Optimizing JPA Performance on Glassfish: Key Strategies
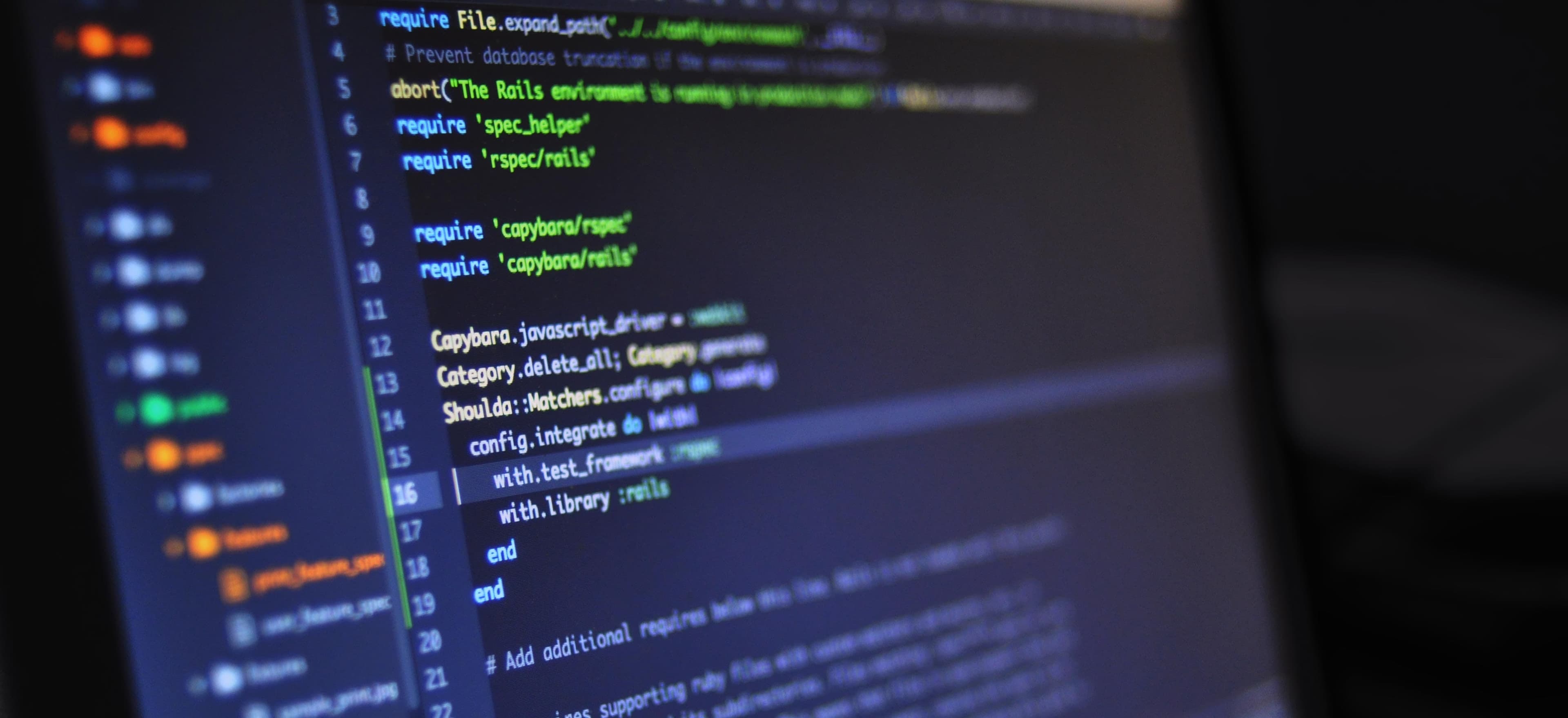
- Published on
Optimizing JPA Performance on Glassfish: Key Strategies
Java Persistence API (JPA) is an essential framework used for data persistence in Java applications. Glassfish, as a powerful application server, pairs well with JPA, but performance optimizations can make a significant difference in the efficiency and responsiveness of your applications. This blog post will guide you through various strategies to optimize JPA performance on Glassfish.
Understanding JPA and Glassfish
At its core, JPA is designed to simplify the management of relational data in Java applications through object-relational mapping (ORM). Glassfish serves as an application server that supports JPA, allowing you to deploy and manage Java EE applications efficiently. By harnessing both, developers can build robust enterprise applications but may face performance bottlenecks without proper optimization.
Key Strategies to Optimize JPA Performance
- Connection Pooling
- Entity Management
- Batch Processing
- Lazy vs. Eager Loading
- Caching
- Query Optimization
Let’s dive into each strategy in detail.
1. Connection Pooling
Connection pooling plays a vital role in improving the performance of JPA applications. In Glassfish, you can configure the connection pool efficiently through the administration console.
