Maximizing Callable Efficiency in Java Concurrency
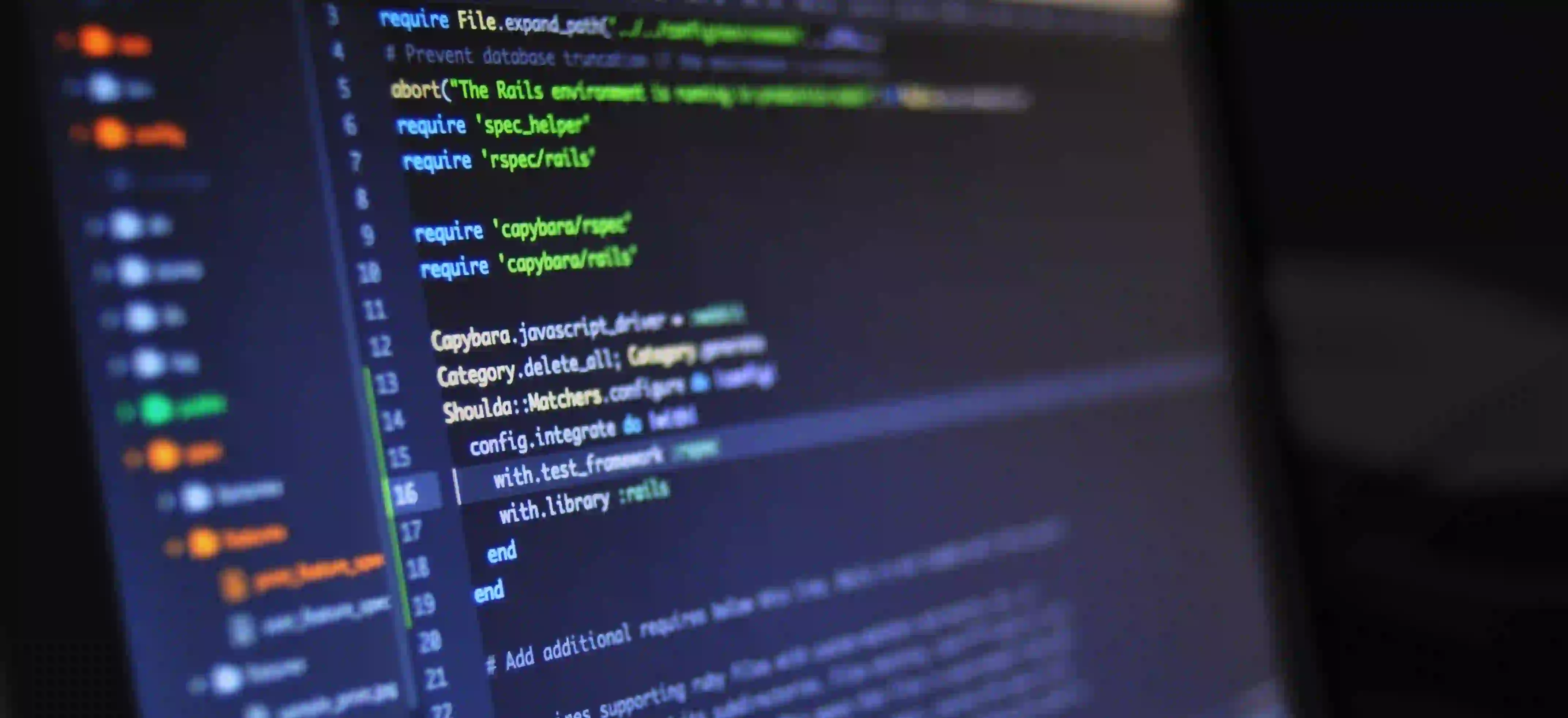
Maximizing Callable Efficiency in Java Concurrency
Concurrency has become a cornerstone technique for optimizing application performance, especially when executing multiple tasks simultaneously. Java provides powerful concurrency utilities to help developers improve the efficiency of their applications. Among these utilities, the Callable
interface stands out as a robust alternative to Runnable
, enabling tasks to return results and throw exceptions. This blog post delves into maximizing the efficiency of Callable
implementations in Java, demonstrating best practices and providing actionable examples.
