Mastering Spock: Overcoming Output Issues in Testing
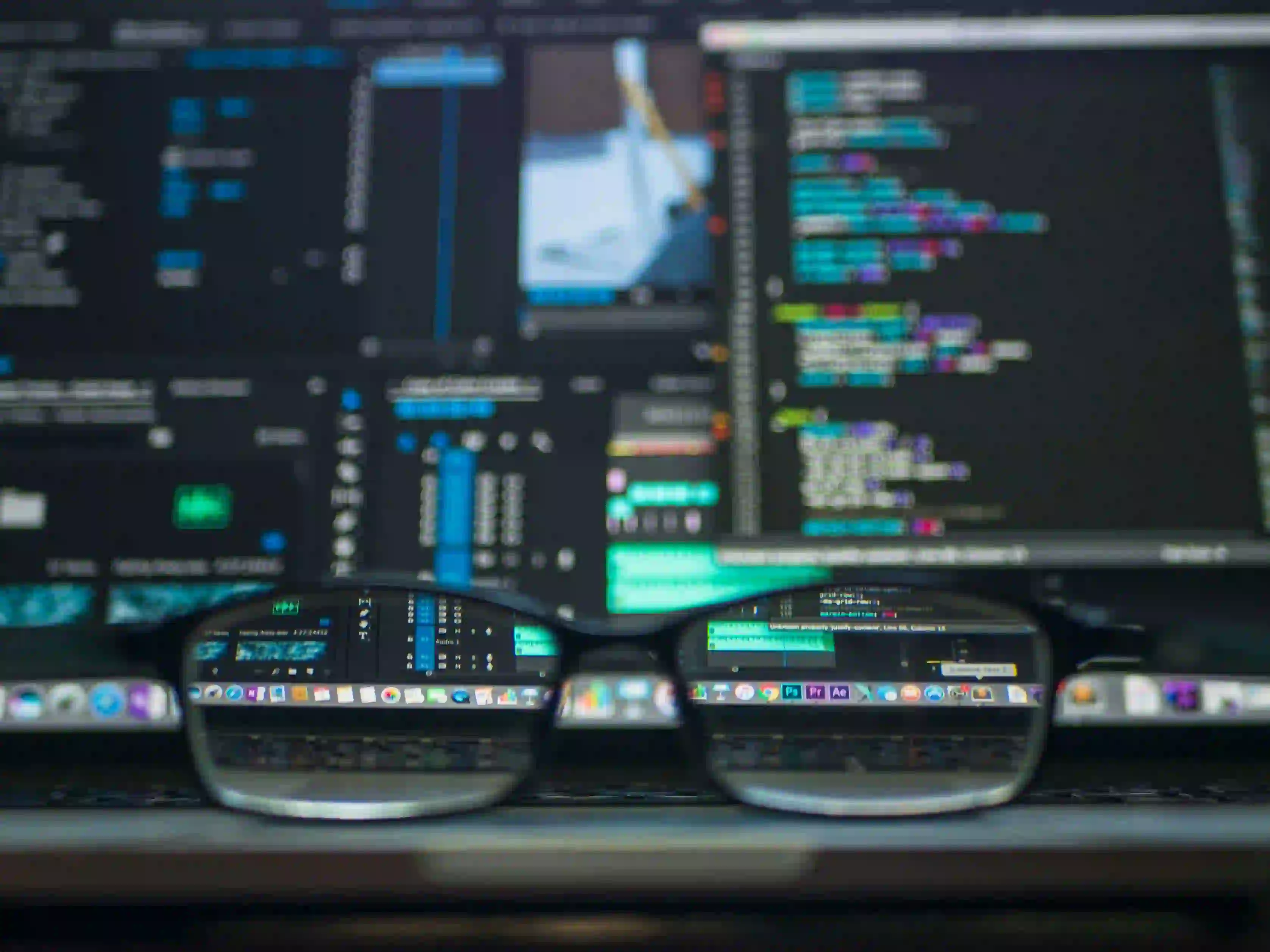
Mastering Spock: Overcoming Output Issues in Testing
Testing is an integral part of software development, ensuring that the application meets intended functionality and performance standards. In the Java ecosystem, Spock is widely recognized for its expressive and readable syntax, making testing easier and more enjoyable. However, output issues can occasionally arise during testing, leading to confusion and errors in understanding test results. In this blog post, we will delve into common output issues in Spock, explore potential solutions, and highlight best practices for effective testing.
What is Spock?
