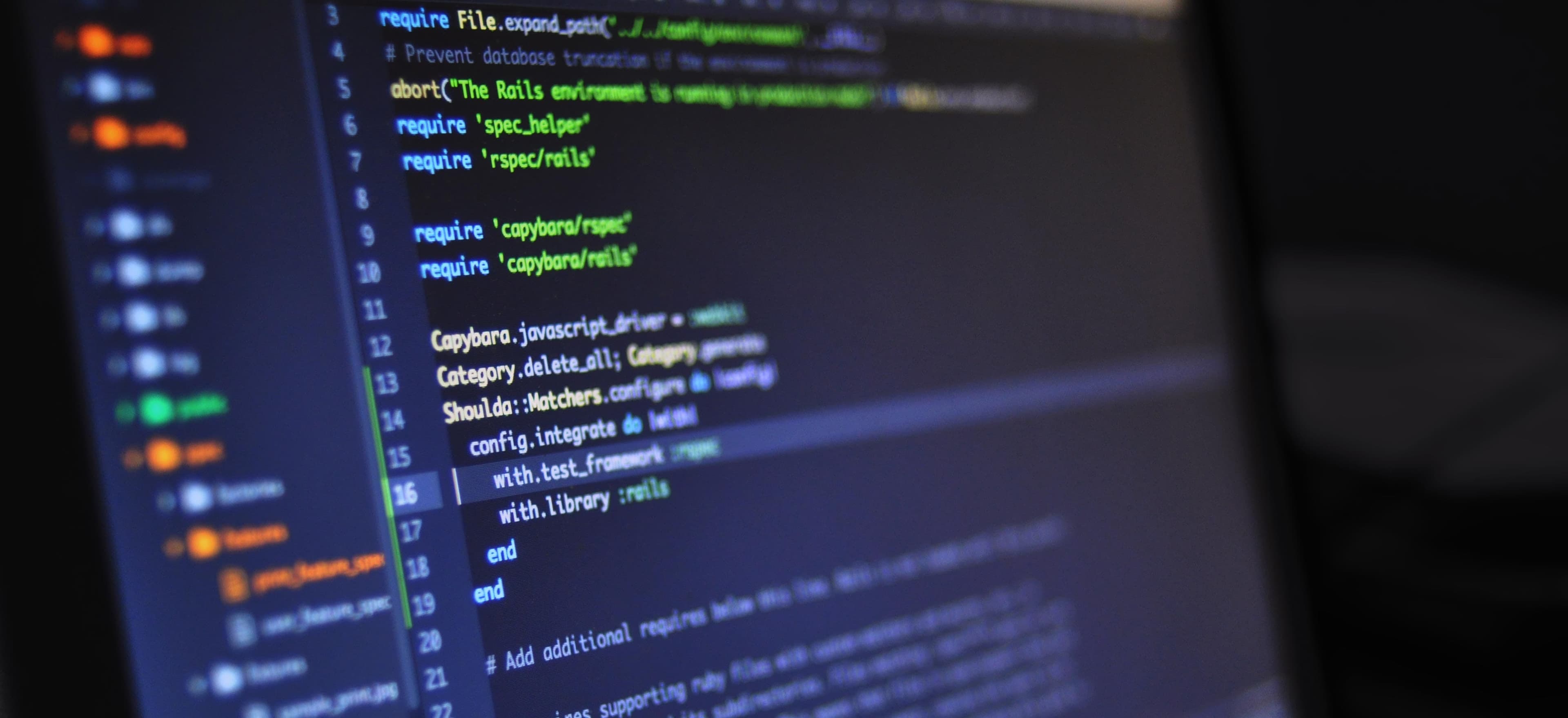
- Published on
Streamlining Async Transaction Management in Spring 4.2
Asynchronous programming has vastly transformed the landscape of software development, enabling applications to perform better by not blocking threads while tasks are executing. If you are working with Spring Framework, especially version 4.2, understanding how to streamline async transaction management is crucial for building efficient and responsive applications.
In this blog post, we will delve into asynchronous transaction management in Spring 4.2, exploring its benefits and providing insightful code snippets illustrating best practices.
Understanding Asynchronous Transactions
In a traditional synchronous process, each instruction is executed one after another. This blocking behavior can lead to performance bottlenecks, especially when I/O operations, such as database calls or service invocations, are involved.
Asynchronous transactions allow these operations to run concurrently, thus freeing up resources and improving throughput. In Spring 4.2, the framework provides excellent support for managing these transactions efficiently.