Understanding the Callable Interface
The Callable
interface, part of the java.util.concurrent
package, is similar to Runnable
, but it can return values and throw checked exceptions. Here is a simple implementation:
import java.util.concurrent.Callable;
public class SimpleCallable implements Callable<String> {
@Override
public String call() throws Exception {
return "Callable task completed.";
}
}
In contrast to Runnable
, the call
method allows you to retrieve the result of the computation. This feature makes Callable
particularly useful in scenarios where a task's outcome is significant.
Key Advantages of Callable
- Return Values:
Callable
can return a result whileRunnable
cannot. - Exception Handling: It can throw checked exceptions, providing better error handling.
- Integration with Executor Framework: Callable works seamlessly with the Executor framework, making it easier to execute tasks concurrently.
Setting Up ExecutorService
To maximize the efficiency of Callable
, it is essential to utilize the ExecutorService
. This framework allows you to manage a pool of threads, offering a straightforward way to run concurrent tasks.
Here’s how to create an ExecutorService
and submit a Callable
task:
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class ExecutorServiceExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
Callable<String> task = new SimpleCallable();
Future<String> future = executor.submit(task);
try {
String result = future.get(); // Blocking call until the result is available
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
} finally {
executor.shutdown();
}
}
}
Why Use ExecutorService?
- Thread Management: It simplifies thread management by providing the ability to create thread pools.
- Task Scheduling: It supports scheduling tasks to run after a given delay or periodically.
- Resource Optimization: By reusing threads, it reduces the overhead of thread creation.
Best Practices for Callable Efficiency
1. Limit Task Duration
Long-running tasks can block the pool, making other tasks wait unnecessarily. Here's an example of a task that calculates Fibonacci numbers:
public class FibonacciCallable implements Callable<Long> {
private final int number;
public FibonacciCallable(int number) {
this.number = number;
}
@Override
public Long call() {
return fibonacci(number);
}
private Long fibonacci(int n) {
if (n < 2) return (long) n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
}
When submitting multiple FibonacciCallable
tasks, consider enforcing a timeout via Future.get(timeout, TimeUnit)
to avoid blocking indefinitely.
2. Batch Processing
When dealing with numerous tasks, consider batching them. This reduces context-switching and optimizes resource usage. Instead of submitting tasks one by one, use a list:
import java.util.ArrayList;
import java.util.List;
ExecutorService executor = Executors.newFixedThreadPool(5);
List<Callable<Long>> callables = new ArrayList<>();
for (int i = 0; i < 10; i++) {
callables.add(new FibonacciCallable(i));
}
try {
List<Future<Long>> results = executor.invokeAll(callables);
for (Future<Long> result : results) {
System.out.println(result.get());
}
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
} finally {
executor.shutdown();
}
3. Avoid State Dependencies
Ensure that Callable
implementations do not depend on shared mutable state. This reduces the risk of concurrency issues. If shared state is necessary, use synchronization or concurrent collections.
Example: Using ConcurrentHashMap
:
import java.util.concurrent.ConcurrentHashMap;
public class StateAccessCallable implements Callable<Void> {
private final ConcurrentHashMap<String, Integer> map;
public StateAccessCallable(ConcurrentHashMap<String, Integer> map) {
this.map = map;
}
@Override
public Void call() {
map.put(Thread.currentThread().getName(), (int) (Math.random() * 100));
return null;
}
}
4. Handle Exceptions Properly
Handling exceptions gracefully enhances the application’s resilience. If a Callable
task throws an exception, it can be extracted from the Future
:
Callable<String> faultyTask = new Callable<String>() {
@Override
public String call() throws Exception {
throw new Exception("Task failed");
}
};
Future<String> future = executor.submit(faultyTask);
try {
String result = future.get();
} catch (ExecutionException e) {
System.err.println("Error occurred: " + e.getCause());
} finally {
executor.shutdown();
}
Lessons Learned
Maximizing the efficiency of Callable
in Java concurrency comes down to implementing practical strategies such as limiting task durations, using batch processing, avoiding state dependencies, and properly handling exceptions. By utilizing these practices along with the power of the ExecutorService
, you can achieve more efficient and scalable applications.
To delve deeper into Java concurrency, check out Java Concurrency in Practice for an extensive resource on managing concurrency in Java. Additionally, Oracle's Java Documentation provides detailed insights and examples on Java's concurrency framework.
Embrace concurrency, and watch your applications thrive!