Mastering gRPC Retry Strategies for Java Applications
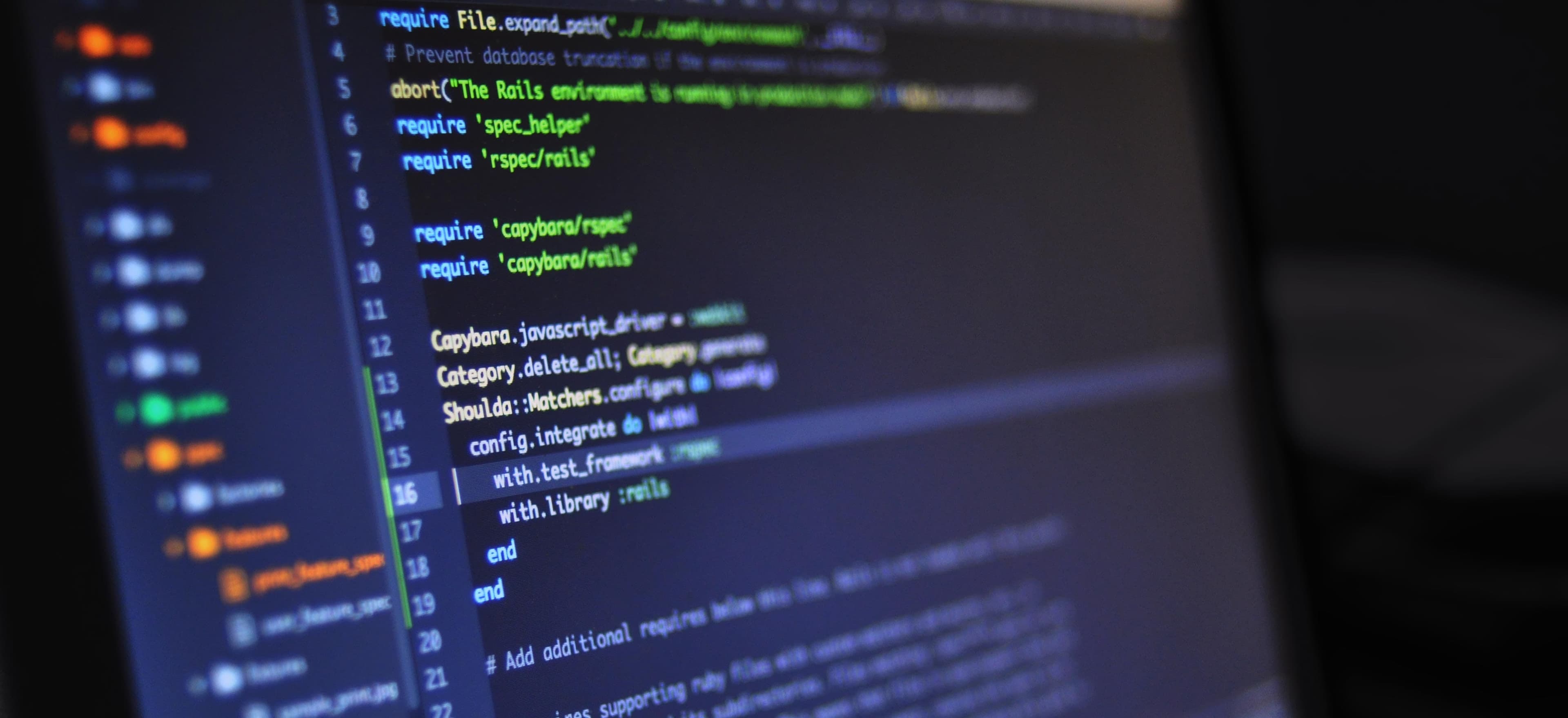
- Published on
Mastering gRPC Retry Strategies for Java Applications
gRPC is a powerful framework for building remote procedure calls (RPCs) that facilitate communication between distributed systems. However, in any networked environment, failures are inevitable. Hence, understanding how to implement proper retry strategies is crucial for ensuring the reliability of your Java applications. This blog post will delve into mastering gRPC retry strategies, demonstrating how developers can handle transient failures effectively.
What is gRPC?
Before delving into retry strategies, let's review what gRPC is. gRPC, a high-performance remote procedure call (RPC) framework developed by Google, uses HTTP/2 for transport, Protocol Buffers as the interface description language, and enables bidirectional streaming and communication.
Key Features of gRPC
- Language-agnostic: gRPC allows developers to create services and clients in any programming language.
