Mastering WebdriverIO: Overcoming Selenium Locator Challenges
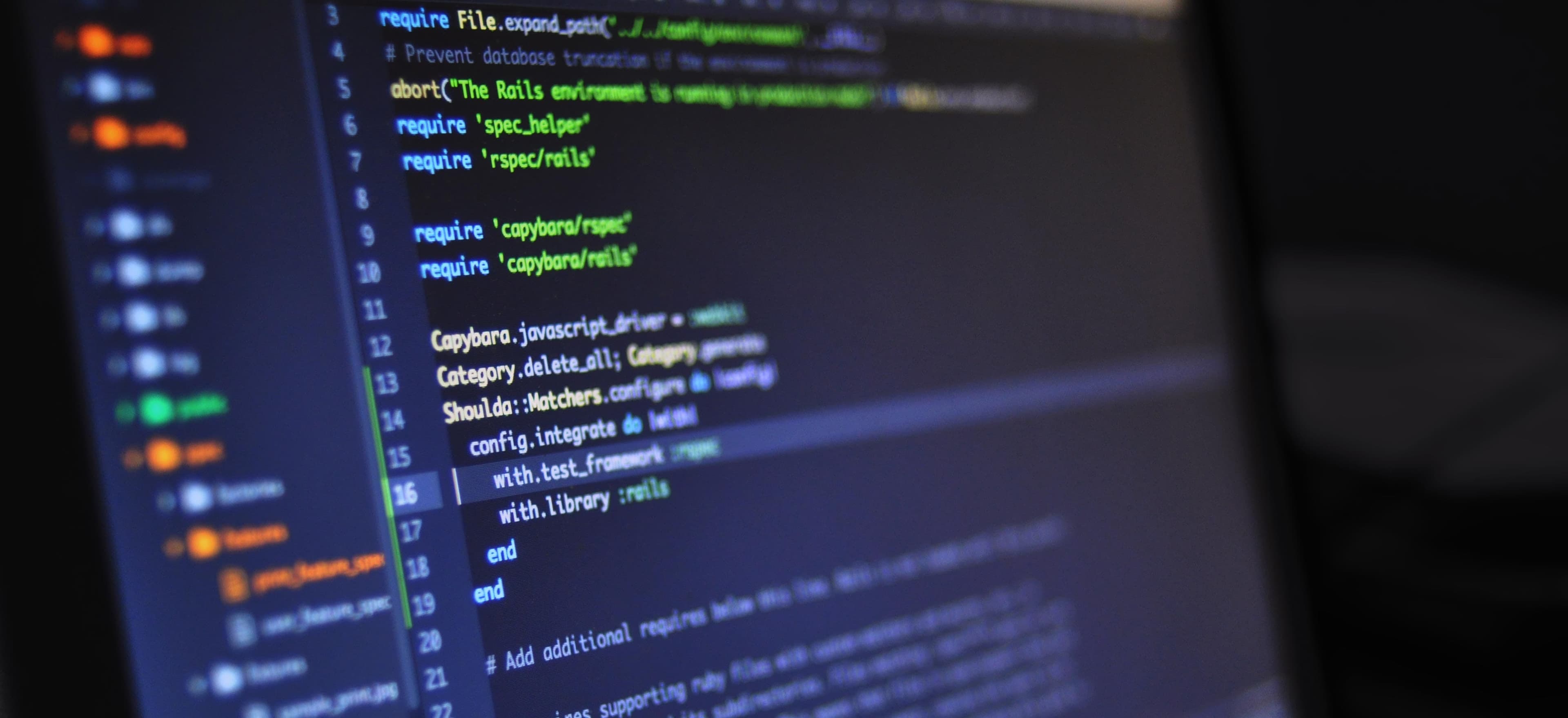
- Published on
Mastering WebdriverIO: Overcoming Selenium Locator Challenges
In the realm of web automation and testing, locators are crucial. They help to identify and interact with web elements in your testing framework, and when dealing with UI testing, the ability to locate elements effectively can make or break your testing success. WebdriverIO has emerged as a powerful tool that streamlines the process, especially for those looking to overcome common Selenium locator challenges.
In this post, we will explore WebdriverIO, its features, and how to effectively utilize locators to resolve issues typically faced in Selenium. We will also share example code snippets for context.
What is WebdriverIO?
WebdriverIO is a custom implementation of Selenium's WebDriver API. It allows you to perform browser automation with a more modern and user-friendly syntax. Unlike other solutions, WebdriverIO often leads to cleaner, more readable code, which facilitates easier collaboration within teams.