Why Use Connection Pooling?
Creating and closing database connections are time-consuming operations. By using a connection pool, you can reuse existing connections, significantly reducing the overhead.
Example Configuration:
To set up connection pooling in Glassfish, follow these steps:
- Log in to the Glassfish administration console.
- Navigate to Resources > JDBC > JDBC Connection Pools.
- Create a new pool or edit an existing one. An example of a standard JDBC connection pool configuration can look like this:
<jdbc-connection-pool name="MyPool" resource-type="javax.sql.DataSource" datasource-classname="org.postgresql.ds.PGSimpleDataSource">
<property name="user" value="your_username"/>
<property name="password" value="your_password"/>
<property name="databaseName" value="my_database"/>
<property name="serverName" value="localhost"/>
<property name="portNumber" value="5432"/>
<property name="connectionPoolSize" value="32"/>
</jdbc-connection-pool>
This pool configuration ensures maximum availability and performance by adjusting the connection pool size according to your application needs.
2. Entity Management
Managing entities efficiently is crucial when working with JPA. Use the EntityManager wisely to control the lifecycle of entities.
Why Proper Entity Management?
Improper management can lead to memory leaks and performance degradation due to unintended persistence contexts.
Example Usage:
Here’s how to correctly utilize EntityManager:
@PersistenceContext
private EntityManager entityManager;
public void manageEntity(Long id) {
MyEntity entity = entityManager.find(MyEntity.class, id);
// Perform operations
entityManager.merge(entity);
}
In this example, invoking find
directly accesses the current persistence context, allowing efficient retrieval without incurring the cost of multiple database queries.
3. Batch Processing
Batch processing allows you to manage multiple entity operations as a single batch, significantly boosting performance for bulk operations.
Why Use Batch Processing?
It minimizes the number of database round trips, improving overall performance when dealing with batch inserts or updates.
Example Implementation:
You can configure batch processing in your persistence.xml:
<persistence-unit name="my-pu">
<properties>
<property name="hibernate.jdbc.batch_size" value="20"/>
</properties>
</persistence-unit>
Then, utilize it in your DAO class:
public void saveEntities(List<MyEntity> entities) {
int batchSize = 20;
for (int i = 0; i < entities.size(); i++) {
entityManager.persist(entities.get(i));
if (i % batchSize == 0) {
entityManager.flush();
entityManager.clear();
}
}
}
This method ensures that once flush()
is called, the changes are committed in batches, enhancing the application's throughput.
4. Lazy vs. Eager Loading
The choice between lazy and eager loading can considerably impact performance. Lazy loading retrieves data only when it's needed, while eager loading fetches all related data upfront.
Why Choose the Right Loading Strategy?
Using eager loading indiscriminately can lead to massive data retrieval, potentially overwhelming memory resources and degrading performance.
Example of Lazy Loading:
@Entity
public class Author {
@OneToMany(mappedBy = "author", fetch = FetchType.LAZY)
private List<Book> books;
}
In this scenario, using FetchType.LAZY
ensures that the books
list is only populated when accessed, not during the initial query.
5. Caching
Effective caching can speed up data retrieval times significantly. JPA providers like Hibernate support both first-level and second-level caching.
Why Implement Caching?
Caching can avoid repeated data access from the database, leading to improved application response times.
Example Configuration for Second-Level Caching:
To enable second-level caching with Hibernate, update your persistence.xml
:
<persistence-unit name="my-pu">
<properties>
<property name="hibernate.cache.use_second_level_cache" value="true"/>
<property name="hibernate.cache.region.factory_class" value="org.hibernate.cache.ehcache.EhCacheRegionFactory"/>
</properties>
</persistence-unit>
Implementing caching needs careful memory management, but when done right, it can drastically enhance application scalability.
6. Query Optimization
Optimizing your queries will yield significant improvements in performance. Avoid fetching unnecessary data and use proper indexing strategies in your database.
Why Optimize Queries?
Efficient queries lead to reduced load times and better user experiences.
Example of a Native Query:
public List<MyEntity> findEntities() {
return entityManager.createNativeQuery("SELECT * FROM MyEntity WHERE status = :status", MyEntity.class)
.setParameter("status", "active")
.getResultList();
}
Using native queries can sometimes be more performant than JPQL, especially for complex queries or when dealing with a large dataset.
Final Thoughts
Optimizing JPA performance on Glassfish is a multi-faceted endeavor. From implementing connection pooling and batch processing to managing entity lifecycles and leveraging caching, each strategy contributes to a robust, efficient application.
By applying these methods, you will create a faster, more responsive JPA-based application. For further reading, consider checking out the official JPA documentation and Hibernate performance tuning guidelines.
Implementing one or more of the strategies discussed in this post can yield significant performance improvements in your Glassfish applications, making you a hero in the eyes of your users!