- Streaming: Support for unary, server-side streaming, client-side streaming, and bidirectional streaming.
- HTTP/2: Provides benefits like multiplexing, flow control, and header compression.
- Error handling: Allows for comprehensive error codes to handle various error scenarios.
Understanding Retry Strategies
A retry strategy dictates how applications respond to failures when calling remote services. In the context of gRPC, retry policies help mitigate issues such as network connectivity problems or service downtime. Implementing an effective retry strategy can improve the user experience by enhancing the perceived reliability of an application.
The Importance of Backoff
Introducing backoff is critical in a retry strategy. Backoff refers to the delayed wait time between retries. Instead of immediately retrying after a failure, the application pauses for a predetermined time interval. This method prevents overwhelming a service during high-load scenarios and gracefully reduces the chances of continuing failures.
Exponential Backoff
Exponential backoff is a strategy where the wait time increases exponentially with each failed attempt. For example, if the time between the first and second attempts is 1 second, the second and third would add up to 2 seconds, then 4 seconds, and so forth.
Implementing Retry Strategies in Java with gRPC
To implement a gRPC retry strategy in your Java application, you can use the built-in gRPC features and Java's ExecutorService
for asynchronous execution and retries. Below is an example code snippet demonstrating how to create a simple gRPC client that implements retry logic.
Step-by-step Implementation
1. Add Dependencies
Make sure you have the required dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty</artifactId>
<version>1.49.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-protobuf</artifactId>
<version>1.49.0</version>
</dependency>
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-stub</artifactId>
<version>1.49.0</version>
</dependency>
2. Define your Service
Define a simple gRPC service in a .proto
file.
syntax = "proto3";
package example;
service Greeter {
rpc SayHello(HelloRequest) returns (HelloReply) {}
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
3. Generate Java code
Use the Protocol Buffers compiler to generate the Java code using the command below:
protoc --java_out=src/main/java --grpc_out=src/main/java --plugin=protoc-gen-grpc=grpc_java_plugin_name your_file.proto
4. Implement Client with Retry Logic
The following code demonstrates a simple gRPC client with a retry mechanism using exponential backoff:
import io.grpc.ManagedChannel;
import io.grpc.ManagedChannelBuilder;
import io.grpc.StatusRuntimeException;
import example.GreeterGrpc;
import example.HelloReply;
import example.HelloRequest;
import java.util.concurrent.TimeUnit;
public class GrpcClient {
private final GreeterGrpc.GreeterBlockingStub blockingStub;
private static final int MAX_RETRIES = 5;
public GrpcClient(ManagedChannel channel) {
this.blockingStub = GreeterGrpc.newBlockingStub(channel);
}
public String greet(String name) {
HelloRequest request = HelloRequest.newBuilder().setName(name).build();
int attempts = 0;
while (attempts < MAX_RETRIES) {
try {
HelloReply response = blockingStub.sayHello(request);
return response.getMessage();
} catch (StatusRuntimeException e) {
attempts++;
System.err.println("RPC failed: " + e.getStatus());
if (attempts < MAX_RETRIES) {
long waitTime = (long) Math.pow(2, attempts); // Exponential backoff
try {
System.out.println("Retrying in " + waitTime + " seconds...");
TimeUnit.SECONDS.sleep(waitTime);
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
} else {
throw new RuntimeException("Failed after " + attempts + " attempts");
}
}
}
return null; // This line should theoretically never hit
}
public static void main(String[] args) {
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 50051)
.usePlaintext()
.build();
try {
GrpcClient client = new GrpcClient(channel);
String response = client.greet("World");
System.out.println(response);
} finally {
channel.shutdown();
}
}
}
Commentary on the Code
In the greet
method, when a remote call fails, the application catches the exception and incrementally retries, introducing an exponential backoff after each failure.
-
Exponential Backoff Logic: This strategy is implemented using
Math.pow(2, attempts)
, whereattempts
tracks the number of failed retries. -
Maximum Retry Limit: A maximum of 5 retries prevents the application from entering an infinite loop of retries.
-
Thread Management: The
TimeUnit.SECONDS.sleep(waitTime)
is essential to pause the execution of the thread for the calculated backoff duration.
The Closing Argument
Implementing a robust retry strategy in your gRPC Java applications enhances reliability, reduces downtime, and improves overall user experience. By utilizing exponential backoff with a controlled number of retries, you ensure your application remains responsive even in the event of transient failures.
For further reading on best practices for gRPC, check out the gRPC documentation. Understanding these concepts forms a solid foundation for creating resilient applications. Happy coding!