Here is the official documentation for those wanting to dive deeper into the setup and foundational concepts.
Key Features of WebdriverIO
- Simplicity: WebdriverIO exposes a powerful API that makes it easier to interact with web elements.
- Support for Async/Await: This modern syntax allows for cleaner and more manageable code.
- Integration with Frameworks: WebdriverIO seamlessly integrates with frameworks like Mocha, Jasmine, and Cucumber.
- Rich Ecosystem: With plugins for visual regression, reporting, and more, you can extend the capabilities of your tests as needed.
The Locator Challenge in Selenium
When using Selenium, you might encounter challenges with locators. Let’s explore some common issues:
- Dynamic Elements: Elements whose properties change or are generated dynamically can be unstable.
- Complex Hierarchies: Elements that are deeply nested may require complex path expressions.
- Performance: Locating elements inefficiently can lead to slow test execution.
WebdriverIO’s Approach to Locators
WebdriverIO offers multiple strategies for locating elements, including:
- CSS Selectors
- XPath
- ID and Name attributes
- Class Name
- Link Text
Let’s delve into each, providing examples to illustrate their efficacy.
CSS Selectors
CSS Selectors are often the first choice due to their speed and flexibility. WebdriverIO supports complex CSS for deeper traversals.
const button = await $('button.submit');
await button.click();
Why: The use of $('button.submit')
is efficient and straightforward. It captures the first button element with the class submit
.
For a nested selector:
const nestedElement = await $('.container .child.active');
await nestedElement.click();
Why: This CSS selector captures .child
within .container
, which is essential when elements are deeply nested.
XPath
XPath is a powerful option, especially when dealing with dynamic content. It allows for location based on the relationship between elements.
const element = await $('//div[@id="main"]//a[text()="Learn More"]');
await element.click();
Why: This XPath query clearly identifies an anchor within a specific div, which is useful for precision.
ID and Name Attributes
These attributes are unique and should be used whenever possible to ensure quick locators.
const usernameInput = await $('#username');
await usernameInput.setValue('testUser');
Why: This uses the ID, a unique locator that minimizes selection time.
Class Name
In scenarios where elements may share the same ID, using class names can be effective, but it can also lead to ambiguities.
const button = await $('.btn');
await button.click();
Why: This approach will find the first button with class btn
. If you need to select multiple buttons, you might want to refine your locator.
Link Text
Directly linking to text can be beneficial, particularly for links.
const link = await await $('=Sign Up');
await link.click();
Why: Using the exact text makes the locator clear and efficient. This is particularly useful for dynamically generated links.
Best Practices for Locating Elements
-
Keep It Simple: While complex locators can work, they can be fragile. Keep locators as simple as possible.
-
Avoid Hard-Coded Paths: When possible, avoid complex paths relying on DOM structure that could change.
-
Monitor Element Visibility: Check if elements are visible before trying to interact with them using
isDisplayed()
. -
Consistency: Maintain consistency in the locators used across your codebase to enhance maintainability.
Handling Dynamic Elements
Dealing with dynamic elements can be particularly daunting. WebdriverIO provides wait commands which can be used to address this.
const dynamicElement = await $('.dynamic');
await dynamicElement.waitForDisplayed({ timeout: 5000 }); // Wait until it is displayed
await dynamicElement.click();
Why: This ensures that your script waits for the element to appear before interaction, reducing flaky tests.
Tips for Performance Optimization
-
Use Efficient Locators: As shown, prefer quick locators such as IDs whenever possible.
-
Reduce Reliance on XPath: Although XPath can offer powerful selection capabilities, it can sometimes be slower than CSS Selectors.
-
Limit Wait Times: Adjust the implicit wait time to cater to your application's loading behavior but keep the values reasonable to avoid longer-than-necessary test times.
Closing Remarks
WebdriverIO stands out in simplifying the locator challenges often faced while using Selenium. Its robust set of features and support for modern JavaScript syntax make it a compelling choice for web automation tests.
By applying best practices such as leveraging simple locators, optimizing performance, and understanding the intricacies of dynamic elements, you can enhance your automation scripts, making them more readable and reliable.
If you're interested in expanding your knowledge about WebdriverIO, check out this comprehensive guide on the WebdriverIO API documentation.
Embrace the power of WebdriverIO and make your test automation journey smoother and more efficient today! Happy Testing!