Why Use Asynchronous Transactions?
- Improved Performance: Asynchronous transactions allow multiple tasks to run in parallel, improving the responsiveness of applications.
- Scalability: By managing threads more effectively, systems can handle more clients without additional overhead.
- Better Resource Utilization: System resources are used more efficiently since threads are released back to the pool while waiting for responses.
Configuring Asynchronous Support in Spring 4.2
To enable asynchronous processing, you need to annotate your configuration class with @EnableAsync
. This tells Spring to look for methods annotated with @Async
.
Step 1: Add Spring's Async Annotation
Here's how you can set it up:
import org.springframework.context.annotation.Configuration;
import org.springframework.scheduling.annotation.EnableAsync;
@Configuration
@EnableAsync
public class AsyncConfig {
}
Why? The @EnableAsync
annotation scans your application for methods that return a Future
, CompletableFuture
, or ListenableFuture
. It subsequently enables asynchronous processing for those methods.
Step 2: Implementing Asynchronous Methods
Once asynchronous processing is set up, you can annotate your service methods with @Async
. Here’s an example of an asynchronous service:
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
@Service
public class AsyncService {
@Async
public void doAsyncWork() {
// Simulate a long-running task
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Asynchronous work completed!");
}
}
Why? The @Async
annotation signals to Spring that this method should be executed in a separate thread, allowing the caller to continue processing without waiting for this method to finish.
Managing Transactions Asynchronously
While asynchronous processing is powerful, managing transactions in an asynchronous context requires careful consideration to ensure data integrity and consistency.
Pro Tip: Use @Transactional
Wisely
When you annotate an asynchronous method with @Transactional
, the transaction should span the method. However, since it’s asynchronous, the transaction may end before the method finishes executing.
Consider this example, contrasting what not to do:
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@Async
@Transactional
public void createUser(User user) {
// This method is not ideal for @Async
repository.save(user);
}
}
Why? Here, the @Transactional
will not behave as expected because the transaction may commit before the asynchronous execution completes. Instead, avoid putting @Transactional
on async methods.
A Better Approach: Transaction Management Outside Async Methods
To manage transactions properly, keep them outside of async methods, like this:
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final AsyncService asyncService;
public UserService(AsyncService asyncService) {
this.asyncService = asyncService;
}
@Transactional
public void createUser(User user) {
repository.save(user);
asyncService.doAsyncWork();
}
}
Why? This pattern ensures that the database operation completes its transaction before beginning an asynchronous task, maintaining data consistency.
Exception Handling in Asynchronous Transactions
Exception handling in an asynchronous context is crucial, as it differs from synchronous execution. Let’s explore this further.
Handling Exceptions with CompletableFuture
Instead of returning void
, you can return a CompletableFuture
. This allows you to handle exceptions more elegantly.
import org.springframework.stereotype.Service;
import java.util.concurrent.CompletableFuture;
@Service
public class AsyncService {
@Async
public CompletableFuture<String> doAsyncWork() {
try {
Thread.sleep(3000);
return CompletableFuture.completedFuture("Asynchronous work completed!");
} catch (InterruptedException e) {
return CompletableFuture.failedFuture(e);
}
}
}
Why? By returning CompletableFuture
, you can chain tasks and manage failure conditions more elegantly, ultimately leading to better resilience in your applications.
Consuming CompletableFuture Results
Here is how you can consume the result of the async method:
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final AsyncService asyncService;
public UserService(AsyncService asyncService) {
this.asyncService = asyncService;
}
@Transactional
public void createUser(User user) {
repository.save(user);
asyncService.doAsyncWork().thenAccept(result -> {
System.out.println(result);
}).exceptionally(ex -> {
System.err.println("Error: " + ex.getMessage());
return null;
});
}
}
Why? Returning a CompletableFuture
not only allows us to react to the result of async processing, but also gives us a way to handle exceptions in a way that's easy to understand.
The Last Word
Spring 4.2 has made significant strides in asynchronous processing and transaction management, allowing developers to build high-performance applications with ease. By leveraging @Async
annotations thoughtfully and managing @Transactional
execution outside async methods, we can ensure data integrity, improve application responsiveness, and handle exceptions gracefully.
Further information can be found in the official Spring documentation on Asynchronous Processing and Transaction Management.
In this post, we simplified complex concepts to facilitate a better understanding of async transaction management within Spring 4.2. Apply these best practices and enrich your skills in developing scalable and responsive applications with Spring!
Checkout our other articles