Spock is a powerful testing framework for Java and Groovy applications. It is particularly popular among developers for its capabilities in unit testing and behavior-driven development (BDD). Spock provides a concise syntax that allows for clear structuring of test cases, assertions, and expectations.
Spock offers many features, including:
- Data-driven testing: Easily run the same test with different sets of input data.
- Readable syntax: Uses BDD—a natural language style of defining tests that is easy to understand.
- Integration: Works well with existing testing frameworks like JUnit.
For more information on Spock, you can check out the Spock Framework Documentation.
Common Output Issues in Spock
While Spock excels at structuring tests, several output issues may arise during testing:
- Misleading Assertions: Assertions can sometimes yield results that are not immediately clear, especially in complex scenarios.
- Verbose Output: Spock’s output can become cluttered, leading to difficulty in identifying relevant results.
- Ignoring Test Failure Context: In some cases, if multiple tests fail, it becomes challenging to pinpoint the nature of each failure.
Let’s tackle each of these issues and provide effective strategies to overcome them.
1. Misleading Assertions
Misleading assertions occur when the test output does not clearly indicate why a test has failed. This often leads to confusion, especially for developers unfamiliar with the codebase.
Example of a Misleading Assertion
class Calculator {
int add(int a, int b) {
return a + b
}
}
class CalculatorSpec extends Specification {
def "test addition"() {
expect:
new Calculator().add(2, 3) == 6 // This assertion is misleading
}
}
In this example, the assertion is clearly incorrect, but without meaningful output, it may take more time to identify the issue.
Solution
You can use descriptive assertions to provide clarity in your tests. Update the assertion to give context about the expected and actual results.
expect:
def result = new Calculator().add(2, 3)
result == 5 // More clear assertion with an appropriate expectation
By crafting clear expectations, the failure message will provide immediate context, allowing easier debugging.
2. Verbose Output
When running multiple tests, verbose outputs can clutter the console or CI pipeline. This issue can especially frustrate developers trying to sift through logs to identify failed tests.
Solution
Configure Spock to produce concise output. You can modify the logging and reporting configurations in your build.gradle
file.
test {
useJUnitPlatform()
testLogging {
events "PASSED", "FAILED"
exceptionFormat "short" // Use 'full' for more details
}
}
By limiting the output to only failed tests, you can maintain focus on the crucial components while reducing clutter in your logging.
3. Ignoring Test Failure Context
Failing to provide adequate context around a test failure can lead to slow diagnosis processes, especially with a large test suite.
Example of Ignoring Context
class StringUtilitySpec extends Specification {
def "concatenate strings"() {
expect:
StringUtility.concatenate("foo", "bar") == "foobaz" // Missing context on failure
}
}
Here, simply conveying the failed assertion does little to aid in understanding.
Solution
To provide detailed context, include descriptive messages in your assertions.
expect:
StringUtility.concatenate("foo", "bar") == "foobar" : "Expected 'foobar', but got '${StringUtility.concatenate("foo", "bar")}'"
This additional message gives clarity about the expected output when a failure occurs and facilitates faster debugging.
Best Practices for Effective Testing with Spock
Here are several best practices to keep in mind while using Spock to ensure that you can tackle output issues efficiently:
-
Use Clear Descriptions: Always provide clear descriptions for your test cases. This will help your fellow developers understand the purpose of each test without needing to review the implementation details.
-
Implement Tags: Utilize tags to group related tests. This enables you to run subsets of tests based on specific criteria, making it easier to manage test execution and isolation.
-
Adopt a Consistent Style: Stick to a consistent coding style throughout your test cases. This includes naming conventions, indentation, and structuring of code. It not only enhances readability but also makes maintenance a breeze.
-
Leverage Data Tables for Parameterized Tests: This can drastically reduce repetitive code. Here’s how you can simplify multiple assertions using data tables:
def "test addition with multiple data sets"() {
expect:
new Calculator().add(a, b) == expected
where:
a | b || expected
1 | 2 || 3
2 | 3 || 5
}
This structure neatly lays out various inputs and expected outcomes, improving clarity.
The Last Word
Spock is a remarkable tool for Java and Groovy developers to write expressive and maintainable tests. By understanding and addressing common output issues, you can enhance the effectiveness and clarity of your testing practices.
Always remember to craft clear assertions, manage verbosity appropriately, and embed contextual messages. By following the aforementioned best practices, you will master Spock and overcome output issues effectively—leading to a more thoughtful and efficient testing process.
If you want to dive deeper into improving your overall testing strategies, consider reading this insightful article on Effective Unit Testing by Martin Fowler.
Happy testing!